Android+本地记事本(上)------实现登陆注册
开发工具:Android Studio3.1.3
数据库:SQLite
本篇博客写作时间:2018年6月28日
总源码下载地址 点击打开链接
若点击无法打开请访问:https://download.csdn.net/download/dear_uu/10505105
前言
:本人刚开始学习Android,若有mistake请留言指正,不胜感激,不足之处还请多多海涵。
转载还请著明出处,谢谢。
一:欢迎界面
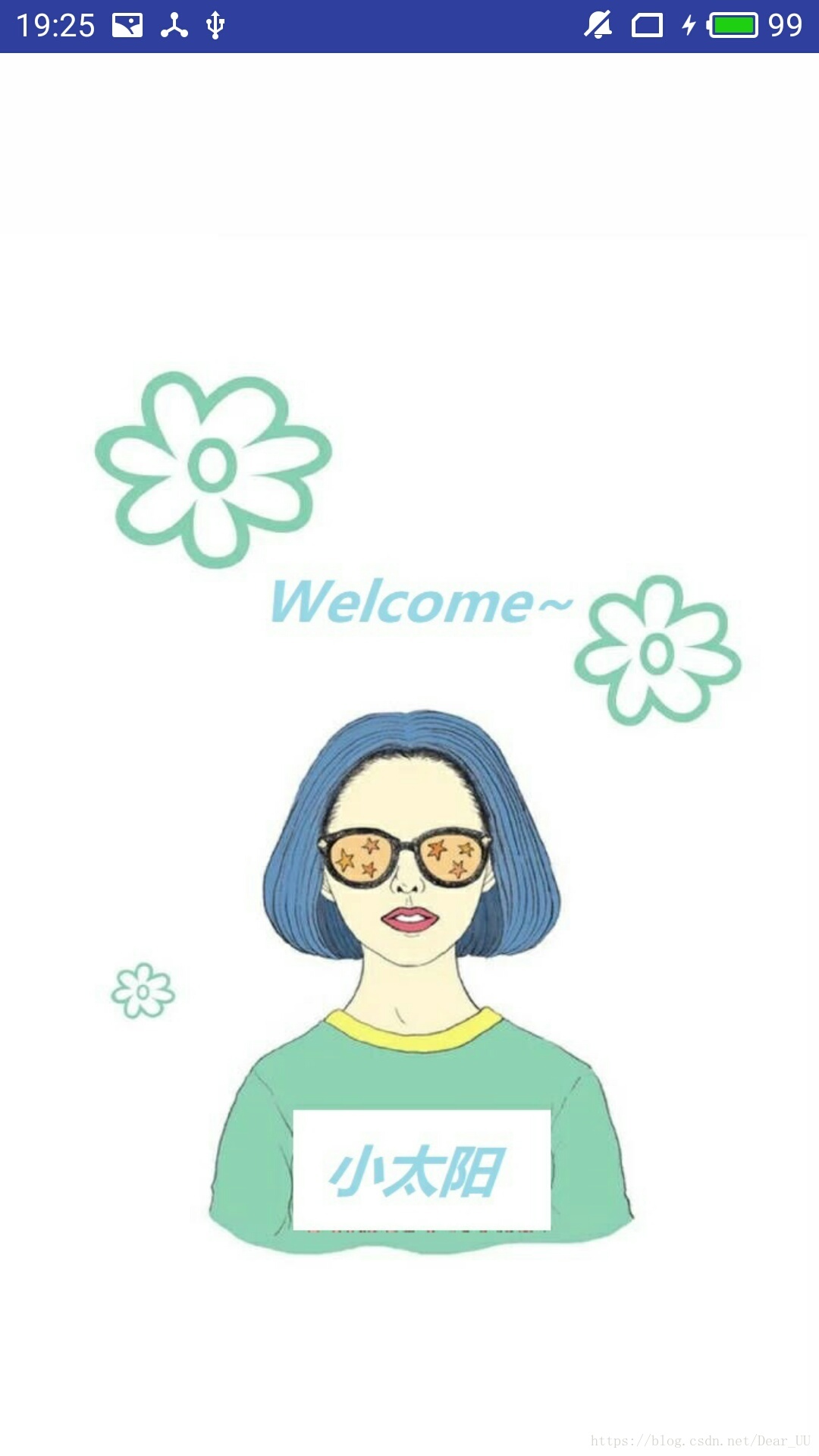
布局:welcome_layout.xml
很简单,就是一个背景图片而已:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/welcome_icon">
</LinearLayout>
活动:WelcomeActivity.java
下面是主要的代码,实现了一个欢迎界面停留2秒,进入登录界面
public class WeclomeActivity extends Activity {
public static final long DELAY_TIME = 2000L;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
this.requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.welcome_layout);
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
startActivity(new Intent(WeclomeActivity.this, LoginActivity.class));
finish();
}
}, DELAY_TIME);
}
}
二: 登录界面
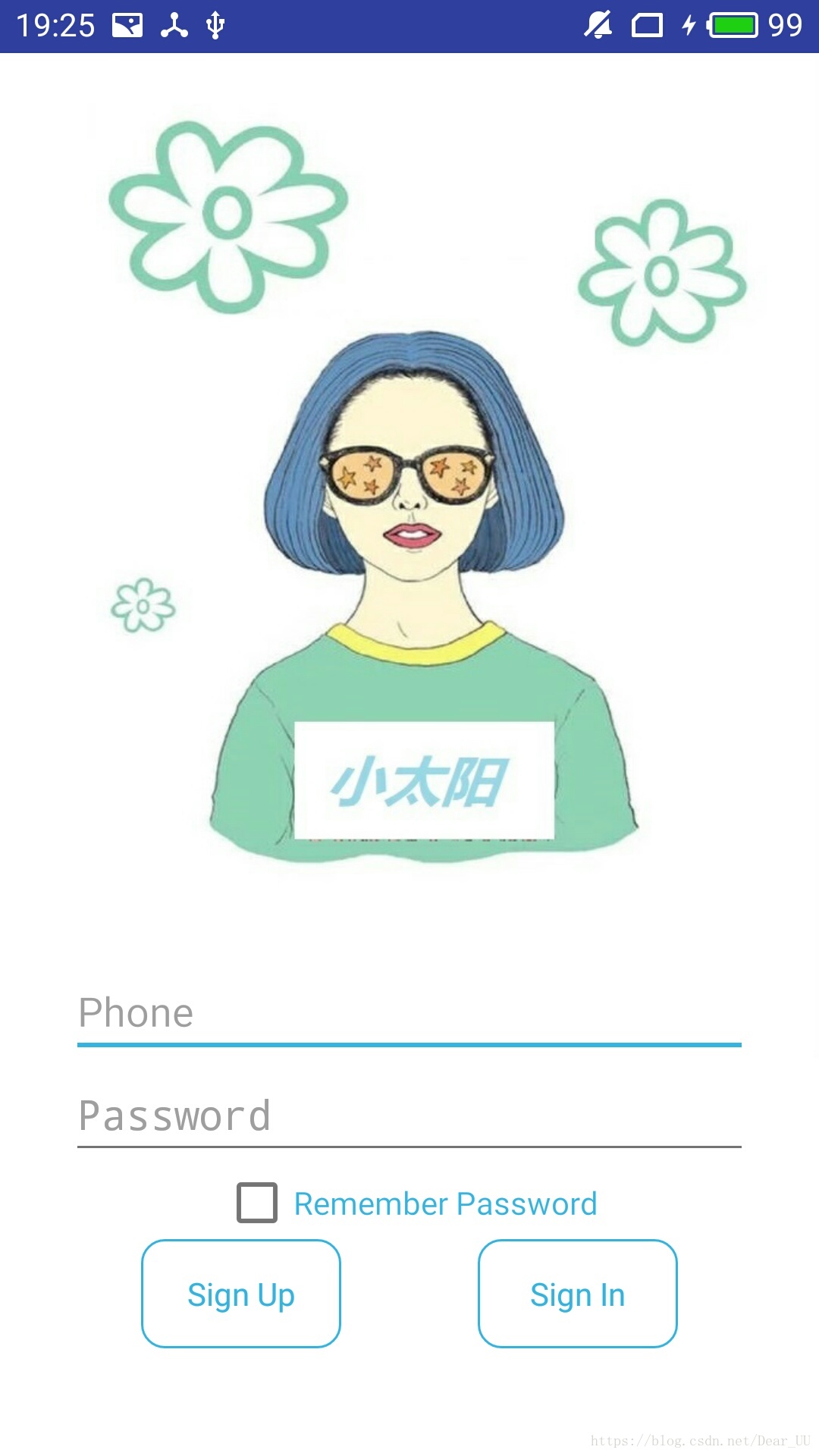
布局:login_layout.xml
从下面的代码不难看出,就是简单的LinearLayout的嵌套了,美化了一下,并修改了系统默认的骚气的粉红色为亮蓝色(style.xml文件中进行修改)。
对Button进行了一个border的封装,这里就不详细说明了。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/loginbg_icon"
android:orientation="vertical"
android:weightSum="1">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="400dp"></LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="100dp"
android:layout_weight="0.73"
android:orientation="vertical">
<EditText
android:id="@+id/phone_text"
android:layout_width="300dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:digits="0,1,2,3,4,5,6,7,8,9"
android:hint="Phone"
android:inputType="number"
android:maxLength="11"
android:textColor="@android:color/holo_blue_light" />
<EditText
android:id="@+id/password_text"
