Json工具网站
https://www.bejson.com/json2javapojo/new
https://www.sojson.com/
Json字符串
{
“service”: {
“count”: “20”,
“item”: [
{
“id”: “EES_SS1830738”,
“option”: [
{
“description”: “答案”,
“id”: “1”
},
{
“description”: “答案2”,
“id”: “2”
},
{
“description”: “答案2”,
“id”: “3”
},
{
“description”: “建议书”,
“id”: “4”
}
],
“question”: “题目1”,
“rightanswer”: “3”,
“type”: “1”,
“value”: “5”
},
{
“id”: “EES_MS0654246”,
“option”: [
{
“description”: “12321321”,
“id”: “1”
},
{
“description”: "12321321 ",
“id”: “2”
},
{
“description”: “12321312”,
“id”: “3”
},
{
“description”: “12321”,
“id”: “4”
}
],
“question”: “题目”,
“rightanswer”: “1,3,4”,
“type”: “2”,
“value”: “5”
},
{
“id”: “EES_JU0561902”,
“option”: [
{
“description”: “是”,
“id”: “1”
},
{
“description”: “否”,
“id”: “0”
}
],
“question”: “12312312”,
“rightanswer”: “0”,
“type”: “3”,
“value”: “5”
}
]
}
}
在这里插入代码片
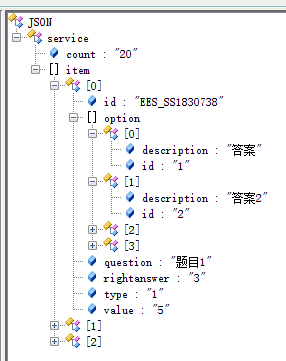
## JsonRootBean
public class JsonRootBean {
private Service service;
public void setService(Service service) {
this.service = service;
}
public Service getService() {
return service;
}
}
## Service
public class Service {
private String count;
private List<Item> item;
public void setCount(String count) {
this.count = count;
}
public String getCount() {
return count;
}
public void setItem(List<Item> item) {
this.item = item;
}
public List<Item> getItem() {
return item;
}
}
## Item
public class Item {
private String id;
private List<Option> option;
private String question;
private String rightanswer;
private String type;
private String value;
//getter
//setter
}
## Option
public class Option {
private String description;
private String id;
public void setDescription(String description) {
this.description = description;
}
public String getDescription() {
return description;
}
public void setId(String id) {
this.id = id;
}
public String getId() {
return id;
}
}
## Controller
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.carlo.test.spboottest.bejson.JsonRootBean;
import com.carlo.test.spboottest.bejson.Service;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
@RestController
public class HelloController {
@RequestMapping("/hello")
public String index() {
return "Hello World";
}
@PostMapping("/add")
public String add(@RequestBody JsonRootBean jsonRootBean){
JsonRootBean jrb = new JsonRootBean();
jrb = jsonRootBean;
ObjectMapper mapper=new ObjectMapper();//定义一个转化对象
String json="";
try {
json = mapper.writeValueAsString(jrb);
} catch (JsonProcessingException e) {
e.printStackTrace();
}
System.out.println(json);
return json;
}
@PostMapping("/add1")
public String add1(@RequestBody Service jsonRootBean){
Service jrb = new Service();
jrb = jsonRootBean;
ObjectMapper mapper=new ObjectMapper();//定义一个转化对象
String json="";
try {
json = mapper.writeValueAsString(jrb);
} catch (JsonProcessingException e) {
e.printStackTrace();
}
System.out.println(json);
return json;
}
}
## POSMAN发送post请求
http://localhost:8080/add1
返回结果:
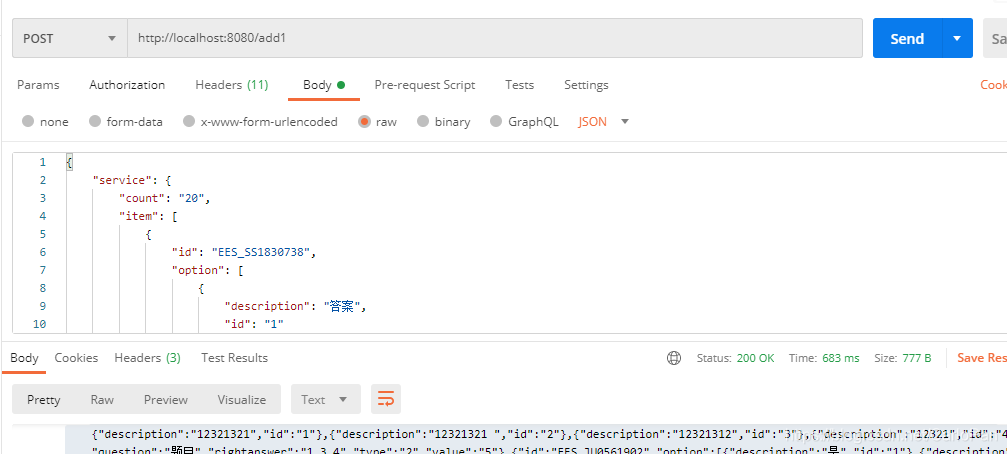
前端传入Json 不包括option时:
{
"count": "20",
"item": [
{
"id": "EES_SS1830738",
"question": "题目1",
"rightanswer": "3",
"type": "1",
"value": "5"
},
{
"id": "EES_MS0654246",
"question": "题目",
"rightanswer": "1,3,4",
"type": "2",
"value": "5"
},
{
"id": "EES_JU0561902",
"question": "12312312",
"rightanswer": "0",
"type": "3",
"value": "5"
}
]
}
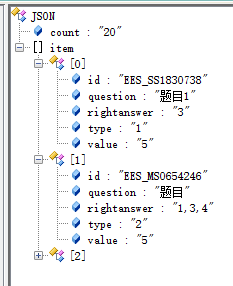
后端收到的结果,option为null
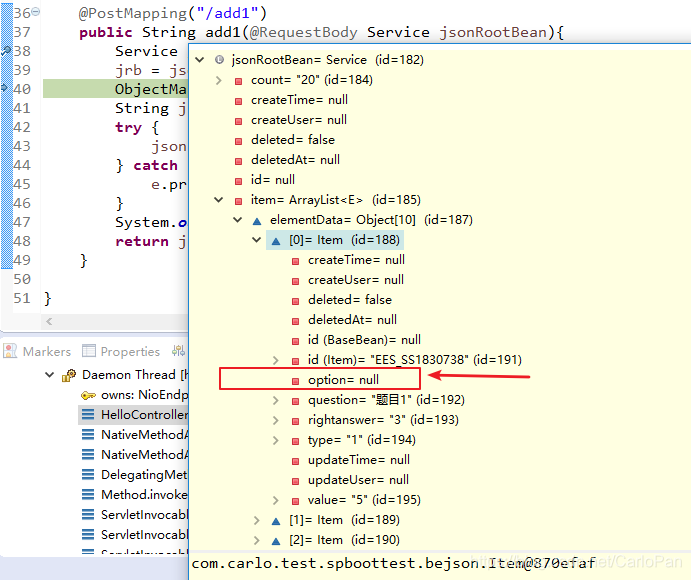