Java安全
密钥和证书
Java安全方面涉及的密钥(Key)和证书(Certificate)是保护数据的关键部分。密钥是用于加密和解密数据的一组数据,而证书是用于数字身份验证的一种数字凭证。
密钥
密钥是用于加密和解密数据的一组数据。密钥可以是对称密钥也可以是非对称密钥。
对称密钥是一种使用同一个密钥进行加解密的加密方法。对称密钥的加解密速度较快,但存在一个缺点,即发送方和接收方必须拥有相同的密钥才能进行通信,这对于密钥交换和公共密钥的保护会产生一定的风险。
非对称密钥是一种使用一组密钥进行加解密的加密方法。一组非对称密钥包括一个公钥和一个私钥,其中公钥可以公开,但私钥不能泄漏。发送方使用接收方的公钥进行加密,然后再由接收方使用私钥进行解密。非对称密钥相对于对称密钥来说更加安全,但加解密速度慢。
密钥生成
Java提供了三种类型的密钥:对称密钥、非对称密钥和密钥对。密钥的生成可以使用Java提供的密钥生成器(KeyGenerator)类、密钥对生成器(KeyPairGenerator)类、KeyFactory类等工具实现。
密钥生成器类可以用来生成对称密钥和非对称密钥,而密钥对生成器类只能用于生成非对称密钥对。
对称密钥生成的代码示例:
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
SecureRandom secureRandom = new SecureRandom();
keyGenerator.init(secureRandom);
SecretKey secretKey = keyGenerator.generateKey();
上述代码生成了一个AES算法的对称密钥。
非对称密钥生成的代码示例:
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
SecureRandom secureRandom = new SecureRandom();
keyPairGenerator.initialize(2048, secureRandom);
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
上述代码生成了一个RSA算法的非对称密钥对,并将公钥和私钥存储在两个变量中。
密钥工厂
密钥工厂(KeyFactory)类是Java提供的用于操作密钥对象的类。密钥工厂支持的操作包括将密钥对象转换为特定类型的密钥对象、将密钥对象编码为字节数组、从字节数组中生成密钥对象等。
密钥工厂类通常与密钥生成器一起使用,用于在不同的系统之间传输和共享密钥对象。
证书
证书是一种数字凭证,用于对数字身份进行验证。Java使用X.509证书格式。每个证书都包含一个公钥,一个私钥和相关的元数据。
证书可以分为自签名证书和第三方签名证书。自签名证书是由自己签名的证书,而第三方签名证书是由受信任的第三方机构签名的证书。在Java安全应用程序中,通常使用第三方签名证书。
证书一般由证书颁发机构(CA)签名后发放,其中包含证书颁发机构的信息、证书持有者的信息、公钥信息以及签名信息等。
密钥,证书与对象
Java Security API中的许多对象都可以使用相应的密钥、证书和别名进行保护。例如,Java
Keystore(密钥库)是一个文件,用于存储密钥和证书,在Java应用程序中使用它来管理证书和密钥。
Java Keystore还支持其他操作,如生成密钥对、添加和删除条目、导入和导出证书和密钥、列出库中的条目等。
序列化
Java中的对象序列化是将对象转换为字节序列以便于进行网络传输、存储和其他操作的过程。序列化包括将对象转换为字节数组,而反序列化则是将字节数组重新转换为对象。
在Java安全中,对象序列化可以用于保存和传输密钥(Key)对象和证书(Certificate)对象。序列化过程将密钥和证书转换为字节数组,而反序列化过程则是将字节数组重新转换为密钥和证书对象。
但是,在Java安全中,对象序列化也存在一些安全风险。攻击者可以修改序列化后的数据,从而破解密钥或者篡改数据。因此,在使用对象序列化时,需要注意安全性问题,特别是要防止针对序列化数据的攻击。
Java Security: Exploring Keys, Certificates, and Serialization
Introduction:
In the realm of Java security, understanding the concepts of keys,
certificates, and serialization is crucial. This article aims to provide a
comprehensive overview of these topics, highlighting their significance in
securing Java applications and data. We will delve into key generation, key
factories, certificates, and their relationship with objects. Additionally, we
will explore the role of serialization in Java security and its associated
techniques.
- Keys and Certificates:
1.1 Overview of Keys:
* Definition and importance of keys in encryption and decryption.
* Symmetric and asymmetric key types.
* Key generation techniques and best practices.
1.2 Understanding Certificates:
- Introduction to digital certificates and their purpose.
- X.509 standard certificates in Java.
