目录
一. 关于图的深度优先遍历
与广度优先遍历不同,深度优先遍历(Depth-First-Search,DFS)类似于树的先序遍历。如其中名称所暗含的意思一样,这种遍历算法所遵循的遍历策略是尽可能“深”的搜索一个图。
深度遍历的全过程其实从一个节点开始,访问节点
,然后寻找这个节点的相邻没有被访问的节点
,然后访问节点
,从节点
开始继续遍历
周围的相邻没有被访问的节点
,然后又从节点
开始访问以此类推下去,直到某一个节点
的周围所有节点都被访问过,然后退回到
的上一个节点继续寻找没有被访问过的节点,假如又是都被访问过,则继续向上退回上一级节点,直到所有节点均被访问完成。
以无向图G所示
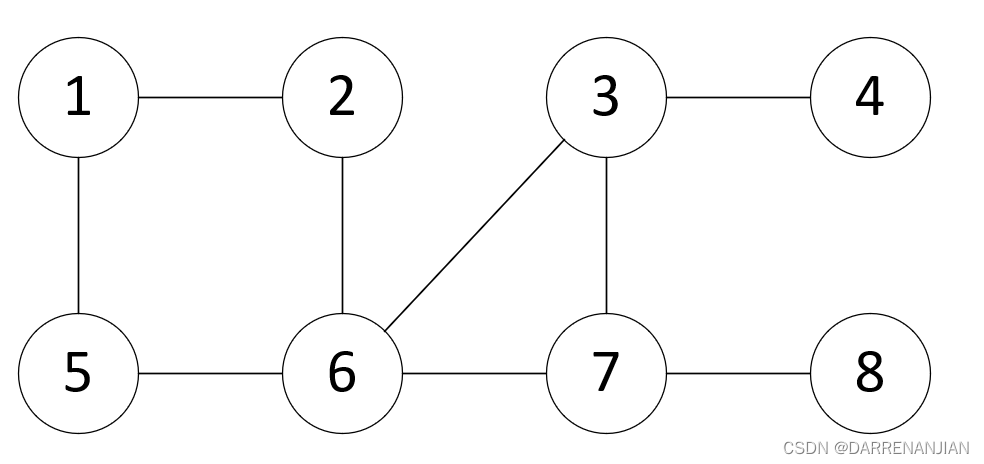
从1号节点开始遍历,不需要回溯所能到达的极限节点。
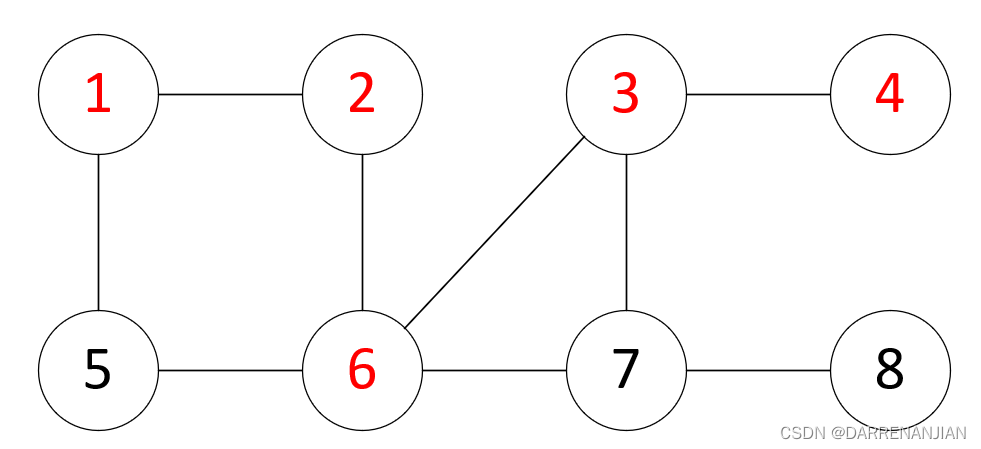
回溯到3号节点,然后访问7,8号节点。
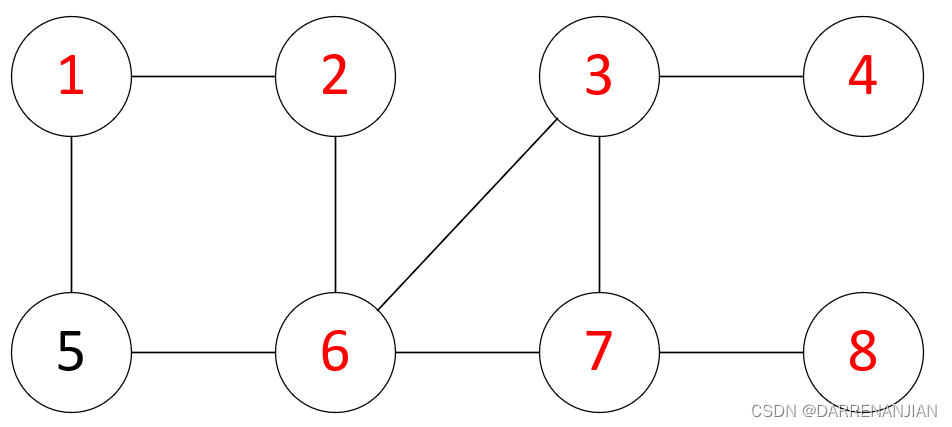
回溯到6号节点,然后访问5号节点。
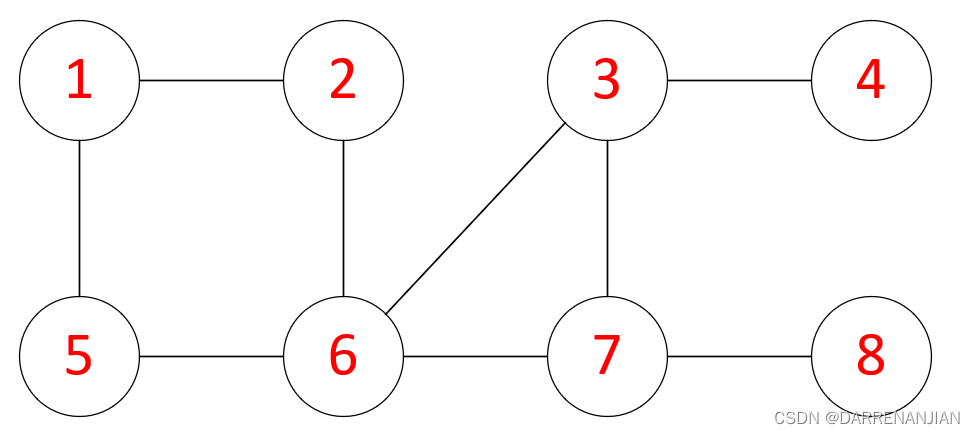
二. 如何实现图的深度优先遍历
介绍完了深度优先遍历的算法,我们现在来实现这个算法,首先这里有一个回溯动作,即需要存储遍历过程中的节点的信息,故在这里我们想到用栈S来存储(由于回溯其本质就是入栈的元素出栈的过程),初始化栈为空;接着需要一个访问矩阵用来记录节点是否被访问,初始化全为false;接着用result记录结果;最后决定从哪一个节点开始(假设以上图G所示,从1号节点开始深度遍历)。
step1:将1号节点的访问位设置为true,result记录结果1;将1号节点压入栈S。
step2:寻找1号节点的没有被访问过且直接相连的节点,这个节点是2号节点;设置2号节点的访问位为true,压入栈,result记录结果。
