计算长方体、四棱锥的表面积和体积
Time Limit: 1000MS
Memory Limit: 65536KB
Problem Description
计算如下立体图形的表面积和体积。
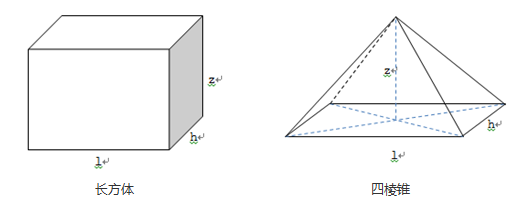
从图中观察,可抽取其共同属性到父类Rect中:长度:l 宽度:h 高度:z
在父类Rect中,定义求底面周长的方法length( )和底面积的方法area( )。
定义父类Rect的子类立方体类Cubic,计算立方体的表面积和体积。其中表面积area( )重写父类的方法。
定义父类Rect的子类四棱锥类Pyramid,计算四棱锥的表面积和体积。其中表面积area( )重写父类的方法。
输入立体图形的长(l)、宽(h)、高(z)数据,分别输出长方体的表面积、体积、四棱锥的表面积和体积。
Input
输入多行数值型数据(double);
每行三个数值,分别表示l h z
若输入数据中有负数,则不表示任何图形,表面积和体积均为0。
Output
行数与输入相对应,数值为长方体表面积 长方体体积 四棱锥表面积 四棱锥体积(中间有一个空格作为间隔,数值保留两位小数)
Example Input
1 2 3 0 2 3 -1 2 3 3 4 5
Example Output
22.00 6.00 11.25 2.00 0.00 0.00 0.00 0.00 0.00 0.00 0.00 0.0094.00 60.00 49.04 20.00
import java.text.DecimalFormat; import java.util.Scanner; class rectangle { double x, y, z; public rectangle(double x1, double y1,double z1) { x = x1; y = y1; z = z1; } double area() { return (x*y*2+x*z*2+y*z*2); } double v() { return (x*y*z); } double area1() { return x*y*z/3; } double v1() { double s1 = x*y; double c1 = Math.sqrt((y/2)*(y/2)+z*z); double c2 = Math.sqrt((x/2)*(x/2)+z*z); double s2 = c1*x; double s3 = c2*y; return s1+s2+s3; } }; public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); while (sc.hasNext()) { DecimalFormat two = new DecimalFormat("#0.00"); double a = sc.nextDouble(); double b = sc.nextDouble(); double c = sc.nextDouble(); if(a<=0||b<=0||c<=0) { System.out.println("0.00 0.00 0.00 0.00"); } else { rectangle rectangle = new rectangle(a,b,c); System.out.println(two.format(rectangle.area())+" "+two.format(rectangle.v())+" "+two.format(rectangle.v1())+" "+two.format(rectangle.area1())); } } } }