设置下拉框的选中项
<body>
<input type="button" id="btn" value="设置">
<select id="selects">
<option value="1">北京</option>
<option value="2">上海</option>
<option value="3">杭州</option>
<option value="4">广州</option>
</select>
<script>
// 要求点击设置按钮,option随机换
// 获取按钮和下拉框
var btn = document.getElementById('btn');
// 给按钮点击事件
btn.onclick = function () {
// 获取全部下拉框
var selects = document.getElementById('selects');
var options = selects.getElementsByTagName('option');
// 随机值
var randomIndex = parseInt(Math.random() * options.length);
var option = options[randomIndex];
// 选中
option.selected = true;
}
</script>
</body>

失去焦点和得到焦点
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>失去焦点和获得焦点</title>
<style>
.gray {
color: gray;
}
.balck {
color: black;
}
</style>
</head>
<body>
<input type="text" value="请输入关键字" id="textSearch" class="gray">
<input type="button" value="搜索">
<script>
// 当获得焦点时,文本框清空里面文字为黑色
var textSearch = document.getElementById('textSearch');
textSearch.onfocus = function () {
if (this.value == '请输入关键字') {
this.value = '';
this.className = 'black';
}
}
// 失去焦点,条件是value为0或者输入的value值为‘请输入关键字’
textSearch.onblur = function () {
if (this.value.length === 0 || this.value === '请输入关键字') {
this.value = '请输入关键字';
this.className = 'gray';
}
}
</script>
</body>
</html>

全选和反选
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>全选和反选 </title>
<style>
* {
padding: 0;
margin: 0;
}
.wrap {
margin: 250px auto;
width: 400px;
}
table {
width: 100%;
border-spacing: 0;
text-align: center;
}
table,
th,
td {
height: 35px;
border: 1px solid #ccc;
;
}
th {
background-color: #0099cc;
}
tbody tr,
tbody td {
cursor: pointer;
}
tbody tr:hover,
tbody td:hover {
background-color: #999;
}
#btns {
width: 50px;
text-align: center;
margin-top: 15px;
cursor: pointer;
}
</style>
</head>
<body>
<div class="wrap">
<table>
<thead>
<tr>
<th><input type="checkbox" id="checkedAll"></th>
<th>商品</th>
<th>价钱</th>
</tr>
</thead>
<tbody id="tb_check">
<tr>
<td><input type="checkbox"></td>
<td>iphone 8</td>
<td>8000</td>
</tr>
<tr>
<td><input type="checkbox"></td>
<td>ipad Pro</td>
<td>5000</td>
</tr>
<tr>
<td><input type="checkbox"></td>
<td>ipad Air</td>
<td>2000</td>
</tr>
<tr>
<td><input type="checkbox"></td>
<td>Apple Watch</td>
<td>2000</td>
</tr>
</tbody>
</table>
<input type="button" id="btns" value="反选">
</div>
<script>
// 全选 点击父CheckBox那么子CheckBox状态保持一致
//获取父CheckBox和子CheckBox
var checkboxAll = document.getElementById('checkedAll');
var tb_check = document.getElementById('tb_check');
var inputs = tb_check.getElementsByTagName('input');
// 全选和全不选
checkboxAll.onclick = function () {
// 遍历子CheckBox
for (let i = 0; i < inputs.length; i++) {
var input = inputs[i];
// 判断是否CheckBox
if (input.type === 'checkbox') {
// 父CheckBox和子CheckBox状态一样
input.checked = this.checked;
}
}
}
// 点击子CheckBox如果全部选中父CheckBox选中,如果有一个没选中也不选中
for (let i = 0; i < inputs.length; i++) {
var input = inputs[i];
// 判断是否CheckBox
if (input.type != 'checkbox') {
// 结束这次循环
continue;
}
// 给子CheckBox点击事件
input.onclick = function () {
// 调用父CheckBox根据子CheckBox变化状态改变
checkAllCheckbox();
}
}
// 父CheckBox的状态
function checkAllCheckbox() {
// 假设所有子CheckBox都选中
var ischecked = true;
for (let i = 0; i < inputs.length; i++) {
var input = inputs[i];
if (input.type !== 'checkbox') {
continue;
}
if (!input.checked) {
ischecked = false;
}
}
// 父checkbox状态
