一. Math类
- public final class Math extends Object:表示Math类是最终类,不能被继承
- Math是一个帮助我们用于进行数学运算的工具类。
- Math的构造器私有,不能创建对象。
- Math类严格来说是一个工具类,工具类是用来做功能的。工具类里面的方法都是静态方法,直接用类名即可调用,不需要创建对象。
- 之前的random是作为一个独立类,它是创建Random对象,调用nextInt方法,而这个random是充当Math类的一个静态方法。
- 静态方法属于类,通过类名来调用。
package com.gch.d12_math;
public class MathDemo {
public static void main(String[] args) {
// 1.abs 获取参数绝对值
System.out.println(Math.abs(10)); // 10
System.out.println(Math.abs(-10.3)); // 10.3
// bug:
// 以int类型为例,取值范围:-2147483648 ~ 2147483647
// 如果没有正数与负数对应,那么传递负数结果有误
// -2147483648 没有正数与之对应,所以abs结果产生bug
System.out.println(Math.abs(-2147483648)); // -2147483648
// 2.向上取整 进一法,往数轴的正方向进一
System.out.println(Math.ceil(4.00000001)); // 5.0
System.out.println(Math.ceil(4.0)); // 4.0
System.out.println(Math.ceil(-12.34));// -12.0
System.out.println(Math.ceil(-12.54));// -12.0
// 3.向下取整 往数轴的负方向进一
System.out.println(Math.floor(4.99999999)); // 4.0
System.out.println(Math.floor(4.0)); // 4.0
System.out.println(Math.floor(12.34)); // 12.0
System.out.println(Math.floor(12.54)); // 12.0
System.out.println(Math.floor(-12.34)); // -13.0
System.out.println(Math.floor(-12.54)); // -13.0
// 4.求指数次方
System.out.println(Math.pow(2 , 3)); // 2 ^ 3 = 8.0
// 开平方根
System.out.println(Math.sqrt(4.0)); // 2.0
// 开立方根
System.out.println(Math.cbrt(8.0)); // 2.0
// 5.四舍五入 返回值类型为long
// 四舍五入的原理是在参数上加0.5然后进行向下取整
System.out.println(Math.round(4.49999)); // 4
System.out.println(Math.round(4.500001)); // 5
System.out.println(Math.round(11.5)); // 12
System.out.println(Math.round(-11.5)); // -11
System.out.println(Math.round(12.34)); // 12
System.out.println(Math.round(12.54)); // 13
System.out.println(Math.round(-12.34)); // -12
System.out.println(Math.round(-12.54)); // -13
// 0.0 - 1.0 包前不包后,包0不包1
System.out.println(Math.random()); // 0.8401287463711933
}
}
二.System类
- System也是一个工具类,提供了一些与系统相关的方法
- 填0表示自己正常的把虚拟机关掉了。
- 1秒 = 1000 毫秒 1s = 1000ms
package com.gch.d13_system;
import java.util.Arrays;
public class SystemDemo {
public static void main(String[] args) {
System.out.println("程序开始....");
// 方法的形参:
// 状态码:
// 0:表示当前虚拟机是征程停止
// 非0:表示当前虚拟机是异常停止
// System.exit(0); // JVM终止! 删库跑路
// 1s = 1000ms
// 2.计算机认为时间有起源:返回1970-1-1 00:00:00 走到此刻的总的毫秒值:时间毫秒值。
long time = System.currentTimeMillis();
System.out.println(time);
// 作用:进行时间的计算:做性能分析
long startTime = System.currentTimeMillis();
for(int i = 0;i < 100000;i++){
System.out.println("输出:" + i);
}
long endTime = System.currentTimeMillis();
System.out.println((endTime - startTime) / 1000.0 + "s");
// 3.做数组拷贝(了解)
/* arraycopy(Object src, int srcPos,
Object dest, int destPos,int length);
参数1:src 被拷贝的数组
参数2:srcPos 从哪个索引位置开始拷贝
参数3:dest 赋值的目标数组
参数4:destPos 粘贴位置
参数5:length 拷贝元素的个数
*/
