单链表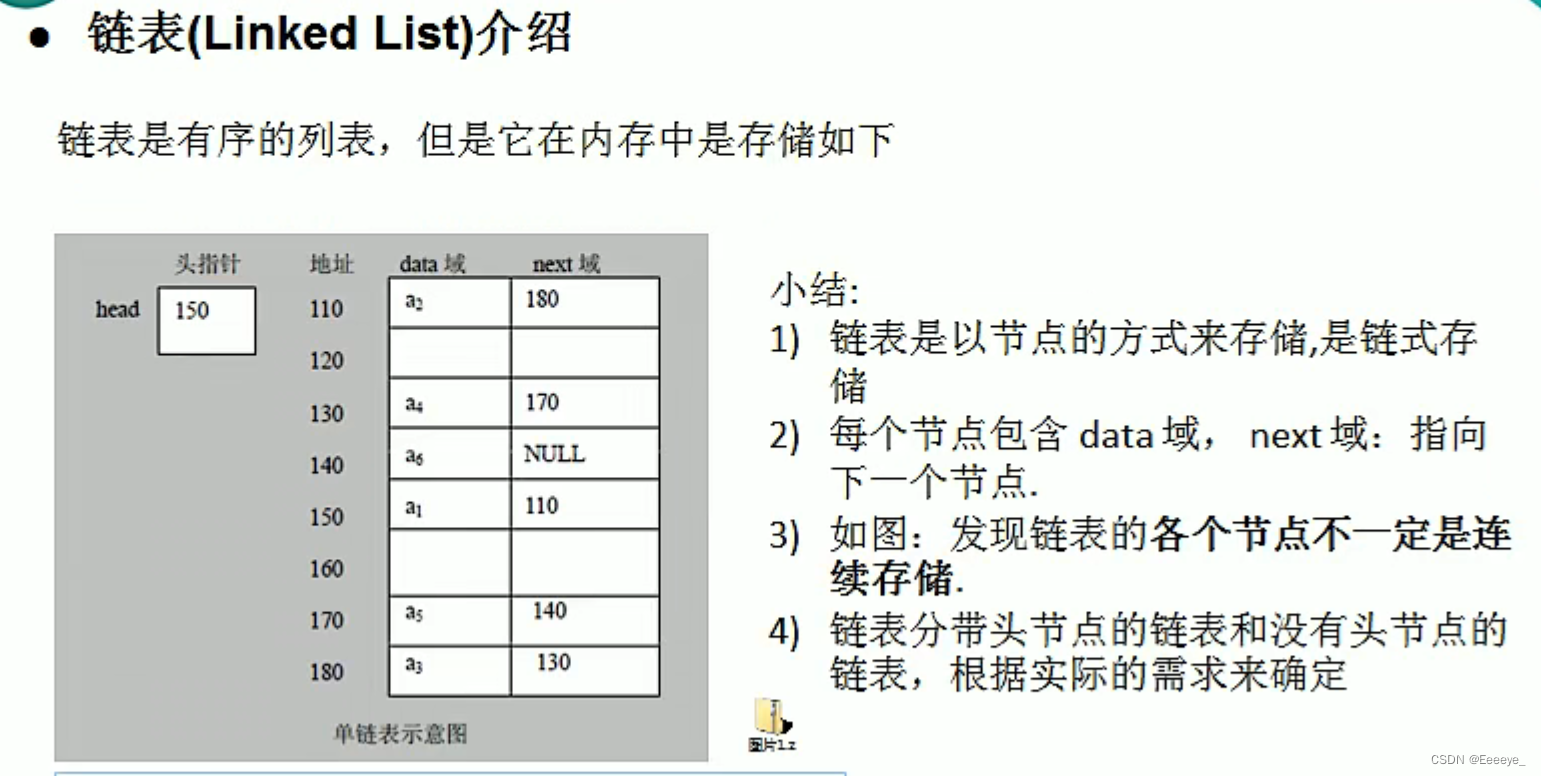
通过next进行结点之间的指向
通过Null判断是非为最后一个节点
- 思路1 将输入的数据简单地放在链表的最后(add方法)
package com.Eeeeye.linkedlist;
public class SingleLinkedListDemo {
public static void main(String[] args) {
//test
HeroNode hero1 = new HeroNode(1, "宋江", "及时雨");
HeroNode hero2 = new HeroNode(2,"卢俊义", "玉麒麟");
SingleLinkedList singleLinkedList = new SingleLinkedList();
singleLinkedList.add(hero1);
singleLinkedList.add(hero2);
singleLinkedList.list();
}
}
class SingleLinkedList{
//先初始化一个头节点,头节点不动,也不存放具体数据
private HeroNode head = new HeroNode(0,"","");
//添加节点到单向链表
//当不考虑编号顺序时
//1.找到当前链表的最后节点
//2.将最后这个节点的next 指向 新的节点
public void add(HeroNode heroNode){
//因为head节点不动,故需要一个辅助。临时 的变量
HeroNode temp = head;
while (true){
if (temp.next == null) {
break;
}
//如果还未找到最后一个节点
temp = temp.next;//将temp后移
}
temp.next = heroNode;//将输入的heroNode赋值给新的一个节点
}
public void list(){
if (head.next == null) {
System.out.println("链表为空");
return;
}
HeroNode temp = head.next;
while (true){
if (temp == null){
break;
}
System.out.println(temp);
temp = temp.next;
}
}
}
class HeroNode{
public int no;
public String name;
public String nickname;
public HeroNode next;
public HeroNode (int no, String name,String nickname){
this.no = no;
this.name = name;
this.nickname = nickname;
}
@Override
public String toString() {
//注意重写的toSrting方法无需带有next,否则会一直next导致第一行就输出了所有节点
return "HeroNode [no=" + no + ",name=" + name + ", nickname=" + nickname + "]";
}
}
- 思路优化(以及链表的增删改)
增(按照次序):heroNode.next = temp.next;
temp.next = heroNode;两段指向使得节点添加到链表中
删:temp.next = temp.next.next;
改: temp.nickname = newheroNode.nickname;
temp.name = newheroNode.name;
package com.Eeeeye.linkedlist;
public class SingleLinkedListDemo {
public static void main(String[] args) {
//test
HeroNode hero1 = new HeroNode(1, "宋江", "及时雨");
HeroNode hero2 = new HeroNode(2, "卢俊义", "玉麒麟");
HeroNode hero3 = new HeroNode(3, "吴用", "智多星");
HeroNode hero4 = new HeroNode(4, "林冲", "豹子头");
SingleLinkedList singleLinkedList = new SingleLinkedList();
singleLinkedList.addByOrder(hero1);
singleLinkedList.addByOrder(hero2);
singleLinkedList.addByOrder(hero4);
singleLinkedList.del(hero1.no);
singleLinkedList.addByOrder(hero1);
singleLinkedList.addByOrder(hero3);
singleLinkedList.list();
HeroNode newHeroNode = new HeroNode(2, "小卢", "玉麒麟33");
singleLinkedList.update(newHeroNode);
singleLinkedList.list();
}
}
class SingleLinkedList {
