C++基础 通讯录管理系统源码 -vs stdio
源码:
#include <iostream>
using namespace std;
struct TellPerson {
string name;
int sex;
int age;
string tell;
string address;
};
struct TellNotice {
struct TellPerson person_cont[1000];
int m_Size;
};
void UI()
{
cout << "***************************" << endl;
cout << "***** 1、添加联系人 *****" << endl;
cout << "***** 2、显示联系人 *****" << endl;
cout << "***** 3、删除联系人 *****" << endl;
cout << "***** 4、查找联系人 *****" << endl;
cout << "***** 5、修改联系人 *****" << endl;
cout << "***** 6、清空联系人 *****" << endl;
cout << "***** 0、退出通讯录 *****" << endl;
cout << "***************************" << endl;
}
int check(TellNotice *tn,string name) {
for (int i = 0; i < tn->m_Size;i++) {
if (tn->person_cont[i].name == name)
{
return i;
}
}
return -1;
}
void add(TellNotice *tn) {
string name;
cout << "请输入姓名:" << endl;
cin >> name;
tn->person_cont[tn->m_Size].name = name;
cout << "请输入性别:" << endl;
cout << "1 -- 男" << endl;
cout << "2 -- 女" << endl;
while (true) {
int sex = 0;
cin >> sex;
if (sex == 1 || sex == 2)
{
tn->person_cont[tn->m_Size].sex = sex;
break;
}
cout << "输入有误,请重新输入";
}
cout << "请输入年龄:" << endl;
int age = 0;
cin >> age;
tn->person_cont[tn->m_Size].age = age;
cout << "请输入联系电话:" << endl;
string phone = "";
cin >> phone;
tn->person_cont[tn->m_Size].tell = phone;
cout << "请输入家庭住址:" << endl;
string address;
cin >> address;
tn->person_cont[tn->m_Size].address = address;
tn->m_Size++;
cout << "添加成功" << endl;
system("pause");
system("cls");
}
void showInfo(TellNotice *tn) {
if (tn->m_Size == 0)
{
cout << "当前记录为空" << endl;
}
else
{
for (int i = 0; i < tn->m_Size; i++)
{
cout << "姓名:" << tn->person_cont[i].name << "\t";
cout << "性别:" << (tn->person_cont[i].sex == 1 ? "男" : "女") << "\t";
cout << "年龄:" << tn->person_cont[i].age << "\t";
cout << "电话:" << tn->person_cont[i].tell << "\t";
cout << "住址:" << tn->person_cont[i].address << endl;
}
}
system("pause");
system("cls");
}
void del(TellNotice *tn) {
cout << "请输入想要删除人的姓名:" << endl;
string name = "";
cin >> name;
int jieguo=check(tn,name);
if (jieguo != -1) {
for (int i = jieguo; i < tn->m_Size; i++) {
tn->person_cont[i] = tn->person_cont[i + 1];
}
tn->m_Size--;
cout << name << "已被删除" << endl;
}
else
{
cout << "查无此人" << endl;
}