- Components of a certificate: public key, identity information, and signature.
- Certificate authorities and the certificate chain.
- Key Generation and Key Factory:
2.1 Key Generation Process:
* Exploring the steps involved in generating cryptographic keys.
* Key length and algorithm considerations.
* Java libraries and APIs for key generation.
2.2 Key Factory:
- Role of KeyFactory class in Java.
- Generating key pairs using KeyFactory.
- Retrieving key parameters and formats.
- Certificates in Java:
3.1 Managing Certificates:
* Storing and accessing certificates in Java.
* KeyStore and TrustStore concepts.
* Loading and manipulating certificates programmatically.
3.2 Certificate Validation:
- Certificate validation process and its importance.
- Verifying the authenticity and integrity of certificates.
- Revocation checks and certificate pinning.
- Keys, Certificates, and Objects:
* Securing objects using encryption and decryption keys.
* Digital signatures and object integrity.
* Applying keys and certificates to ensure secure communication and data exchange.
- Serialization in Java Security:
* Understanding serialization and its role in Java.
* Serialization vulnerabilities and security risks.
* Techniques for securing serialized data.
* Encryption and signing of serialized objects.
Conclusion:
In this blog post, we have explored the fundamental aspects of Java security,
focusing on keys, certificates, and serialization. We discussed the generation
of keys, the role of key factories, and the importance of certificates in
verifying identities. Additionally, we examined how keys, certificates, and
objects are interconnected in securing data and communication. Finally, we
touched upon the significance of serialization in Java security and the
measures to protect serialized data. By understanding these concepts,
developers can enhance the security of their Java applications and safeguard
sensitive information.
Note: The above article is a general outline and can be expanded further with
detailed explanations, code examples, and references as per your requirements.
使用示例
2. Key Generation and Key Factory
2.1 Key Generation Process
In Java, generating cryptographic keys involves several steps to ensure their
strength and security. Let’s take a look at a basic key generation process:
// Generate a symmetric key
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
keyGenerator.init(256); // Set key length to 256 bits
SecretKey secretKey = keyGenerator.generateKey();
// Generate an asymmetric key pair
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048); // Set key length to 2048 bits
KeyPair keyPair = keyPairGenerator.generateKeyPair();
// Retrieve the generated keys
SecretKey symmetricKey = secretKey;
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
In the code snippet above, we first initialize a KeyGenerator with the desired
symmetric algorithm (in this case, AES) and set the key length to 256 bits. We
then generate a symmetric key using the generateKey() method.
Similarly, for asymmetric key pairs, we use a KeyPairGenerator and initialize
it with the desired algorithm (here, RSA) and key length. The
generateKeyPair() method generates a public-private key pair, which we can
retrieve using the getPublic() and getPrivate() methods.
2.2 Key Factory
The KeyFactory class in Java provides a way to generate keys from their
encoded representations. Here’s an example of using KeyFactory to generate a
public key from its encoded form:
byte[] publicKeyBytes = // Retrieve the encoded public key bytes
X509EncodedKeySpec publicKeySpec = new X509EncodedKeySpec(publicKeyBytes);
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
PublicKey publicKey = keyFactory.generatePublic(publicKeySpec);
In the code snippet above, we assume that the encoded public key bytes are
retrieved from a source (e.g., a file or network). We create an
X509EncodedKeySpec object using the public key bytes, and then use the
generatePublic() method of KeyFactory to generate the PublicKey instance.
最后
从时代发展的角度看,网络安全的知识是学不完的,而且以后要学的会更多,同学们要摆正心态,既然选择入门网络安全,就不能仅仅只是入门程度而已,能力越强机会才越多。
因为入门学习阶段知识点比较杂,所以我讲得比较笼统,大家如果有不懂的地方可以找我咨询,我保证知无不言言无不尽,需要相关资料也可以找我要,我的网盘里一大堆资料都在吃灰呢。
干货主要有:
①1000+CTF历届题库(主流和经典的应该都有了)
②CTF技术文档(最全中文版)
③项目源码(四五十个有趣且经典的练手项目及源码)
④ CTF大赛、web安全、渗透测试方面的视频(适合小白学习)
⑤ 网络安全学习路线图(告别不入流的学习)
⑥ CTF/渗透测试工具镜像文件大全
⑦ 2023密码学/隐身术/PWN技术手册大全
如果你对网络安全入门感兴趣,那么你需要的话可以点击这里👉网络安全重磅福利:入门&进阶全套282G学习资源包免费分享!
