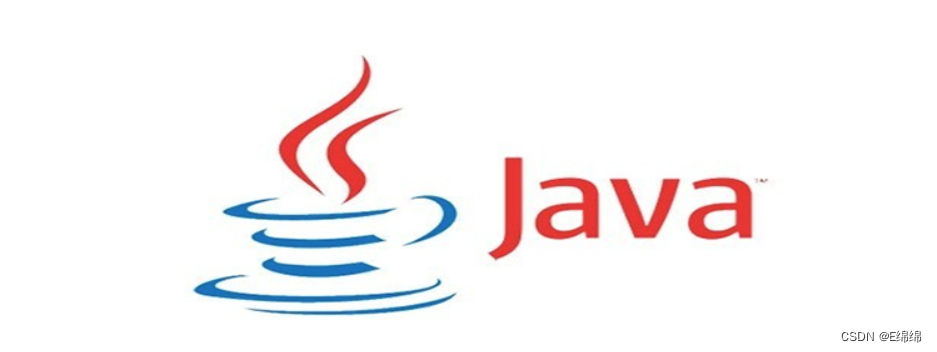
引言
在当今的软件开发环境中,微服务架构已经成为一种主流趋势。微服务架构的核心思想是将应用程序分解为一组小的、自治的服务,每个服务负责单一的业务功能。这种架构的优势在于其灵活性、可扩展性和易于维护性。SpringBoot作为一个强大的框架,为开发现代化微服务架构提供了极大的便利。本文将详细探讨如何使用SpringBoot来构建和管理微服务。
微服务架构概述
什么是微服务架构
微服务架构是一种设计风格,它将应用程序划分为一组小型、独立部署的服务。这些服务可以独立开发、测试、部署和扩展。每个微服务通常负责特定的业务功能,并通过轻量级的通信协议(通常是HTTP/REST或消息队列)进行交互。
微服务的优势
- 模块化:将应用程序分解为多个独立的模块,使得每个模块可以独立开发和部署。
- 灵活性:不同的微服务可以使用不同的技术栈和数据库,适应不同的业务需求。
- 可扩展性:可以独立地扩展每个微服务,根据业务需求进行水平扩展。
- 故障隔离:一个微服务的故障不会影响到整个系统,提高了系统的可靠性。
使用SpringBoot构建微服务
创建SpringBoot微服务项目
使用SpringBoot创建微服务项目非常简单。通过Spring Initializr,开发者可以快速生成一个包含基本配置的SpringBoot项目。
示例:创建订单服务
- 访问 Spring Initializr,选择合适的项目选项(如Maven项目、Java语言、Spring Boot版本等)。
- 添加必要的依赖项,如Spring Web、Spring Data JPA、H2 Database等。
- 生成项目并下载到本地。
解压下载的项目,并导入到IDE中。
配置数据库
在application.properties
文件中配置H2数据库:
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=password
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
spring.h2.console.enabled=true
创建实体类和Repository
创建一个订单实体类:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Order {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String product;
private Integer quantity;
private Double price;
// getters and setters
}
创建一个OrderRepository接口:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface OrderRepository extends JpaRepository<Order, Long> {
}
创建服务层和控制器
服务层:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class OrderService {
@Autowired
private OrderRepository orderRepository;
public List<Order> getAllOrders() {
return orderRepository.findAll();
}
public Order getOrderById(Long id) {
return orderRepository.findById(id).orElse(null);
}
public Order createOrder(Order order) {
return orderRepository.save(order);
}
public void deleteOrder(Long id) {
orderRepository.deleteById(id);
}
}
控制器:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/orders")
public class OrderController {
@Autowired
private OrderService orderService;
@GetMapping
public List<Order> getAllOrders() {
return orderService.getAllOrders();
}
@GetMapping("/{id}")
public Order getOrderById(@PathVariable Long id) {
return orderService.getOrderById(id);
}
@PostMapping
public Order createOrder(@RequestBody Order order) {
return orderService.createOrder(order);
}
@DeleteMapping("/{id}")
public void deleteOrder(@PathVariable Long id) {
orderService.deleteOrder(id);
}
}
微服务间通信
在微服务架构中,各个服务需要通过某种方式进行通信。常见的通信方式包括HTTP RESTful API和消息队列。SpringBoot提供了多种工具和框架来简化微服务间的通信。
使用RestTemplate进行同步通信
RestTemplate是Spring提供的一个同步HTTP客户端,用于向其他服务发起HTTP请求。
示例:调用用户服务
假设我们有一个用户服务(User Service),需要在订单服务中调用用户服务来获取用户信息。
- 创建一个RestTemplate Bean:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.client.RestTemplate;
@Configuration
public class AppConfig {
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
- 在OrderService中使用RestTemplate:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
@Service
public class OrderService {
@Autowired
private RestTemplate restTemplate;
public User getUserById(Long userId) {
String url = "http://localhost:8081/users/" + userId;
return restTemplate.getForObject(url, User.class);
}
}
使用Feign进行声明式通信
Feign是一个声明式HTTP客户端,它使得HTTP调用变得更加简单和直观。Spring Cloud集成了Feign,使其与SpringBoot应用无缝结合。
示例:使用Feign调用用户服务
- 添加Feign依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
- 启用FeignClient:
import org.springframework.cloud.openfeign.EnableFeignClients;
import org.springframework.context.annotation.Configuration;
