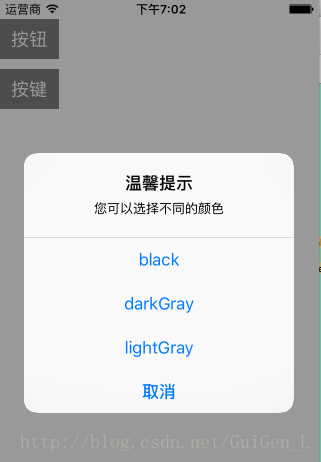
UIButton* btn = [UIButton buttonWithType:UIButtonTypeCustom];
btn.frame = CGRectMake(0, 20, 60, 40);
btn.backgroundColor = [UIColor grayColor];
[btn setTitle:@"按钮" forState:UIControlStateNormal];
[btn addTarget:self action:@selector(btnAction:) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:btn];
UIButton* btn1 = [UIButton buttonWithType:UIButtonTypeCustom];
btn1.frame = CGRectMake(0, 70, 60, 40);
btn1.backgroundColor = [UIColor grayColor];
[btn1 setTitle:@"按键" forState:UIControlStateNormal];
[btn1 addTarget:self action:@selector(btnAction1:) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:btn1];
_alertView = [[UIAlertView alloc]initWithTitle:@"温馨提示" message:@"您可以选择不同的颜色" delegate:self cancelButtonTitle:@"取消" otherButtonTitles:@"black",@"darkGray",@"lightGray", nil];
_alertView.tag = 1;
[self.view addSubview:_alertView];
_alertView1 = [[UIAlertView alloc]initWithTitle:@"温馨提示" message:@"您可以选择不同的颜色" delegate:self cancelButtonTitle:@"取消" otherButtonTitles:@"green",@"blue",@"cyan", nil];
_alertView1.tag = 2;
[self.view addSubview:_alertView1];
调用的方法:
-(void)btnAction:(UIButton*)sender
{
UIAlertView* alertView = [self.view viewWithTag:1];
[alertView show];
}
-(void)btnAction1:(UIButton*)sender
{
UIAlertView* alertView1 = [self.view viewWithTag:2];
[alertView1 show];
}
//取消按钮的索引值固定为0,其他按钮从上往下依次是1,2.。。。。。。
- (void)alertView:(UIAlertView *)alertView clickedButtonAtIndex:(NSInteger)buttonIndex
{
NSLog(@"----%ld",buttonIndex);
//获取被点击按钮的标题,参数是按钮的索引值
NSString* title = [alertView buttonTitleAtIndex:buttonIndex];
NSLog(@"====%@",title);
//isEqualToString 判断两个字符串是否相等,返回的是BOOL的值
//第一种方法⚠️
/*
if (alertView.tag == 1) {
switch (buttonIndex) {
case 1:
self.view.backgroundColor = [UIColor blackColor];
break;
case 2:
self.view.backgroundColor = [UIColor darkGrayColor];
break;
case 3:
self.view.backgroundColor = [UIColor lightGrayColor];
break;
case 0://点取消键
self.view.backgroundColor = [UIColor whiteColor];
break;
default:
break;
}
}
if (alertView.tag == 2) {
switch (buttonIndex) {
case 1:
self.view.backgroundColor = [UIColor greenColor];
break;
case 2:
self.view.backgroundColor = [UIColor blueColor];
break;
case 3:
self.view.backgroundColor = [UIColor cyanColor];
break;
case 0://点取消键
self.view.backgroundColor = [UIColor whiteColor];
break;
default:
break;
}
}
*/
//第二种方法⚠️
//按钮
/*
if ([title isEqualToString:@"black"]) {
self.view.backgroundColor = [UIColor blackColor];
}
if ([title isEqualToString:@"darkGray"]) {
self.view.backgroundColor = [UIColor darkGrayColor];
}
if ([title isEqualToString:@"lightGray"]) {
self.view.backgroundColor = [UIColor lightGrayColor];
}
if ([title isEqualToString:@"取消"]) {
self.view.backgroundColor = [UIColor whiteColor];
}
//按键
if ([title isEqualToString:@"green"]) {
self.view.backgroundColor = [UIColor greenColor];
}
if ([title isEqualToString:@"blue"]) {
self.view.backgroundColor = [UIColor blueColor];
}
if ([title isEqualToString:@"cyan"]) {
self.view.backgroundColor = [UIColor cyanColor];
}
if ([title isEqualToString:@"取消"]) {
self.view.backgroundColor = [UIColor whiteColor];
}
*/
//第三种方法
if (alertView == _alertView) {
switch (buttonIndex) {
case 1:
self.view.backgroundColor = [UIColor blackColor];
break;
case 2:
self.view.backgroundColor = [UIColor darkGrayColor];
break;
case 3:
self.view.backgroundColor = [UIColor lightGrayColor];
break;
case 0://点取消键
self.view.backgroundColor = [UIColor whiteColor];
break;
default:
break;
}
}
if (alertView == _alertView1) {
switch (buttonIndex) {
case 1:
self.view.backgroundColor = [UIColor greenColor];
break;
case 2:
self.view.backgroundColor = [UIColor blueColor];
break;
case 3:
self.view.backgroundColor = [UIColor cyanColor];
break;
case 0://点取消键
self.view.backgroundColor = [UIColor whiteColor];
break;
default:
break;
}
}
/*
switch (buttonIndex) {
case 1:
self.view.backgroundColor = [UIColor redColor];
break;
case 2:
self.view.backgroundColor = [UIColor greenColor];
break;
case 3:
self.view.backgroundColor = [UIColor blackColor];
break;
case 0:
self.view.backgroundColor = [UIColor whiteColor];
break;
default:
break;
}
*/