目录
string 概述 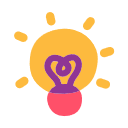
- String objects are a special type of container, specifically designed to operate with sequences of characters.
- Unlike traditional c-strings, which are mere sequences of characters in a memory array, C++ string objects belong to a class with many built-in features to operate with strings in a more intuitive way and with some additional useful features common to C++ containers.
- 字符串对象是一种特殊的容器,专门用于操作字符序列。
- 与仅仅是内存数组中的字符序列的传统C字符串不同,c++字符串对象属于这样一个类,它具有许多内置特性,可以以更直观的方式操作字符串,还具有一些c++容器共有的有用特性。
成员函数 (Member Functions) 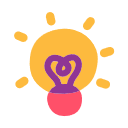
1 构造器 string::string
- 构造器构造一个标准的string对象并初始化它的内容
- 不同的构造器提供string的多种不同的初始化方式
有没有兴趣阅读一下原API? Come on
/*
Constructs a standard string object and initializes its content.
The different constructor versions allow for the content to be initialized in different ways:
*/
string ( ); // Content is initialized to an empty string. 初始化一个空字符串
// Content is initialized to a copy of the string object str.
string ( const string& str ); // 这里传入的是字符串的引用&(引用传递)注意不是值传递
/*
Content is initialized to a copy of a substring of str. 将str的字字符串作为初始化值
The substring is the portion of str that begins at the character position pos
and takes up to n characters
(it takes less than n if the end of str is reached before). // 如果长度n比str还大 则实际只会截取到str的最后一个字符
*/
string ( const string& str, size_t pos, size_t n = npos ); // pos开始位置 n为从开始位置截取的长度
/*
Content is initialized to a copy of the string
formed by the first n characters in the array of characters pointed by s.
*/
string ( const char * s, size_t n ); // 将str的头n个字符作为初始化内容
/*
Content is initialized to a copy of the string formed by the null-terminated
character sequence (C string) pointed by s.
The length of the caracter sequence is determined by the first occurrence
of a null character (as determined by traits.length(s)).
This version can be used to initialize a string object using a string literal constant.
*/
string ( const char * s ); // 将整个字符数组中的字符作为初始化内容
// 注意传入的字符数组必须是null-terminated character sequence的,即这个字符数组要以空字符'\0'结尾
// Content is initialized as a string formed by a repetition of character c, n times.
string ( size_t n, char c ); // 这个就简单了 就是把n个字符c作为初始化内容
/*
If InputIterator is an integral type, behaves as the sixth constructor version
(the one right above this) by typecasting begin and end to call it:
string(static_cast<size_t>(begin),static_cast<char>(end));
In any other case, the parameters are taken as iterators,
and the content is initialized with the values of the elements that
go from the element referred by iterator begin to the element right
before the one referred by iterator end.
*/
// 如果对STL其他容器有所了解 那么这个就一眼看懂 这是传入了两个STL容器的迭代器
// 将某容器的[begin, end)部分的内容作为字符串的初始化内容
template<class InputIterator> string (InputIterator begin, InputIterator end);
感觉是不是神清气爽 ಠᴗಠ 下面是精简版
string ( ); // 初始化一个空字符串
string ( const string& str ); // 这里传入的是字符串的引用& 将str的值作为初始化内容
// 将str的子串作为初始化内容 从pos开始位置截取长度n的str的子串
string ( const string& str, size_t pos, size_t n = npos );
string ( const char * s, size_t n ); // 将str的头n个字符作为初始化内容
// 把一个字符数组的所有字符作为初始化内容 注意这个字符数组必须以空字符'\0'结尾
string ( const char * s );
string ( size_t n, char c ); // 这个就简单了 就是把n个字符c作为初始化内容
/* 如果对STL其他容器有所了解 那么这个就一眼看懂 这是传入了两个STL容器的迭代器
将某容器的[begin, end)部分的内容作为字符串的初始化内容 */
template<class InputIterator> string (InputIterator begin, InputIterator end);
验证构造器(细节都在代码和注释中):
/**
* created by Liu Xianmeng on 2022/12/5
*/
#include <bits/stdc++.h>
using namespace std;
int main(){
//string ( ); // 初始化一个空字符串
string str1;
//string ( const string& str ); // 这里传入的是字符串的引用& 将str的值作为初始化内容
string oriStr = "BigXMeng";
string str2_1 = string(oriStr); // str2_1和str2_2的构造时等同的
string str2_2 = string("BigXMeng"); // str2_1和str2_2的构造时等同的
//string ( const string& str, size_t pos, size_t n = npos );
string str3 = string(oriStr, 2, 3); // 获取从下标2往后的3个字符
//string ( const char * s, size_t n ); // 将str的头n个字符作为初始化内容
string str4 = string(&oriStr[0], 3); // 字符数组的前3个字符
// ( const char * s ); // 把一个字符数组作为初始化内容
char chs1[8] = {'B', 'i', 'g', 'X', 'M', 'e', 'n', 'g'}; // 数组末尾没有存储'\0'
