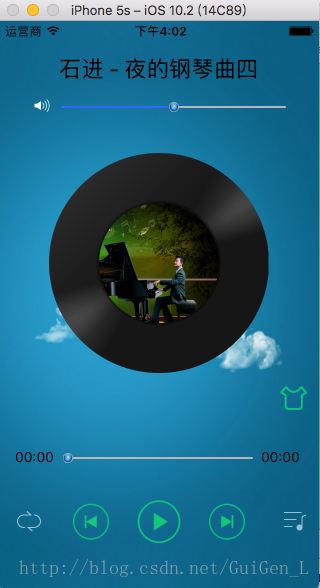
我添加的是这几首歌曲
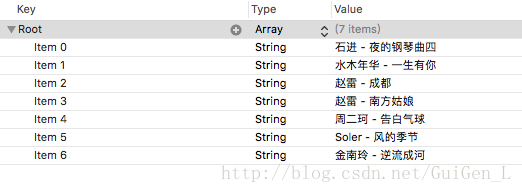
1.主界面和歌曲列表都是拖控件的
2.ViewController里的代码
#import "ViewController.h"
#import "MusicList_ViewController.h"
#import <AVFoundation/AVFoundation.h>
@interface ViewController ()<AVAudioPlayerDelegate>
@property (weak, nonatomic) IBOutlet UILabel *nameLabel;
@property (weak, nonatomic) IBOutlet UILabel *timeLabel;
@property (weak, nonatomic) IBOutlet UILabel *timeLabel2;
@property (weak, nonatomic) IBOutlet UISlider *volumeSlider;
@property (weak, nonatomic) IBOutlet UISlider *processSlider;
@property (weak, nonatomic) IBOutlet UIButton *transmitBTN;
@property (weak, nonatomic) IBOutlet UIButton *PlaytheorderAction;
@property (weak, nonatomic) IBOutlet UIImageView *Setting;
@property (weak, nonatomic) IBOutlet UIImageView *imageView;
@property (weak, nonatomic) IBOutlet UIImageView *imageView2;
@property (retain)AVAudioPlayer* player;
@property (retain)NSString* musicName;
@property (assign)BOOL isBool;
@property (assign)NSInteger number;
@property (retain)NSMutableArray* dataSource;
@property (assign)NSInteger Musicorder;
@property (assign)NSInteger backInt;
@property (assign)double Turn;
@property (retain)NSArray* SingerpicturesArr;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
[_volumeSlider setThumbImage:[UIImage imageNamed:@"TB"] forState:UIControlStateNormal];
[_processSlider setThumbImage:[UIImage imageNamed:@"TB"] forState:UIControlStateNormal];
NSString* path1 = [[NSBundle mainBundle]pathForResource:@"Song information" ofType:@"plist"];
NSArray* arr = [NSArray arrayWithContentsOfFile:path1];
_dataSource = [NSMutableArray arrayWithArray:arr];
NSString* path = [[NSBundle mainBundle]pathForResource:@"石进 - 夜的钢琴曲四" ofType:@"mp3"];
NSURL* url = [NSURL fileURLWithPath:path];
_player = [[AVAudioPlayer alloc]initWithContentsOfURL:url error:nil];
_player.delegate = self;
[_player prepareToPlay];
_Musicorder = 0;
[[NSNotificationCenter defaultCenter]addObserver:self selector:@selector(changeMusic:) name:@"music" object:nil];
[[NSNotificationCenter defaultCenter]addObserver:self selector:@selector(subscriptMusic:) name:@"subscript" object:nil];
NSTimer* timer2 = [NSTimer scheduledTimerWithTimeInterval:0.1 target:self selector:@selector(TurnthePicture) userInfo:nil repeats:YES];
_SingerpicturesArr = @[@"1_1",@"2_2",@"3_3",@"4_4",@"5_5",@"6_6",@"7_7"];
_imageView2.layer.cornerRadius = _imageView2.frame.size.width/2;
_imageView2.layer.masksToBounds = YES;
}
-(void)changeMusic:(NSNotification*)notication
{
_musicName = notication.object;
NSLog(@"notication ===== %@",notication.object);
[self playMusic];
}
-(void)subscriptMusic:(NSNotification*)notication
{
_number = [notication.object integerValue];
NSLog(@"notication.object ==== %@", notication.object);
NSLog(@"_number ==== %ld", (long)_number);
_imageView2.image = [UIImage imageNamed:_SingerpicturesArr[_number]];
}
- (IBAction)shuffleplayAction:(UIButton *)sender {
NSLog(@"播放顺序");
NSArray* arr = @[@"player_btn_repeat_normal",@"player_btn_repeatone_normal",@"player_btn_random_normal"];
if (_Musicorder != 2) {
_Musicorder++;
}else{
_Musicorder = 0;
}
[_PlaytheorderAction setImage:[UIImage imageNamed:arr[_Musicorder]] forState:UIControlStateNormal];
}
- (IBAction)OnaAction:(UIButton *)sender {
NSLog(@"上一首");
if (_Musicorder == 2) {
NSLog(@"随机播放===================");
NSInteger RandomPlay = arc4random()%_dataSource.count;
NSLog(@"RandomPlay === %ld", (long)RandomPlay);
if (_number != RandomPlay) {
_number = RandomPlay;
}else{
_number = arc4random()%_dataSource.count;
