效果图
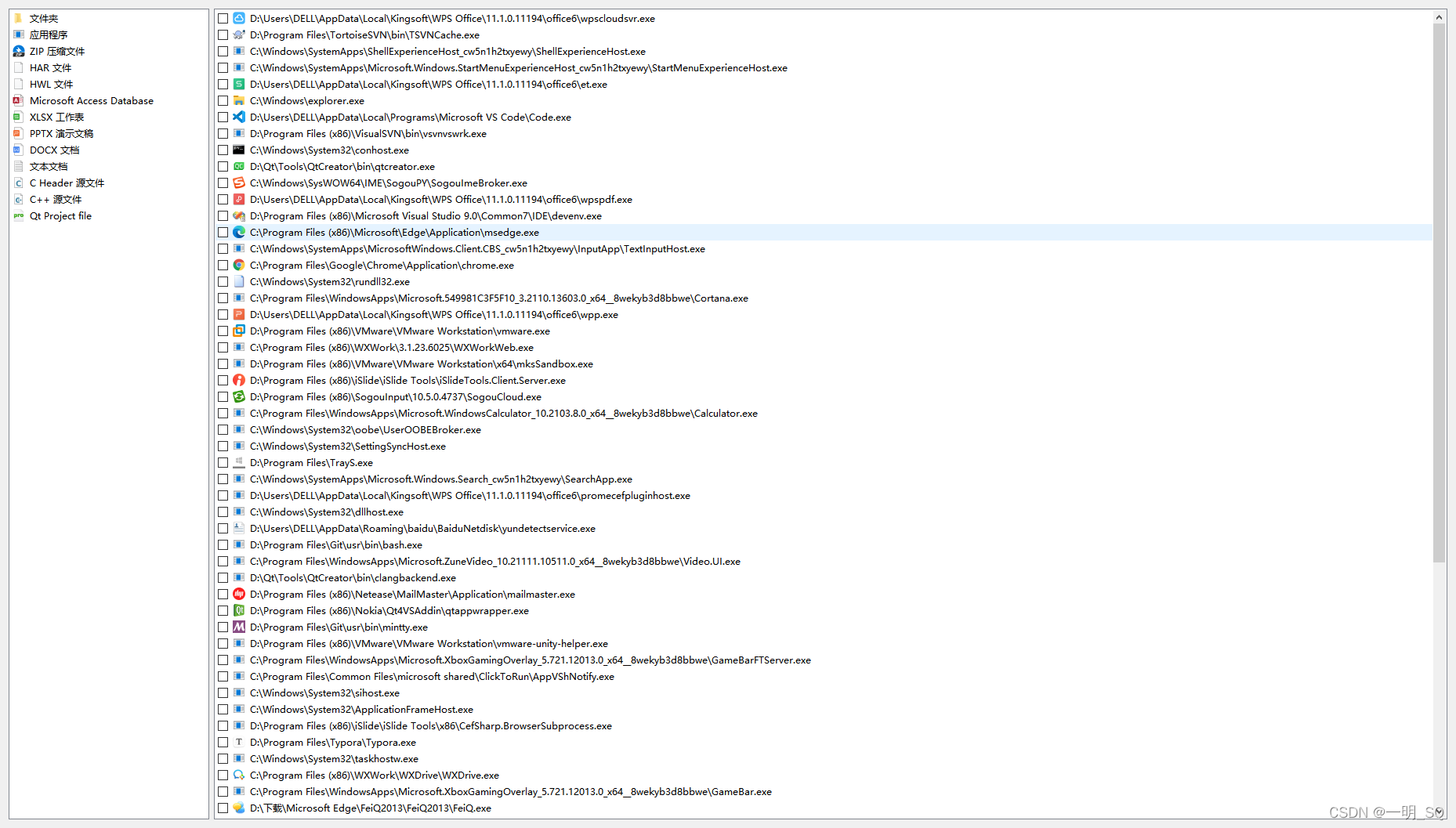
运行和编译环境
- Win 10
- Qt 5.9 MSVC2017 64位
- gitee源码👈 clone后可直接编译
git clone https://gitee.com/fole-del/qt_-process-with-icon_show.git
Qt 获取当前系统进程并展示
实现手段
- 调用Win API先获取系统当前的进程名和PID
- 根据进程
PID
(PROCESSENTRY32W.th32ProcessID
)获取进程全路径,全路径接口为GetModuleFileNameEx
Icon
:获取Icon并显示,根据第二步获取的全路径,依旧是调用系统API,获取Icon
,得到HICON句柄,使用Qt的QtWin::fromHICON
接收即可获取QIcon
。
获取Icon函数
HICON QueryExeIcon(LPCTSTR lpszExePath)
{
HICON hIcon = NULL;
SHFILEINFO FileInfo;
DWORD_PTR dwRet = ::SHGetFileInfo(lpszExePath, 0, &FileInfo, sizeof(SHFILEINFO), SHGFI_ICON);
if (dwRet)
{
hIcon = FileInfo.hIcon;
}
return hIcon;
}
获取进程相关函数
int Widget::getAllProcess()
{
int countProcess = 0;
HANDLE hProcessSnap = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
PROCESSENTRY32W currentProcess;
currentProcess.dwSize = sizeof(currentProcess);
HANDLE hProcess = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
if (hProcess == INVALID_HANDLE_VALUE)
{
printf("CreateToolhelp32Snapshot()调用失败!\n");
return -1;
}
bool bMore = Process32First(hProcessSnap, ¤tProcess);
while (bMore) {
QString str;
TCHAR szProcessName[MAX_PATH] = { 0 };
GetProcessFullPath(currentProcess.th32ProcessID, szProcessName, str);
m_ProList.append(str);
bMore = Process32Next(hProcessSnap, ¤tProcess);
countProcess++;
}
CloseHandle(hProcess);
}
源码
widget.h
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include <QtWin>
#include <QIcon>
#include <QString>
#include <QListWidget>
#include <QListWidgetItem>
#include <QTableWidgetItem>
#include <QHBoxLayout>
#include <QMessageBox>
#include <QDebug>
#include <QStandardItemModel>
#include <QTableWidget>
#include <QTableView>
#include <QSet>
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
public:
int getAllProcess();
private:
void getExtIcon();
void getProIcon();
private:
QListWidget *m_ExtListWidget;
QListWidget *m_ProListWidget;
QHBoxLayout *m_Hbox;
private:
QStringList m_ProList;
};
#endif
widget.cpp
#include "widget.h"
#include <iostream>
#include <windows.h>
#include <tlhelp32.h>
#include <string>
#include <stdio.h>
#include <tchar.h>