//先初始化一个头节点,头节点不动,也不存放具体数据
private HeroNode head = new HeroNode(0, "", "");
//添加节点到单向链表
//当不考虑编号顺序时
//1.找到当前链表的最后节点
//2.将最后这个节点的next 指向 新的节点
public void add(HeroNode heroNode) {
//因为head节点不动,故需要一个辅助"临时"的变量
HeroNode temp = head;
while (true) {
if (temp.next == null) {
break;
}
//如果还未找到最后一个节点
temp = temp.next;//将temp后移
}//while循环结束
temp.next = heroNode;//将输入的heroNode赋值给新的一个节点
}
//有序添加
public void addByOrder(HeroNode heroNode) {
//因为单链表,所以应该找插入的前一个节点
HeroNode temp = head;//确保temp在插入的点的前面
boolean flag = false;//默认为编号存在可添加
while (true) {
if (temp.next == null) {//说明temp已经在链表最后
break;
}
if (temp.next.no > heroNode.no) {//位置已找到,添加到temp和temp.next中间
break;
} else if (temp.next.no == heroNode.no) {
flag = true;
break;
}
temp = temp.next;
}
if (flag) {
System.out.println("已存在" + heroNode.no);
} else {
heroNode.next = temp.next;
temp.next = heroNode;//倒过来可以吗
}
}
public void update(HeroNode newheroNode) {//修改
//先判断链表是否为空,再遍历查找匹配的heroNode
if (head.next == null) {
System.out.println("链表为空");
return;
}
HeroNode temp = head;
boolean flag = false;
while (true) {
if (null == temp) {
System.out.println("已到底");
break;
} else if (temp.no == newheroNode.no) {
System.out.println("已捕获");
flag = true;
break;
}
temp = temp.next;
}
if (flag) {
temp.nickname = newheroNode.nickname;
temp.name = newheroNode.name;
} else {
System.out.println("链表中无此节点%d,无法修改" + newheroNode.no);
}
}
//删除链表中的节点
public void del(int no) {
HeroNode temp = head;//必须从头节点开始,因为单链表要找前一个节点
boolean flag = false;//标志着默认是未找到删除的节点
while (true) {
if (temp.next == null) {
break;
}
if (temp.next.no == no) {
System.out.println("已找到删除的链表节点");
flag = true;
break;
}
temp = temp.next;
}
if (flag) {
temp.next = temp.next.next;//找前一个节点的原因(否则在删除时链表会断开
} else {
System.out.println("要删除的节点%d不存在" + no);
}
}
public void list() {
if (head.next == null) {
System.out.println("链表为空");
return;
}
HeroNode temp = head.next;
while (true) {
if (temp == null) {
break;
}
System.out.println(temp);
temp = temp.next;
}
}
}
class HeroNode {
public int no;
public String name;
public String nickname;
public HeroNode next;
public HeroNode(int no, String name, String nickname) {
this.no = no;
this.name = name;
this.nickname = nickname;
}
@Override
public String toString() {
//注意重写的toSrting方法无需带有next,否则会一直next导致第一行就输出了所有节点
return "HeroNode [no=" + no + ",name=" + name + ", nickname=" + nickname + "]";
}
}
面试题
//得到链表中的节点个数)