checkboxAll.checked = ischecked;
}
// 反选
// 获取反选按钮给点击事件
var btns = document.getElementById('btns');
btns.onclick = function () {
// 遍历子CheckBox
for (let i = 0; i < inputs.length; i++) {
var input = inputs[i];
if (input.type !== 'checkbox') {
continue;
}
// 让子CheckBox反选状态
input.checked = !input.checked;
// 设置父CheckBox的状态
checkAllCheckbox();
}
}
</script>
</body>
</html>
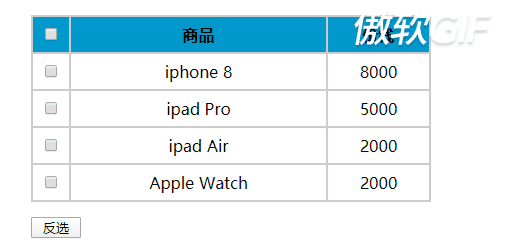
自定义属性
<body>
<div id="box" age="18" personId="1">张三</div>
<script>
var box = document.getElementById('box');
// 自有的属性
console.log(box);
// 获取不到自定义属性
console.log(box.age);
// 获取自定义属性 getAttribute()
console.log(box.getAttribute('age'));
console.log(box.getAttribute('personId'));
// 设置自定义属性
box.setAttribute('sex', 'male');
box.setAttribute('class', 'test');
// 移除属性
box.removeAttribute('age');
box.removeAttribute('personId');
</script>
</body>
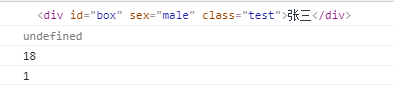
高亮显示input框
<body>
<input type="text"><br>
<input type="text"><br>
<input type="text"><br>
<input type="text"><br>
<input type="text"><br>
<input type="text"><br>
<script>
// 获取全部input框
var inputs = document.getElementsByTagName('input');
// 遍历input框
for (let i = 0; i < inputs.length; i++) {
var input = inputs[i];
if (input.type !== 'text') {
continue;
}
// 获得焦点事件
input.onfocus = function () {
for (var i = 0; i < inputs.length; i++) {
//这里要写因为作用域
var input = inputs[i];
if (input.type !== 'text') {
continue;
}
// 将所有的input框背景色去掉
input.style.backgroundColor = '';
}
// 给正在点击的input高亮显示
this.style.backgroundColor = 'lightgray';
}
}
</script>
</body>
</html>
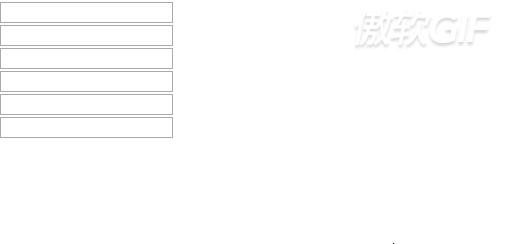
设置大小位置
<style>
.box {
width: 200px;
height: 200px;
background-color: pink;
}
.box1 {
width: 400px;
height: 400px;
background-color: pink;
position: absolute;
left: 250px;
top: 150px;
}
</style>
</head>
<body>
<input type="button" value="设置" id="btn">
<div class="box"></div>
<script>
// 获取box
var box = document.getElementsByClassName('box')[0];
var btn = document.getElementById('btn');
btn.onclick = function () {
// 第一种方法改变ClassName
// box.className = 'box1';
// 第二种用style
box.style.width = '400px';
box.style.height = '400px';
box.style.position = 'absolute';
box.style.left = '150px';
box.style.top = '150px';
}
</script>
</body>
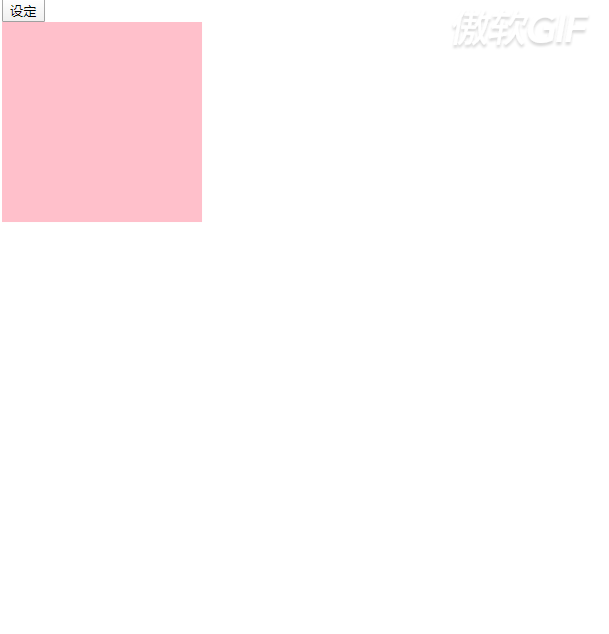
倒计时
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>倒计时</title>
<style>
* {
padding: 0;
margin: 0;
}
.box {
height: 150px;
width: 300px;
margin: 100px auto;
}
.tip {
font-size: 26px;
font-weight: bolder;
}
.date {
margin-top: 25px;
color: chocolate;
font-size: 26px;
}
</style>
</head>
<body>
<div class="box">
<div class="tip">距离光棍节,还有</div>
<div class="date">
<span><span id="day">00</span>天</span>
<span><span id="hour">00</span>时</span>
<span><span id="minute">00</span>分</span>
<span><span id="second">00</span>秒</span>
</div>
</div>
<script>
// 目标时间
var endDate = new Date('2020-11-11 0:0:0');
// 获取span
var spanDay = document.getElementById('day');
var spanHour = document.getElementById('hour');
var spanMinute = document.getElementById('minute');
var spanSecond = document.getElementById('second');
setInterval(countDown, 1000);
countDown();
// 倒计时
function countDown() {
// 计算时间差
// 当前时间
var startDate = new Date();
// 计算两个时间差
var interval = getInterval(startDate, endDate);
// console.log(interval);
setInnerText(spanDay, interval.day);