#include <Psapi.h>
#pragma comment (lib,"Psapi.lib")
using namespace std;
#ifdef UNICODE
#define QStringToTCHAR(x) (wchar_t*) x.utf16()
#define PQStringToTCHAR(x) (wchar_t*) x->utf16()
#define TCHARToQString(x) QString::fromUtf16((x))
#define TCHARToQStringN(x,y) QString::fromUtf16((x),(y))
#else
#define QStringToTCHAR(x) x.local8Bit().constData()
#define PQStringToTCHAR(x) x->local8Bit().constData()
#define TCHARToQString(x) QString::fromLocal8Bit((x))
#define TCHARToQStringN(x,y) QString::fromLocal8Bit((x),(y))
#endif
BOOL DosPathToNtPath(LPTSTR pszDosPath, LPTSTR pszNtPath)
{
TCHAR szDriveStr[500];
TCHAR szDrive[3];
TCHAR szDevName[100];
INT cchDevName;
INT i;
if (!pszDosPath || !pszNtPath)
return FALSE;
if (GetLogicalDriveStrings(sizeof(szDriveStr), szDriveStr))
{
for (i = 0; szDriveStr[i]; i += 4)
{
if (!wcscmp(&(szDriveStr[i]), (L"A:\\")) || !wcscmp(&(szDriveStr[i]), _T(L"B:\\")))
continue;
szDrive[0] = szDriveStr[i];
szDrive[1] = szDriveStr[i + 1];
szDrive[2] = '\0';
if (!QueryDosDevice(szDrive, szDevName, 100))
return FALSE;
cchDevName = lstrlen(szDevName);
if ((pszDosPath, szDevName, cchDevName) == 0)
{
lstrcpy(pszNtPath, szDrive);
lstrcat(pszNtPath, pszDosPath + cchDevName);
return TRUE;
}
}
}
lstrcpy(pszNtPath, pszDosPath);
return FALSE;
}
BOOL GetProcessFullPath(DWORD dwPID, TCHAR pszFullPath[MAX_PATH], __out QString& str)
{
HANDLE hProcess = OpenProcess(PROCESS_ALL_ACCESS, FALSE, dwPID);
if (!hProcess)
{
str = "";
}
WCHAR filePath[MAX_PATH];
DWORD ret= GetModuleFileNameEx(hProcess, NULL, filePath, MAX_PATH) ;
QString file = QString::fromStdWString( filePath );
CloseHandle(hProcess);
str = ret == 0 ? "" : file;
return true;
}
string GetProcessInfo(__in HANDLE hProcess, __in WCHAR* processName)
{
PROCESSENTRY32* pinfo = new PROCESSENTRY32;
MODULEENTRY32* minfo = new MODULEENTRY32;
char shortpath[MAX_PATH];
int flag = Process32First(hProcess, pinfo);
while (flag) {
if (wcscmp(pinfo->szExeFile, processName) == 0) {
HANDLE hModule = CreateToolhelp32Snapshot(
TH32CS_SNAPMODULE,
pinfo->th32ProcessID
);
Module32First(
hModule,
minfo
);