@Configuration
@EnableFeignClients
public class AppConfig {
}
- 创建Feign客户端接口:
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
@FeignClient(name = "user-service", url = "http://localhost:8081")
public interface UserClient {
@GetMapping("/users/{id}")
User getUserById(@PathVariable("id") Long id);
}
- 在OrderService中使用Feign客户端:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class OrderService {
@Autowired
private UserClient userClient;
public User getUserById(Long userId) {
return userClient.getUserById(userId);
}
}
服务发现与注册
在微服务架构中,服务的数量可能会动态变化。为了管理这些服务,可以使用服务发现与注册机制。Spring Cloud提供了Eureka作为服务发现与注册的解决方案。
配置Eureka Server
- 创建Eureka Server项目并添加依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
- 启用Eureka Server:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
- 配置Eureka Server:
server.port=8761
eureka.client.register-with-eureka=false
eureka.client.fetch-registry=false
配置Eureka Client
在每个微服务中,添加Eureka Client依赖并进行配置。
- 添加依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
- 配置Eureka Client:
eureka.client.service-url.defaultZone=http://localhost:8761/eureka/
- 启用Eureka Client:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableEurekaClient
public class OrderServiceApplication {
public static void main(String[] args) {
SpringApplication.run(OrderServiceApplication.class, args);
}
}
通过Eureka,微服务可以自动注册和发现其他服务,从而简化了服务间的通信和管理。
API网关
在微服务架构中,API网关是一个关键组件。它充当所有客户端请求的入口,并将请求路由到相应的微服务。Spring Cloud Gateway是一个高效的API网关解决方案。
配置Spring Cloud Gateway
- 创建API网关项目并添加依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
- 配置路由:
spring.cloud.gateway.routes[0].id=order-service
spring.cloud.gateway.routes[0].uri=http://localhost:8080
spring.cloud.gateway.routes[0].predicates[0]=Path=/orders/**
- 启动API网关:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ApiGatewayApplication {
public static void main(String[] args) {
SpringApplication.run(ApiGatewayApplication.class, args);
}
}
通过API网关,所有客户端请求将通过统一入口,从而简化了客户端与微服务的交互。
配置管理
在微服务架构中,每个服务可能有不同的配置需求。为了统一管理配置,可以使用Spring Cloud Config。
配置Spring Cloud Config Server
- 创建Config Server项目并添加依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
- 启用Config Server:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
- 配置Config Server:
server.port=8888
spring.cloud.config.server.git.uri=https://github.com/your-repo/config-repo
配置Spring Cloud Config Client
在每个微服务中,添加Config Client依赖并进行配置。
- 添加依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
- 配置Config Client:
spring.cloud.config.uri=http://localhost:8888
通过Spring Cloud Config,可以集中管理和分发配置文件,提高配置管理的效率和一致性。
服务监控与管理
微服务架构需要有效的监控和管理机制。Spring Boot Actuator和Spring Cloud Sleuth是两种常用的工具,用于监控和分布式跟踪。
使用Spring Boot Actuator进行监控
Spring Boot Actuator提供了一系列的端点,用于监控和管理Spring Boot应用。
- 添加Actuator依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
- 启用Actuator端点:
management.endpoints.web.exposure.include=*
通过访问/actuator
端点,可以获取应用的各种监控信息,如健康检查、指标、环境等。
使用Spring Cloud Sleuth进行分布式跟踪
Spring Cloud Sleuth为Spring Boot应用提供了分布式跟踪功能,帮助开发者了解请求在微服务中的传播路径。
- 添加Sleuth依赖:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-sleuth</artifactId>
</dependency>
- 配置Sleuth:
spring.sleuth.sampler.probability=1.0
通过Spring Cloud Sleuth,可以追踪每个请求的调用链,帮助识别性能瓶颈和故障点。
结论
SpringBoot通过其简化配置、自动化和强大的生态系统,显著提升了微服务架构的开发效率。无论是创建微服务、实现服务间通信、管理配置还是进行服务监控,SpringBoot和Spring Cloud都提供了丰富的工具和解决方案。通过合理利用这些工具和框架,开发者可以构建出高性能、可扩展和易维护的现代化微服务架构。希望这篇文章能够帮助开发者更好地理解和使用SpringBoot,在实际项目中取得成功。