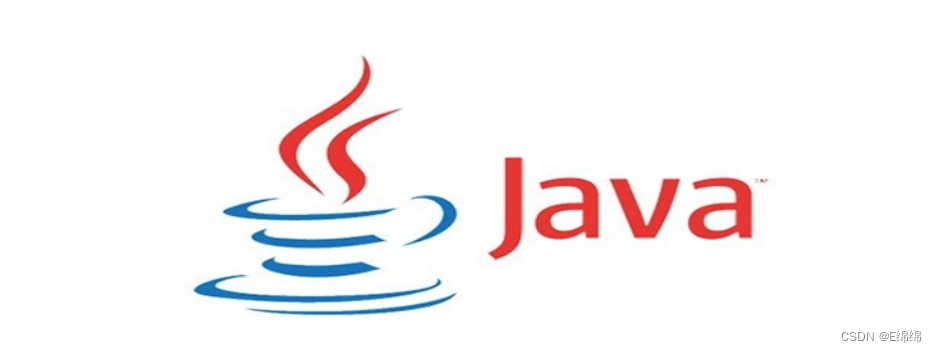
Spring Boot 已成为现代 Java 开发中不可或缺的工具,其简化配置和自动化功能极大地提高了开发效率。然而,Spring Boot 的强大不仅仅体现在这些基础功能上,还有许多中高级特性可以进一步提升应用的性能和可维护性。本文将详细介绍 Spring Boot 的一些中高级特性,包括异步处理、安全管理、监控和调试等方面。
1. 异步处理
1.1 什么是异步处理?
在现代应用中,处理大量并发请求和长时间运行的任务时,异步处理是一种有效的方式。Spring Boot 通过 @EnableAsync
注解和 @Async
注解实现了异步方法的调用,从而避免了阻塞主线程,提高了系统的响应速度和吞吐量。
1.2 实现异步处理
1.2.1 启用异步支持
在 Spring Boot 应用中启用异步支持非常简单,只需在配置类中添加 @EnableAsync
注解:
@Configuration
@EnableAsync
public class AsyncConfig {
}
1.2.2 使用 @Async 注解
在需要异步执行的方法上添加 @Async
注解即可:
@Service
public class AsyncService {
@Async
public void asyncMethod() {
// 长时间运行的任务
System.out.println("异步方法开始执行");
}
}
1.2.3 调用异步方法
在其他类中调用异步方法时,该方法会在单独的线程中执行,不会阻塞调用者线程:
@RestController
public class AsyncController {
@Autowired
private AsyncService asyncService;
@GetMapping("/async")
public String executeAsync() {
asyncService.asyncMethod();
return "异步方法已调用";
}
}
2. 安全管理
2.1 Spring Security 集成
Spring Boot 与 Spring Security 无缝集成,提供了强大的认证和授权功能。默认情况下,Spring Security 会保护所有的 HTTP 端点,并要求用户进行身份验证。
2.2 基础安全配置
2.2.1 添加依赖
在 pom.xml
文件中添加 Spring Security 依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
2.2.2 默认配置
默认情况下,Spring Security 会生成一个安全密码,用户需要在登录时使用。可以通过自定义配置类来调整安全设置:
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().and()
.httpBasic();
}
}
2.2.3 自定义用户认证
可以通过实现 UserDetailsService
接口来自定义用户认证逻辑:
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
// 根据用户名查找用户信息并返回
return new User(username, "password", new ArrayList<>());
}
}
3. 监控和调试
3.1 Spring Boot Actuator
Spring Boot Actuator 提供了一套生产环境监控和管理功能,通过一组端点暴露应用的运行状态、健康状况和各种指标。
3.1.1 添加依赖
在 pom.xml
文件中添加 Spring Boot Actuator 依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
3.1.2 配置 Actuator 端点
在 application.properties
文件中配置 Actuator 端点的访问权限:
management.endpoints.web.exposure.include=*
management.endpoint.health.show-details=always
3.1.3 常用端点
/actuator/health
:显示应用的健康状况。/actuator/info
:显示应用的基本信息。/actuator/metrics
:显示应用的各种运行指标。
3.2 自定义健康检查
可以通过实现 HealthIndicator
接口来自定义健康检查逻辑:
@Component
public class CustomHealthIndicator implements HealthIndicator {
@Override
public Health health() {
// 自定义健康检查逻辑
boolean isHealthy = checkHealth();
if (isHealthy) {
return Health.up().build();
} else {
return Health.down().withDetail("Error", "Custom error message").build();
}
}
private boolean checkHealth() {
// 执行健康检查逻辑
return true;
}
}
4. 日志管理
4.1 Spring Boot 日志系统
Spring Boot 使用 SLF4J 作为日志接口,并默认集成了 Logback 作为日志实现。通过配置文件可以灵活管理日志级别和输出格式。
4.2 配置日志级别
在 application.properties
文件中配置日志级别:
logging.level.org.springframework.web=DEBUG
logging.level.com.example=TRACE
4.3 自定义日志配置
可以通过 logback-spring.xml
文件自定义 Logback 的日志配置:
<configuration>
<appender name="console" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
<root level="info">
<appender-ref ref="console"/>
</root>
</configuration>
5. 外部配置与属性注入
5.1 外部配置
Spring Boot 支持多种外部配置方式,如配置文件、环境变量、命令行参数等。可以在 application.properties
或 application.yml
文件中进行配置。
5.2 属性注入
通过 @Value
注解可以将配置文件中的属性注入到 Spring Bean 中:
@Component
public class MyBean {
@Value("${my.custom.property}")
private String myProperty;
@PostConstruct
public void init() {
System.out.println("Property value: " + myProperty);
}
}
5.3 类型安全的配置
Spring Boot 提供了类型安全的配置绑定功能,通过 @ConfigurationProperties
注解将配置文件中的属性绑定到 POJO 类中:
@ConfigurationProperties(prefix = "my")
@Component
public class MyProperties {
private String customProperty;
// getters and setters
}
在 application.properties
文件中定义属性:
my.custom-property=Some value
6. 总结
Spring Boot 提供了丰富的中高级特性,使得开发和维护企业级应用更加高效和便捷。通过掌握异步处理、安全管理、监控和调试、日志管理以及外部配置与属性注入等功能,开发者可以构建出更加健壮和灵活的应用程序。
Spring Boot 的生态系统仍在不断发展,未来版本将引入更多新特性和改进。通过不断学习和实践,开发者可以充分利用 Spring Boot 的优势,提升开发效率和应用质量。