android:layout_width="300dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:digits="0,1,2,3,4,5,6,7,8,9,qwertyuiopasdfghjklzxcvbnm,_,."
android:hint="Password"
android:inputType="numberPassword"
android:maxLength="18"
android:textColor="@android:color/holo_blue_light" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:orientation="horizontal"
android:weightSum="1">
<CheckBox
android:id="@+id/remember_pass"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Remember Password"
android:textColor="@android:color/holo_blue_light" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:orientation="horizontal"
android:weightSum="1">
<Button
android:id="@+id/signUp_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="60dp"
android:background="@drawable/button_selector_draw"
android:text="Sign Up"
android:textAllCaps="false"
android:textColor="@android:color/holo_blue_light" />
<Button
android:id="@+id/signIn_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/button_selector_draw"
android:text="Sign In"
android:textAllCaps="false"
android:textColor="@android:color/holo_blue_light" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
活动:LoginActivity.java
下面是主要的代码,实现了记住密码,感觉没啥好说的,有疑问的话评论中问吧。
public class LoginActivity extends Activity implements View.OnClickListener { private static final int REQUEST_CODE_GO_TO_REGIST = 100; private EditText edit_account, edit_password; private Button btn_signIn, btn_signUp; private String account, password; private CheckBox box_rememberpsw; private DBHelper dbHelper = new DBHelper(this); private SharedPreferences sharedPreferences; private SharedPreferences.Editor editor; private static final long DELAY_TIME = 