实现效果
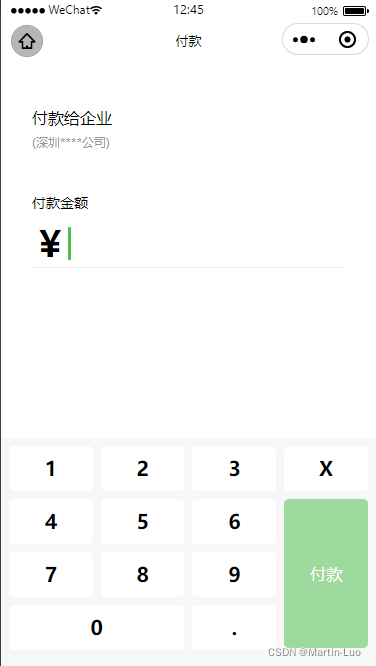
简介
- 点击金额模块区域,获取焦点。
- 点击非金额模块区域,失去焦点且隐藏支付键盘。
- 支持小数点两位数字。
- 付款金额默认置灰,点击付款按钮无效。当输入付款金额后,付款按钮还原正常状态。
- 删除按钮可以通过icon图标或者图片来替换,达到和微信数字支付键盘一样的效果。
- 有更合适的实现方式或者代码不合理的地方,欢迎留言交流
实现代码
// index.wxml
<view class="pay-container" catchtap="handleHiddenKey">
<view class="pay-header">
<view>
付款给企业
<view class="company-name">(深圳****公司)</view>
</view>
</view>
<view class="pay-main">
<view class="pay-money-title">付款金额</view>
<view class="pay-input-wrapper" catchtap="handleShowKey">
<text class="pay-icon">¥</text>
<view hidden="{{!payMoney}}" class="pay-money">{{ payMoney }}</view>
<view hidden="{{keyHidden}}" class="pay-cursor"></view>
</view>
</view>
</view>
<view class="keyboard {{ !keyHidden && 'hind_box' }}">
<view class="key-box">
<view class="number-box clearfix">
<view
wx:for="{{KeyboardKeys}}"
wx:key="*this"
data-key="{{item}}"
class="key {{ index === 9 ? 'key-zero' : ''}}"
hover-class="number-box-hover"
catchtap="handleKey"
>{{item}}</view>
</view>
<view class="btn-box">
<view class="key" hover-class="number-box-hover" data-key="X" catchtap="handleKey">X</view>
<view class="key pay_btn {{payMoney ? '' : 'pay-btn-display'}}" hover-class="pay-btn-hover" catchtap="handlePay">付款</view>
</view>
</view>
</view>
// index.wxss
.clearfix::after {
content: '';
display: table;
clear: both;
}
.pay-container {
padding: 0 62rpx;
height: 100%;
}
.pay-header {
height: 260rpx;
display: flex;
align-items: center;
}
.company-name {
font-size: 24rpx;
margin-top: 12rpx;
color: #909399;
}
.pay-money-title {
font-size: 28rpx;
}
.pay-input-wrapper {
margin-top: 12rpx;
position: relative;
display: flex;
height: 100rpx;
line-height: 100rpx;
align-items: center;
}
.pay-input-wrapper:after {
content: " ";
position: absolute;
left: -50%;
right: -50%;
bottom: 0;
border-bottom: 1px solid rgb(235, 237, 240);
transform: scaleX(.5);
}
.pay-icon,
.pay-money {
font-size: 72rpx;
font-weight: bold;
}
.pay-money {
margin-right: 4rpx;
}
.pay-cursor {
width: 6rpx;
height: 66rpx;
background: #1AAD19;
border-radius: 6rpx;
animation: twinkling 1.5s infinite;
}
@keyframes twinkling {
0% {
opacity: 0;
}
90% {
opacity: .8;
}
100% {
opacity: 1;
}
}
.keyboard {
position: fixed;
left: 0;
right: 0;
bottom: 0;
z-index: 999;
height: 0;
background: #f7f7f7;
transition: height 0.3s;
}
.hind_box {
height: 456rpx;
}
.key-box {
display: flex;
padding-left: 16rpx;
padding-bottom: 16rpx;
padding-bottom: calc(16rpx + constant(safe-area-inset-bottom));
padding-bottom: calc(16rpx + env(safe-area-inset-bottom));
}
.number-box {
flex: 3;
}
.number-box .key {
float: left;
margin: 16rpx 16rpx 0 0;
width: calc(100% / 3 - 16rpx);
height: 90rpx;
border-radius: 10rpx;
line-height: 90rpx;
text-align: center;
font-size: 40rpx;
font-weight: bold;
background-color: #fff;
}
.number-box .key.key-zero {
width: calc((100% / 3) * 2 - 16rpx);
}
.keyboard .number-box-hover {
background-color: #e1e1e1 !important;
}
.btn-box {
flex: 1;
}
.btn-box .key {
margin: 16rpx 16rpx 0 0;
height: 90rpx;
border-radius: 10rpx;
line-height: 90rpx;
text-align: center;
font-size: 40rpx;
font-weight: bold;
background-color: #fff;
}
.btn-box .pay_btn {
height: 298rpx;
line-height: 298rpx;
font-weight: normal;
background-color: #1AAD19;
color: #fff;
font-size: 32rpx;
}
.btn-box .pay_btn.pay-btn-display{
background-color: #9ED99D !important;
}
.btn-box .pay_btn.pay-btn-hover{
background-color: #179B16;
}
Page({
data: {
KeyboardKeys: [1, 2, 3 , 4, 5, 6, 7, 8, 9, 0,'.'],
payMoney: '',
keyHidden: false
},
onLoad: function (options) {
},
handleHiddenKey() {
this.setData({
keyHidden: true
})
},
handleShowKey() {
this.setData({
keyHidden: false
})
},
handleKey(e) {
const that = this
const { key } = e.currentTarget.dataset
const { payMoney } = this.data
if (key === 'X') {
if (payMoney !== '') {
const money = payMoney.slice(0, payMoney.length - 1)
that.setData({
payMoney: money
})
}
} else {
const money = payMoney + key
console.log(/^(\d+\.?\d{0,2})$/.test(money), money, 'money')
if (/^(\d+\.?\d{0,2})$/.test(money)) {
that.setData({
payMoney: money
})
}
}
},
handlePay() {
const { payMoney } = this.data
if (payMoney === '') return
console.log(Number(payMoney), '付款')
}
})