集合
集合的由来:由于数组操作数据的弊端,所以产生集合用来代替数组
数组的弊端
1.只能添加相同类型的元素(基本数据类型 和 引用数据类型都能保存)
2.长度一旦确定就不能改变,要添加超出数组长度个数的元素,操作就比较复杂
集合的特点:
1.能添加不同数据类型的元素
注意:集合中只能添加引用数据类型(即只能添加对象类型),保存基本数据类型是以自动装箱的形式进行存储
2.没有固定的长度,长度可变
集合分类
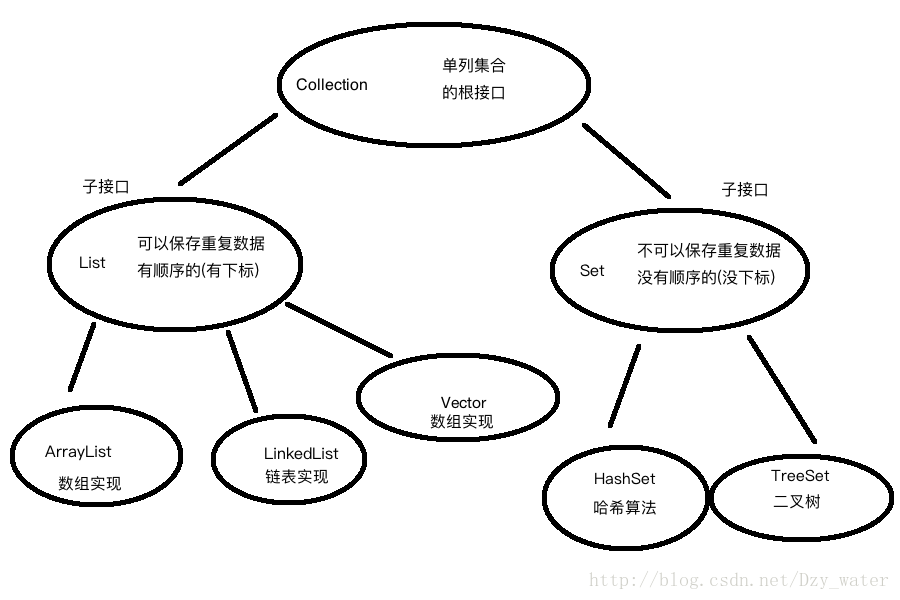
集合 Collection 接口中的方法
集合 Collection 的基本方法
注意:@SuppressWarnings({"unchecked","rawtypes"}) 放到类上整个类不写泛型都不会报黄
注解 rawtypes 保持原有类型
unchecked 不检查插入数据的类型
代码示例:
// 创建一个集合 多态的声明方法
Collection collection = new ArrayList()
// 添加方法
boolean add = collection.add("a")
boolean add2 = collection.add("b")
boolean add3 = collection.add("c")
// 当你向集合当中添加基本数据类型的时候 系统会帮你进行自动装箱
// 把基本数据类型变成它的包装类
boolean add4 = collection.add(10)
boolean add5 = collection.add(true)
// 打印集合
System.out.println(collection)
System.out.println(add)
System.out.println(add2)
System.out.println(add3)
System.out.println(add4)
System.out.println(add5)
注意:ArrayList中的add不可能返回失败,设计返回值是因为要适用所有子类接口
// 获取集合的长度
System.out.println(collection.size())
// 判断是否包含某个元素
boolean contains = collection.contains("d")
System.out.println(contains)
// 从集合中删除一个元素
boolean remove = collection.remove("a")
// 操作的是 集合本身
System.out.println(collection)
System.out.println(remove)
// 判断集合是否为空
boolean empty = collection.isEmpty()
System.out.println(empty)
// 清空 集合
collection.clear()
System.out.println(collection)
addAll、removeAll、retainAll
代码示例:
Collection collection = new ArrayList()
collection.add("a")
collection.add("b")
collection.add("c")
System.out.println(collection)
// 测试 addAll
Collection collection1 = new ArrayList()
collection1.add("x")
collection1.add("y")
collection1.add("z")
collection1.addAll(collection)
System.out.println(collection1)
// add 是把集合collection中所有元素 以元素的形式放入集合collection1中
collection1.add(collection)
System.out.println(collection1)
// 测试 containsAll 是否
boolean containsAll = collection1.containsAll(collection)
System.out.println(containsAll)
// addAll方法是 把集合collection的元素取出来加在集合collection1的末尾
// removeAll 删除 集合collection2 与 集合collection 的重合元素,另一个集合不变只删除交集,重复也可以删除
Collection collection2 = new ArrayList()
collection2.add("a")
collection2.add("b")
collection2.add("c")
collection2.add("d")
collection2.removeAll(collection)
System.out.println(collection2)
// retainAll 把两个集合的交集取出来 保存在调用的集合
// 如果c1和原来对比发生变化返回true
Collection collection3 = new ArrayList()
collection3.add("a")
collection3.add("b")
collection3.add("c")
collection3.add("d")
collection3.retainAll(collection)
System.out.println(collection3)
toArray
打印字符串:
Collection collection = new ArrayList();
collection.add("a");
collection.add("b");
collection.add("c");
collection.add("d");
Object[] array = collection.toArray();
for (int i = 0; i < array.length; i++) {
System.out.println(array[i]);
}
需求:创建一个数组长度为5,保存三个学生 遍历学生的信息 只打印姓名
Collection collection = new ArrayList();
collection.add(new Student("ygs", 18));
collection.add(new Student("sxm", 18));
collection.add(new Student("dp", 18));
Object[] array = collection.toArray();
for (int i = 0; i < array.length; i++) {
Student student = (Student)array[i];
System.out.println(student.getName());
}
迭代器(遍历)
测试迭代器中的方法:
Collection collection = new ArrayList()
collection.add("a")
collection.add("b")
collection.add("c")
collection.add("d")
// 获取集合中的迭代器
Iterator iterator = collection.iterator()
// 先判断集合中是否有元素
boolean hasNext = iterator.hasNext()
System.out.println(hasNext)
// 从集合中取出元素
Object next = iterator.next()
System.out.println(next)
运用迭代器遍历集合:
Collection collection = new ArrayList()
// 迭代时 有一个指针 指向集合的首位置
// 每调用一次 next方法 这个指针向下移一格
collection.add("a")
collection.add("b")
collection.add("c")
collection.add("d")
Iterator iterator = collection.iterator()
// 如果有元素 就获取 没元素 就停止循环
while (iterator.hasNext()) {
// 注意: 迭代时 循环 只能使用一次next()方法
Object next = iterator.next()
System.out.println(next)
}
需求:创建一个数组长度为5,保存三个学生 遍历学生的信息 只打印姓名
Collection collection = new ArrayList()
collection.add(new Student("ygs", 18))
collection.add(new Student("dp", 18))
collection.add(new Student("sxm", 18))
Iterator iterator = collection.iterator()
while (iterator.hasNext()) {
Object next = iterator.next()
Student student = (Student)next
System.out.println(student.getName())
}