public static int getLength(HeroNode head) {
while (head.next == null) {
return 0;
}
HeroNode temp = head.next;
int length = 0;
while (temp != null ) {
length++;
temp = temp.next;
}
return length;
}
//查找倒数第N个节点
public static HeroNode findLastIndexNode(HeroNode head, int index) {
if (head.next == null) {
return null;
}
int size = getLength(head);
if (index <= 0 || index > size) {
return null;
}
HeroNode cur = head.next;
//通过size - index得到正向的索引,再次遍历得到具体的节点
for (int i = 0; i < size - index; i++) {
cur = cur.next;
}
return cur;
}
//使用栈的特性(先进后出)逆序打印链表,且没有破坏链表的结构
public static void reversePrint(HeroNode head) {
if (head.next == null) {
return;//空链表,无法打印
}
//创建一个栈,将节点压入栈中
Stack<HeroNode> stack = new Stack<>();
HeroNode cur = head.next;
while (cur != null) {
stack.push(cur);
//将cur后移,这样就可以压入下一个节点
cur = cur.next;
}
while (stack.size() > 0) {
System.out.println(stack.pop());
}
}
//将链表反转
public static void reverseList(HeroNode head){
//特殊情况的意识
if (head.next == null || head.next.next == null) {
return;
}
HeroNode cur = head.next;
HeroNode next = null;
HeroNode reverseHead = new HeroNode(0,"","");
while (cur != null){
next = cur.next;//暂存cur.next节点,因为下面的操作将会改变cur.next的指向
cur.next = reverseHead.next;//不要将其理解为把reverseHead.next赋值给cur.next,而是理解为cur的next是reverseHead.next
reverseHead.next = cur;//将反转链表的最前端指向此时的cur节点,同样是理解为reverseHead的next是cur
cur = next;//将cur后移
}
head.next = reverseHead.next;
}
双向链表
单向链表的局限性:只能单向遍历,需要依靠temp进行一系列操作
双向链表:在原有的基础上添加pre属性
package com.Eeeeye.linkedlist;
public class DoubleLinkedListDemo {
public static void main(String[] args) {
HeroNode2 hero1 = new HeroNode2(1, "宋江", "及时雨");
HeroNode2 hero2 = new HeroNode2(2, "卢俊义", "玉麒麟");
HeroNode2 hero3 = new HeroNode2(3, "吴用", "智多星");
HeroNode2 hero4 = new HeroNode2(4, "林冲", "豹子头1");
HeroNode2 hero5 = new HeroNode2(2, "林冲", "豹子头2");
DoubleLinkedList doubleLinkedList = new DoubleLinkedList();
/*
doubleLinkedList.add(hero1);
doubleLinkedList.add(hero2);
doubleLinkedList.add(hero4);
doubleLinkedList.add(hero3);
doubleLinkedList.list();
doubleLinkedList.del(3);
doubleLinkedList.list();
doubleLinkedList.update(hero5);
doubleLinkedList.list();
*/
doubleLinkedList.addByOrder(hero3);
doubleLinkedList.addByOrder(hero4);
doubleLinkedList.addByOrder(hero1);
doubleLinkedList.addByOrder(hero2);
doubleLinkedList.list();
}
}
class DoubleLinkedList {
private HeroNode2 head = new HeroNode2(0, "", "");
//添加节点到单向链表
//当不考虑编号顺序时