step3:2号节点有相邻节点且没有被访问过的节点6,同样的道理,压入栈,result记录结果。
step4:访问节点3,压入栈;访问节点4,压入栈。
step5:当节点4 没有邻接节点可以访问的时候,节点4出栈,这时候栈不为空,再出栈3号节点,在3号节点的寻找未被访问过的邻接节点
step6:找到节点7,压入栈。
step7:在7的节点的周围寻找没有被访问且直接相连的节点8,压入栈。
step:8:8的周围没有邻接节点,8号节点出栈,这时候栈不为空,再出栈7号节点,在7号节点的周围寻找没有被访问且直接相连的节点。
step9:没有找到,再出栈,在6号节点的周围寻找没有被访问过的节点,这时找到节点5,访问完成。
三. 深度优先遍历的代码实现
1. 初始化:
构造一个栈tempStack用来存储回溯的过程;构造一个字符串resultString用来记录访问的结果;tempNumNodes用来记录邻接矩阵的维数;tempVisitedArray用来记录访问过的节点的数值,初始化都为false;从paraStartIndex节点开始,设置其访问矩阵为true,resultString记录结果,将paraStartIndex节点入栈,设置正在访问的参数tempIndex=paraStartIndex,下一个访问节点的参数tempNext。
ObjectStack tempStack = new ObjectStack();
String resultString = "";
int tempNumNodes = connectivityMatrix.getRows();
boolean[] tempVisitedArray = new boolean[tempNumNodes];
//Initialize the stack.
