结构:
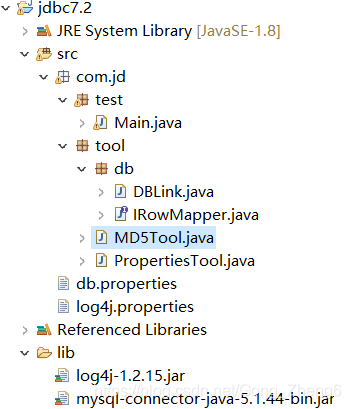
Main:
package com.jd.test;
import java.util.Scanner;
import java.util.UUID;
import com.jd.tool.MD5Tool;
import com.jd.tool.db.DBLink;
public class Main {
public static void main(String[] args) {
System.out.println("*********************************");
System.out.println("*\t\t\t\t*");
System.out.println("*\t欢迎使用注册登录系统\t*");
System.out.println("*\t\t\t\t*");
System.out.println("*********************************");
while (true) {
menu();
}
}
static void menu() {
System.out.println("1、注册");
System.out.println("2、登录");
System.out.println("3、退出");
System.out.println("请输入操作,以Enter键结束:");
Scanner scanner = new Scanner(System.in);
int option = scanner.nextInt();
DBLink dblink = new DBLink();
switch (option) {
case 1:{
dblink.getConnection();
System.out.println("请输入用户名:");
String userName = scanner.next();
System.out.println("请输入密码:");
String password = scanner.next();
System.out.println("请确认密码:");
String repassword = scanner.next();
String sql = "select id from user_info where user_name=?";
if(dblink.exist(sql,userName)) {
System.out.println("用户名已存在");
return;
}
if(!password.equals(repassword)) {
System.out.println("密码与确认密码不一致");
return;
}
String id = UUID.randomUUID().toString();
sql = "insert into user_info (id,user_name,password) values ('"+id+"',?,?)";
password = new MD5Tool().encrypt(password);
if(dblink.update(sql,userName,password)) {
System.out.println("注册成功");
return;
}
System.out