}
_musicName = _dataSource[_number];
_imageView2.image = [UIImage imageNamed:_SingerpicturesArr[_number]];
}else{
if (_number != 0) {
_number--;
}else{
_number = _dataSource.count-1;
}
_musicName = _dataSource[_number];
_imageView2.image = [UIImage imageNamed:_SingerpicturesArr[_number]];
}
[self playMusic];
}
- (IBAction)playmusicAction:(UIButton *)sender {
_isBool =! _isBool;
if (_isBool) {
[_player play];
NSTimer* timer = [NSTimer scheduledTimerWithTimeInterval:0.1 target:self selector:@selector(updateUI) userInfo:nil repeats:YES];
[_transmitBTN setImage:[UIImage imageNamed:@"hp_player_btn_pause_normal"] forState:UIControlStateNormal];
}else{
[_player stop];
[_transmitBTN setImage:[UIImage imageNamed:@"hp_player_btn_play_normal"] forState:UIControlStateNormal];
}
}
- (IBAction)ThefollowingpieceAction:(UIButton *)sender {
NSLog(@"下一首");
if (_Musicorder == 2) {
NSLog(@"随机播放===================");
NSInteger RandomPlay = arc4random()%_dataSource.count;
NSLog(@"RandomPlay === %ld", (long)RandomPlay);
if (_number != RandomPlay) {
_number = RandomPlay;
}else{
_number = arc4random()%_dataSource.count;
}
_musicName = _dataSource[_number];
_imageView2.image = [UIImage imageNamed:_SingerpicturesArr[_number]];
}else{
if (_number != _dataSource.count-1) {
_number++;
}else{
_number = 0;
}
_musicName = _dataSource[_number];
_imageView2.image = [UIImage imageNamed:_SingerpicturesArr[_number]];
}
[self playMusic];
}
-(void)TurnthePicture
{
if (_isBool)
{
_Turn=_Turn+0.01;
if (_Turn>6.28) {
_Turn=0;
}
_imageView.transform=CGAffineTransformMakeRotation(_Turn);
_imageView2.transform=CGAffineTransformMakeRotation(_Turn);
}
}
- (IBAction)ThesonglistAction:(UIButton *)sender {
MusicList_ViewController* musiclistVC = [[MusicList_ViewController alloc]init];
[self presentViewController:musiclistVC animated:YES completion:nil];
}
- (IBAction)volumeAction:(UISlider *)sender {
[_player setVolume:sender.value*100];
}
- (IBAction)processAction:(UISlider *)sender {
[_player stop];
_player.currentTime = sender.value*_player.duration;
}
- (IBAction)touchLeaveAcyion:(UISlider *)sender {
_isBool =! _isBool;
[self playmusicAction:nil];
}
-(void)updateUI
{
_processSlider.value = _player.currentTime/_player.duration;
NSInteger allmm = _player.duration/60;
NSInteger allss = (NSInteger)_player.duration%60;
NSInteger cuMM = _player.currentTime/60;
NSInteger cuSS = (NSInteger)_player.currentTime%60;
_timeLabel.text = [NSString stringWithFormat:@"%.2ld:%.2ld", (long)cuMM, (long)cuSS];
_timeLabel2.text = [NSString stringWithFormat:@"%.2ld:%.2ld", (long)allmm, (long)allss];
}
-(void)playMusic
{
_nameLabel.text = _musicName;
NSString* path = [[NSBundle mainBundle]pathForResource:_musicName ofType:@"mp3"];
NSURL* url = [NSURL fileURLWithPath:path];
if (_player) {
_player = nil;
}
_player = [[AVAudioPlayer alloc]initWithContentsOfURL:url error:nil];
_player.delegate = self;
[_player prepareToPlay];
_isBool = NO;
[self playmusicAction:nil];
if (_player.play) {
[_transmitBTN setImage:[UIImage imageNamed:@"hp_player_btn_pause_normal"] forState:UIControlStateNormal];
}
}
-(void)audioPlayerDidFinishPlaying:(AVAudioPlayer *)player successfully:(BOOL)flag
{
NSLog(@"播放完成");
if (_Musicorder == 0) {
NSLog(@"循环播放");
[self ThefollowingpieceAction:nil];
}else if(_Musicorder == 1){
NSLog(@"单曲循环");
_isBool = NO;
[self playmusicAction:nil];
}else if(_Musicorder == 2){
NSLog(@"随机播放===================");
NSInteger RandomPlay = arc4random()%_dataSource.count;
NSLog(@"RandomPlay === %ld", (long)RandomPlay);
if (_number != RandomPlay) {
_number = RandomPlay;