//Visit before push.
tempVisitedArray[paraStartIndex] = true;
resultString += paraStartIndex;
tempStack.push(new Integer(paraStartIndex));
System.out.println("Push " + paraStartIndex);
System.out.println("Visited " + resultString);
//Now visit the rest of the graph.
int tempIndex = paraStartIndex;
int tempNext;
Integer tempInteger;
2. 核心代码:
第一层循环用来使所有节点都被遍历过,下一个节点还没有寻找,故设置tempNext的值为-1;开始第二层for循环,寻找没有被访问且直接和正在被访问的节点tempIndex连通的节点。找到之后设置访问位为true,result记录结果,并且将这个节点压入栈,tempNext记录这个节点(下一个要访问的值),接着跳出for循环。
判断tempNext的值是否发生改变,若发生了改变则tempIndex(正在访问节点)赋值为tempNext,然后再进行第一次大循环。若没有发生改变(意味着这个节点的周围已经没有未被访问过且直接相连的节点了),将这个正在访问节点出栈。这时再判断栈是否为空,为空结束循环遍历结束;不为空的话tempIndex指向这时栈顶的第一个元素,然后又开始第一次大循环。
放眼整个核心代码段,其实就是从一个顶点开始,压入栈;接着寻找这个节点的邻接节点(未被访问过),找到就压入栈,没有找到就出栈,然后指向这时栈顶的元素,继续寻找邻接节点(未被访问过),直到栈为空。
while (true){
//Find an unvisited neighbor.
tempNext = -1;
for (int i = 0; i < tempNumNodes; i ++) {
if (tempVisitedArray[i]) {
continue; //Already visited.
}//Of if
if (connectivityMatrix.getData()[tempIndex][i] == 0) {
continue; //Not directly connected.
}//Of if
//Visit this one.
tempVisitedArray[i] = true;
resultString += i;
tempStack.push(new Integer(i));
System.out.println("Push " + i);
tempNext = i;
//One is enough.
break;
}//Of for i
if (tempNext == -1) {
tempInteger = (Integer) tempStack.pop();
System.out.println("Pop " + tempInteger);
if (tempStack.isEmpty()) {
// No unvisited neighbor. Backtracking to the last one
// stored in the stack.
// Attention: This is the terminate condition!
break;
} else {
// Back to the previous node, however do not remove it.
tempInteger = (Integer) tempStack.pop();
tempIndex = tempInteger.intValue();
tempStack.push(tempInteger);
} // Of if
} else {
tempIndex = tempNext;
} // Of if
}//Of while
四. 代码展示
主函数:
package Day_34;
import Day_32.Graph;
public class demo1 {
/**
*********************
* The entrance of the program.
*
* @param args
* Not used now.
*********************
*/
public static void main(String args[]) {
System.out.println("Hello!");
Graph tempGraph = new Graph(3);
// System.out.println(tempGraph);
// Unit test.
tempGraph.depthFirstTraversalTest();
}// Of main
}
调用类:
package Day_32;
import Day_25.ObjectStack;
import Day_22.CircleObjectQueue;
import Day_31.IntMatrix;
public class Graph {
/**
* The connectivity matrix.
*/
IntMatrix connectivityMatrix;
/**
*********************
* The first constructor.
*
* @param paraNumNodes
* The number of nodes in the graph.
*********************
*/
public Graph(int paraNumNodes) {
connectivityMatrix = new IntMatrix(paraNumNodes, paraNumNodes);
}// Of the first constructor
/**
*********************
* The second constructor.
*
* @param paraMatrix
* The data matrix.
*********************
*/
public Graph(int[][] paraMatrix) {
connectivityMatrix = new IntMatrix(paraMatrix);
}// Of the second constructor
/**
*********************
* Overrides the method claimed in Object, the superclass of any class.
*********************
*/
public String toString() {
String resultString = "This is the connectivity matrix of the graph.\r\n"
+ connectivityMatrix;
return resultString;
}// Of toString
/**
*********************
* Get the connectivity of the graph.
*
* @throws Exception
* for internal error.
*********************
*/
public boolean getConnectivity() throws Exception {
// Step 1. Initialize accumulated matrix: M_a = I.
IntMatrix tempConnectivityMatrix = IntMatrix.getIdentityMatrix(connectivityMatrix.getData().length);
// Step 2. Initialize M^1.