int[] arr1 = {10, 20, 30, 40, 50, 60, 70};
int[] arr2 = new int[6]; // [0, 0, 0, 0, 0, 0]==>[0, 0, 40, 50, 60, 0]
System.arraycopy(arr1,3, arr2, 2, 3);
System.out.println(Arrays.toString(arr2));
System.out.println("程序结束....");
}
}
package com.system.api;
public class SystemDemo {
public static void main(String[] args) {
Object obj = new Object();
// 细节:
// System:类名
// out:静态变量
// System.out:获取打印的对象
// println():方法
// 参数:表示打印的内容
// 核心逻辑:
// 当我们打印一个对象的时候,底层会调用String.valueOf方法,把对象变成字符串
// 然后再打印再控制台上,打印完毕换行处理。
// 直接输出对象变量,默认省略toString调用不写的
System.out.println(obj.toString()); // java.lang.Object@4554617c
}
}
三. Runtime类
Runtime表示当前虚拟机的运行环境,而一个电脑只能有一个运行环境
- 在外界不管你是在哪个类当中去调用getRuntime()的方法,你获取的都是同一个对象。
package com.runtime.api;
import java.io.IOException;
/**
* @author A.G.H
*/
public class RuntimeDemo {
public static void main(String[] args) throws IOException {
// 1.public static Runtime getRuntime():获取当前系统的运行环境 / Runtime对象
// Runtime currentRuntime1 = Runtime.getRuntime();
// Runtime currentRuntime2 = Runtime.getRuntime();
// System.out.println(currentRuntime1 == currentRuntime2); // true
// 2.public void exit(int status):停止虚拟机
// Runtime.getRuntime().exit(0);
// Runtime currentRuntime = Runtime.getRuntime();
// currentRuntime.exit(0);
// 3.public int availableProcessors:获得CPU的线程数
System.out.println(Runtime.getRuntime().availableProcessors()); // 12
// 4.public long maxMemory():JVM能从系统中获取总内存大小(单位byte) byte / 1024:KB || byte / 1024 / 1024:M
System.out.println(Runtime.getRuntime().maxMemory() / 1024 / 1024 + "M"); // 3609M
// 5.public long totalMemory():JVM已经从系统中获取总内存大小(单位:byte)
System.out.println(Runtime.getRuntime().totalMemory() / 1024 / 1024 + "M"); // 243M
// 6.public long freeMemory():JVM剩余内存大小(单位:byte)
System.out.println(Runtime.getRuntime().freeMemory() / 1024 / 1024 + "M"); // 239M
// 7.public Process exec(String command):运行cmd命令
System.out.println(Runtime.getRuntime().exec("qq")); // 打开QQ
System.out.println(Runtime.getRuntime().exec("notepad")); // 打开记事本
// shutdown:关机
// 必须加上参数才能执行
// -s:默认在1分钟之后关机
// -s -t + 指定时间:指定时间关机 单位:秒s
// -a:取消关机操作
// -r:关机并重启
Runtime.getRuntime().exec("shutdown -s -t 3600");
}
}
四. BigInteger类
package com.biginteger.api;
import java.math.BigInteger;
import java.util.Random;
/**
BigInteger的构造方法:
public BigInteger(int num,Random rnd):获取随机大整数,范围:[0 ~ 2的num次方 - 1]
public BigInteger(String val):获取指定的大整数
public BigInteger(String val,int radix):获取指定进制的大整数
public static BigInteger valueOf(long val):静态方法获取BigInteger的对象,内部有优化
细节:
对象一旦创建里面的数据不能发生改变
*/
public class BigIntegerDemo1 {
public static void main(String[] args) {
// 1.获取一个随机的大整数
// public BigInteger(int num,Random rnd) 范围:[0 ~ 2的num次方 - 1]
Random r = new Random();
