一、基于C++类模板定义一个链表,管理学生成绩信息( 学号和成绩),进行排序后再输出。
#include
#include
using namespace std;
template
class list{
struct node{
T data;
node *next;
}
*head, *tail;
public:
list(){
head = tail = NULL;
};
void Insert(T *item){
node *pn;
pn = new node;
pn->next + head;
pn->data = *item;
head = pn;
if (tail == NULL){
tail = pn;
}
};
void Append(T *item){
node *pn;
pn = new node;
pn->next = NULL;
pn->data = *item;
if (tail == NULL){
head = tail = pn;
}
else{
tail->next = pn;
tail = pn;
}
};
T Get(){
if (head == NULL){
return 0;
}
T tmp = head->data;
node *pn = head;
if (head->next == NULL){
head = tail = NULL;
}
else{
head = head->next;
}
delete(pn);
return tmp;
}
};
class Student{
public:
int num;
int score;
};
int main(){
Student s;
list
link1;
list
link2; for (int i = 0; i < 5; i++){ cin >> s.num >> s.score; link2.Insert(&s); link1.Append(&s.score); } cout<<"result"<
程序结果
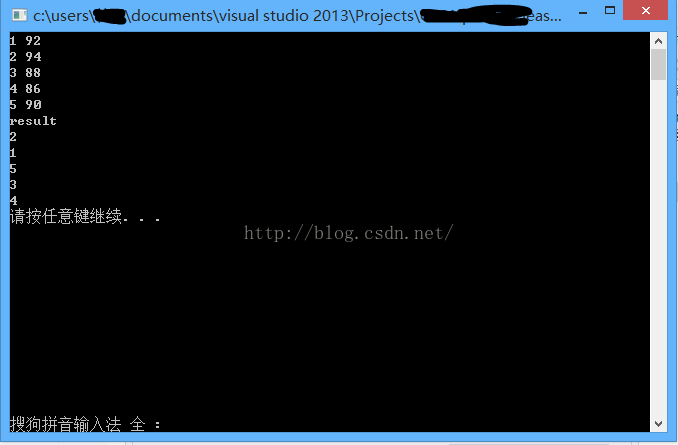
二、编写程序,从键盘输入若干整形数据,以单链表的形式存储并按升序排列,再向其中插入任一整形数据,重新按照升序方式输出。
#include
using namespace std;
class List{
protected:
int length;
public:
int GetLength(){
return length;
}
virtual int Get(int i) = 0;
virtual bool Set(int x, int i) = 0;
virtual void MakeEmpty() = 0;
virtual bool Insert(int value, int i) = 0;
virtual int Remove(int i) = 0;
virtual int RemoveValue(int value) = 0;
};
class Node{
public:
Node(){
next = NULL;
}
Node(const int &item, Node *next1 = NULL){
data = item;
next = next1;
}
int data;
Node *next;
};
class Linklist :public List{
protected:
Node *head;
public:
Node *GetHead(){
return head;
}
Node *GetNext(Node &n){
if (n.next == head){
n.next->next;
}
else{
return n.next;
}
}
int Get(int i){
Node *p = Find(i);
return p->data;
}
bool Set(int x, int i){
Node *p = Find(i);
if (p == NULL || p == head){
return false;
}
else{
p->data = x;
}
return true;
}
Node *Find(int i){
if (i<0 || i>length){
return NULL;
}
if (i=0){
return head;
}
Node *p = head->next;
int j = 1;
while(p!=NULL&&j<1){
p = p->next;
j++;
}
return p;
}
Node *FindValue(int value){
Node *p = head->next;
int i = 1;
while (i++ <= length&&p->data!=value){
p = p->next;
}
return p;
}
void MakeEmpty(){
Node *q = head->next;
int i = 1;
while (i++ <= length){
head->next=q->next;
delete q;
q = head->next;
}
length = 0;
}
virtual bool Insert(int value, int i) = 0;
virtual int Remove(int i) = 0;
virtual int RemoveValue(int value) = 0;
};
class Singlelist:public Linklist{
public:
Singlelist(){
head = new Node;
length = 0;
}
Singlelist(Singlelist &l){
Copy(l);
}
~Singlelist(){
MakeEmpty();
delete head;
}
Singlelist &Copy(Singlelist &l){
Node *p, *q, *r;
head = NULL;
length = l.length;
if (!l.head){
return *this;
}
head = new Node;
if (!l.head){
return *this;
}
head->data = (l.head)->data;
head-> next = NULL;
r = NULL;
p = head;
q = l.head->next;
while (q){
r = new Node;
if (!r){
return *this;
}
r->data = q->data;
r->next = NULL;
p->next = r;
p = p->next;
q = q->next;
}
return *this;
}
bool Insert(int value, int i){
Node *p = Find(i - 1);
if (p == NULL){
return false;
}
Node *newnode = new Node(value, p->next);
p->next = newnode;
length++;
return true;
}
int Remove(int i){
Node *p = Find(i - 1),*q;
q = p->next;
p->next = q->next;
int value = q->data;
delete q;
length--;
return value;
}
int RemoveValue(int value){
Node *p = head, *q;
while (p->next != NULL&&p->next->data != value){
p = p->next;
}
q = p->next;
p->next = q->next;
delete q;
length--;
return value;
}
Singlelist &operator=(Singlelist &l){
if (head){
MakeEmpty();
}
Copy(l);
return *this;
}
friend ostream &operator << (ostream &out, Singlelist &l){
Node *p = l.head->next;
while (p){
out << p->data << " ";
p->next;
}
out << endl;
return out;
}
void sort(){
Node *tmp = head;
while (tmp != NULL){
Node *q = tmp->next;
while (q != NULL){
if (q->data > tmp->data){
int r = tmp->data;
tmp->data = q->data;
q->data = r;
}
}
tmp = tmp->next;
}
}
};
int main(){
Singlelist a;
int i,t,x,length;
cout << "input length"<
> length;
cout << "input data" << endl;
for (i = 0; i <= length; i++){
cin >> t;
a.Insert(t, i);
}
a.sort();
cout << "after sort" << endl;
cout << a << endl;
cout << "input another one" << endl;
cin >> x;
a.Insert(x, length++);
cout << "after sort" << endl;
cout<
<
结果
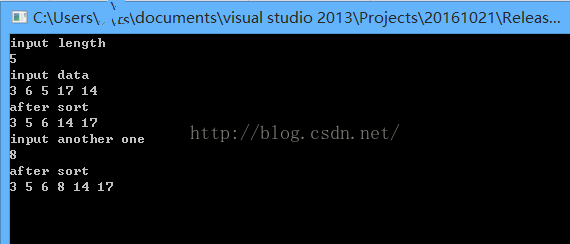