IntMatrix tempMultipliedMatrix = new IntMatrix(connectivityMatrix);
// Step 3. Determine the actual connectivity.
for (int i = 0; i < connectivityMatrix.getData().length - 1; i++) {
// M_a = M_a + M^k
tempConnectivityMatrix.add(tempMultipliedMatrix);
// M^k
tempMultipliedMatrix = IntMatrix.multiply(tempMultipliedMatrix, connectivityMatrix);
} // Of for i
// Step 4. Check the connectivity.
System.out.println("The connectivity matrix is: " + tempConnectivityMatrix);
int[][] tempData = tempConnectivityMatrix.getData();
for (int i = 0; i < tempData.length; i++) {
for (int j = 0; j < tempData.length; j++) {
if (tempData[i][j] == 0) {
System.out.println("Node " + i + " cannot reach " + j);
return false;
} // Of if
} // Of for j
} // Of for i
return true;
}// Of getConnectivity
/**
*********************
* Unit test for getConnectivity.
*********************
*/
public static void getConnectivityTest() {
// Test an undirected graph.
int[][] tempMatrix = { { 0, 1, 0 }, { 1, 0, 1 }, { 0, 1, 0 } };
Graph tempGraph2 = new Graph(tempMatrix);
System.out.println(tempGraph2);
boolean tempConnected = false;
try {
tempConnected = tempGraph2.getConnectivity();
} catch (Exception ee) {
System.out.println(ee);
} // Of try.
System.out.println("Is the graph connected? " + tempConnected);
// Test a directed graph.
// Remove one arc to form a directed graph.
tempGraph2.connectivityMatrix.setValue(1, 0, 0);
tempConnected = false;
try {
tempConnected = tempGraph2.getConnectivity();
} catch (Exception ee) {
System.out.println(ee);
} // Of try.
System.out.println("Is the graph connected? " + tempConnected);
}// Of getConnectivityTest
/**
*********************
* Breadth first traversal.
*
* @param paraStartIndex The start index.
* @return The sequence of the visit.
*********************
*/
public String breadthFirstTraversal(int paraStartIndex) {
CircleObjectQueue tempQueue = new CircleObjectQueue();
String resultString = "";
int tempNumNodes = connectivityMatrix.getRows();
boolean[] tempVisitedArray = new boolean[tempNumNodes];
//Initialize the queue.
//Visit before enqueue.
tempVisitedArray[paraStartIndex] = true;
resultString += paraStartIndex;
tempQueue.enqueue(new Integer(paraStartIndex));
//Now visit the rest of the graph.
int tempIndex;
Integer tempInteger = (Integer)tempQueue.dequeue();
while (tempInteger != null) {
tempIndex = tempInteger.intValue();
//Enqueue all its unvisited neighbors.
for (int i = 0; i < tempNumNodes; i ++) {
if (tempVisitedArray[i]) {
continue; //Already visited.
}//Of if
if (connectivityMatrix.getData()[tempIndex][i] == 0) {
continue; //Not directly connected.
}//Of if
//Visit before enqueue.
tempVisitedArray[i] = true;
resultString += i;
tempQueue.enqueue(new Integer(i));
}//Of for i
//Take out one from the head.
tempInteger = (Integer)tempQueue.dequeue();
}//Of while
return resultString;
}//Of breadthFirstTraversal
/**
*********************
* Unit test for breadthFirstTraversal.
*********************
*/
public static void breadthFirstTraversalTest() {
// Test an undirected graph.
int[][] tempMatrix = { { 0, 1, 1, 0 }, { 1, 0, 0, 1 }, { 1, 0, 0, 1}, { 0, 1, 1, 0} };
Graph tempGraph = new Graph(tempMatrix);
System.out.println(tempGraph);
String tempSequence = "";
try {
tempSequence = tempGraph.breadthFirstTraversal(2);
} catch (Exception ee) {
System.out.println(ee);
} // Of try.
System.out.println("The breadth first order of visit: " + tempSequence);
}//Of breadthFirstTraversalTest
/**
*********************
* Depth first traversal.
*
* @param paraStartIndex The start index.
* @return The sequence of the visit.
*********************
*/
public String depthFirstTraversal(int paraStartIndex) {
ObjectStack tempStack = new ObjectStack();
String resultString = "";
int tempNumNodes = connectivityMatrix.getRows();
boolean[] tempVisitedArray = new boolean[tempNumNodes];
//Initialize the stack.
//Visit before push.
tempVisitedArray[paraStartIndex] = true;
resultString += paraStartIndex;
tempStack.push(new Integer(paraStartIndex));
System.out.println("Push " + paraStartIndex);
System.out.println("Visited " + resultString);
//Now visit the rest of the graph.
int tempIndex = paraStartIndex;
int tempNext;
Integer tempInteger;
while (true){
//Find an unvisited neighbor.
tempNext = -1;
for (int i = 0; i < tempNumNodes; i ++) {
if (tempVisitedArray[i]) {
continue; //Already visited.