BigInteger bd1 = new BigInteger(4, r);// [0 ~ 15]
System.out.println(bd1); // 8
// 2.获取一个指定的大整数(用的比较多)
// public BigInteger(String val)
// 细节:字符串中必须是整数,不能是1.1或者"abc"之类的,否则运行会报错:NumberFormarException(数字格式化异常)
BigInteger bd2 = new BigInteger("10"); // 默认是十进制
System.out.println(bd2); // 10
// 3.获取一个指定进制的大整数
// public BigInteger(String val,int radix)
// 细节:
// 1.字符串中的数字必须是整数
// 2.字符串中的数字必须要跟进制吻合
// 比如二进制中,就只能写0和1,写其他的就报错
BigInteger bd3 = new BigInteger("10",2);
System.out.println(bd3); // 2 会转成十进制打印出来
// 4.静态方法获取BigInteger的对象,内部有优化(常用)
// public static BigInteger valueOf(long val)
// 细节:
// 1.能表示的范围比较小,在long的取值范围之内,如果超出long的范围就不行了。
// 2.在内部对常用的数字: -16 ~ 16进行了优化
// 提前把-16 ~ 16先创建好BigInteger的对象,如果多次获取不会创建新的。
// 会节约内存
BigInteger bd4 = BigInteger.valueOf(100);
System.out.println(bd4); // 100
BigInteger bd5 = BigInteger.valueOf(9223372036854775807L);
System.out.println(bd5); // 922337203685477580
BigInteger bd6 = BigInteger.valueOf(16);
BigInteger bd7 = BigInteger.valueOf(16);
System.out.println(bd6 == bd7); // true
BigInteger bd8 = BigInteger.valueOf(17);
BigInteger bd9 = BigInteger.valueOf(17);
System.out.println(bd8 == bd9); // false
System.out.println(Long.MAX_VALUE); // 9223372036854775807
System.out.println(Integer.MAX_VALUE); // 2147483647
// 5.对象一旦创建内部的数据不能发生改变
BigInteger bd10 = BigInteger.valueOf(1);
BigInteger bd11 = BigInteger.valueOf(2);
BigInteger result = bd10.add(bd11);
System.out.println(result); // 3
// 此时,不会修改参与计算的BigInteger对象中的值,而是产生了一个新的BigInteger对象记录3
System.out.println(bd10 == result); // false
System.out.println(bd11 == result); // false
}
}
BigInteger的常用方法: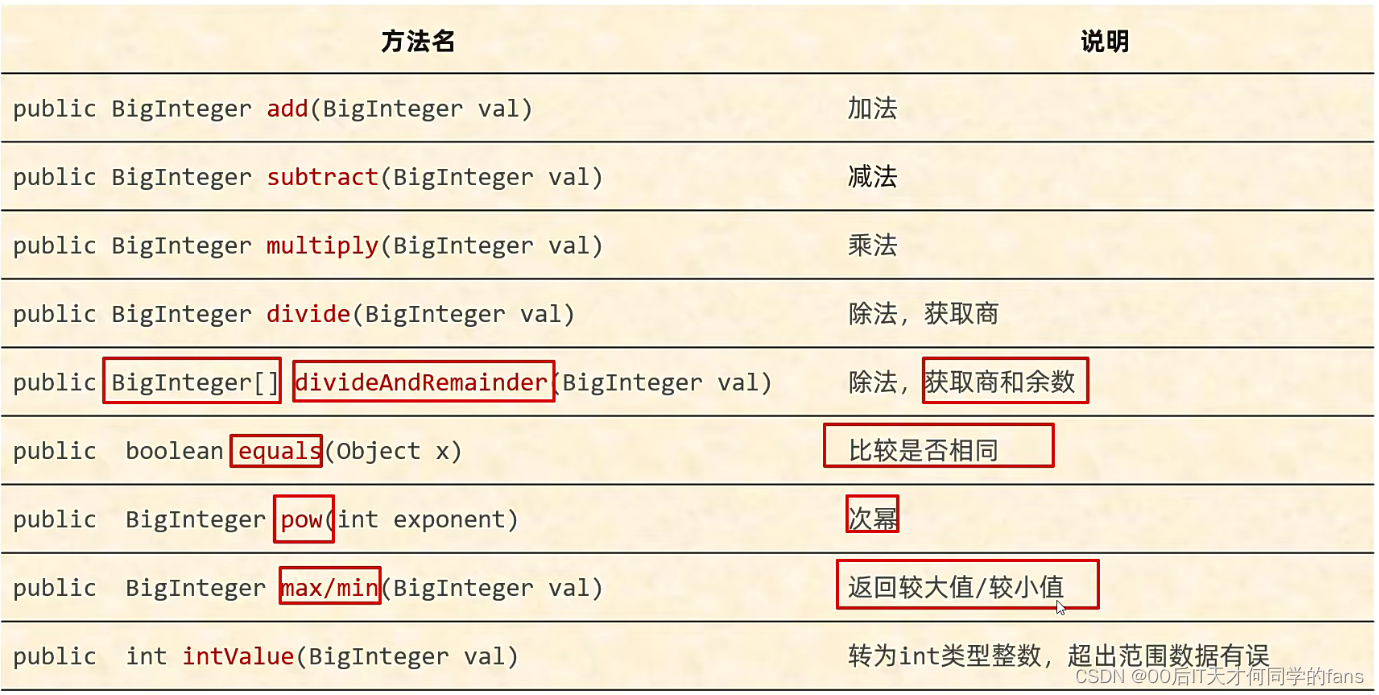
注意:BigInteger是一个对象,对象是不能直接加直接减直接乘直接除,也不能直接用大于小于进 行操作的,所有的操作都要用方法去完成。
package com.biginteger.api;
import java.math.BigInteger;
/**
BigInteger的常用API:
public BigInteger add(BigInteger val):加法
public BigInteger subtract(BigInteger val):减法
public BigInteger multiply(BigInteger val):乘法
public BigInteger divide(BigInteger val):除法,获取商
public BigInteger[] divideAndRemainder(BIgInteger val):除法,获取商和余数
public boolean equals(Object x):比较是否相同
public BigInteger pow(int exponent):次幂
public BigInteger max/min(BigInteger val):返回较大值 / 较小值
public int intValue(BigInteger val):转为int类型整数,超出范围数据有误
*/
public class BigIntegerDemo2 {
public static void main(String[] args) {
// 1.创建两个BigInteger对象
BigInteger bd1 = BigInteger.valueOf(10);
BigInteger bd2 = BigInteger.valueOf(5);
// 比较是否相同
boolean result = bd1.equals(bd2);
System.out.println(result); // false
// 2.加法
// public BigInteger add(BigInteger val)
BigInteger bd3 = bd1.add(bd2);
System.out.println(bd3); // 15
// 3.减法
// public BigInteger subtract(BigInteger val)
BigInteger bd4 = bd1.subtract(bd2);
System.out.println(bd4); // 5
// 4.乘法
