GridView和下拉菜单DropDownList结合:
public SqlDataReader ddlbind() {
string sqlstr = "select distinct 员工性别 from 飞狐工作室";
sqlcon = new SqlConnection(strCon);
SqlCommand sqlcom = new SqlCommand(sqlstr, sqlcon);
sqlcon.Open();
return sqlcom.ExecuteReader();
}
<asp:TemplateField HeaderText="员工性别"> <ItemTemplate> <asp:DropDownList ID="DropDownList1" runat="server" DataSource='<%# ddlbind()%>' DataValueField="员工性别" DataTextField="员工性别"> </asp:DropDownList> </ItemTemplate> </asp:TemplateField> GridView和CheckBox结合: 效果图:
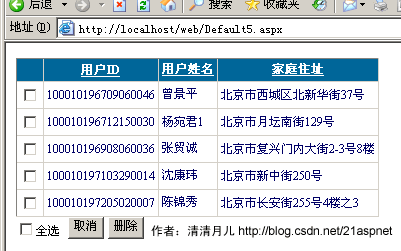 后台代码: public partial class Default5 : System.Web.UI.Page { SqlConnection sqlcon; string strCon = "Data Source=(local);Database=北风贸易;Uid=sa;Pwd=sa"; protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { bind(); } } //全选响应 protected void CheckBox2_CheckedChanged(object sender, EventArgs e) { for (int i = 0; i <= GridView1.Rows.Count - 1; i++) { CheckBox cbox = (CheckBox)GridView1.Rows[i].FindControl("CheckBox1"); if (CheckBox2.Checked == true) { cbox.Checked = true; } else { cbox.Checked = false; } } } //删除所选择的项 protected void Button2_Click(object sender, EventArgs e) { sqlcon = new SqlConnection(strCon); SqlCommand sqlcom; for (int i = 0; i <= GridView1.Rows.Count - 1; i++) { CheckBox cbox = (CheckBox)GridView1.Rows[i].FindControl("CheckBox1"); if (cbox.Checked == true) { string sqlstr = "delete from 飞狐工作室 where 身份证号码='" + GridView1.DataKeys[i].Value + "'"; sqlcom = new SqlCommand(sqlstr, sqlcon); sqlcon.Open(); sqlcom.ExecuteNonQuery(); sqlcon.Close(); } } bind(); } //取消选择 protected void Button1_Click(object sender, EventArgs e) { CheckBox2.Checked = false; for (int i = 0; i <= GridView1.Rows.Count - 1; i++) { CheckBox cbox = (CheckBox)GridView1.Rows[i].FindControl("CheckBox1"); cbox.Checked = false; } } 在asp.net 2.0中,如果要在绑定列中显示比如日期格式等,如果用下面的方法是显示不了的 <asp :BoundField DataField="CreationDate" DataFormatString="{0:M-dd-yyyy}" HeaderText="CreationDate" /> 主要是由于htmlencode属性默认设置为true,已防止XSS攻击,安全起见而用的,所以,可以有以下两种方法解决 1、 <columns> <asp :BoundField DataField="CreationDate" DataFormatString="{0:M-dd-yyyy}" HtmlEncode="false" HeaderText="CreationDate" /> </columns> 将htmlencode设置为false即可 另外的解决方法为,使用模版列 <asp :TemplateField HeaderText="CreationDate" > <edititemtemplate> <asp :Label ID="Label1" runat="server" Text='<%# Eval("CreationDate", "{0:M-dd-yyyy}") %>'> </asp> </edititemtemplate> GridView实现用“...”代替超长字符串: 效果图:
