题目
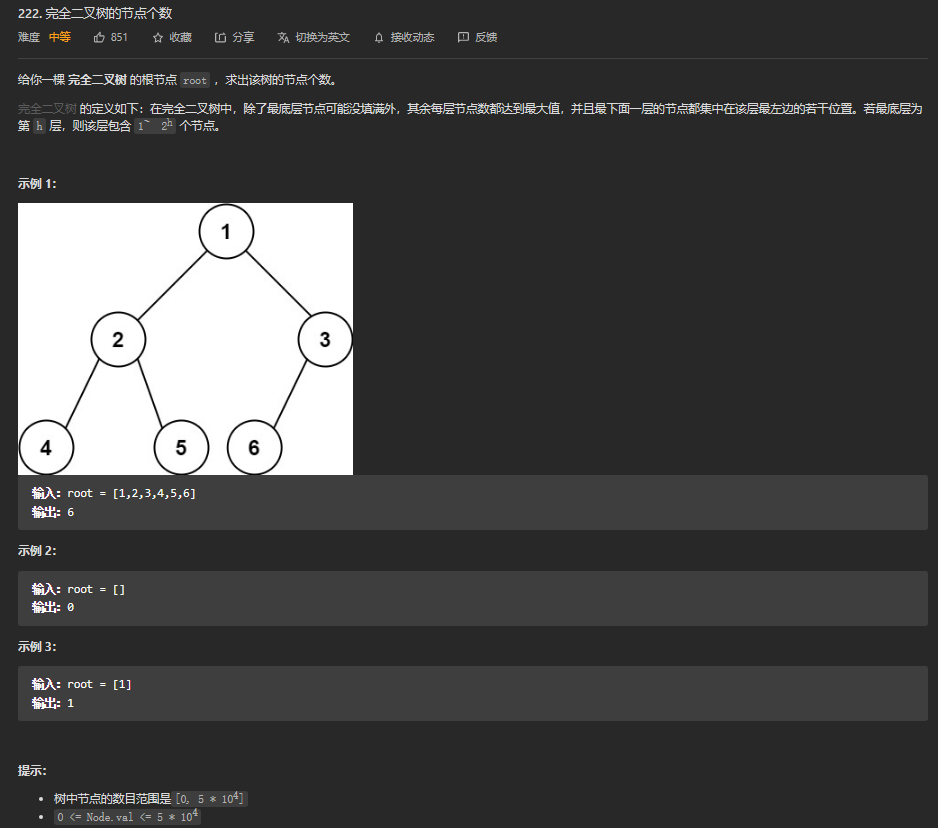
题解
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* struct TreeNode *left;
* struct TreeNode *right;
* };
*/
int countNodes(struct TreeNode* root){
//层序遍历记录个数
int SIZE = 50000/2;
struct TreeNode* temp[SIZE];
int front = 0,rear = 0;
//根结点进队列
if(root == NULL){
return 0;
}else{
temp[rear++] = root;
}
//遍历过程
int count = 0;
while(front != rear){
struct TreeNode* one = temp[front];
front = (front+1)%SIZE;
count++;
if(one->left != NULL){
temp[rear] = one->left;
rear = (rear+1)%SIZE;
}
if(one->right != NULL){
temp[rear] = one->right;
rear = (rear+1)%SIZE;
}
}
return count;
}
要点
使用循环队列来进行树的层序遍历,循环队列需要有队列,队列长度,头指针,尾指针四个要素。
循环队列指针的移动是rear = (rear+1) %SIZE;