2000L; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); this.requestWindowFeature(Window.FEATURE_NO_TITLE); setContentView(R.layout.login_layout); init(); } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { switch (requestCode) { case REQUEST_CODE_GO_TO_REGIST: if (resultCode == RESULT_OK) { String name = data.getStringExtra("Phone"); String psw = data.getStringExtra("Password"); edit_account.setText(name); edit_password.setText(psw); } break; } } private void init() { edit_account = (EditText) findViewById(R.id.phone_text); edit_account.setOnEditorActionListener(new TextView.OnEditorActionListener() { @Override public boolean onEditorAction(TextView v, int actionId, KeyEvent event) { return false; } }); edit_password = (EditText) findViewById(R.id.password_text); box_rememberpsw = (CheckBox) findViewById(R.id.remember_pass); box_rememberpsw = (CheckBox) findViewById(R.id.remember_pass); btn_signIn = (Button) findViewById(R.id.signIn_btn); btn_signUp = (Button) findViewById(R.id.signUp_btn); btn_signIn.setOnClickListener(this); btn_signUp.setOnClickListener(this); sharedPreferences = PreferenceManager.getDefaultSharedPreferences(this); boolean isRemember = sharedPreferences.getBoolean("remember_password", false); if (isRemember) { String name = sharedPreferences.getString("Account", ""); String psw = sharedPreferences.getString("Password", ""); edit_account.setText(name); edit_password.setText(psw); box_rememberpsw.setChecked(true); } } @Override public void onClick(View view) { switch (view.getId()) { case R.id.signUp_btn: Intent intent = new Intent(LoginActivity.this, RegisterActivity.class); startActivityForResult(intent, REQUEST_CODE_GO_TO_REGIST); break; case R.id.signIn_btn: if (edit_account.getText().toString().equals("") || edit_password.getText().toString().trim().equals("")) { Toast.makeText(LoginActivity.this, "手机号或密码不能为空!", Toast.LENGTH_SHORT).show(); } else { readUserInfo(); } break; } } private void readUserInfo() { account = edit_account.getText().toString(); password = edit_password.getText().toString().trim(); editor = sharedPreferences.edit(); if (login(account, password)) { if (box_rememberpsw.isChecked()) { editor.putBoolean("remember_password", true); editor.putString("Account", account); editor.putString("Password", password); } else { editor.clear(); } editor.apply(); Toast.makeText(LoginActivity.this, "登录成功!", Toast.LENGTH_SHORT).show(); Intent intent = new Intent(LoginActivity.this, MainActivity.class); intent.putExtra("UserPhone", account); startActivity(intent); finish(); } else { Toast.makeText(LoginActivity.this, "手机号或密码错误,请重新输入!", Toast.LENGTH_SHORT).show(); } } private boolean login(String account, String password) { SQLiteDatabase db = dbHelper.getWritableDatabase(); String sql = "select * from " + USER_TABLE + " where " + PHONE + "=? and " + PASSWORD + "=?"; Cursor cursor = db.rawQuery(sql, new String[]{account, password}); if (cursor.moveToFirst()) { cursor.close(); return true; } return false; } }
三: 注册界面
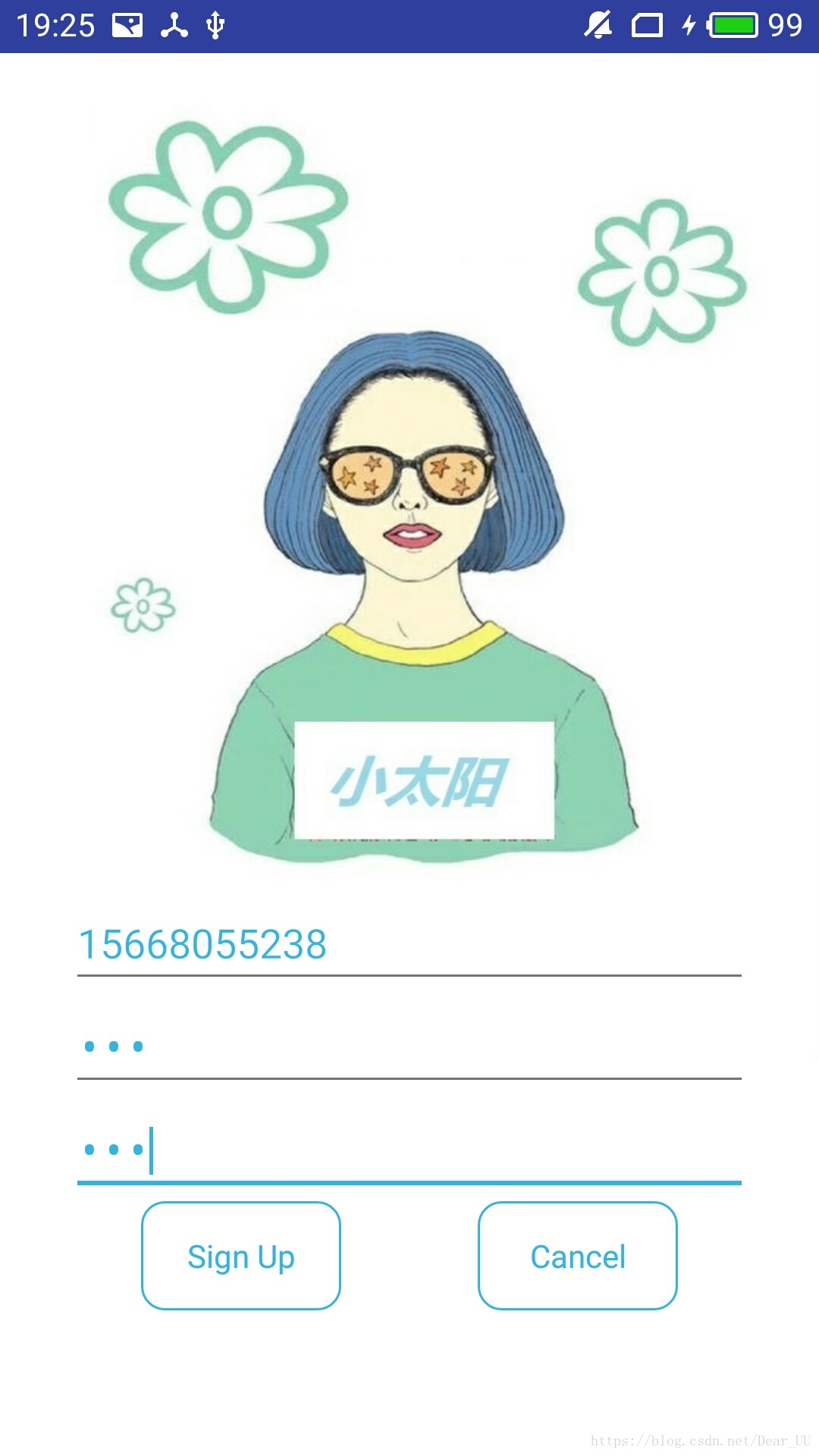
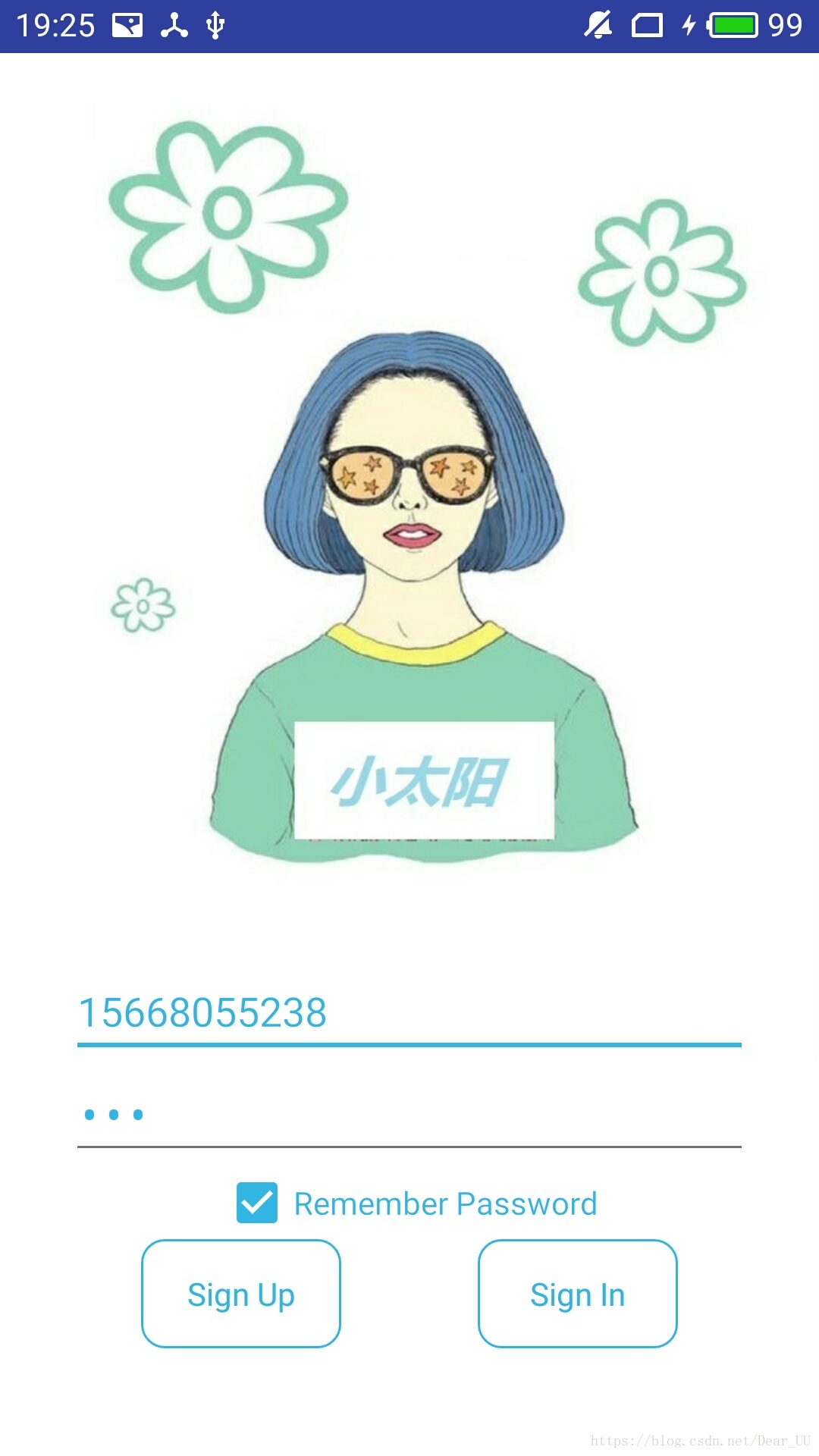
布局:register_layout.xml
注册成功后会把手机号和密码传回登陆界面
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/loginbg_icon"
android:orientation="vertical"
android:weightSum="1">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="370dp"></LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="100dp"