//1.找到当前链表的最后节点
//2.将最后这个节点的next 指向 新的节点
public HeroNode2 getHead() {
return head;
}
public void list() {
if (head.next == null) {
System.out.println("链表为空");
return;
}
HeroNode2 temp = head.next;
while (true) {
if (temp == null) {
break;
}
System.out.println(temp);
temp = temp.next;
}
}
//添加
public void add(HeroNode2 heroNode) {
//因为head节点不动,故需要一个辅助"临时"的变量
HeroNode2 temp = head;
while (true) {
if (temp.next == null) {
break;
}
//如果还未找到最后一个节点,将temp后移
temp = temp.next;
}//while循环结束
//将输入的heroNode赋值给新的一个节点
temp.next = heroNode;
heroNode.pre = temp;
}
//修改
public void update(HeroNode2 newheroNode) {
//先判断链表是否为空,再遍历查找匹配的heroNode
if (head.next == null) {
System.out.println("链表为空");
return;
}
HeroNode2 temp = head.next;
boolean flag = false;
while (true) {
if (null == temp) {
System.out.println("已到底");
break;
} else if (temp.no == newheroNode.no) {
System.out.println("已捕获");
flag = true;
break;
}
temp = temp.next;
}
if (flag) {
temp.nickname = newheroNode.nickname;
temp.name = newheroNode.name;
} else {
System.out.println("链表中无此节点%d,无法修改" + newheroNode.no);
}
}
//按序添加
public void addByOrder(HeroNode2 heroNode) {
//因为单链表,所以应该找插入的前一个节点
HeroNode2 temp = head;//确保temp在插入的点的前面
boolean flag = false;//默认为编号存在可添加
while (true) {
if (temp.next == null) {//说明temp已经在链表最后
break;
}
if (temp.next.no > heroNode.no) {//位置已找到,添加到temp和temp.next中间
break;
} else if (temp.no == heroNode.no) {
flag = true;
break;
}
temp = temp.next;
}
if (flag) {
System.out.println("已存在" + heroNode.no);
} else {
//通过下面两个代码将新节点插入
heroNode.next = temp.next;//使得新节点指向temp的下一个节点
heroNode.pre = temp;//倒过来可以吗
//不可以,上面这一行代码是将temp指向新节点
temp.next = heroNode;//若缺少这一行会出现空指针异常
temp.next.pre = heroNode;
}
}
//删除链表中的节点
public void del(int no) {
HeroNode2 temp = head.next;
boolean flag = false;//标志着默认是未找到删除的节点
while (true) {
//说明链表为空
if (temp == null) {
break;
}
if (temp.no == no) {
System.out.println("已找到删除的链表节点");
flag = true;
break;
}
temp = temp.next;
}
if (flag) {
temp.pre.next = temp.next;
//如果temp.next==null,再调用temp.next.pre会出现空指针异常
if (temp.next != null) {
temp.next.pre = temp.pre;
}
} else {
System.out.println("要删除的节点%d不存在" + no);
}
}
}
class HeroNode2 {
public int no;
public String name;
public String nickname;
public HeroNode2 next;
public HeroNode2 pre;
public HeroNode2(int no, String name, String nickname) {
this.no = no;
this.name = name;
this.nickname = nickname;
}
@Override
