uni-app页面
计算属性
不需要在data中定义
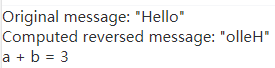
计算属性
<template>
<view>
<view>Original message: "{{ message }}"</view>
<view>Computed reversed message: "{{ reversedMessage }}"</view>
<view>a + b = {{testFun}}</view>
</view>
</template>
<script>
export default {
data() {
return {
message: 'Hello',
a:1,
b:2,
}
},
computed: {
// 计算属性的 getter
reversedMessage: function () {
// `this` 指向 vm 实例
return this.message.split('').reverse().join('')
},
testFun: function () {
return this.a + this.b
}
}
}
</script>
监听属性
需要在data中定义

监听属性
<template>
<view>
<view>fullName: {{ fullName }}</view>
</view>
</template>
<script>
export default {
data() {
return {
firstName: 'Foo',
lastName: 'Bar',
fullName: 'Foo Bar',
}
},
watch: {
firstName: function (oldVal, newVal) {
this.fullName = newVal + ' ' + this.lastName
},
lastName: function (oldVal, newVal) {
this.fullName = this.firstName + ' ' + newVal
}
},
}
</script>
但是使用计算属性会更简洁
<template>
<view>
<view>fullName: {{ fullName }}</view>
</view>
</template>
<script>
export default {
data() {
return {
firstName: 'Foo',
lastName: 'Bar',
}
},
computed: {
fullName: function () {
return this.firstName + ' ' + this.lastName
}
},
}
</script>