android:layout_weight="0.73"
android:orientation="vertical">
<EditText
android:id="@+id/phone_text"
android:layout_width="300dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:hint="Phone"
android:inputType="number"
android:maxLength="11"
android:textColor="@android:color/holo_blue_light" />
<EditText
android:id="@+id/password_text"
android:layout_width="300dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:hint="Password"
android:inputType="numberPassword"
android:maxLength="18"
android:textColor="@android:color/holo_blue_light" />
<EditText
android:id="@+id/passwordagain_text"
android:layout_width="300dp"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:hint="Password Again"
android:inputType="numberPassword"
android:maxLength="18"
android:textColor="@android:color/holo_blue_light" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:orientation="horizontal"
android:weightSum="1">
<Button
android:id="@+id/signUp_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="60dp"
android:background="@drawable/button_selector_draw"
android:text="Sign Up"
android:textAllCaps="false"
android:textColor="@android:color/holo_blue_light" />
<Button
android:id="@+id/cancel_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/button_selector_draw"
android:text="Cancel"
android:textAllCaps="false"
android:textColor="@android:color/holo_blue_light" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
活动:RegisterActivity.java
public class RegisterActivity extends Activity implements View.OnClickListener {
private static final int MAX_SIZE = 11;
private EditText edit_setPhone, edit_setPassword, edit_resetPassword;
private Button btn_confirm, btn_cancel;
