//server
package com.test.javaSe02;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.net.ServerSocket;
import java.net.Socket;
import com.ctl.util.PropertiesUtil;
public class ECHOServer {
public static void main(String[] args) {
ServerSocket server = null;
int port = Integer.parseInt(PropertiesUtil.loadProperties(
"E:\\JavaWeb\\A\\src\\com\\test\\javaSe02\\tcp.properties")
.getProperty("port"));
try {
server = new ServerSocket(port);
} catch (IOException e) {
e.printStackTrace();
}
PrintStream out = null;
BufferedReader br = null;
while (true) {
Socket client = null;
try {
client = server.accept();
br = new BufferedReader(new InputStreamReader(
client.getInputStream()));
out = new PrintStream(client.getOutputStream());
boolean flag = true;
while (flag) {
String str = br.readLine();
if (str.equals("") || null == str)
flag = false;
else {
if ("bye".equals(str.toLowerCase()))
flag = false;
else
out.println("server echo:" + str);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
//client
package com.test.javaSe02;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.PrintStream;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.Properties;
import com.ctl.util.PropertiesUtil;
public class ECHOClient {
public static void main(String[] args) {
Properties pro = PropertiesUtil
.loadProperties("E:\\JavaWeb\\A\\src\\com\\test\\javaSe02\\tcp.properties");
Socket client = null;
try {
client = new Socket(pro.getProperty("ip"), Integer.parseInt(pro
.getProperty("port")));
} catch (IOException e) {
e.printStackTrace();
}
try {
PrintStream out = null;
boolean flag = true;
while (flag) {
out = new PrintStream(client.getOutputStream());
String str = javax.swing.JOptionPane.showInputDialog("message");
//System.out.println(str);
out.println(str);
if (str.equalsIgnoreCase("bye"))
flag = false;
else {
System.out.println(new BufferedReader(
new InputStreamReader(client.getInputStream())).readLine());
}
}
client.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
//PropertiesUtil
package com.ctl.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
/**
* @deprecated 该类可以用来创建properties资源文件
* @author Administrator
* @see www.ctl.com.cn
* @category SDGHDHSDFG
* @serial dfgds
*/
public class PropertiesUtil {
/**
*
* @param propertityPath
* 资源文件要存放的路径例如H:/mysql.properties
* @param map
* 将要写入的资源放入该map中 HashMap<String, String>
* @param comment
* 对在资源文件的描述
*/
public static void createPropertityFile(String propertityPath, String comment, Map<String, String> map) {
File file = new File(propertityPath);
new File(file.getParent()).mkdirs();
Properties pro = new Properties();
for (Map.Entry<String, String> entry : map.entrySet()) {
pro.setProperty(entry.getKey(), entry.getValue());
}
try {
pro.store(new FileOutputStream(propertityPath), comment);
} catch (Exception e) {
System.err.println("存储properties出错");
}
}
/**
* @param map
* 将要写入的资源放入该map中 HashMap<String, String>
* @param comment
* 对在资源文件的描述
* @param propertityPath
* 资源文件要存放的路径例如H:/mysql.xml
*/
public static void createPropertityXMLFile(String propertityPath, String comment, Map<String, String> map) {
File file = new File(propertityPath);
new File(file.getParent()).mkdirs();
Properties pro = new Properties();
for (Map.Entry<String, String> entry : map.entrySet()) {
pro.setProperty(entry.getKey(), entry.getValue());
}
try {
pro.storeToXML(new FileOutputStream(propertityPath), comment,"utf-8");
} catch (Exception e) {
System.err.println("存储properties出错");
}
}
/**
*
* @param filePath "H:/mysql.properties"
* @return Properties的实例对象
*/
public static Properties loadProperties(String filePath){
Properties pro=new Properties();
try {
pro.load(new FileInputStream(new File(filePath)));
} catch (FileNotFoundException e) {
System.err.println("文件未找到");
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return pro;
}/**
*
* @param filePath "H:/mysql.xml"
* @return Properties的实例对象
*/
public static Properties loadXMLProperties(String filePath){
Properties pro=new Properties();
try {
pro.loadFromXML(new FileInputStream(new File(filePath)));
} catch (FileNotFoundException e) {
System.err.println("文件未找到");
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return pro;
}
/**
* @param args
*/
public static void main(String[] args) {
Map<String, String> map = new HashMap<String, String>();
map.put("a", "value1");
map.put("b", "value2");
map.put("c", "value3");
String path="H:" + File.separator + "a//a/"+File.separator;
createPropertityFile(path+"mysql.properties", "mysql数据库资源配置",
map);
createPropertityXMLFile(path+"mysql.xml", "mysql数据库资源配置",
map);
Properties pro=loadProperties(path+"mysql.properties");
System.out.println(pro);
pro=loadXMLProperties(path+"mysql.xml");
System.out.println(pro);
}
}
ECHO TCP Server Client
最新推荐文章于 2023-06-16 08:56:02 发布
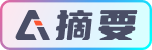