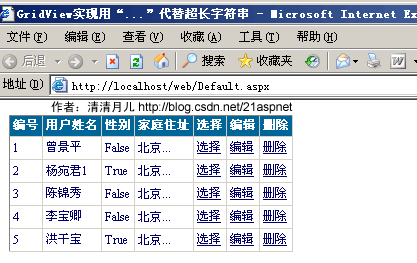 public void bind() { for (int i = 0; i <= GridView1.Rows.Count - 1; i++) { DataRowView mydrv; string gIntro; if (GridView1.PageIndex == 0) { mydrv = myds.Tables["飞狐工作室"].DefaultView[i]; gIntro = Convert.ToString(mydrv["家庭住址"]); GridView1.Rows[i].Cells[3].Text = SubStr(gIntro, 2); } else { mydrv = myds.Tables["飞狐工作室"].DefaultView[i + (5 * GridView1.PageIndex)]; gIntro = Convert.ToString(mydrv["家庭住址"]); GridView1.Rows[i].Cells[3].Text = SubStr(gIntro, 2); } } sqlcon.Close(); } public string SubStr(string sString, int nLeng) { if (sString.Length <= nLeng) { return sString; } string sNewStr = sString.Substring(0, nLeng); sNewStr = sNewStr + "..."; return sNewStr; } GridView一般换行与强制换行: 首先设置<asp:BoundField DataField="家庭住址" HeaderText="家庭住址" ItemStyle-Width="100" /> gridview里有一列绑定的数据很长,显示的时候在一行里面显示,页面拉得很宽。 原因是连续英文段为一个整体导致的,在RowDataBound中添加上了一句e.Row.Cells[2].Style.Add("word-break", "break-all")就可以。 如果要给所有的列增加此属性: protected void Page_Load(object sender, EventArgs e) { //正常换行 GridView1.Attributes.Add("style", "word-break:keep-all;word-wrap:normal"); //下面这行是自动换行 GridView1.Attributes.Add("style", "word-break:break-all;word-wrap:break-word"); if (!IsPostBack) { bind();//调用数据绑定即可 } } 总之:善用CSS的word-break:break-all;word-wrap:break-word属性即可,这个属性是通用的对于顽固的南换行问题都可以解决,不局限于GridView。
GridView固定表头 <style> .Freezing { position:relative ; table-layout:fixed; top:expression(this.offsetParent.scrollTop); z-index: 10; } .Freezing th{text-overflow:ellipsis;overflow:hidden;white-space: nowrap;padding:2px;} </style>
<asp:GridView> <HeaderStyle BackColor="#006699" Font-Bold="True" ForeColor="White" CssClass="Freezing"/> </asp:GridView>
GridView突出显示某一单元格(例如金额低于多少,分数不及格等)
效果图:
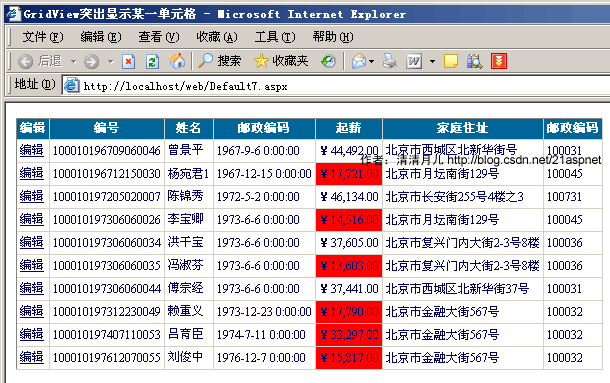
解决方案:主要是绑定后过滤 GridView1.DataBind(); for (int i = 0; i <= GridView1.Rows.Count - 1; i++) { DataRowView mydrv = myds.Tables["飞狐工作室"].DefaultView[i]; string score = Convert.ToString(mydrv["起薪"]); if (Convert.ToDouble(score) < 34297.00)//大家这里根据具体情况设置可能ToInt32等等 { GridView1.Rows[i].Cells[4].BackColor = System.Drawing.Color.Red; } }
GridView加入自动求和求平均值小计
效果图: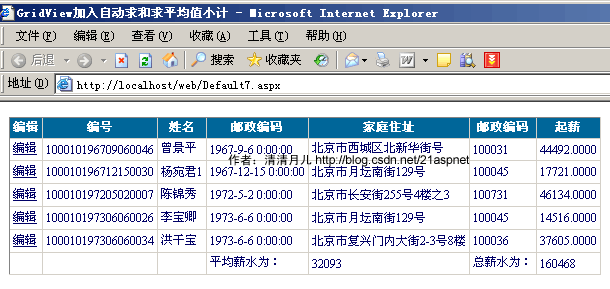 解决方案: private double sum = 0;//取指定列的数据和,你要根据具体情况对待可能你要处理的是int protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e) { if (e.Row.RowIndex >= 0) { sum += Convert.ToDouble(e.Row.Cells[6].Text); } else if (e.Row.RowType == DataControlRowType.Footer) { e.Row.Cells[5].Text = "总薪水为:"; e.Row.Cells[6].Text = sum.ToString(); e.Row.Cells[3].Text = "平均薪水为:"; e.Row.Cells[4].Text = ((int)(sum / GridView1.Rows.Count)).ToString(); } }
|
|