地图技术概述:
地图应用场景:
网约车行业:
智能穿戴产品:
智能物流网行业:
智能景区行业:
车联网行业:
国内常见的地图厂商比较:
百度地图基本API应用:
百度地图提供了各种平台的SDK,地址:https://lbsyunbaidu.com/如下:
设置测试的应用名称与类型: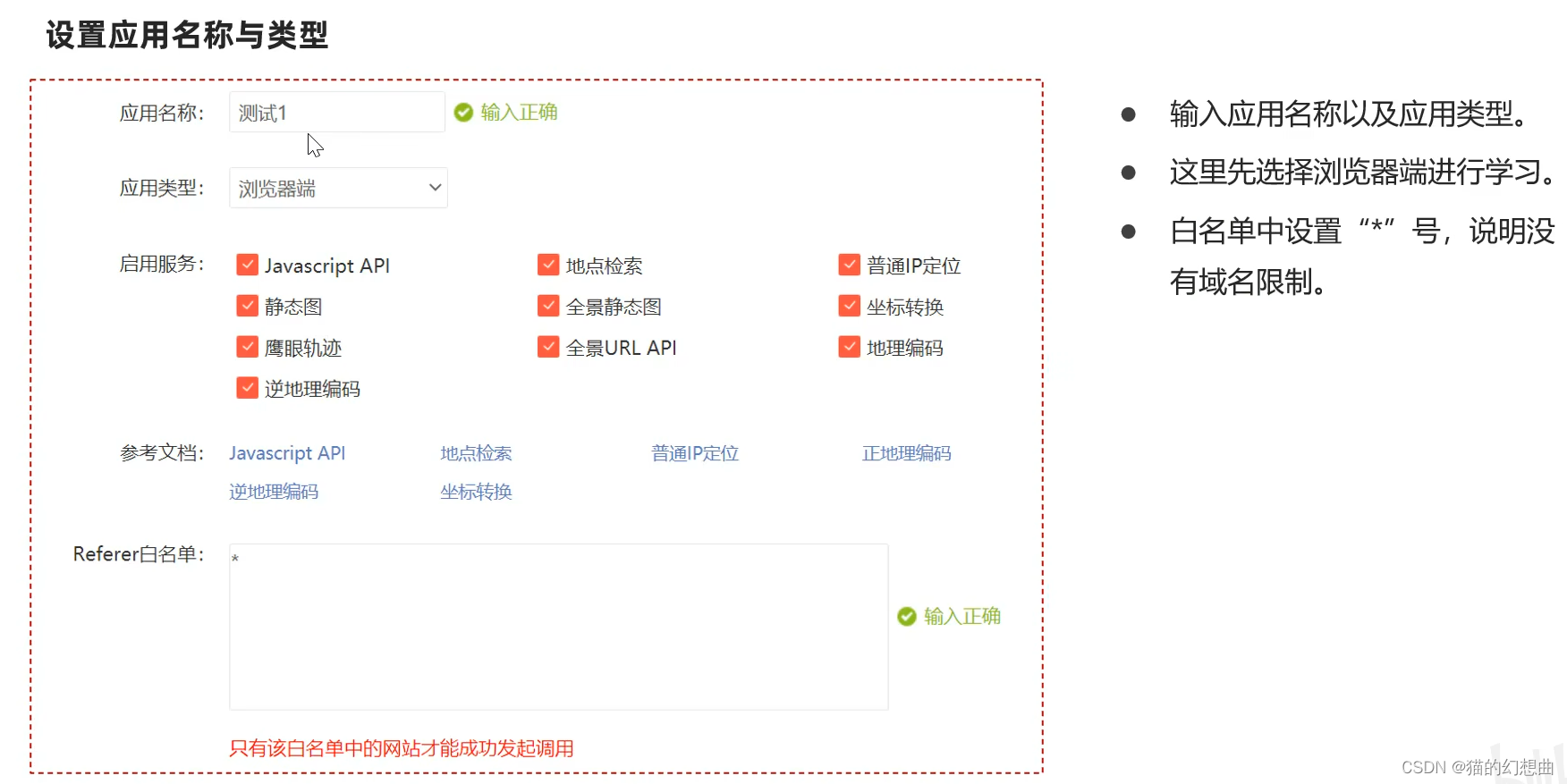
1.创建地图:
创建地图的基本步骤如下:
- 编写HTML页面的基本代码
- 引入百度地图API文件
- 初始化地图逻辑以及设置各种参数
参考官方文档:https://lbsyun.baidu.com/index.php?title=jspopularGL/guide/show
2.添加控件:
控件是负责与地图交互的UI元素,百度地图API支持比例尺、缩放、定位、城市选择列表、版权,以及自定义控件。
文档:https://lbsyun.baidu.com/index.php?title=jspopularGL/guide/widget
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no" />
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Baidu Map </title>
<style type="text/css">
html{height:100%}
body{height:100%;margin:0px;padding:0px}
#container{height:100%}
</style>
<script type="text/javascript" src="https://api.map.baidu.com/api?v=1.0&type=webgl&ak=自己的ak值"></script>
</head>
<body>
<div id="container"></div>
<script type="text/javascript">
var map = new BMapGL.Map("container"); // 创建地图实例
var point = new BMapGL.Point(116.404, 39.915); // 创建点坐标
map.centerAndZoom(point, 15); // 初始化地图,设置中心点坐标和地图级别
map.enableScrollWheelZoom(true); //开启鼠标滚轮缩放
map.setHeading(64.5); //设置地图旋转角度
map.setTilt(70); //设置地图的倾斜角度
var scaleCtrl = new BMapGL.ScaleControl(); // 添加比例尺控件
map.addControl(scaleCtrl);
var zoomCtrl = new BMapGL.ZoomControl(); // 添加缩放控件
map.addControl(zoomCtrl);
var cityCtrl = new BMapGL.CityListControl(); // 添加城市列表控件
map.addControl(cityCtrl);
</script>
</body>
</html>
3.添加覆盖物:
所有叠加或覆盖到地图的内容,我们统称为地图覆盖物。覆盖物拥有自己的地理坐标,当您拖动或缩放地图时,它们会相应的移动。目前JSAPI GL版支持的覆盖物以基本图形为主。
文档:https://lbsyun.baidu.com/index.php?title=jspopularGL/guide/addOverlay
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no" />
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Baidu Map - 覆盖物</title>
<style type="text/css">
html{height:100%}
body{height:100%;margin:0px;padding:0px}
#container{height:100%}
</style>
<script type="text/javascript" src="https://api.map.baidu.com/api?v=1.0&type=webgl&ak=自己的ak值"></script>
</head>
<body>
<div id="container"></div>
<script type="text/javascript">
var map = new BMapGL.Map("container"); // 创建地图实例
var point = new BMapGL.Point(116.404, 39.915); // 创建点坐标
map.centerAndZoom(point, 15); // 初始化地图,设置中心点坐标和地图级别