// public BigInteger multiply(BigInteger val)
BigInteger bd5 = bd1.multiply(bd2);
System.out.println(bd5); // 20
// 5.除法,获取商
// public BigInteger divide(BigInteger val)
BigInteger bd6 = bd1.divide(bd2);
System.out.println(bd6); // 2
// 5.除法,获取商和余数
BigInteger[] arr = bd1.divideAndRemainder(bd2);
System.out.println(arr.length); // 2
System.out.println(arr[0]); // 2
System.out.println(arr[1]); // 0
// 6.次幂
// public BigInteger pow(int exponent)
BigInteger bd7 = bd1.pow(2);
System.out.println(bd7); // 100
// 7.返回较大值 / 较小值
// public BigInteger max/min(BigInteger val)
// 细节:
// 比较完方法在底层不会创建新的BigInteger对象,而是返回比较完原有的对象
BigInteger bd8 = bd1.max(bd2);
System.out.println(bd8); // 10
System.out.println(bd8 == bd1); // true
BigInteger bd9 = bd1.min(bd2);
System.out.println(bd9); // 5
System.out.println(bd9 == bd2); // true
// 8.转为int类型整数,超出范围数据有误
// public int intValue()
BigInteger bd10 = BigInteger.valueOf(2147483648L);
int i1 = bd10.intValue(); // -2147483648
System.out.println(i1); // int最大值为:2147483647
BigInteger bd11 = BigInteger.valueOf(2147483647);
int i2 = bd11.intValue(); // 2147483647
System.out.println(i2);
// 转为double类型小数
// public double doubleValue()
double d = bd1.doubleValue();
System.out.println(d); // 10.0
// public long longValue()
}
}
五. BigDecimal类
- BigDecimal:大数据类型的意思。
- 方法名相同,参数不同,都是重载的方法。
- 调方法得对象永远是最好的方法。
package com.gch.d14_bigdecimal;
import java.math.BigDecimal;
import java.math.RoundingMode;
public class BigDecimalDemo {
public static void main(String[] args) {
// 浮点数运算的时候直接 + * / 可能会出现数据失真(精度问题)。
System.out.println(0.09 + 0.01);
System.out.println(1.0 - 0.32);
System.out.println(1.015 * 100);
System.out.println(1.301 / 100);
System.out.println("-----------------------");
double a = 0.1;
double b = 0.2;
double c = a + b;
System.out.println(c); // 0.30000000000000004
System.out.println("------------------------");
// 包装浮点数类型成为大数据对象 BigDecimal 手段
BigDecimal a1 = BigDecimal.valueOf(a);
BigDecimal b1 = BigDecimal.valueOf(b);
// BigDecimal c1 = a1.add(b1); // 引用数据类型的变量输出的是数据而不是地址,说明重写了toString方法
// BigDecimal c1 = a1.subtract(b1); // 相减
// BigDecimal c1 = a1.multiply(b1); // 相乘
BigDecimal c1 = a1.divide(b1); // 相除
System.out.println(c1); // 0.5
// 目的:double 把BigDecimal类型转成Double类型
double rs = c1.doubleValue();
System.out.println(rs); // 0.5
// 注意事项:BigDecimal是一定要精度运算的
BigDecimal a11 = BigDecimal.valueOf(10.0);
BigDecimal b11 = BigDecimal.valueOf(3.0);
// BigDecimal c11 = a11.divide(b11); // 运行报错
/**
参数1:除数 参数2:保留小数位数 参数3:舍入模式
*/
BigDecimal c11 = a11.divide(b11 , 2 , RoundingMode.HALF_UP);
System.out.println(c11); // 3.33
double rs11 = c11.doubleValue();
System.out.println(rs11); // 3.33
System.out.println("--------------------------");
}
}