首先你要有两张星星图片
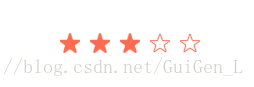
在ViewController中创建评论星星等级的滑杆
#import "ViewController.h"
#import "StarSliderView.h"
@interface ViewController ()<StarSLiderDelegate>
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
[self createStarSliderView];
}
#pragma mark - - 创建评论星星等级的滑杆
-(void)createStarSliderView{
StarSliderView *star = [[StarSliderView alloc]initWithFrame:CGRectMake(self.view.frame.size.width/4, 400, self.view.frame.size.width/2, 100) andWithCurrentStarNum:1 andUserEnabled:YES andWithDistance:10];
star.delegate = self;
[self.view addSubview:star];
}
#pragma mark - - 代理方法
-(void)starSliderMoveWithCurrentNum:(int)starNum{
NSLog(@"^^^^^^^^^%d^^^^^^^^^^^^^",starNum);
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
}
@end
在StarSliderView中星星滑动动画
#import <UIKit/UIKit.h>
@protocol StarSLiderDelegate <NSObject>
@required
-(void)starSliderMoveWithCurrentNum:(int)starNum;
@end
@interface StarSliderView : UIView
-(instancetype)initWithFrame:(CGRect)frame
andWithCurrentStarNum:(int)currentStarNum
andUserEnabled:(BOOL)nabled
andWithDistance:(float)distance;
@property(nonatomic,assign)float distance;
@property(nonatomic,strong)id<StarSLiderDelegate>delegate;
@end
#import "StarSliderView.h"
@interface StarSliderView(){
NSMutableArray *_imgViewArray;
}
@end
#define kStarsNum 5
#define kstarImgWitch (self.frame.size.width-_distance*(kStarsNum+1))/kStarsNum
@implementation StarSliderView
-(instancetype)initWithFrame:(CGRect)frame andWithCurrentStarNum:(int)currentStarNum andUserEnabled:(BOOL)nabled andWithDistance:(float)distance{
self = [super initWithFrame:frame];
if (self) {
self.userInteractionEnabled = nabled;
_distance =distance;
[self createView];
if (currentStarNum != 0 &¤tStarNum <= kStarsNum) {
[self highlightStarShow:currentStarNum];
}
}
return self;
}
#pragma mark - -
-(void)createView{
_imgViewArray = [NSMutableArray array];
for (int i = 0; i<kStarsNum; i++) {
UIImageView *imgViewNomal = [[UIImageView alloc]initWithImage:[UIImage imageNamed:@"btn_star_evaluation_normal"]];
imgViewNomal.frame = CGRectMake(_distance+(kstarImgWitch+_distance)*i, 0, kstarImgWitch, kstarImgWitch);
imgViewNomal.tag = i;
[self addSubview:imgViewNomal];
UIImageView *imgViewSeleted = [[UIImageView alloc]initWithImage:[UIImage imageNamed:@"btn_star_evaluation_press"]];
imgViewSeleted.frame = CGRectMake(_distance+(kstarImgWitch+_distance)*i, 0, kstarImgWitch, kstarImgWitch);
imgViewSeleted.hidden = YES;
imgViewSeleted.tag = i;
[self addSubview:imgViewSeleted];
[_imgViewArray addObject:imgViewSeleted];
}
self.frame = CGRectMake(self.frame.origin.x, self.frame.origin.y, self.frame.size.width, kstarImgWitch);
}
#pragma mark - -
- (void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event{
CGPoint pt = [[touches anyObject] locationInView:self];
[self showStarSlider:pt.x];
[self selectAnimal:pt.x];
}
#pragma mark - -
- (void)touchesMoved:(NSSet *)touches withEvent:(UIEvent *)event{
CGPoint pt = [[touches anyObject] locationInView:self];
[self showStarSlider:pt.x];
}
#pragma mark - -
- (void)touchesEnded:(NSSet *)touches withEvent:(UIEvent *)event{
CGPoint pt = [[touches anyObject] locationInView:self];
[self showStarSlider:pt.x];
[self selectAnimal:pt.x];
}
#pragma mark - - 展示滑杆
-(void)showStarSlider:(float)pt{
int num;
if (pt<_distance) {
for (int i = 0; i<_imgViewArray.count; i++) {
UIImageView *imgView = _imgViewArray[i];
imgView.hidden = YES;
}
num = 0;
}else if (pt>=(kstarImgWitch*kStarsNum+_distance*kStarsNum)){
for (int i = 0; i<_imgViewArray.count; i++) {
UIImageView *imgView = _imgViewArray[i];
imgView.hidden = NO;
}
num = kStarsNum;
}else{
int currentInt = pt/(kstarImgWitch+_distance);
for (int i =0; i<=currentInt; i++) {
UIImageView *imgView = _imgViewArray[i];
imgView.hidden = NO;
}
for (int i = currentInt+1; i<=_imgViewArray.count-1; i++) {
UIImageView *imgView = _imgViewArray[i];
imgView.hidden = YES;
}
num = currentInt +1;
}
if ( [_delegate respondsToSelector:@selector(starSliderMoveWithCurrentNum:)]) {
[_delegate starSliderMoveWithCurrentNum:num];
}
}
#pragma mark - - 简单动画效果
-(void)selectAnimal:(float)pt{
UIImageView *imgViewanimal = [[UIImageView alloc]initWithImage:[UIImage imageNamed:@"btn_star_evaluation_press"]];
imgViewanimal.frame = CGRectMake(pt,0, 19, 19);
imgViewanimal.alpha = 0.3;
[self addSubview:imgViewanimal];
[UIView animateWithDuration:0.5 animations:^{
imgViewanimal.frame = CGRectMake(pt,-30, 19, 19);
imgViewanimal.alpha = 0;
}];
[self performSelector:@selector(delayMethod:) withObject:imgViewanimal afterDelay:0.5];
}
#pragma mark - - 动画延时
-(void)delayMethod:(UIView*)view{
[view removeFromSuperview];
view = nil;
}
#pragma mark - -
-(void)highlightStarShow:(int)currentInt{
for (int i =0; i<=currentInt-1; i++) {
UIImageView *imgView = _imgViewArray[i];
imgView.hidden = NO;
}
for (int i = currentInt; i<=_imgViewArray.count-1; i++) {
UIImageView *imgView = _imgViewArray[i];
imgView.hidden = YES;
}
}
@end