system("pause");
system("cls");
}
void search(TellNotice* tn) {
cout << "请输入想要查找人的姓名:" << endl;
string name = "";
cin >> name;
int jieguo = check(tn, name);
if (jieguo != -1) {
for (int i = jieguo; i < tn->m_Size; i++) {
cout << "姓名:" << tn->person_cont[i].name << "\t";
cout << "性别:" << (tn->person_cont[i].sex == 1 ? "男" : "女") << "\t";
cout << "年龄:" << tn->person_cont[i].age << "\t";
cout << "电话:" << tn->person_cont[i].tell << "\t";
cout << "住址:" << tn->person_cont[i].address << endl;
}
}
else
{
cout << "查无此人" << endl;
}
system("pause");
system("cls");
}
void update(TellNotice *tn) {
cout << "请输入想要修改人的姓名:" << endl;
string name = "";
cin >> name;
int jieguo = check(tn, name);
if (jieguo != -1) {
for (int i = jieguo; i < tn->m_Size; i++) {
string name;
cout << "请输入姓名:" << endl;
cin >> name;
tn->person_cont[jieguo].name = name;
cout << "请输入性别:" << endl;
cout << "1 -- 男" << endl;
cout << "2 -- 女" << endl;
int sex = 0;
while (true)
{
cin >> sex;
if (sex == 1 || sex == 2)
{
tn->person_cont[jieguo].sex = sex;
break;
}
cout << "输入有误,请重新输入";
}
cout << "请输入年龄:" << endl;
int age = 0;
cin >> age;
tn->person_cont[jieguo].age = age;
cout << "请输入联系电话:" << endl;
string phone = "";
cin >> phone;
tn->person_cont[jieguo].tell = phone;
cout << "请输入家庭住址:" << endl;
string address;
cin >> address;
tn->person_cont[jieguo].address = address;
cout << "修改成功" << endl;
}
}
else
{
cout << "查无此人" << endl;
}
system("pause");
system("cls");
}
void unset(TellNotice* tn) {
cout << "您确定要一键删除所有联系人吗?输入'是'继续" << endl;
string jieguo = "";
cin >> jieguo;
if (jieguo == "是") {
tn->m_Size = 0;
cout << "通讯录已清空" << endl;
system("pause");
system("cls");
}
else
{
cout << "输入错误" << endl;
}
system("pause");
system("cls");
}
int main() {
TellNotice tn;
tn.m_Size = 0;
int select = 0;
while (true) {
UI();
cin >> select;
switch (select)
{
case 0://退出
cout << "欢迎下次使用!" << endl;
system("pause");
return 0;
break;
case 1:add(&tn);
break;
case 2: showInfo(&tn);
break;
case 3:del(&tn);
break;
case 4: search(&tn);
break;
case 5:update(&tn);
break;
case 6: unset(&tn);
break;
default:
break;
}
}
system("pause");
return 0;
}
效果如下:
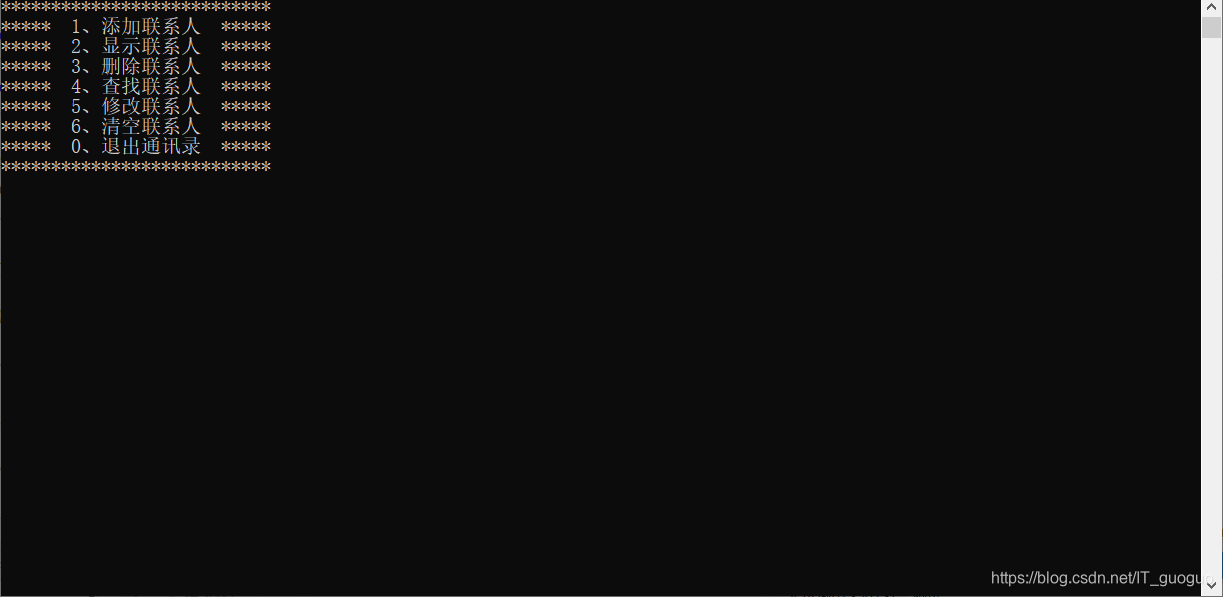
源码项目文件:
https://pan.baidu.com/s/1bo-RfPaNVq6qk9AjLrSNvA
提取码:m8y8