setInnerText(spanHour, interval.hour);
setInnerText(spanMinute, interval.minute);
setInnerText(spanSecond, interval.second);
}
// 处理innerText和textContent的兼容性问题
// 设置标签之间的内容
function setInnerText(element, content) {
// 判断当前浏览器是否支持 innerText
if (typeof element.innerText === 'string') {
element.innerText = content;
} else {
element.textContent = content;
}
}
// 获取两个日期的时间差
function getInterval(start, end) {
// 两个日期对象,相差的毫秒数
var interval = end - start;
// 求 相差的天数/小时数/分钟数/秒数
var day, hour, minute, second;
// 两个日期对象,相差的秒数
// interval = interval / 1000;
interval /= 1000;
day = Math.round(interval / 60 / 60 / 24);
hour = Math.round(interval / 60 / 60 % 24);
minute = Math.round(interval / 60 % 60);
second = Math.round(interval % 60);
return {
day: day,
hour: hour,
minute: minute,
second: second
}
}
// //格式化日期对象,返回yyyy-MM-dd HH:mm:ss的形式
// function formatDate(date) {
// // 判断参数date是否是日期对象
// // instanceof instance 实例(对象) of 的
// // console.log(date instanceof Date);
// if (!(date instanceof Date)) {
// console.error('date不是日期对象')
// return;
// }
// var year = date.getFullYear(),
// month = date.getMonth() + 1,
// day = date.getDate(),
// hour = date.getHours(),
// minute = date.getMinutes(),
// second = date.getSeconds();
// month = month < 10 ? '0' + month : month;
// day = day < 10 ? '0' + day : day;
// hour = hour < 10 ? '0' + hour : hour;
// minute = minute < 10 ? '0' + minute : minute;
// second = second < 10 ? '0' + second : second;
// return year + '-' + month + '-' + day + ' ' + hour + ':' + minute + ':' + second;
// }
</script>
</body>
</html>
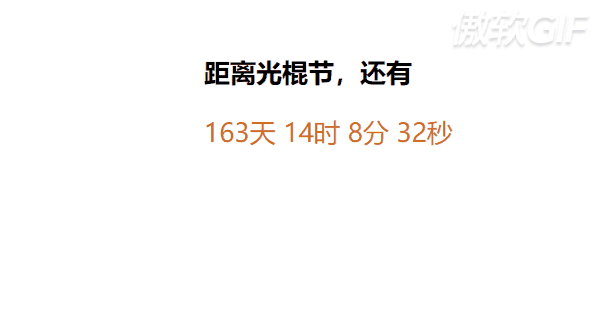
offsetLeft 简单动画
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>简单动画</title>
<style>
#box {
position: relative;
width: 100px;
height: 100px;
background-color: red;
}
</style>
</head>
<body>
<input type="button" value="点击" id="btn">
<div id="box"></div>
<script>
// 获取按钮和box
var btn = document.getElementById('btn');
var box = document.getElementById('box');
btn.onclick = function () {
// offsetLeft 获取盒子当前的位置
// for (let i = 0; i < 100; i++) {
// box.style.left = box.offsetLeft + 5 + 'px';
// }
// 让盒子停在500px的位置
var timerId = setInterval(() => {
var target = 600;
// 步进
var step = 6;
if (box.offsetLeft >= target) {
// 停止定时器
clearInterval(timerId);
// 设置横坐标为500
box.style.left = target + 'px';
console.log(box.style.left);
// 退出函数
return;
}
box.style.left = box.offsetLeft + step + 'px';
console.log(box.style.left);
}, 30);
}
</script>
</body>
</html>
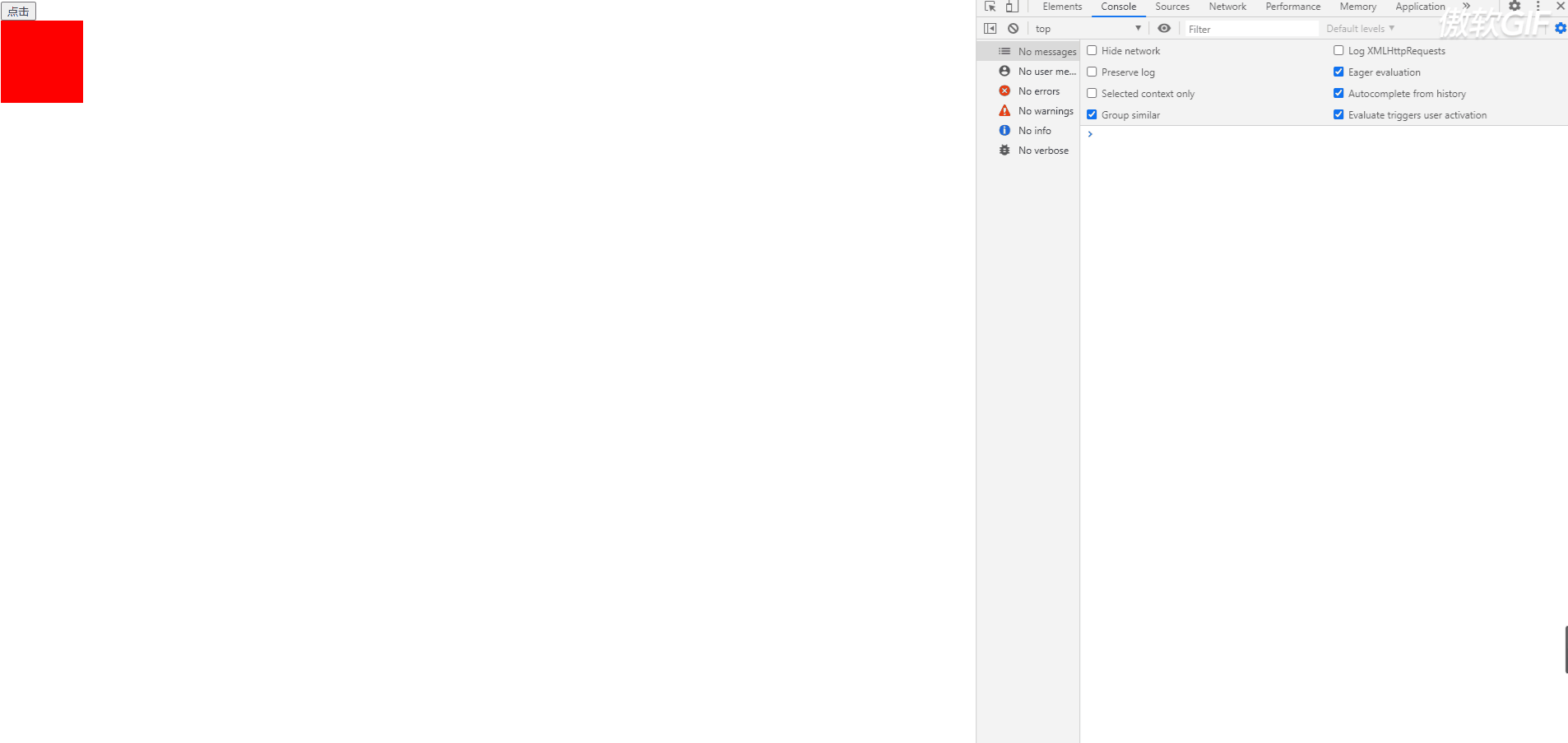
兼容性事件
function my$(id) {
return document.getElementById(id);
}
// 处理浏览器兼容性
// 获取第一个子元素
function getFirstElementChild(element) {
var node, nodes = element.childNodes, i = 0;
while (node = nodes[i++]) {
if (node.nodeType === 1) {
return node;
}
}
return null;
}
// 处理浏览器兼容性
// 获取下一个兄弟元素
function getNextElementSibling(element) {
var el = element;
while (el = el.nextSibling) {
if (el.nodeType === 1) {
return el;
}
}
return null;
}
// 处理innerText和textContent的兼容性问题
// 设置标签之间的内容
function setInnerText(element, content) {
// 判断当前浏览器是否支持 innerText
if (typeof element.innerText === 'string') {
element.innerText = content;
} else {
element.textContent = content;
}
}
// 处理注册事件的兼容性问题
// eventName, 不带on, click mouseover mouseout
function addEventListener(element, eventName, fn) {
