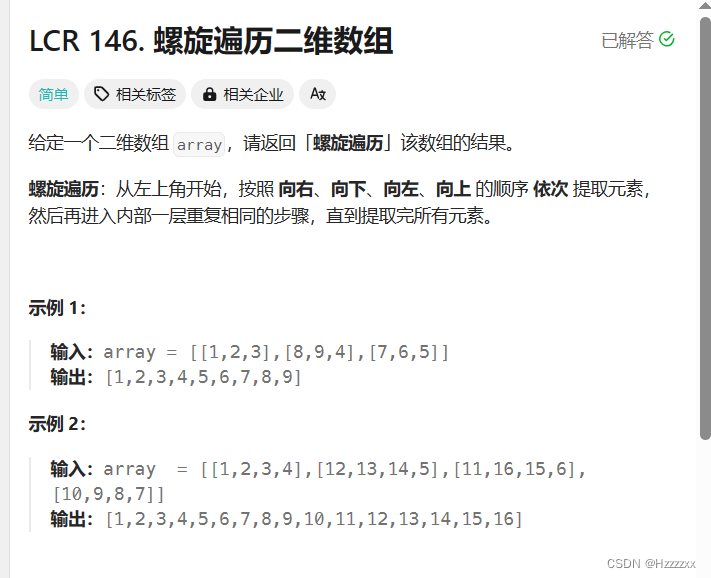
class Solution {
public:
vector<int> spiralArray(vector<vector<int>>& array) {
vector<int> v;
if(array.size() == 0 || array[0].size()==0) return v;
int top = 0;
int bottom = array.size()-1;
int left = 0;
int right = array[0].size()-1;
while(true)
{
for(int i = left;i<=right;i++)
{
v.push_back(array[top][i]);
}
if(++top > bottom) break;
for(int i = top;i<=bottom;i++)
{
v.push_back(array[i][right]);
}
if(--right < left) break;
for(int i = right;i>=left;i--)
{
v.push_back(array[bottom][i]);
}
if(--bottom < top) break;
for(int i = bottom;i>=top;i--)
{
v.push_back(array[i][left]);
}
if(++left > right) break;
}
return v;
}
};
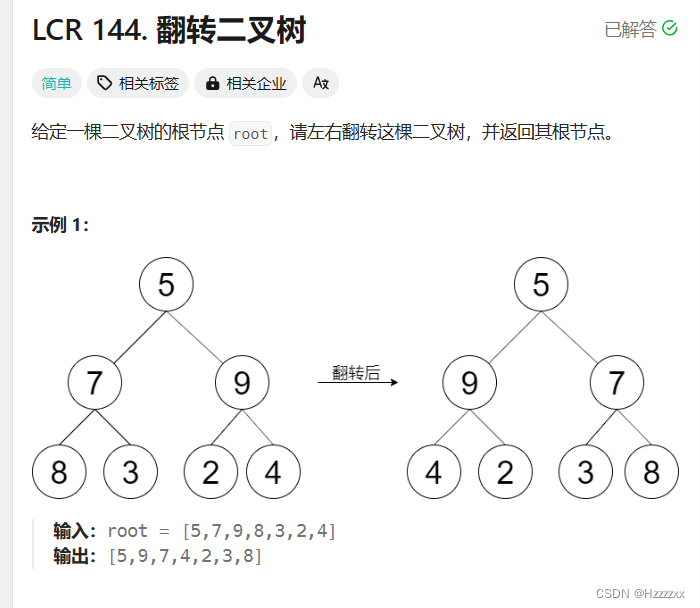
class Solution {
public:
TreeNode* mirrorTree(TreeNode* root) {
if(!root) return root;
TreeNode* tmp = root->right;
root->right = mirrorTree(root->left);
root->left = mirrorTree(tmp);
return root;
}
};