GetShortPathName(
minfo->szExePath,
(LPWSTR)shortpath,
256
);
return shortpath;
}
flag = Process32Next(hProcess, pinfo);
}
return NULL;
}
int Widget::getAllProcess()
{
int countProcess = 0;
HANDLE hProcessSnap = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
PROCESSENTRY32W currentProcess;
currentProcess.dwSize = sizeof(currentProcess);
HANDLE hProcess = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
if (hProcess == INVALID_HANDLE_VALUE)
{
printf("CreateToolhelp32Snapshot()调用失败!\n");
return -1;
}
bool bMore = Process32First(hProcessSnap, ¤tProcess);
while (bMore) {
QString str;
TCHAR szProcessName[MAX_PATH] = { 0 };
GetProcessFullPath(currentProcess.th32ProcessID, szProcessName, str);
m_ProList.append(str);
bMore = Process32Next(hProcessSnap, ¤tProcess);
countProcess++;
}
CloseHandle(hProcess);
}
HICON fileIcon(std::string extention)
{
HICON icon = NULL;
if (extention.length() > 0)
{
LPCSTR name = extention.c_str();
SHFILEINFOA info;
if (SHGetFileInfoA(name,
FILE_ATTRIBUTE_NORMAL,
&info,
sizeof(info),
SHGFI_SYSICONINDEX | SHGFI_ICON | SHGFI_USEFILEATTRIBUTES))
{
icon = info.hIcon;
}
}
return icon;
}
HICON QueryExeIcon(LPCTSTR lpszExePath)
{
HICON hIcon = NULL;
SHFILEINFO FileInfo;
DWORD_PTR dwRet = ::SHGetFileInfo(lpszExePath, 0, &FileInfo, sizeof(SHFILEINFO), SHGFI_ICON);
if (dwRet)
{
hIcon = FileInfo.hIcon;
}
return hIcon;
}
std::string fileType(std::string extention)
{
std::string type = "";
if (extention.length() > 0)
{
LPCSTR name = extention.c_str();
SHFILEINFOA info;
if (SHGetFileInfoA(name,
FILE_ATTRIBUTE_NORMAL,
&info,
sizeof(info),
SHGFI_TYPENAME | SHGFI_USEFILEATTRIBUTES))
{
type = info.szTypeName;
}
}
return type;
}
HICON folderIcon()
{
std::string str = "folder";
LPCSTR name = str.c_str();
HICON icon = NULL;
SHFILEINFOA info;
if (SHGetFileInfoA(name,
FILE_ATTRIBUTE_DIRECTORY,
&info,
sizeof(info),
SHGFI_SYSICONINDEX | SHGFI_ICON | SHGFI_USEFILEATTRIBUTES))
{
icon = info.hIcon;
}
return icon;
}
std::string folderType()
{
std::string str = "folder";
LPCSTR name = str.c_str();
std::string type;
SHFILEINFOA info;
if (SHGetFileInfoA(name,