// 判断当前浏览器是否支持addEventListener 方法
if (element.addEventListener) {
element.addEventListener(eventName, fn); // 第三个参数 默认是false
} else if (element.attachEvent) {
element.attachEvent('on' + eventName, fn);
} else {
// 相当于 element.onclick = fn;
element['on' + eventName] = fn;
}
}
// 处理移除事件的兼容性处理
function removeEventListener(element, eventName, fn) {
if (element.removeEventListener) {
element.removeEventListener(eventName, fn);
} else if (element.detachEvent) {
element.detachEvent('on' + eventName, fn);
} else {
element['on' + eventName] = null;
}
}
// 获取页面滚动距离的浏览器兼容性问题
// 获取页面滚动出去的距离
function getScroll() {
var scrollLeft = document.body.scrollLeft || document.documentElement.scrollLeft;
var scrollTop = document.body.scrollTop || document.documentElement.scrollTop;
return {
scrollLeft: scrollLeft,
scrollTop: scrollTop
}
}
// 获取鼠标在页面的位置,处理浏览器兼容性
function getPage(e) {
var pageX = e.pageX || e.clientX + getScroll().scrollLeft;
var pageY = e.pageY || e.clientY + getScroll().scrollTop;
return {
pageX: pageX,
pageY: pageY
}
}
动画
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>动画</title>
<style>
#box {
position: relative;
width: 100px;
height: 100px;
background-color: red;
}
</style>
</head>
<body>
<input type="button" value="动画800" id="btn1">
<input type="button" value="动画400" id="btn2">
<div id="box"></div>
<script>
var box = document.getElementById('box');
var btn1 = document.getElementById('btn1');
var btn2 = document.getElementById('btn2');
btn1.onclick = function () {
animate(box, 800);
}
btn2.onclick = function () {
animate(box, 400);
}
var timerId = null;
// 封装动画的函数
function animate(element, target) {
// 通过判断,保证页面上只有一个定时器在执行动画
if (timerId) {
clearInterval(timerId);
timerId = null;
}
timerId = setInterval(function () {
// 步进
var step = 10;
// 盒子当前位置
var current = element.offsetLeft;
console.log(current);
if (current >= target) {
// 让定时器停止
clearInterval(timerId);
// 让盒子回到target的位置
element.style.left = target + 'px';
return;
}
// 移动盒子
current += step;
element.style.left = current + 'px';
}, 30);
}
</script>
</body>
</html>
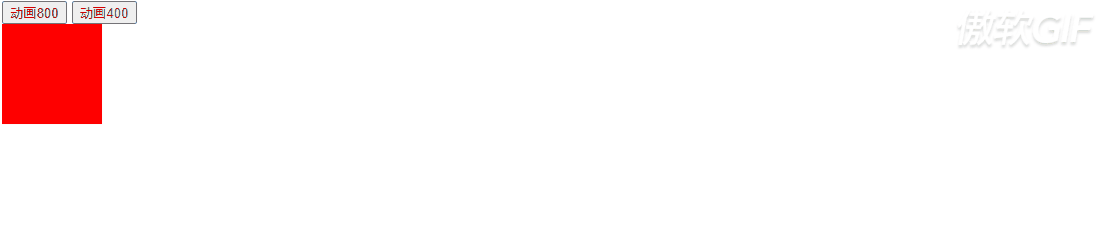
拖拽
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>拖拽</title>
<style>
* {
margin: 0;
padding: 0;
}
.nav {
height: 30px;
background-color: #036663;
border-bottom: 1px solid #369;
line-height: 30px;
padding-left: 30px;
}
.nav a {
color: #fff;
text-align: center;
font-size: 14px;
text-decoration: none;
}
.d-box {
width: 400px;
height: 300px;
border: 5px solid #eee;
box-shadow: 2px 2px 2px 2px #666;
position: absolute;
left: 40%;
top: 40%;
background-color: white;
/* 不让文字被选中 */
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.hd {
width: 100%;
height: 25px;
background-color: #7c9299;
border-bottom: 1px solid #369;
line-height: 25px;
color: white;
cursor: move;
}
#box_close {
float: right;
cursor: pointer;
}
</style>
</head>
<body>
<div class="nav">
<a href="javascript:viod(0)" id="register">注册信息</a>
</div>
<div class="d-box" id="d-box">
<div class="hd" id="drop">注册信息(可以拖拽)
<span id="box_close">【关闭】</span>
</div>
<div class="bd"></div>
</div>
<script>
var box = document.getElementById('d-box');
var drop = document.getElementById('drop');
drop.onmousedown = function (e) {
// 兼容性处理
e = e || window.event;
// 当鼠标按下的时候,求鼠标在盒子中的位置
// 鼠标在盒子中的位置 = 鼠标在页面上的位置 - 盒子的位置
var x = e.pageX - box.offsetLeft;
