详细讲解
详细讲解
SList.c
#include"SList.h"
void SListPrint(SLTNode* phead)
{
SLTNode *cur = phead;
while (cur != NULL)
{
printf("%d->", cur->data);
cur = cur->next;
}
printf("NULL\n");
}
SLTNode* BuySListNode(SLTDataType x)
{
SLTNode* node = (SLTNode*)malloc(sizeof(SLTNode));
if (node == NULL)
{
printf("malloc fail\n");
exit(-1);
}
node->data = x;
node->next = NULL;
return node;
}
void SListPushBack(SLTNode **pphead, SLTDataType x)
{
if (*pphead == NULL)
{
SLTNode *newnode = BuySListNode(x);
*pphead = newnode;
}
else
{
SLTNode* tail = *pphead;
while (tail->next != NULL)
{
tail = tail->next;
}
SLTNode *newnode = BuySListNode(x);
tail->next = newnode;
}
}
void SListPushFront(SLTNode **pphead, SLTDataType x)
{
SLTNode *newnode = BuySListNode(x);
newnode->next = *pphead;
*pphead = newnode;
}
void SListPopBack(SLTNode **pphead)
{
assert(pphead);
assert(*pphead);
if ((*pphead)->next == NULL)
{
free(*pphead);
*pphead = NULL;
}
else
{
SLTNode *prev = NULL;
SLTNode *tail = *pphead;
while (tail->next != NULL)
{
prev = tail;
tail = tail->next;
}
free(tail);
prev->next = NULL;
}
}
void SListPopFront(SLTNode** pphead)
{
assert(pphead);
assert(*pphead);
SLTNode *next = (*pphead)->next;
free(*pphead);
(*pphead) = next;
}
int SListSize(SLTNode* phead)
{
int size = 0;
SLTNode* cur = phead;
while (cur)
{
size++;
cur = cur->next;
}
return size;
}
bool SListEmpty(SLTNode* phead)
{
return phead == NULL ? true : false;
}
SLTNode* SListFind(SLTNode* phead, SLTDataType x)
{
SLTNode* cur = phead;
while (cur)
{
if (cur->data == x)
{
return cur;
}
else
{
cur = cur->next;
}
}
return NULL;
}
void SListInsert(SLTNode** pphead, SLTNode* pos, SLTDataType x)
{
assert(pphead);
assert(pos);
if (*pphead == pos)
{
SListPushFront(pphead, x);
}
else
{
SLTNode* prev = *pphead;
while (prev->next != pos)
{
prev = prev->next;
}
SLTNode* newnode = BuySListNode(x);
newnode->next = pos;
prev->next = newnode;
}
}
void SListInsertAfter(SLTNode* pos, SLTDataType x)
{
assert(pos);
SLTNode* newnode = BuySListNode(x);
newnode->next = pos->next;
pos->next = newnode;
}
void SListErase(SLTNode** pphead, SLTNode* pos)
{
assert(pphead);
assert(pos);
if (pos == *pphead)
{
SListPopFront(pphead);
}
else
{
SLTNode* prev = *pphead;
while (prev->next != pos)
{
prev = prev->next;
}
prev->next = pos->next;
free(pos);
pos = NULL;
}
}
void SListEraseAfter(SLTNode* pos)
{
assert(pos);
assert(pos->next);
SLTNode* next = pos->next;
pos->next = next->next;
free(next);
next = NULL;
}
void SListDestory(SLTNode** pphead)
{
assert(pphead);
SLTNode* cur = *pphead;
while (cur)