map.enableScrollWheelZoom(true); //开启鼠标滚轮缩放
// var marker = new BMapGL.Marker(point); // 创建标注
// map.addOverlay(marker); // 将标注添加到地图中
var myIcon = new BMapGL.Icon("logo.png", new BMapGL.Size(180, 53), {
// 指定定位位置。
// 当标注显示在地图上时,其所指向的地理位置距离图标左上
// 角各偏移10像素和25像素。您可以看到在本例中该位置即是
// 图标中央下端的尖角位置。
anchor: new BMapGL.Size(10, 25),
// 设置图片偏移。
// 当您需要从一幅较大的图片中截取某部分作为标注图标时,您
// 需要指定大图的偏移位置,此做法与css sprites技术类似。
imageOffset: new BMapGL.Size(0, 0 - 25) // 设置图片偏移
});
// 创建标注对象并添加到地图
var marker = new BMapGL.Marker(point, {icon: myIcon});
map.addOverlay(marker);
marker.addEventListener("click", function(){
alert("您点击了标注");
});
//折线
var polyline = new BMapGL.Polyline([
new BMapGL.Point(116.399, 39.910),
new BMapGL.Point(116.405, 39.920),
new BMapGL.Point(116.425, 39.900)
], {strokeColor:"blue", strokeWeight:2, strokeOpacity:0.5});
map.addOverlay(polyline);
//多边形
var polygon = new BMapGL.Polygon([
new BMapGL.Point(116.387112,39.920977),
new BMapGL.Point(116.385243,39.913063),
new BMapGL.Point(116.394226,39.917988),
new BMapGL.Point(116.401772,39.921364),
new BMapGL.Point(116.41248,39.927893)
], {strokeColor:"blue", strokeWeight:2, strokeOpacity:0.5});
map.addOverlay(polygon);
//圆形
var circle = new BMapGL.Circle(point,1000,{
strokeColor:"red",
strokeOpacity:0.8,
strokeWeight:3
});
map.addOverlay(circle);
</script>
</body>
</html>
4.地图事件:
百度地图API拥有一个自己的事件模型,程序员可监听地图API对象的自定义事件,使用方法和DOM事件类似。但请注意API事件是独立的,与标准DOM事件不同。
文档:https://lbsyun.baidu.com/index.php?title=jspopularGL/guide/event
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no" />
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Baidu Map - 事件</title>
<style type="text/css">
html{height:100%}
body{height:100%;margin:0px;padding:0px}
#container{height:100%}
</style>
<script type="text/javascript" src="https://api.map.baidu.com/api?v=1.0&type=webgl&ak=自己的ak值"></script>
</head>
<body>
<div id="container"></div>
<script type="text/javascript">
var map = new BMapGL.Map("container"); // 创建地图实例
var point = new BMapGL.Point(116.404, 39.915); // 创建点坐标
map.centerAndZoom(point, 15); // 初始化地图,设置中心点坐标和地图级别
map.enableScrollWheelZoom(true); //开启鼠标滚轮缩放
// map.addEventListener('click', function(e) {
// alert('click!')