private DBHelper dbHelper;
private String phone, password, passwordAgain;
private DBManager dbManager;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
this.requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.register_layout);
init();
}
private void init() {
dbHelper = new DBHelper(this);
edit_setPhone = (EditText) findViewById(R.id.phone_text);
edit_setPhone.setOnEditorActionListener(new TextView.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId, KeyEvent event) {
return false;
}
});
edit_setPassword = (EditText) findViewById(R.id.password_text);
edit_resetPassword = (EditText) findViewById(R.id.passwordagain_text);
btn_confirm = (Button) findViewById(R.id.signUp_btn);
btn_confirm.setOnClickListener(this);
btn_cancel = (Button) findViewById(R.id.cancel_btn);
btn_cancel.setOnClickListener(this);
}
@Override
public void onClick(View view) {
switch (view.getId()) {
case R.id.cancel_btn:
Intent intent = new Intent(RegisterActivity.this, LoginActivity.class);
startActivity(intent);
break;
case R.id.signUp_btn:
phone = edit_setPhone.getText().toString();
password = edit_setPassword.getText().toString().trim();
passwordAgain = edit_resetPassword.getText().toString().trim();
Log.d("phone+",phone);
Log.d("phone++",password);
Log.d("phone++",passwordAgain);
if (phone.equals("") || password.equals("") || passwordAgain.equals("")) {
Toast.makeText(RegisterActivity.this, "不能为空!", Toast.LENGTH_SHORT).show();
} else {
if (phone.length() != MAX_SIZE) {
Toast.makeText(RegisterActivity.this, "非法的手机号,请重新输入!", Toast.LENGTH_SHORT).show();
} else {
if (CheckIsDataAlreadyInDBOrNot(phone)) {
Toast.makeText(RegisterActivity.this, "该手机号已被注册,注册失败!", Toast.LENGTH_SHORT).show();