var y = e.pageY - box.offsetTop;
// 鼠标在文档中移动
document.onmousemove = function (e) {
e = e || window.event;
// 当鼠标在页面上移动的时候,求盒子的坐标
// 盒子的坐标 = 鼠标当前在页面中的位置 - 鼠标在盒子中的位置
var boxX = e.pageX - x;
var boxY = e.pageY - y;
box.style.left = boxX + 'px';
box.style.top = boxY + 'px';
}
}
// 当鼠标弹起的时候,移除鼠标移动事件
document.onmouseup = function () {
document.onmousemove = null;
}
// 点击关闭按钮,隐藏盒子
var box_close = document.getElementById('box_close');
box_close.onclick = function () {
box.style.display = 'none';
}
</script>
</body>
</html>
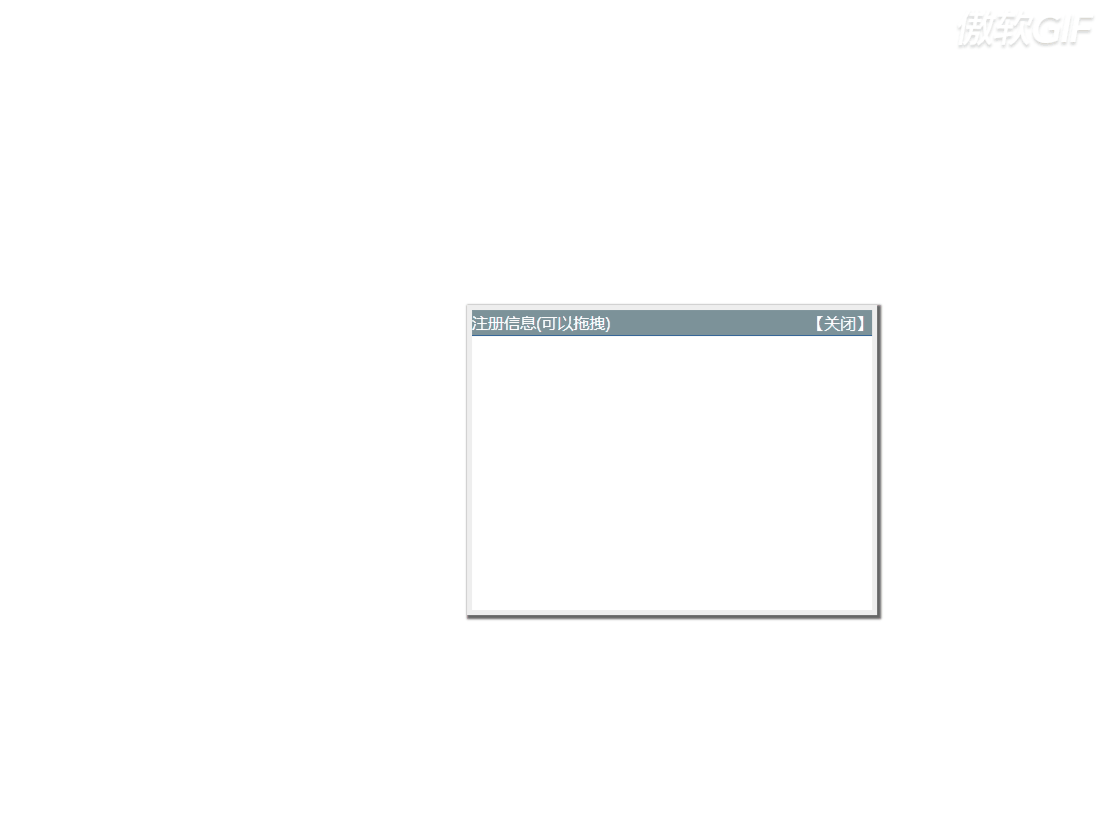
弹出层
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>弹出层</title>
<style>
.login-header {
width: 100%;
text-align: center;
height: 30px;
font-size: 24px;
line-height: 30px;
}
ul,
li,
ol,
dl,
dt,
dd,
div,
p,
span,
h1,
h2,
h3,
h4,
h5,
h6,
a {
padding: 0px;
margin: 0px;
}
.login {
width: 512px;
height: 280px;
position: absolute;
border: #ebebeb solid 1px;
left: 50%;
right: 50%;
background: #ffffff;
box-shadow: 0px 0px 20px #ddd;
z-index: 9999;
margin-left: -256px;
margin-top: 140px;
display: none;
}
.login-title {
width: 100%;
margin: 10px 0px 0px 0px;
text-align: center;
line-height: 40px;
height: 40px;
font-size: 18px;
position: relative;
cursor: move;
-moz-user-select: none;
/*火狐*/
-webkit-user-select: none;
/*webkit浏览器*/
-ms-user-select: none;
/*IE10*/
-khtml-user-select: none;
/*早期浏览器*/
user-select: none;
}
.login-input-content {
margin-top: 20px;
}
.login-button {
width: 50%;
margin: 30px auto 0px auto;
line-height: 40px;
font-size: 14px;
border: #ebebeb 1px solid;
text-align: center;
}
.login-bg {
width: 100%;
height: 100%;
position: fixed;
top: 0px;
left: 0px;
background: #000000;
filter: alpha(opacity=30);
-moz-opacity: 0.3;
-khtml-opacity: 0.3;
opacity: 0.3;
display: none;
}
a {
text-decoration: none;
color: #000000;
}
.login-button a {
display: block;
}
.login-input input.list-input {
float: left;
line-height: 35px;
height: 35px;
width: 350px;
border: #ebebeb 1px solid;
text-indent: 5px;
}
.login-input {
overflow: hidden;
margin: 0px 0px 20px 0px;
}
.login-input label {
float: left;
width: 90px;
padding-right: 10px;
text-align: right;
line-height: 35px;
height: 35px;
font-size: 14px;
}
.login-title span {
position: absolute;
font-size: 12px;
right: -20px;
top: -30px;
background: #ffffff;
border: #ebebeb solid 1px;
width: 40px;
height: 40px;
border-radius: 20px;
}
</style>
</head>
<body>
<div class="login-header"><a id="link" href="javascript:void(0);">点击,弹出登录框</a></div>
<div id="login" class="login">
<div id="title" class="login-title">登录会员
<span><a id="closeBtn" href="javascript:void(0);" class="close-login">关闭</a></span>
</div>
<div class="login-input-content">
<div class="login-input">
<label>用户名:</label>
<input type="text" placeholder="请输入用户名" name="info[username]" id="username" class="list-input">
</div>
<div class="login-input">
<label>登录密码:</label>
<input type="password" placeholder="请输入登录密码" name="info[password]" id="password" class="list-input">
</div>
</div>
<div id="loginBtn" class="login-button"><a href="javascript:void(0);" id="login-button-submit">登录会员</a></div>
</div>
<!-- 遮盖层 -->
<div id="bg" class="login-bg"></div>
<script>
// 显示登录框和遮盖层
var login = document.getElementById('login');
var bg = document.getElementById('bg');
// 点击按钮,弹出登录框和遮盖层
var link = document.getElementById('link');