public String toString() {
//注意重写的toSrting方法无需带有next,否则会一直next导致第一行就输出了所有节点
return "HeroNode [no=" + no + ",name=" + name + ", nickname=" + nickname + "]";
}
}
单向环形链表(约瑟夫)
package com.Eeeeye.linkedlist;
public class Josephu {
public static void main(String[] args) {
CircleSingleLinkedList circleSingleLinkedList = new CircleSingleLinkedList();
circleSingleLinkedList.addBoy(5);
circleSingleLinkedList.showBoy();
}
}
class CircleSingleLinkedList {
private Boy first = null;
public void addBoy(int nums) {
if (nums < 1) {
System.out.println("nums的值不正确");
return;
}
//创建辅助指针
Boy curBoy = null;
//使用for循环进行遍历
for (int i = 1; i <= nums; i++) {
//根据编号创建小孩节点
Boy boy = new Boy(i);
if (i == 1) {
first = boy;
first.setNext(first);//考虑一下是否等效于boy.setNext(boy);
curBoy = first;
} else {
curBoy.setNext(boy);
boy.setNext(first);
curBoy = boy;//将Curboy向后推移
}
}
}
public void showBoy() {
if (first == null) {
System.out.println("没有任何小孩");
return;
}
Boy curBoy = first;
while (true){
System.out.printf("小孩的编号%d \n",curBoy.getNo());
if (curBoy.getNext() == first){
break;
}
curBoy = curBoy.getNext();//使curBoy后移
}
}
}
class Boy {
private int no ;
private Boy next;
public Boy() {
}
public Boy(int no) {
this.no = no;
}
public String toString() {
return "Boy{no = " + no + "}";
}
/**
* 获取
* @return no
*/
public int getNo() {
return no;
}
/**
* 设置
* @param no
*/
public void setNo(int no) {
this.no = no;
}
/**
* 获取
* @return next
*/
public Boy getNext() {
return next;
}
/**
* 设置
* @param next
*/
public void setNext(Boy next) {
this.next = next;
}
}
出圈
public void countBoy(int startNo, int countNum, int nums) {
if (startNo < 1 || first == null || startNo > nums) {
System.out.println("参数输入有误");
return;
}
//创建辅助指针,并使它在first的前一个位置
Boy helper = first;
while (true) {
if (helper.getNext() == first) {
break;
}
helper = helper.getNext();
}
//使first移动到开始报数的节点
for (int i = 0; i < startNo - 1; i++) {
helper = helper.getNext();
first = first.getNext();
}
//当小孩报数时,先让first和helper指针同时移动countNum-1次,然后出圈
//这是一个死循环直到圈中只剩一个节点
while (true) {
if (helper == first) {//此时只剩一个节点
break;
}
for (int i = 0; i < countNum - 1; i++) {
helper = helper.getNext();
first = first.getNext();
}
//此时first指向的节点就是要出圈的小孩节点
System.out.printf("小孩出圈编号为%d\n", first.getNo());
//执行出圈的操作(类似删除)
first = first.getNext();
helper.setNext(first);//将helper所在节点指向新的first
}//结束while死循环
System.out.printf("最后留在圈中的小孩编号%d\n",first.getNo());
}