char chs2[20] = {'B', 'i', 'g', 'X', 'M', 'e', 'n', 'g'}; // 数组末尾存储有'\0'
string str5_1 = string(chs1);
string str5_2 = string(chs2);
// ( size_t n, char c ); // 这个就简单了 就是把n个字符c作为初始化内容 这里的c既可以是字符 也可以是ASCLL码
string str6 = string(6, 'Q');
//template<class InputIterator> string (InputIterator begin, InputIterator end); 用其他容器进行构造
vector<char> v; for(int i=97; i<=101; ++i) v.push_back((char)i);
string str7 = string(v.begin(), v.begin()+3); // 用v的第1到第3个元素初始化字符串
string str8 = string(oriStr.begin(), oriStr.begin()+3); // 用v的第1到第3个元素初始化字符串
/ 打印字符串 /
cout<<"str1 = "<<str1<<endl;
cout<<"str2_1 = "<<str2_1<<endl;
cout<<"str2_2 = "<<str2_2<<endl;
cout<<"str3 = "<<str3<<endl;
cout<<"str4 = "<<str4<<endl;
cout<<"str5_1 = "<<str5_1<<" (字符数组中如果最后没有存储'\\0',打印会出现乱码)"<<endl;
cout<<"str5_2 = "<<str5_2<<" (这个数组定义的足够大,后面默认存储的是\\'0',所以不会出现乱码)"<<endl;
cout<<"str6 = "<<str6<<endl;
cout<<"str7 = "<<str7<<endl;
cout<<"str8 = "<<str8<<endl;
/*
str1 =
str2_1 = BigXMeng
str2_2 = BigXMeng
str3 = gXM
str4 = Big
str5_1 = BigXMeng��%� (字符数组中如果最后没有存储'\0',打印会出现乱码)
str5_2 = BigXMeng (这个数组定义的足够大,后面默认存储的是\'0',所以不会出现乱码)
str6 = QQQQQQ
str7 = abc
str8 = Big
*/
cout<<"*****************************"<<endl;
string s0 ("Initial string");
// constructors used in the same order as described above:
string s1;
string s2 (s0);
string s3 (s0, 8, 3);
string s4 ("A character sequence", 6);
string s5 ("Another character sequence");
string s6 (10, 'x');
string s7a (10, 42); // 使用连续的10个ASCLL码为42的字符进行初始化
string s7b (s0.begin(), s0.begin()+7);
cout << "s1: " << s1 << "\ns2: " << s2 << "\ns3: " << s3;
cout << "\ns4: " << s4 << "\ns5: " << s5 << "\ns6: " << s6;
cout << "\ns7a: " << s7a << "\ns7b: " << s7b << endl;
/*
s1:
s2: Initial string
s3: str
s4: A char
s5: Another character sequence
s6: xxxxxxxxxx
s7a: **********
s7b: Initial
*/
}
2 string::operator= (等号操作符)
概述
/*
等号'='操作符 返回一个字符串的引用
Sets a copy of the argument as the new content for the string object.
The previous content is dropped. 赋值后原字符串的内容会被舍弃
*/
string& operator= ( const string& str ); // 传入一个字符串的引用
string& operator= ( const char* s ); // 传入一个字符指针(字符数组名)
string& operator= ( char c ); // 单个字符也可以被赋值给字符串对象
验证operator=
/**
* created by Liu Xianmeng on 2022/12/5
*/
#include <bits/stdc++.h>
using namespace std;
int main(){
string str1, str2, str3;
str1 = "Test string: "; // c-string
str2 = 'x'; // single character
str3 = str1 + str2; // string
cout << str3 << endl;
/* 【result】
Test string: x
*/
return 0;
}
3 Iterators: 迭代器相关
迭代器在其他函数的演示中体现
4 Capacity:容量相关
string::max_size
Returns the maximum number of characters that the string object can hold.
返回字符串对象可以持有的最大字符数量
string::reserve
void reserve ( size_t res_arg=0 ); // 声明
- Requests that the capacity of the allocated storage space in the string be at least res_arg.
- If the requested size to allocate is greater than the maximum size (string::max_size) a length_error exception is thrown.
- 请求字符串的容量至少为传入的无符号整型res_arg大小,如果参数比max_size还大,则会抛出异常
- 这个函数较为特殊,放在后续学习
string::clear
void clear(); // 函数声明
- The string content is set to an empty string, erasing any previous content and thus leaving its size at 0 characters.
- 清空string原先的所有内容,使得size=0
string::[Capacity:] 验证
/**
* created by Liu Xianmeng on 2022/12/5
* 除了reverse以外 其他Capacity成员函数的验证
*/
#include <bits/stdc++.h>
using namespace std;
int main(){
string str1 ("BigXMeng");
cout << "The size of str1 is " << str1.size() << " characters.\n";
cout << "The length of str1 is " << str1.length() << " characters.\n";
cout << "The capacity of str1 is " << str1.capacity() << " characters.\n";
cout << "The max_size of str1 is " << str1.max_size() << " characters.\n";
str1.clear();
cout << "The length of str1 is " << str1.length() << " characters.\n";
cout << "The capacity of str1 is " << str1.capacity() << " characters.\n";
if(str1.empty()) printf("str1 is empty now.");
/* 【除了reverse以外 其他Capacity成员函数的验证】
The size of str1 is 8 characters.
The length of str1 is 8 characters.
The capacity of str1 is 15 characters.
The max_size of str1 is 4611686018427387903 characters.
The length of str1 is 0 characters.
The capacity of str1 is 15 characters.
str1 is empty now.
*/
return 0;
}