装饰器模式
1. 介绍
2. UML 演示
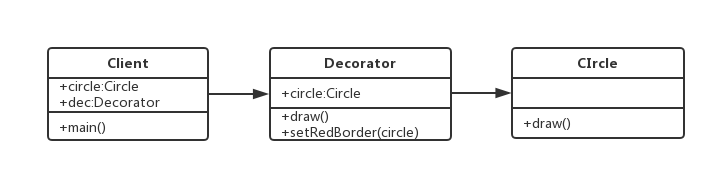
3. 代码演示
class Circle {
draw() {
console.log('画圆形')
}
}
class Decorator {
constructor(circle) {
this.circle = new Circle()
}
draw() {
this.circle.draw()
this.setDecorator(circle)
}
setDecorator(circle) {
console.log('设置为红色边框')
}
}
let circle = new Circle()
circle.draw()
console.log('--------')
let decorator = new Decorator()
decorator.draw()
4. 场景
4.1 装饰类
@testDec
class Demo {
}
function testDec(target) {
target.isDec = true
}
alert(Demo.isDec)
@decorator
class A {}
class A {}
A = decorator(A) || A
function testDec(isDec) {
return function(target) {
target.isDec = isDec
}
}
@testDec(true)
class Demo {
}
alert(Demo.isDec)
4.2 mixin 示例
function mixins(...list) {
return function(target) {
Object.assign(target.proto, ...list)
}
}
const Foo = {
foo() {
alert('foo')
}
}
@mixins(Foo)
class MyClass {}
let obj = new MyClass()
obj.foo()
4.3 装饰方法(1)
class Person {
constructor() {
this.first = 'A'
this.last = 'B'
}
@readonly
name() {
return `${this.first} ${this.last}`
}
}
var p = new Person()
console.log(p.name())
function readonly(target, name, descriptor) {
descriptor.writable = false
return descriptor
}
readonly(Person.prototype, 'name', descriptor)
Object.defineProperty(Person.prototype, 'name', descriptor)
4.4 装饰方法(2)
class Math {
@log
add(a, b) {
return a + b
}
}
let math = new Math()
const result = math.add(2, 4)
console.log(result)
function log(target, name, descriptor) {
var oldValue = descriptor.value
descriptor.value = function() {
console.log(`Calling ${name} with`, arguments)
return oldValue.apply(this, arguments)
}
return descriptor
}
4.5 core-decorators
- 第三方开源 lib
- 提供常用的装饰器
- 点击此处查阅文档
import { readonly } from 'core-decorators'
class Person {
@readonly
name() {
return 'zhang'
}
}
let p = new Person()
alert(p.name())
5. 设计原则
- 将现有对象和装饰器分离,两者独立存在
- 符合开放封闭原则