link.onclick = function () {
login.style.display = 'block';
bg.style.display = 'block';
return false;
}
// 点击关闭按钮,隐藏 登录框和遮盖层
var closeBtn = document.getElementById('closeBtn');
closeBtn.onclick = function () {
// 隐藏 登录框和遮盖层
login.style.display = 'none';
bg.style.display = 'none';
}
// 拖拽
var title = document.getElementById('title');
title.onmousedown = function (e) {
// 鼠标按下,求鼠标在盒子中的位置
var x = e.pageX - login.offsetLeft;
var y = e.pageY - login.offsetTop;
document.onmousemove = function (e) {
var loginX = e.pageX - x;
var loginY = e.pageY - y;
login.style.left = loginX + 256 + 'px';
login.style.top = loginY - 140 + 'px';
}
}
document.onmouseup = function () {
// 移除鼠标移动的事件
document.onmousemove = null;
}
</script>
</body>
</html>
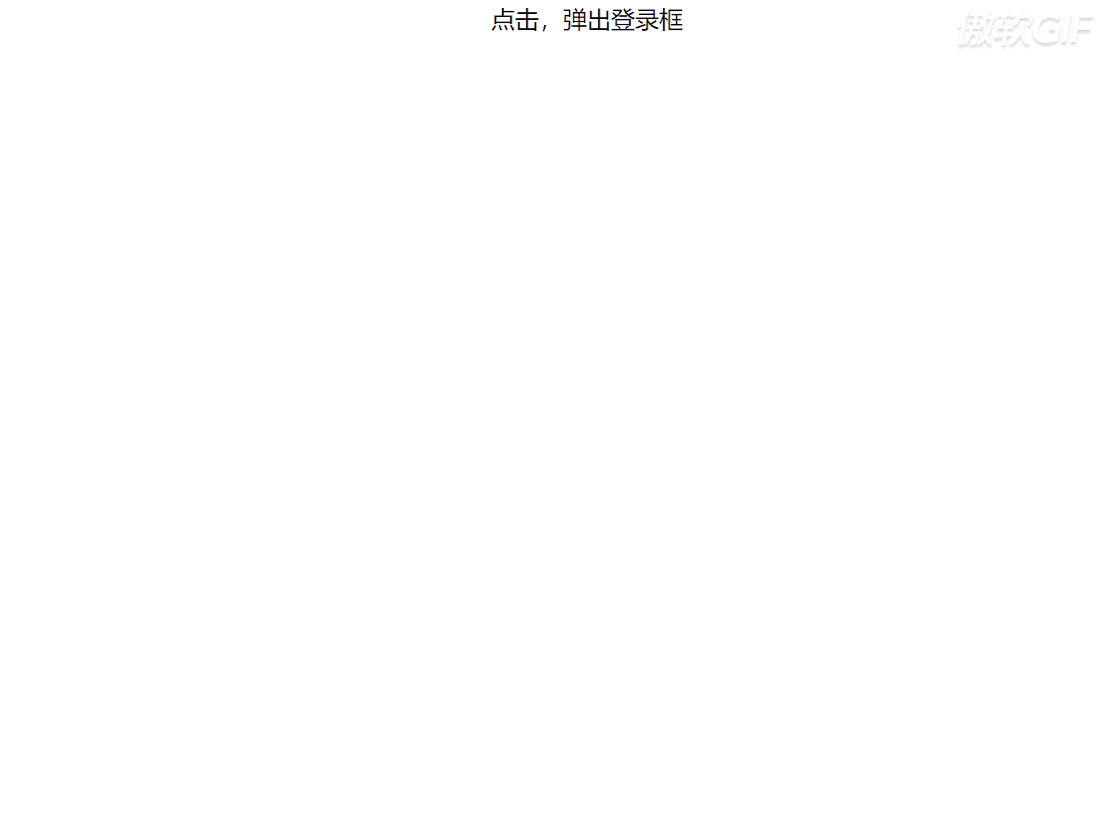
放大镜
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>放大镜</title>
<style>
* {
margin: 0;
padding: 0;
}
.box {
width: 350px;
height: 350px;
margin: 100px;
border: 1px solid #ccc;
position: relative;
}
.big {
width: 400px;
height: 400px;
position: absolute;
left: 360px;
top: 0;
border: 1px solid #ccc;
overflow: hidden;
display: none;
}
.big img {
position: absolute;
}
.mask {
width: 175px;
height: 175px;
background: rgba(255, 255, 0, 0.4);
position: absolute;
top: 0;
left: 0;
cursor: move;
display: none;
}
.small {
position: relative;
}
</style>
</head>
<body>
<div class="box" id="box">
<div class="small">
<img src="small.jpg" width="350" alt="">
<div class="mask"></div>
</div>
<div class="big">
<img src="big.jpg" width="800" alt="">
</div>
</div>
<script>
var box = document.getElementById('box');
var smallBox = box.children[0];
var bigBox = box.children[1];
var smallImage = smallBox.children[0];
var mask = smallBox.children[1];
var bigImage = bigBox.children[0];
// 鼠标经过的时候,显示mask和bigBox,当鼠标离开box的时候隐藏mask和bigBox
// mouseenter mouseleave 不会触发事件冒泡
// mouseover mouseout 会触发事件冒泡
box.onmouseenter = function () {
// 显示mask和bigBox
mask.style.display = 'block';
bigBox.style.display = 'block';
}
box.onmouseleave = function () {
mask.style.display = 'none';
bigBox.style.display = 'none';
}
// 当鼠标在盒子中移动的时候,让mask和鼠标一起移动
box.onmousemove = function (e) {
e = e || window.event;
// 获取鼠标在盒子中的位置就是mask的坐标
var maskX = e.pageX - box.offsetLeft;
var maskY = e.pageY - box.offsetTop;
// 让鼠标出现在中心点
maskX = maskX - mask.offsetWidth / 2;
maskY = maskY - mask.offsetHeight / 2;
// 把mask限制到box中
maskX = maskX < 0 ? 0 : maskX;
maskY = maskY < 0 ? 0 : maskY;
maskX = maskX > box.offsetWidth - mask.offsetWidth ? box.offsetWidth - mask.offsetWidth : maskX;
maskY = maskY > box.offsetHeight - mask.offsetHeight ? box.offsetHeight - mask.offsetHeight : maskY;
mask.style.left = maskX + 'px';
mask.style.top = maskY + 'px';
// 当mask移动的时候,让大图移动
// mask移动的距离/mask最大能够移动的距离=大图片移动的距离/大图片最大能够移动的距离
// mask最大能够移动的距离
var maskMax = box.offsetWidth - mask.offsetWidth;
// 大图片最大能够移动的距离
var bigImageMax = bigImage.offsetWidth - bigBox.offsetWidth;
var bigImageX = maskX * bigImageMax / maskMax;
var bigImageY = maskY * bigImageMax / maskMax;
bigImage.style.left = -bigImageX + 'px';
bigImage.style.top = -bigImageY + 'px';
}
</script>
</body>
</html>
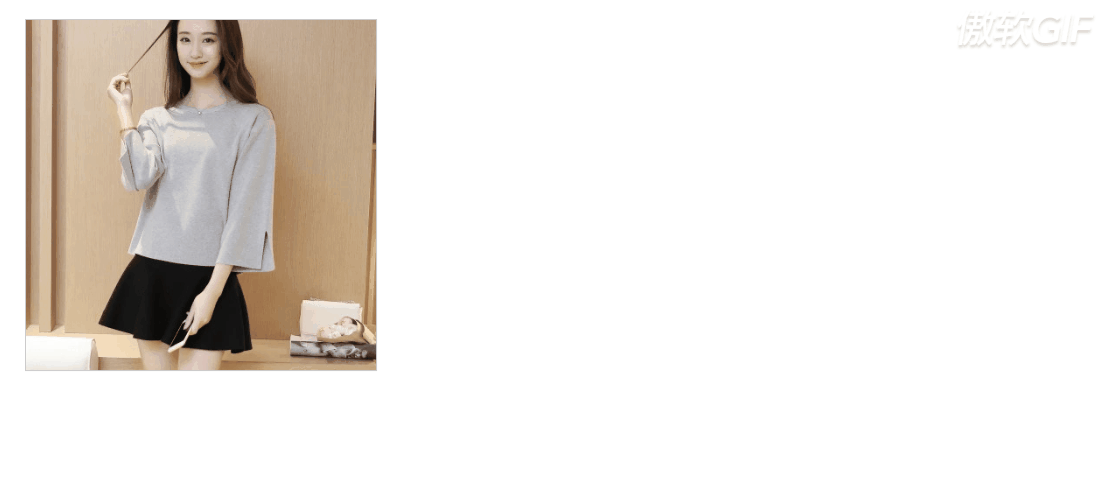
回到顶部
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>回到顶部</title>
<style>
.header {
position: fixed;
z-index: 20;
left: 0;
top: 0;
height: 90px;
width: 100%;