}else{
_number = arc4random()%_dataSource.count;
}
_musicName = _dataSource[_number];
_imageView2.image = [UIImage imageNamed:_SingerpicturesArr[_number]];
[self playMusic];
}
}
- (IBAction)TheSkinAction:(UIButton *)sender {
NSLog(@"pihu");
NSArray* settingArr = @[@"1",@"2",@"3",@"4",@"5",@"6",@"7",@"8",@"9",@"10"];
if (_backInt != settingArr.count-1) {
_backInt++;
}else{
_backInt = 0;
}
_Setting.image = [UIImage imageNamed:[NSString stringWithFormat:@"%@", settingArr[_backInt]]];
}
-(void)audioPlayerBeginInterruption:(AVAudioPlayer *)player
{
NSLog(@"音乐被突然中断,比如系统来电话了");
}
-(void)audioPlayerEndInterruption:(AVAudioPlayer *)player withOptions:(NSUInteger)flags
{
NSLog(@"中断以结束,可以正常播放音乐了");
[self playmusicAction:nil];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
}
@end
3.MusicList_ViewController(歌曲列表)里的代码
#import "MusicList_ViewController.h"
#import "ViewController.h"
@interface MusicList_ViewController ()<UITableViewDelegate,UITableViewDataSource>
@property (weak, nonatomic) IBOutlet UITableView *tableView;
@property (retain)NSMutableArray* dataSource;
@end
@implementation MusicList_ViewController
- (void)viewDidLoad {
[super viewDidLoad];
NSString* path = [[NSBundle mainBundle]pathForResource:@"Song information" ofType:@"plist"];
NSArray* arr = [NSArray arrayWithContentsOfFile:path];
_dataSource = [NSMutableArray arrayWithArray:arr];
UIImageView *backImageView=[[UIImageView alloc]initWithFrame:self.view.bounds];
[backImageView setImage:[UIImage imageNamed:@"2"]];
_tableView.backgroundView = backImageView;
}
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return _dataSource.count;
}
-(UITableViewCell*)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString* cellID = @"cell";
UITableViewCell* cell = [tableView dequeueReusableCellWithIdentifier:cellID];
if (cell == nil) {
cell = [[UITableViewCell alloc]initWithStyle:UITableViewCellStyleValue1 reuseIdentifier:cellID];
}
cell.textLabel.text = _dataSource[indexPath.row];
cell.backgroundColor = [UIColor clearColor];
return cell;
}
-(void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
[tableView deselectRowAtIndexPath:indexPath animated:YES];
[self dismissViewControllerAnimated:YES completion:nil];
[[NSNotificationCenter defaultCenter]postNotificationName:@"music" object:_dataSource[indexPath.row]];
[[NSNotificationCenter defaultCenter]postNotificationName:@"subscript" object:[NSString stringWithFormat:@"%ld", (long)indexPath.row]];
}
-(CGFloat)tableView:(UITableView *)tableView heightForHeaderInSection:(NSInteger)section
{
return 44;
}
-(CGFloat)tableView:(UITableView *)tableView heightForFooterInSection:(NSInteger)section
{
return 44;
}
-(UIView*)tableView:(UITableView *)tableView viewForHeaderInSection:(NSInteger)section
{
UILabel* label = [[UILabel alloc]initWithFrame:CGRectMake(0, 20, tableView.frame.size.width, 44)];
label.text = @"--歌曲列表--";
label.textAlignment = NSTextAlignmentCenter;
label.backgroundColor = [UIColor clearColor];
return label;
}
-(UIView*)tableView:(UITableView *)tableView viewForFooterInSection:(NSInteger)section
{
UIButton* btn = [UIButton buttonWithType:UIButtonTypeCustom];
btn.frame = CGRectMake(0, 0, tableView.frame.size.width, 44);
btn.backgroundColor = [UIColor clearColor];
[btn setTitle:@"返回" forState:UIControlStateNormal];
[btn addTarget:self action:@selector(btnAction:) forControlEvents:UIControlEventTouchUpInside];
return btn;
}
-(void)btnAction:(UIButton*)sender
{
[self dismissViewControllerAnimated:YES completion:nil];
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
}
@end