} else {
if (password.equals(passwordAgain)) {
RegisterUserInfo(phone, password);
Toast.makeText(RegisterActivity.this, "注册成功!", Toast.LENGTH_SHORT).show();
Intent intent_1 = new Intent(RegisterActivity.this, LoginActivity.class);
intent_1.putExtra("Phone", phone);
intent_1.putExtra("Password", password);
setResult(RESULT_OK, intent_1);
finish();
} else {
Toast.makeText(RegisterActivity.this, "两次输入密码不一致!", Toast.LENGTH_SHORT).show();
}
}
}
}
break;
}
}
private void RegisterUserInfo(String phone, String password) {
SQLiteDatabase db = dbHelper.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(PHONE, phone);
values.put(PASSWORD, password);
db.insert(USER_TABLE, null, values);
db.close();
}
private boolean CheckIsDataAlreadyInDBOrNot(String phone) {
SQLiteDatabase db = dbHelper.getWritableDatabase();
String sql = "select * from "+USER_TABLE+" where "+PHONE+"=?";
Cursor c = db.rawQuery(sql, new String[]{phone});
if (c.getCount() > 0) {
c.close();
return true;
}
c.close();
return false;
}
}
四:数据库SQlite新建表
BDHelper.java
public class DBHelper extends SQLiteOpenHelper {
public static final int DATABASE_VERSION = 1;
public static final String DATABASE_NAME = "Date.db";
public static final String USER_TABLE = "usersinfo";
public static final String PHONE = "userphone";
public static final String PASSWORD = "password";
public DBHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
//新建用户信息表
db.execSQL("CREATE TABLE " + USER_TABLE + " ("
+ PHONE + " TEXT PRIMARY KEY, "
+ PASSWORD + " TEXT) ");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("alter table " + USER_TABLE + " add column other string");
}
}
好了,先到这里吧,源码地址:在上面,希望对看文章的你会有帮助。