第一章.Math类
1.Math类介绍
1.概述:数学工具类
2.作用:做数学运算
3.特点:
a.构造私有
b.方法都是静态的
4.使用:
类名直接调用
2.Math类方法
static int abs(int a) -> 求参数的绝对值
static double ceil(double a) -> 向上取整
static double floor(double a) ->向下取整
static long round(double a) -> 四舍五入
static int max(int a, int b) ->求两个数之间的较大值
static int min(int a, int b) ->求两个数之间的较小值
public static void main(String[] args) {
//static int abs(int a) -> 求参数的绝对值
System.out.println(Math.abs(-1));
//static double ceil(double a) -> 向上取整
System.out.println(Math.ceil(3.4));
System.out.println(Math.ceil(-3.4));
//static double floor(double a) ->向下取整
System.out.println(Math.floor(3.4));
System.out.println(Math.floor(-3.4));
//static long round(double a) -> 四舍五入 -> 参数先加0.5,再向下取整
System.out.println(Math.round(2.5));
System.out.println(Math.round(-2.4));
System.out.println(Math.round(-1.8));
//static int max(int a, int b) ->求两个数之间的较大值
System.out.println(Math.max(10,20));
//static int min(int a, int b) ->求两个数之间的较小值
System.out.println(Math.min(10,20));
}
第二章.BigInteger
1.BigInteger介绍
1.描述:将来我们肯定会操作超大的整数,这个整数已经超出了int以及long的范围了,我们跟这样的整数叫做"对象"
2.作用:处理超大整数的
2.BigInteger使用
1.构造:
BigInteger(String val)-> val必须是数字格式
2.方法:
BigInteger add(BigInteger val) 返回其值为 (this + val) 的 BigInteger
BigInteger subtract(BigInteger val) 返回其值为 (this - val) 的 BigInteger
BigInteger multiply(BigInteger val) 返回其值为 (this * val) 的 BigInteger
BigInteger divide(BigInteger val) 返回其值为 (this / val) 的 BigInteger
public static void main(String[] args) {
BigInteger b1 = new BigInteger("1212121212121212121212121212121212121212121212112121");
BigInteger b2 = new BigInteger("1212121212121212121212121212121212121212121212112121");
//BigInteger add(BigInteger val) 返回其值为 (this + val) 的 BigInteger
BigInteger add = b1.add(b2);
System.out.println("add = " + add);
//BigInteger subtract(BigInteger val) 返回其值为 (this - val) 的 BigInteger
BigInteger subtract = b1.subtract(b2);
System.out.println("subtract = " + subtract);
//BigInteger multiply(BigInteger val) 返回其值为 (this * val) 的 BigInteger
BigInteger multiply = b1.multiply(b2);
System.out.println("multiply = " + multiply);
//BigInteger divide(BigInteger val) 返回其值为 (this / val) 的 BigInteger
BigInteger divide = b1.divide(b2);
System.out.println("divide = " + divide);
}
int intValue() -> 将BigInteger转成int型
long longValue()-> 将BIgInteger转成long型
第三章.BigDecimal类
1.BigDecimal介绍