background-color: #fff;
transition: height 1.3s;
border: 1px solid #ccc;
}
.header.fixed {
height: 50px;
border: 1px solid #ccc;
box-shadow: 0 0 1px 0 rgba(0, 0, 0, .3), 0 0 6px 2px rgba(0, 0, 0, .15);
}
.box {
height: 1700px;
}
.top {
position: fixed;
right: 100px;
bottom: 100px;
height: 50px;
width: 50px;
background-color: gray;
opacity: 0.7;
cursor: pointer;
display: none;
}
</style>
</head>
<body>
<header class="header" id="header">我是头部</header>
<div class="box"></div>
<div class="top" id="top"></div>
<script>
var header = document.getElementById('header');
var tops = document.getElementById('top');
// 当滚动条滚动时判断
window.onscroll = function () {
var scrollTop = getScroll().scrollTop;
// 滚动距离大于10的时候
if (scrollTop > 10) {
header.className = 'header fixed';
tops.style.display = 'block';
} else {
header.className = 'header';
tops.style.display = 'none';
}
}
// 获取页面滚动距离的浏览器兼容性问题
// 获取页面滚动出去的距离
function getScroll() {
var scrollLeft = document.body.scrollLeft || document.documentElement.scrollLeft;
var scrollTop = document.body.scrollTop || document.documentElement.scrollTop;
return {
scrollLeft: scrollLeft,
scrollTop: scrollTop
}
}
// 当点击回到顶部按钮的时候,动画的方式,回答最上面,让滚动距离为0
var timerId = null;
top.onclick = function () {
if (timerId) {
clearInterval(timerId);
timerId = null;
}
timerId = setInterval(function () {
// 步进
var step = 5;
// 最终目标
var target = 0;
// 获取当前位置
var current = getScroll().scrollTop;
if (current > target) {
step = -Math.abs(step);
}
// 判断当前是否到达目标位置
if (Math.abs(current - target) <= Math.abs(step)) {
clearInterval(timerId);
document.body.scrollTop = target;
document.documentElement.scrollTop = target;
return;
}
current += step;
document.body.scrollTop = current;
document.documentElement.scrollTop = current;
}, 5);
}
</script>
</body>
</html>
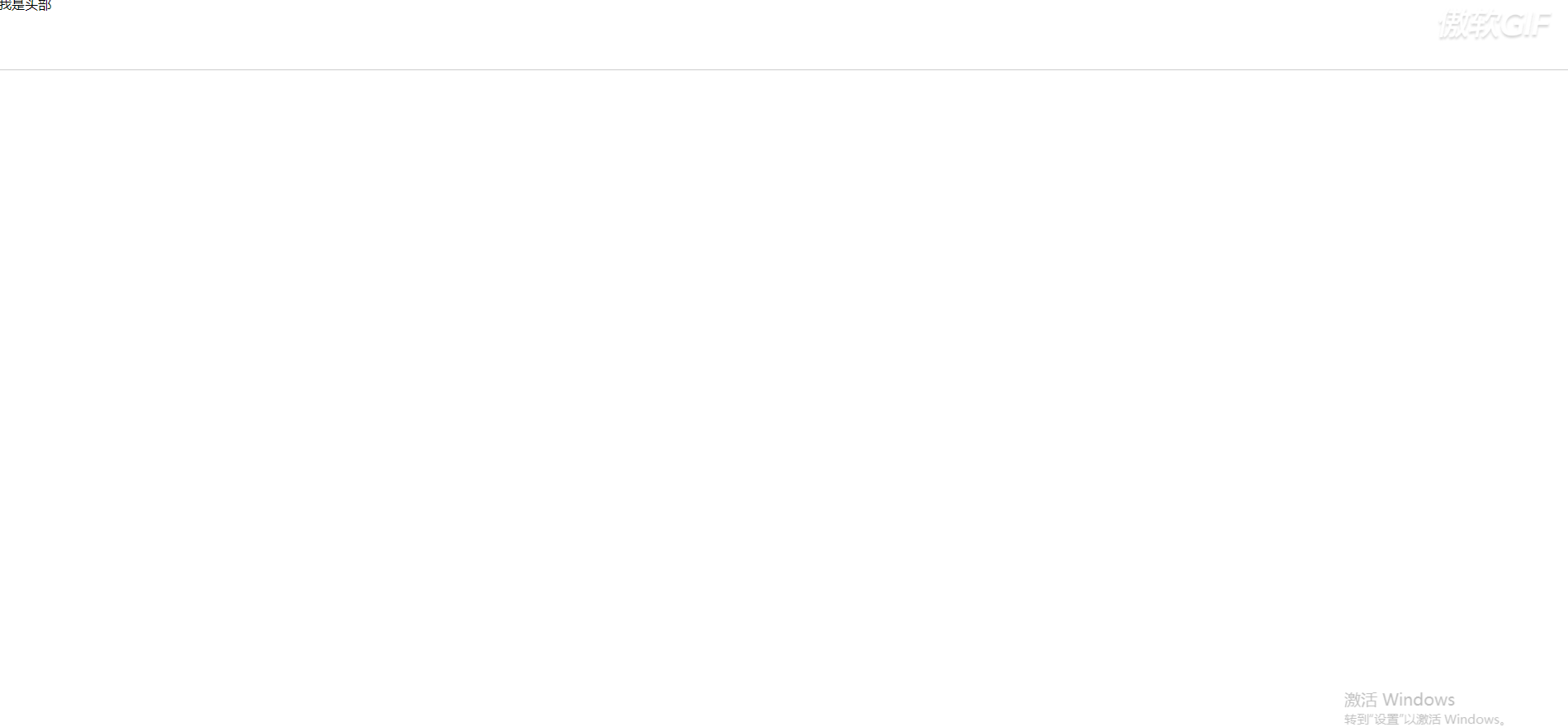
轮播图
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>轮播图</title>
<style>
* {
padding: 0;
margin: 0;
}
.all {
width: 500px;
height: 200px;
padding: 7px;
border: 1px solid #ccc;
margin: 100px auto;
position: relative;
}
.screen {
width: 500px;
height: 200px;
position: relative;
overflow: hidden;
}
.screen li {
width: 500px;
height: 200px;
overflow: hidden;
float: left;
}
.screen ul {
position: absolute;
left: 0;
top: 0;
width: 3000px;
}
.all ol {
position: absolute;
right: 10px;
bottom: 10px;
line-height: 20px;
text-align: center;
}
.all ol li {
float: left;
width: 20px;
height: 20px;
background-color: #fff;
border: 1px solid #ccc;
margin-left: 10px;
cursor: pointer;
}
.all ol li.current {
background-color: yellow;
}
#arr {
display: none;
z-index: 1000;
}
#arr span {
width: 40px;
height: 40px;
position: absolute;
left: 5px;
top: 50%;
margin-top: -20px;
background-color: #000;
cursor: pointer;
line-height: 40px;
text-align: center;
font-weight: bold;
font-family: '黑体';
font-size: 30px;
color: #fff;
opacity: 0.3;
border: 1px solid #fff;
}
#arr #right {
right: 5px;
left: auto;
}
</style>
</head>
<body>
<div class="all" id="box">
<div class="screen">
<ul>
<li><img src="wf1.jpg" width="500" height="200"> </li>
<li><img src="wf2.jpg" width="500" height="200"> </li>
<li><img src="wf3.jpg" width="500" height="200"> </li>
<li><img src="wf4.jpg" width="500" height="200"> </li>
<li><img src="wf5.jpg" width="500" height="200"> </li>
</ul>
<ol></ol>
</div>
<div id="arr"><span id="left"><</span><span id="right">></span></div>