{
SLTNode* next = cur->next;
free(cur);
cur = next;
}
*pphead = NULL;
}
test.c
#include"SList.h"
void Test()
{
printf("test1:\n\n");
SLTNode* n1 = (SLTNode*)malloc(sizeof(SLTNode));
n1->data = 1;
SLTNode* n2 = (SLTNode*)malloc(sizeof(SLTNode));
n2->data = 2;
SLTNode* n3 = (SLTNode*)malloc(sizeof(SLTNode));
n3->data = 3;
SLTNode* n4 = (SLTNode*)malloc(sizeof(SLTNode));
n4->data = 4;
n1->next = n2;
n2->next = n3;
n3->next = n4;
n4->next = NULL;
SLTNode* plist = n1;
SListPrint(plist);
printf("\n");
}
void Test2()
{
printf("test2:\n\n");
SLTNode* plist = NULL;
SListPushFront(&plist, -1);
SListPushBack(&plist,1);
SListPushBack(&plist,2);
SListPushBack(&plist,3);
SListPushBack(&plist,4);
SListPushBack(&plist,5);
SListPushFront(&plist, 0);
SListPrint(plist);
SListPopBack(&plist);
SListPrint(plist);
SListPopFront(&plist);
SListPrint(plist);
printf("size:%d\n", SListSize(plist));
printf("size:%d\n",SListEmpty(plist));
while (!SListEmpty(plist))
{
SListPopFront(&plist);
SListPrint(plist);
}
printf("SListSize:%d\n", SListSize(plist));
printf("SListEmpty:%d\n", SListEmpty(plist));
printf("\n");
}
void Test3()
{
printf("test3:\n\n");
SLTNode* plist = NULL;
SListPushBack(&plist, 1);
SListPushBack(&plist, 2);
SListPushBack(&plist, 3);
SListPushBack(&plist, 4);
SListPushBack(&plist, 5);
SListPrint(plist);
SLTNode* pos = SListFind(plist, 3);
if (pos)
{
printf("找到了\n");
}
pos->data = 30;
SListPrint(plist);
SListInsert(&plist, pos, 40);
SListPrint(plist);
SListInsert(&plist, plist, 40);
SListPrint(plist);
if (pos)
{
SListErase(&plist, pos);
}
SListPrint(plist);
pos = SListFind(plist, 4);
if (pos)
{
SListEraseAfter(pos);
}
SListPrint(plist);
SListDestory(&plist);
SListPrint(plist);
}
int main()
{
Test();
Test2();
Test3();
}
SList.h
#pragma once
#include<stdio.h>
#include<assert.h>
#include<stdbool.h>
typedef int SLTDataType;
typedef struct SListNode
{
SLTDataType data;
struct SListNode*next;
}SLTNode;
void SListPrint(SLTNode* phead);
int SListSize(SLTNode* phead);
bool SListEmpty(SLTNode* phead);
void SListPushBack(SLTNode **pphead, SLTDataType x);
void SListPushFront(SLTNode **pphead, SLTDataType x);
void SListPopBack(SLTNode **pphead);
void SListPopFront(SLTNode** pphead);
void SListInsert(SLTNode** pphead, SLTNode* pos, SLTDataType x);
void SListInsert(SLTNode** pphead, SLTNode* pos, SLTDataType x);
void SListInsertAfter(SLTNode* pos, SLTDataType x);
void SListErase(SLTNode** pphead, SLTNode* pos);
void SListEraseAfter(SLTNode* pos);
void SListDestory(SLTNode** pphead);
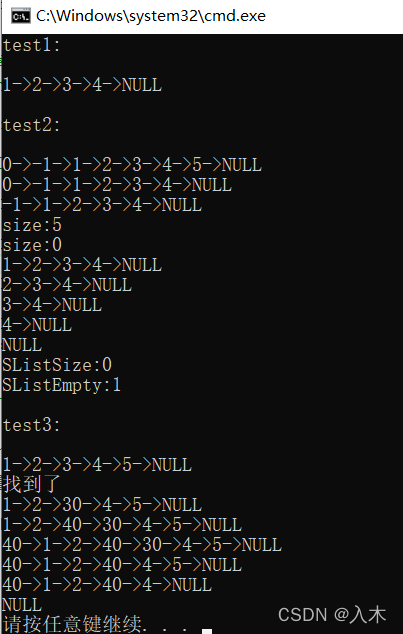