// });
map.addEventListener('click', function(e) {
alert('点击的经纬度:' + e.latlng.lng + ', ' + e.latlng.lat);
var mercator = map.lnglatToMercator(e.latlng.lng, e.latlng.lat);
alert('点的墨卡托坐标:' + mercator[0] + ', ' + mercator[1]);
});
</script>
</body>
</html>
5.地图样式:
百度地图支持设置地图样式,比如将地图设置为地球模式,
文档:https://lbsyun.baidu.com/index.php?title=jspopularGL/guide/maptype
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no" />
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Baidu Map - 样式</title>
<style type="text/css">
html{height:100%}
body{height:100%;margin:0px;padding:0px}
#container{height:100%}
</style>
<script type="text/javascript" src="https://api.map.baidu.com/api?v=1.0&type=webgl&ak=自己的ak值"></script>
</head>
<body>
<div id="container"></div>
<script type="text/javascript">
var map = new BMapGL.Map("container"); // 创建地图实例
var point = new BMapGL.Point(116.404, 39.915); // 创建点坐标
map.centerAndZoom(point, 15); // 初始化地图,设置中心点坐标和地图级别
map.enableScrollWheelZoom(true); //开启鼠标滚轮缩放
map.setMapType(BMAP_EARTH_MAP); // 设置地图类型为地球模式
</script>
</body>
</html>
6.地图检索:
百度地图支持在地图中兴趣点的检索服务,示例:
https://lbsyun.baidu.com/jsdemohtm#localSearchKey
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no" />
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Baidu Map - 搜索</title>
<style type="text/css">
html{height:100%}
body{height:100%;margin:0px;padding:0px}
#container{height:100%}
</style>
<script type="text/javascript" src="https://api.map.baidu.com/api?v=1.0&type=webgl&ak=自己的ak值"></script>
</head>
<body>
<div id="container"></div>
<script type="text/javascript">
var map = new BMapGL.Map("container"); // 创建地图实例
var point = new BMapGL.Point(116.404, 39.915); // 创建点坐标
map.centerAndZoom(point, 15); // 初始化地图,设置中心点坐标和地图级别
map.enableScrollWheelZoom(true); //开启鼠标滚轮缩放
var circle = new BMapGL.Circle(point,1000,{fillColor:"blue", strokeWeight: 1 ,fillOpacity: 0.3, strokeOpacity: 0.3});
map.addOverlay(circle);
var local = new BMapGL.LocalSearch(map, {renderOptions: {map: map, autoViewport: false}});
local.searchNearby('景点',point,1000);
</script>
</body>
</html>
7.数据可视化:
MapVGL,是一款基于WebGL的地理信息可视化库,可以用来展示大量基于3D的地理信息点线面数据。
文档:https://lbsyun.baidu.com/solutions/mapvdata