<script>
// 获取元素
var timerId = null;
var box = document.getElementById('box');
var screen = box.children[0];
var ul = screen.children[0];
var ol = screen.children[1];
// 箭头 arr
var arr = document.getElementById('arr');
var arrLeft = document.getElementById('left');
var arrRight = document.getElementById('right');
// 图片的宽度
var imgWidth = screen.offsetWidth;
// 动态生成序号
// 页面上总共有多少张图片
var count = ul.children.length;
for (var i = 0; i < count; i++) {
var li = document.createElement('li');
ol.appendChild(li);
setInnerText(li, i + 1);
// 点击序列号 动画的方式切换图片
li.onclick = liClick;
// 让当前里记录他的索引
// 设置标签自定义属性
li.setAttribute('index', i);
function liClick() {
// 取消其它li得到高亮显示,让当前的li高亮显示
for (let i = 0; i < ol.children.length; i++) {
const li = ol.children[i];
li.className = '';
}
// 让当前li高亮显示
this.className = 'current';
// 点击序列号,动画的方式切换到当前点击的图片位置
// 获取自定义属性
var liIndex = parseInt(this.getAttribute('index'));
animate(ul, -liIndex * imgWidth);
// 全局变量index和li中的index保持一致
index = liIndex;
}
// 让序列号1高亮显示
ol.children[0].className = 'current';
// 鼠标放到盒子上显示箭头
box.onmouseenter = function () {
arr.style.display = 'block';
// 清除定时器
clearInterval(timerId);
}
box.onmouseleave = function () {
arr.style.display = 'none';
// 重新开启定时器
timerId = setInterval(function () {
arrRight.click();
}, 2000);
}
// 实现上一张和下一张的功能
// 下一张
var index = 0; //第一张图片的索引
arrRight.onclick = function () {
// 判断是否是克隆的第一张图片,如果是克隆的第一张图片,此时修改ul的坐标,显示真正的第一张图片
if (index === count) {
ul.style.left = '0px';
index = 0;
}
// 总共有五张图片,但是还有一张克隆的图片,克隆的图片的索引是5
index++;
if (index < count) {
// 获取图片对应的序列号,让序列号高亮显示
ol.children[index].click();
} else {
// 如果是最后一张图片,以动画的方式,移动到克隆的第一张图片
animate(ul, -index * imgWidth);
// 取消所有序号的高亮显示,让第一序号高亮显示
for (let i = 0; i < ol.children.length; i++) {
const li = ol.children[i];
li.className = '';
}
ol.children[0].className = 'current';
}
}
// 上一张
arrLeft.onclick = function () {
// 如果当前是第一张图片,此时要偷偷的切换到最后一张图片的位置(克隆的第一张图片)
if (index == 0) {
index = count;
ul.style.left = -index * imgWidth + 'px';
}
index--;
ol.children[index].click();
}
// 无缝滚动
// 获取ul中的第一个li
var firstLi = ul.children[0];
// 克隆li cloneNode() 复制节点
// 参数 true 复制节点中的内容
// false 只复制当前节点,不复制里面的内容
var cloneLi = firstLi.cloneNode(true);
ul.appendChild(cloneLi);
}
// 自动切换图片
var timerId = setInterval(function () {
// 切换到下一张图片
arrRight.click();
}, 2000);
// 封装动画的函数
function animate(element, target) {
// 通过判断,保证页面上只有一个定时器在执行动画
if (element.timerId) {
clearInterval(element.timerId);
element.timerId = null;
}
element.timerId = setInterval(function () {
// 步进 每次移动的距离
var step = 10;
// 盒子当前的位置
var current = element.offsetLeft;
// 当从400 到 800 执行动画
// 当从800 到 400 不执行动画
// 判断如果当前位置 > 目标位置 此时的step 要小于0
if (current > target) {
step = - Math.abs(step);
}
// Math.abs(current - target) <= Math.abs(step)
if (Math.abs(current - target) <= Math.abs(step)) {
// 让定时器停止
clearInterval(element.timerId);
// 让盒子到target的位置
element.style.left = target + 'px';
return;
}
// 移动盒子
current += step;
element.style.left = current + 'px';
}, 5);
}
// 处理innerText和textContent的兼容性问题
// 设置标签之间的内容
function setInnerText(element, content) {
// 判断当前浏览器是否支持 innerText
if (typeof element.innerText === "string") {
element.innerText = content;
} else {
element.textContent = content;
}
}
</script>
</div>
</body>
</html>
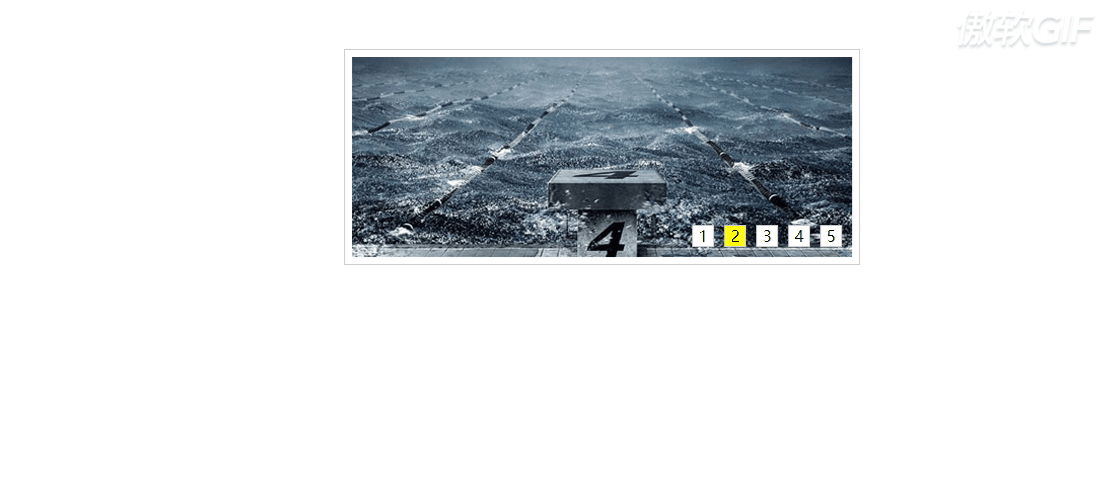