FILE_ATTRIBUTE_DIRECTORY,
&info,
sizeof(info),
SHGFI_TYPENAME | SHGFI_USEFILEATTRIBUTES))
{
type = info.szTypeName;
}
return type;
}
Widget::Widget(QWidget *parent)
: QWidget(parent)
{
setGeometry(200, 200, 800, 800);
setSizePolicy(QSizePolicy::Preferred,QSizePolicy::Preferred);
m_Hbox = new QHBoxLayout(this);
m_ExtListWidget = new QListWidget;
m_ExtListWidget->setSizePolicy(QSizePolicy::Maximum,QSizePolicy::Preferred);
m_ProListWidget = new QListWidget;
m_ProListWidget->setSizePolicy(QSizePolicy::Preferred,QSizePolicy::Preferred);
m_Hbox->addWidget(m_ExtListWidget);
m_Hbox->addWidget(m_ProListWidget);
m_Hbox->setStretch(0,3);
m_Hbox->setStretch(1,7);
getExtIcon();
getProIcon();
}
Widget::~Widget()
{
}
void Widget::getExtIcon()
{
m_ExtListWidget->setViewMode(QListView::ListMode);
std::string strArray[13] = {"folder", ".exe", ".zip", ".har", ".hwl", ".accdb",
".xlsx", ".pptx", ".docx", ".txt", ".h", ".cpp", ".pro"};
int nCount = sizeof(strArray) / sizeof(std::string);
for (int i = 0; i < nCount ; ++i)
{
QPixmap pixmap;
std::string type;
int nPos = -1;
nPos = strArray[i].find(".");
if (nPos >= 0)
{
pixmap = QtWin::fromHICON(fileIcon(strArray[i]));
type = fileType(strArray[i]);
}
else
{
pixmap = QtWin::fromHICON(folderIcon());
type = folderType();
}
QIcon icon;
icon.addPixmap(pixmap);
QString strType = QString::fromLocal8Bit(type.c_str());
QListWidgetItem *pItem = new QListWidgetItem;
pItem->setIcon(icon);
pItem->setText(strType);
m_ExtListWidget->addItem(pItem);
}
}
void Widget::getProIcon()
{
getAllProcess();
QSet<QString> SetList;
for(auto it : m_ProList)
{
SetList.insert(it);
}
int nCount = SetList.size();
foreach(const QString& value, SetList)
{
if(value.isEmpty())
{
continue;
}
QPixmap pixmap;
std::string type;
pixmap = QtWin::fromHICON(QueryExeIcon(value.toStdWString().c_str()));
type = fileType(value.toStdString());
QIcon icon;
icon.addPixmap(pixmap);
QString strType = value;
QListWidgetItem *pItem = new QListWidgetItem;
pItem->setCheckState(Qt::Unchecked);
pItem->setIcon(icon);
pItem->setText(strType);
m_ProListWidget->addItem(pItem);
}
}