/*
前言:
当你看完这个代码 你就会越发感觉到 C#是一个控制类型的 面向对象的语言 你的东西的实现是需要借助 Console 去实现的。
而 C 的逻辑构架更像是对值的处理 输入值 处理值 再返回值 作为一个函数存在 主函数将其链接在一起。
C# 不涉及这 更多像是对属性的描述 但是 C# 的使用习惯和 C 有很多相似的地方。
这个教程主要展现 字符串 和 数据结构 有关的内容。
*/
Using System
namespace LearningStrings
{
// Structs
public struct Point {
public double X { get; }
public double Y { get; }
public Point (double x, double y) => (X,Y) = (x, y);
}
// Hello World, Using $ and {} to import variables, Using number. And Replace
class sayHello
{
/*
普普通通的Hello World.
*/
static void PrintSayHello () {
string sayHello = "Hello Alan"; // Basic knowledges about strings.
Console.WriteLine ($"Friends {sayHello}"); // {} Could be used to contain variables.
}
/*
Replace可以用来实现字符串的替代
*/
static void StringReplace () {
// Replace could used to replace part.
sayHello = sayHello.Replace ("Hello". "Greeting");
Console.WriteLine ($"Friends {sayHello}");
// .Length could show length of string, size of shows the memory bytes used.
Console.WriteLine ($"Length is {SayHello.length}");
Console.WriteLine ($"Length is {0}", sizeof(SayHello));
}
/*
Trim可以用来分割空白部分的字符串
*/
static void PrintStringTrim () {
// Basic knowledges about strings about Trims, cut spaces off.
string Trimstring = " Cuiyuan-CY.Liu@aia.com ";
string trimdEmail = "";
trimdEmail = Trimstring.TrimStart ();
Console.WriteLine ([{Trimstring}]); // [Cuiyuan-CY.Liu@aia.com ]
trimdEmail = Trimstring.TrimEnd ();
Console.WriteLine ([{Trimstring}]); // [ Cuiyuan-CY.Liu@aia.com]
trimdEmail = Trimstring.Trim ();
Console.WriteLine ([{Trimstring}]); // [Cuiyuan-CY.Liu@aia.com]
}
// string Contains
static void StringContains () {
// This function return a boolean displays whether or not contain the idented string.
string songLyrics = "You say greetings and I say goodbye.";
var result = songLyrics.Contains (goodbyes);
Console.Writeline (result);
}
}
class LearnNumber
{
static void learnInt () {
// Consider int as an array here?
int max = int.MaxValue;
int min = int.MinValue;
Console.WriteLine ($"The range of integer is {min} to {max}"); // 和c 的 %c 不太一样
int input = 22;
// A basic example of if
if ((input < max) && (input > min)) {
Console.WriteLine ("That number is good!");
} else {
Console.WriteLine ("That number is bad");
break;
}
// A basic example of while
int count = 0;
while (count < 10) {
Console.WriteLine (count.ToString);
count++;
}
// A basic example of for loop
for (int counter = 0; counter < 10; counter++) {
Console.WriteLine (counter.ToString);
}
}
}
class BasicExampleOfDataStructure
{
/*
List 是一个很好用的数据结构 可以用 List 去添加删除 管理 items 不需要预先规划大小
你可以这样理解 在C#中 IndexOf 是作为一个属性函数 所以用 names.IndexOf () 去描述.
同样地 对于在 List 中进行值的添加和删去 你可以用 List.Add 或者 List.Remove 去实现
*/
static void ListManage () {
// List 下是里面的变量类型 你也可以是数组 甚至是一个 List
var names = new List<string> {"Apple","Oppo","Huawei","Banana"};
// 添加或去掉 List 里的东西
names.Add ("Samsung"); // Add an item.
names.Remove ("Banana"); // Remove an item.
// foreach 是循环的一种 可以用来做集合类型的循环 for each sth. in the set 记得定义 while 不能定义
foreach (var name in names) {
Console.WriteLine (name);
}
// Sort List Alphabetically
names.Sort ();
foreach (var name in names) Console.WriteLine (name);
// Index
var index = names.IndexOf ("Huawei"); // Return the index of an item in the set.