扫码领取
## 最后
从时代发展的角度看,网络安全的知识是学不完的,而且以后要学的会更多,同学们要摆正心态,既然选择入门网络安全,就不能仅仅只是入门程度而已,能力越强机会才越多。
因为入门学习阶段知识点比较杂,所以我讲得比较笼统,大家如果有不懂的地方可以找我咨询,我保证知无不言言无不尽,需要相关资料也可以找我要,我的网盘里一大堆资料都在吃灰呢。
干货主要有:
①1000+CTF历届题库(主流和经典的应该都有了)
②CTF技术文档(最全中文版)
③项目源码(四五十个有趣且经典的练手项目及源码)
④ CTF大赛、web安全、渗透测试方面的视频(适合小白学习)
⑤ 网络安全学习路线图(告别不入流的学习)
⑥ CTF/渗透测试工具镜像文件大全
⑦ 2023密码学/隐身术/PWN技术手册大全
如果你对网络安全入门感兴趣,那么你需要的话可以点击这里👉网络安全重磅福利:入门&进阶全套282G学习资源包免费分享!
扫码领取
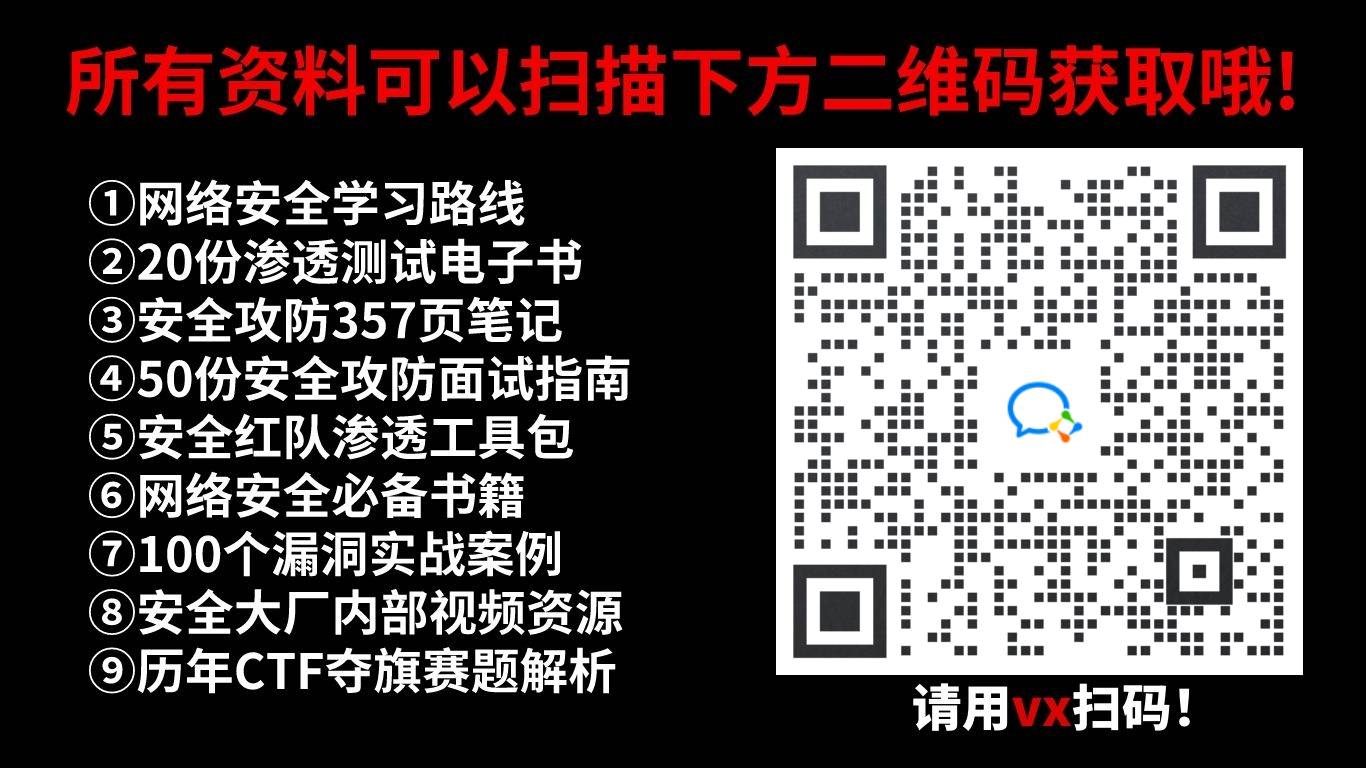