百度地图web API应用:
百度地图Web服务API为开发者提供http/https接口,即开发者通过http/https形式发起检索请求,获取返回json或xml格式的检索数据。用户可以基于此开发JavaScript、C#、C++、Java等语言的地图应用。
地址:https://lbsyun.baidu.com/index.php?title=webapi
![]() | ![]() | ![]() |
创建web类型应用:
1.坐标转换
目前中国主要有以下三种坐标系:
- WGS84:为一种大地坐标系,也是目前广泛使用的GPS全球卫星定位系统使用的坐标系
- GCJ02:是由中国国家测绘局制定的地理信息系统的坐标系系统,由WGS84坐标系经加密后的坐标系
- BD09:为百度坐标系,在GCJ02坐标系基础上再次加密,其中bd09ll表示百度纬度坐标,bd09mc表示百度墨卡托米制坐标
Web api中提供了将非百度坐标转换成百度坐标体系的接口服务。
文档:https://lbsyun.baidu.com/index.php?title=webapi/guide/changeposition
private String ak = "您自己的ak值";
/**
* 测试坐标转换服务
*/
@Test
public void testGeoconv(){
String url = "https://api.map.baidu.com/geoconv/v1/?coords=114.21892734521,29.575429778924&from=1&to=5&ak={} ";
url = StrUtil.format(url,ak);
//发起get请求
String body = HttpRequest.get(url).execute().body();
System.out.println(body);
}
2.IP定位服务:
普通ip定位是一套以HTTP/HTTPS形式提供的轻量级定位接口,用户可以通过该服务,根据IP定位来获取大致的位置。
文档:https://lbsyun.baidu.com/index.php?title=webapi/ip-api
/**
* 测试ip定位
*/
@Test
public void testLocation(){
String url = "https://api.map.baidu.com/location/ip?ak={}&ip=140.206.149.83&coor=bd09ll ";
url = StrUtil.format(url,ak);
//发起get请求
String body = HttpRequest.get(url).execute().body();
System.out.println(body);
}
3.地点输入提示服务:
用户可通过该服务,匹配用户输入关键词的地点推荐列表。
文档:https://lbsyun.baidu.com/index.php?title=webapi/place-suggestion-api
/**
* 测试地点输入提示服务
*/
@Test
public void testSuggestion(){
String url = "https://api.map.baidu.com/place/v2/suggestion?query=清华大®ion=北京&city_limit=true&output=json&ak={}";
url = StrUtil.format(url,ak);
//发起get请求
String body = HttpRequest.get(url).execute().body();
System.out.println(body);
}
4.路线规划:
路线规划服务(又名Direction API)是一套REST风格的Web服务API,以HTTP/HTTPS形式提供了路线规划服务,目前,Direction API支持公交、骑行、驾车路线规划,Direction API支持中国大陆地区。
文档:https://lbsyun.baidu.com/index.php?title=webapi/direction-api-v2
/**
* 测试路线规划
*/
@Test
public void testDriving(){
String url = "https://api.map.baidu.com/direction/v2/driving?alternatives=0&origin=40.009645,116.333374&destination=39.937016,116.453576&ak={}";
url = StrUtil.format(url,ak);
//发起get请求
String body = HttpRequest.get(url).execute().body();
System.out.println(body);
}
百度地图的基本API使用以及WebApi的应用实例。
文档说明,及供参考。