}//Of if
if (connectivityMatrix.getData()[tempIndex][i] == 0) {
continue; //Not directly connected.
}//Of if
//Visit this one.
tempVisitedArray[i] = true;
resultString += i;
tempStack.push(new Integer(i));
System.out.println("Push " + i);
tempNext = i;
//One is enough.
break;
}//Of for i
if (tempNext == -1) {
tempInteger = (Integer) tempStack.pop();
System.out.println("Pop " + tempInteger);
if (tempStack.isEmpty()) {
// No unvisited neighbor. Backtracking to the last one
// stored in the stack.
// Attention: This is the terminate condition!
break;
} else {
// Back to the previous node, however do not remove it.
tempInteger = (Integer) tempStack.pop();
tempIndex = tempInteger.intValue();
tempStack.push(tempInteger);
} // Of if
} else {
tempIndex = tempNext;
} // Of if
}//Of while
return resultString;
}//Of depthFirstTraversal
/**
*********************
* Unit test for depthFirstTraversal.
*********************
*/
public static void depthFirstTraversalTest() {
// Test an undirected graph.
int[][] tempMatrix = { { 0, 1, 1, 0 }, { 1, 0, 0, 1 }, { 1, 0, 0, 0}, { 0, 1, 0, 0} };
Graph tempGraph = new Graph(tempMatrix);
System.out.println(tempGraph);
String tempSequence = "";
try {
tempSequence = tempGraph.depthFirstTraversal(0);
} catch (Exception ee) {
System.out.println(ee);
} // Of try.
System.out.println("The depth first order of visit: " + tempSequence);
}//Of depthFirstTraversalTest
}
五. 数据测试
无向图G依据对应的邻接矩阵M如下图所示
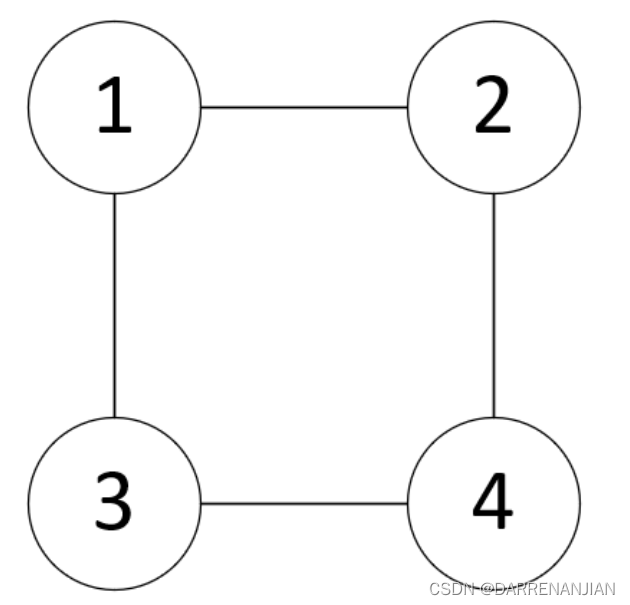
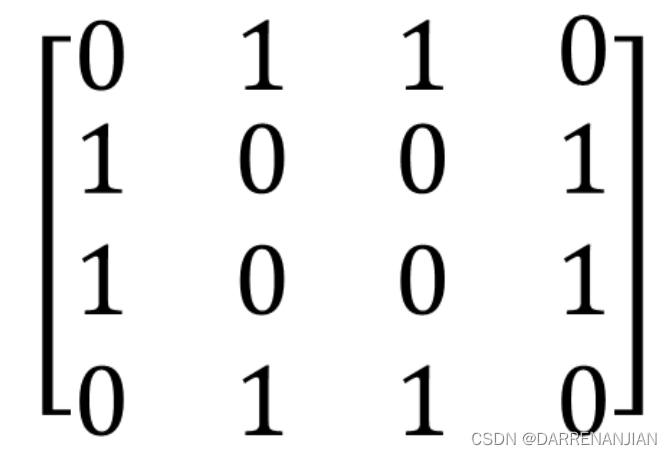
六. 总结与反思
这一小节关键是理解回溯的用法以及怎样实现回溯,在这里我们没有用递归的方式解决,而是采用了效率更高的栈(其实递归本质上就是栈的应用,只不过是系统自主的为你构造一个栈,将每一段调用压入栈,知道得到最后的结果)。