队列的知识点
队列的特点:
队列只能操纵队头队尾元素
数据先进先出,(数据在队尾插入,从队头取出)
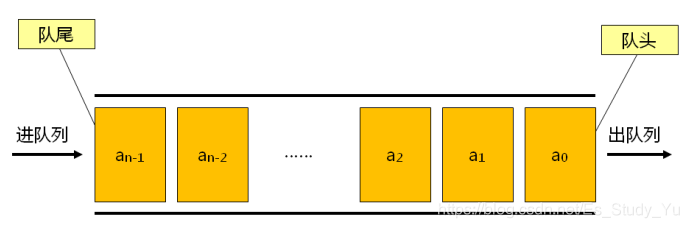
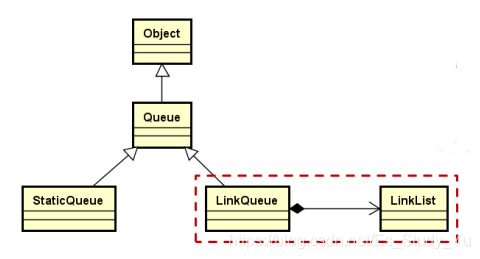
Queue
Queue.h
#ifndef __Queue_H_
#define __Queue_H_
#include "Object.h"
namespace JYlib
{
/*
抽象父类 队列(先进先出)
*/
template < typename T >
class Queue : public Object
{
public:
virtual void add(const T& e) =0;
virtual void remove() =0;
virtual T front()const =0;
virtual void clear() =0;
virtual int length()const =0;
};
}
#endif
StaticQueue
StaticQueue的特点:
队列容量大小固定(通过模板参数确定)
队列内的数据是拷贝过来的,与原对象无关
静态队列初始化时,(原生数组)会进行无参构造,数据加入时发生拷贝构造效率低下
#ifndef __StaticQueue_H_
#define __StaaticQueue_H_
#include "Queue.h"
#include "Exception.h"
namespace JYlib
{
/*
顺序队
使用循环队列方式,队列呈环形
出队列后不需要移动队列至0处,仅移动队头标志,效率提高
*/
template < typename T,int N >
class StaticQueue : public Queue<T>
{
protected:
T m_space[N];
int m_front;//队头标识
int m_rear;//队尾标识
int m_length;//当前队列长度
public:
StaticQueue()
{
m_front = 0;
m_rear = 0;
m_length = 0;
}
int capacity()const
{
return N;
}
void add(const T& e)
{
if(m_length < N)
{
m_space[m_rear] = e;
m_rear = (m_rear + 1) % N;
m_length++;
}
else
{
THROW_EXCEPTION(InvalidParameterException,"NO space in current queue ...");
}
}
void remove()
{
if(m_length > 0)
{
m_front = (m_front + 1) % N;
m_length--;
}
else
{
THROW_EXCEPTION(InvalidOperationException,"NO element in current queue ...");
}
}
T front()const
{
if(m_length>0)
{
return m_space[m_front];
}
else
{
THROW_EXCEPTION(InvalidOperationException,"NO element in current queue ...");
}
}
void clear()
{
m_front = 0;
m_rear = 0;
m_length = 0;
}
int length()const
{
return m_length;
}
};
}
#endif
LinkQueue
链式队的特点:
队列容量大小可以一直拓展
组合使用LinuxList(Linux的双向循环链表),插入与删除都无需遍历链表(比单链表效率高)
队列内的数据是通过链表指针指向的,与原对象有关
链式队列初始化时,仅仅是初始化链表,与指针指向数据,避免了无参构造以及拷贝构造,效率高
#ifndef __LinkQueue_H_
#define __LinkQueue_H_
#include "include/LinuxList.h"
#include "include/Queue.h"
#include "include/Exception.h"
namespace JYlib
{
/*
链式队
使用LinuxList(双向循环链表)
插入与删除都无需遍历链表,提高效率
*/
template < typename T >
class LinkQueue : public Queue<T>
{
protected:
struct Node : public Object
{
list_head head;
T value;
};
list_head m_header;
int m_length;
public:
LinkQueue()
{
INIT_LIST_HEAD(&m_header);
m_length = 0;
}
void add(const T& e)//增加至队尾
{
Node* new_node = new Node();
if(new_node != NULL)
{
new_node->value = e;
list_add_tail(&new_node->head,&m_header);
m_length++;
}
else
{
THROW_EXCEPTION(NoEnoughMemoryException,"No memory to add new element ...");
}
}
void remove()//移除首元素
{
if(m_length > 0)
{
list_head* remove = m_header.next;
list_del(remove);
m_length--;
delete list_entry(remove,Node,head);
}
else
{
THROW_EXCEPTION(InvalidOperationException,"NO element in current queue ...");
}
}
T front()const//返回队首值
{
if(m_length > 0)
{
return list_entry(m_header.next,Node,head)->value;
}
else
{
THROW_EXCEPTION(InvalidOperationException,"NO element in current queue ...");
}
}
void clear()
{
while(m_length > 0)
{
remove();
}
}
int length()const
{
return m_length;
}
~LinkQueue()
{
clear();
}
};
}
#endif