开发不要用double或者float直接做运算,否则很容易出现精度损失问题,所以我们一般都是用BigDecimal去进行小数运算
作用:处理float或者double直接做运算出现的精度损失问题
2.BigDecimal使用
1.构造:
BigDecimal(String val) -> val必须是数字格式
2.方法:
static BigDecimal valueOf(double val)-> 根据double型数据创建BigDecimal对象
BigDecimal add(BigDecimal val) 返回其值为 (this + val) 的 BigDecimal
BigDecimal subtract(BigDecimal val) 返回其值为 (this - val) 的 BigDecimal
BigDecimal multiply(BigDecimal val) 返回其值为 (this * val) 的 BigDecimal
BigDecimal divide(BigDecimal val) 返回其值为 (this / val) 的 BigDecimal
3.注意:
如果除不尽,会出现算术异常的问题
BigDecimal divide(BigDecimal divisor, int scale, RoundingMode roundingMode)
divisor:除号后面的数
scale:保留几位小数
roundingMode:取舍方式 -> 是一个枚举类
UP:向上加1
DOWN:直接舍去
HALF_UP:四舍五入
public static void main(String[] args) {
BigDecimal b1 = BigDecimal.valueOf(3.55);
BigDecimal b2 = BigDecimal.valueOf(2.12);
BigDecimal divide = b1.divide(b2,2, RoundingMode.DOWN);
System.out.println("divide = " + divide);
}
第四章.System类
public static void main(String[] args) {
long time = System.currentTimeMillis();
System.out.println(time);
Date date = new Date(time);
System.out.println("date = " + date);
int[] arr = {1,2,3,4,5,6,7,8,9};
int[] arr2 = new int[10];
System.arraycopy(arr,0,arr2,0,2);
System.out.println(Arrays.toString(arr2));
}
第五章.Arrays数组工具类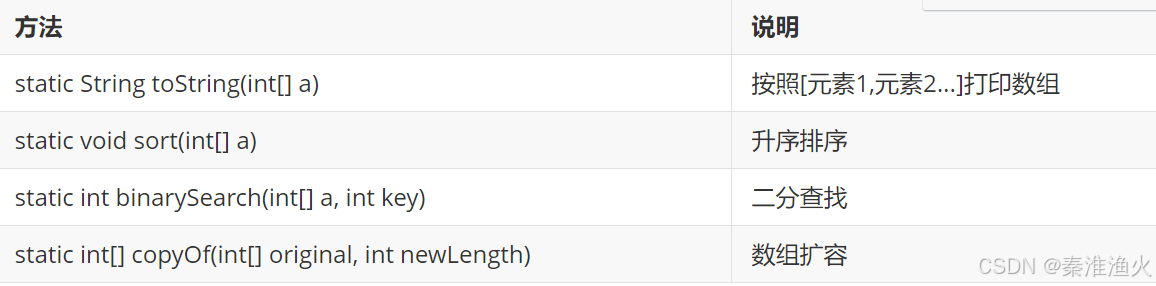
public static void main(String[] args) {
int[] arr = {5,4,3,2,1};
System.out.println(Arrays.toString(arr));
Arrays.sort(arr);
System.out.println(Arrays.toString(arr));
int[] arr2 = {11,22,33,44,55,66,77,88};
int index = Arrays.binarySearch(arr2, 33);
System.out.println("index = " + index);
int[] arr3 = {1,2,3,4,5};
int[] newArr = Arrays.copyOf(arr3, 10);
arr3 = newArr;
System.out.println(Arrays.toString(arr3));
}
第六章.包装类
1.基本数据类型对应的引用数据类型(包装类)
1.概述:包装类是基本类型对应的引用类型,将基本类型转成包装类,使基本类型拥有了类的属性,就可以使用类中的方法去操作数据
2.为啥要将基本类型转成包装类?
将来调用某个方法,方法参数要求传递包装类,所以我们需要将基本类型转成包装类传进去
比如ArrayList中的add方法 -> add(Integer i)-> 此时就不能直接往add方法中传递基本类型
我们需要将基本类型转成包装类才能传递进去
3.将来我们还需要将包装类转会基本类型:
包装类不能直接做+ - * /,所以需要先转成基本类型,才能使用运算符
2.Integer
1.构造:
Integer(int i)
Integer(String s)
1.装箱:将基本类型转成包装类
2.方法:
static Integer valueOf(int i)
1.拆箱:将包装类转成基本类型
2.方法:
int intValue()
3.基本类型和String之间的转换
3.1 基本类型往String转
方式1:
+拼接
方式2:String中的方法:
static String valueOf(int i)
public static void main(String[] args) {
int i = 10;
String s = i+"";
System.out.println(s+1);
String s1 = String.valueOf(10);
System.out.println(s1+1);
}