一、编码格式介绍
1.1、常见的字符编码格式
1.2、Python字符编码格式
Python的解释器使用的是Unicode(内存)
.py文件在磁盘上使用UTF-8存储(外存)
#encoding=gbk
print('你好,中国')
二、文件的读写原理
2.1、文件的读写
文件的读写俗称“IO操作”,即输入输出操作
2.2、文件读写操作流程
2.3、操作原理
三、文件读写操作
3.1、内置函数open()创建文件对象
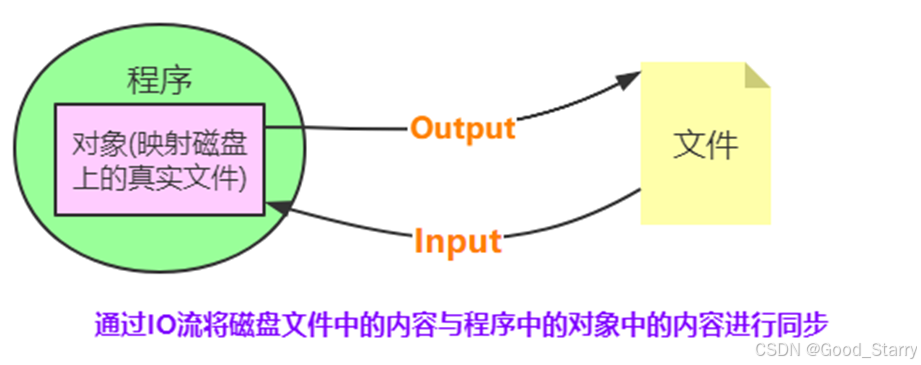
3.2、语法规则
file = open(filename [,mode,encoding])
3.3、文件的类型
按文件中数据的组织形式,文件分为以下两大类
1、文本文件:存储的是普通“字符”文本,默认为unicode字符集,可以使用记本事程序打开
2、二进制文件:把数据内容用“字节”进行存储,无法用记事本打开,必须使用专用的软件打开 ,举例:mp3音频文件,jpg图片 .doc文档等
3.4、常用的文件打开模式
file=open('a.txt','r')
print(file.readlines())
file.close()
file=open('b.txt','w')
file.write('Python')
file.close()
file=open('b.txt','a')
file.write('Python')
file.close()
src_file=open('logo.png','rb')
target_file=open('copylogo.png','wb')
target_file.write(src_file.read())
target_file.close()
src_file.close()
四、文件对象常用的方法
4.1、常用方法
4.2、示例
file=open('a.txt','r')
#print(file.read(2))
#print(file.readline())
print(file.readlines())
file.close()
file=open('c.txt','a')
#file.write('hello')
lst=['java','go','python']
file.writelines(lst)
file.close()
file=open('c.txt','r')
file.seek(2)
print(file.read())
print(file.tell())
file.close()
file=open('d.txt','a')
file.write('hello')
file.flush()
file.write('world')
file.close()
file=open('d.txt','a')
file.write('hello')
file.close()
#file.write('world')
#file.flush()
五、with语句(上下文管理器)
5.1、with语句
with语句可以自动管理上下文资源,不论什么原因跳出with块,都能确保文件正确的关闭,以此来达到释放资源的目的
with open('a.txt','r') as file:
print(file.read())
with open('logo.png','rb') as src_file:
with open('copy2logo.png','wb') as target_file:
target_file.write(src_file.read())
5.2、上下文管理器
MyContentMgr实现了特殊方法__enter__(),__exit__()称为该类对象遵守了上下文管理器协议
该类对象的实例对象,称为上下文管理器
class MyContentMgr(object):
def __enter__(self):
print('enter方法被调用执行了')
return self
def __exit__(self, exc_type, exc_val, exc_tb):
print('exit方法被调用执行了')
def show(self):
print('show方法被调用执行了',1/0)
with MyContentMgr() as file: #相当于file=MyContentMgr()
file.show()
六、目录操作
6.1、os模块
1、os模块是Python内置的与操作系统功能和文件系统相关的模块,该模块中的语句的执行结果通常与操作系统有关,在不同的操作系统上运行,得到的结果可能不一样。
2、os模块与os.path模块用于对目录或文件进行操作
3、示例
#os模块与操作系统相关的一个模块
import os
#os.system('notepad.exe')
#os.system('calc.exe')
#直接调用可执行文件
os.startfile('C:\\Program Files\\Tencent\QQ\\Bin\\qq.exe')
6.2、os模块操作目录相关函数
6.2.1、相关函数
import os
print(os.getcwd())
lst=os.listdir('../chap15')
print(lst)
#os.mkdir('newdir2')
#os.makedirs('A/B/C')
#os.rmdir('newdir2')
#os.removedirs('A/B/C')
os.chdir('E:\\vippython\\chap15')
print(os.getcwd())
import os
path=os.getcwd()
lst=os.listdir(path)
for filename in lst:
if filename.endswith('.py'):
print(filename)
6.2.2、os.walk()函数
import os
path=os.getcwd()
lst_files=os.walk(path)
for dirpath,dirname,filename in lst_files:
'''print(dirpath)
print(dirname)
print(filename)
print('-------------------------------------')'''
for dir in dirname:
print(os.path.join(dirpath,dir))
for file in filename:
print(os.path.join(dirpath,file))
print('----------------------------------')
6.3、os.path模块操作目录相关函数
import os.path
print(os.path.abspath('demo13.py'))
print(os.path.exists('demo13.py'),os.path.exists('demo18.py'))
print(os.path.join('E:\\Python','demo13.py'))
print(os.path.split('E:\\vipython\\chap15\\demo13.py'))
print(os.path.splitext('demo13.py'))
print(os.path.basename('E:\\vippython\\chap15\\demo13.py'))
print(os.path.dirname('E:\\vippython\\chap15\\demo13.py'))
print(os.path.isdir('E:\\vippython\\chap15\\demo13.py'))