Console.WriteLine ($"Found Huawei at {Index}");
Console.WriteLine ($"Found Banana at {0}", names.IndexOf ("Banana")); // If not found, the index = -1
if (Index == -1) {
Console.WriteLine ($"When Item not found, returns {Index}");
} else if (Index >= 0) {
Console.WriteLine ($"The name {names[Index]} is at {Index}"); // 注意的是 在C#中 函数包东西用"()"
}
}
/*
List 小实战 生成 Fibonacci 数列
Fibonacci 数列是由两个1产生 1 1 2 3 5 8 13 ... 的数列 后者的项是前两者之和
*/
static void FibonacciList () {
var FibonacciNumbers = new List<int> {1,1};
var prep1 = 0, prep2 = 0;
for (int counter = 0; counter < 20; counter ++) {
// 生成前两项的数字
prep1 = FibonacciNumbers[FibonacciNumbers.Count - 1]; // 前一项
prep2 = FibonacciNumbers[FibonacciNumbers.Count - 2]; // 前第二项
// 加在一起求和并添加进 Fibonacci 数列中
FibonacciNumbers.Add (prep1 + prep2);
}
// 写出 Fibonacci 数列
foreach (var item in FibonacciNumbers) {
Console.WriteLine (item);
}
}
}
}
/*
这个实例介绍 C# 方法 定义一个方法时 实际上是在说明它结构的元素
<Access Specifier> <Return Type> <Method Name> (Parameter List)
{
Method Body
}
Access Specifier 访问修饰符
public 可以用在另外的类中
static 只能用在当前类下
Return type 返回类型
一个防范可以返回一个值 返回类型则是返回值的数据类型 如果不返回任何值 则我们选择用 void
Method Name 方法名称
可以视作方法名或者函数名
Parameter List 参数列表
用圆括号括起来, 该参数是用来传递和接受方法的数据, 参数列表是指方法的参数类型 顺序 数量。
也就是说 一个方法可能不包含参数。
Method body 方法内容
这个是函数的主体部分。
*/
using System;
namespace Caculator
{
class NumberManipulator
{
// 这一段真的很有 C 的感觉 是的 方法是可以视作函数的。但是是类下属的属性。
public int FindMax (int num1, int num2) {
int result = 0;
if (num1 > num2) {
result = num1;
} else {
result = num2;
}
return result;
}
// 主函数
static void Main (string[] args) {
int a = 100, b = 200, ret = 0;
NumberManipulator n = new NumberManipulator();
ret = n.FindMax (a, b);
Console.WriteLine ("The max value is {0}", ret);
Console.ReadLine ();
}
}
}
/*
前言:
当你看完这个代码 你就会越发感觉到 C#是一个控制类型的 面向对象的语言 你的东西的实现是需要借助 Console 去实现的。
而 C 的逻辑构架更像是对值的处理 输入值 处理值 再返回值 作为一个函数存在 主函数将其链接在一起。
C# 不涉及这 更多像是对属性的描述 但是 C# 的使用习惯和 C 有很多相似的地方。
这个教程主要展现 字符串 和 数据结构 有关的内容。
*/
Using System
namespace LearningStrings
{
// Structs
public struct Point {
public double X { get; }
public double Y { get; }
public Point (double x, double y) => (X,Y) = (x, y);
}
// Hello World, Using $ and {} to import variables, Using number. And Replace
class sayHello
{
/*
普普通通的Hello World.
*/
static void PrintSayHello () {
string sayHello = "Hello Alan"; // Basic knowledges about strings.
Console.WriteLine ($"Friends {sayHello}"); // {} Could be used to contain variables.
}
/*
Replace可以用来实现字符串的替代
*/
static void StringReplace () {
// Replace could used to replace part.
sayHello = sayHello.Replace ("Hello". "Greeting");
Console.WriteLine ($"Friends {sayHello}");
// .Length could show length of string, size of shows the memory bytes used.
Console.WriteLine ($"Length is {SayHello.length}");
Console.WriteLine ($"Length is {0}", sizeof(SayHello));
}
/*
Trim可以用来分割空白部分的字符串
*/
static void PrintStringTrim () {
// Basic k
C# Tutorials
最新推荐文章于 2024-10-16 22:52:46 发布
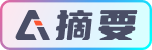