最近在学习窗体,也给大家分享下自己简单的思路
首先需要使用的软件是eclipse(mars)
因为学习嘛 需要循序渐进,有生成工具的帮助自然是更好,
不过也需要自己能看懂哦,建议生成时多看看代码。
其次需要一个驱动包,自行下载
--------------------------------------------------
大概的分层是这样的
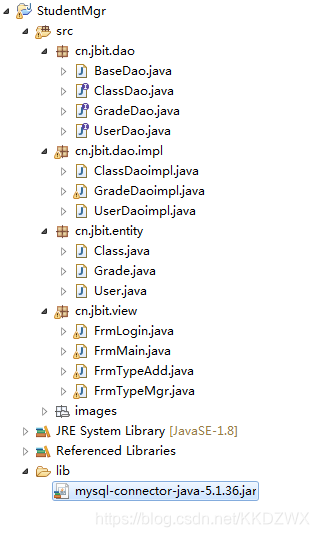
数据库以及驱动和图片下载:
下载地址
提取码:wuj5
代码如下:
BaseDao:
package cn.jbit.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class BaseDao {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
private static final String driver = "com.mysql.jdbc.Driver";
private static final String url = "jdbc:mysql://localhost:3306/db_studentinfo";
private static final String user = "root";
private static final String password = "zwx123z";
static {
try {
Class.forName(driver);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public Connection getConnection() throws SQLException {
return DriverManager.getConnection(url, user, password);
}
public ResultSet executeQuery(String sql, Object... obje) throws Exception {
conn = getConnection();
ps = conn.prepareStatement(sql);
for (int i = 0; i < obje.length; i++) {
ps.setObject(i + 1, obje[i]);
}
rs = ps.executeQuery();
return rs;
}
public void closeAll(Connection conn, Statement st, ResultSet rs) {
try {
if (rs != null) {
rs.close();
}
if (st != null) {
st.close();
}
if (conn != null) {
conn.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
public int myExecuteUpdate(String sql, Object... params) {
int result = 0;
try {
conn = getConnection();
ps = conn.prepareStatement(sql);
if (params != null && params.length > 0) {
for (int i = 0; i < params.length; i++) {
ps.setObject(i + 1, params[i]);
}
}
result = ps.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
closeAll(conn, ps, null);
}
return result;
}
}
User实体类setget方法:
package cn.jbit.entity;
public class User {
private int id;
private String userName;
private String userPwd;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getUserPwd() {
return userPwd;
}
public void setUserPwd(String userPwd) {
this.userPwd = userPwd;
}
}
Grade实体类setget方法:
package cn.jbit.entity;
public class Grade {
private int id;
private String gradeName;
private String gradeRemarks;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getGradeName() {
return gradeName;
}
public void setGradeName(String gradeName) {
this.gradeName = gradeName;
}
public String getGradeRemarks() {
return gradeRemarks;
}
public void setGradeRemarks(String gradeRemarks) {
this.gradeRemarks = gradeRemarks;
}
}
Class实体类setget方法:
package cn.jbit.entity;
public class Class {
private int id;
private String className;
private int gradeId;
private String classRemarks;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getClassName() {
return className;
}
public void setClassName(String className) {
this.className = className;
}
public int getGradeId() {
return gradeId;
}
public void setGradeId(int gradeId) {
this.gradeId = gradeId;
}
public String getClassRemarks() {
return classRemarks;
}
public void setClassRemarks(String classRemarks) {
this.classRemarks = classRemarks;
}
}
User接口:
package cn.jbit.dao;
import cn.jbit.entity.User;
public interface UserDao {
public User Login(String userName, String userPwd) throws Exception;
}
Class接口:
package cn.jbit.dao;
import java.util.List;
import cn.jbit.entity.Class;
public interface ClassDao {
List<Class> getgradeId() throws Exception;
}
Grade接口:
package cn.jbit.dao;
import java.util.List;
import cn.jbit.entity.Grade;
public interface GradeDao {
int add(Grade grade);
List<Grade> PD(String gradeName) throws Exception;
List<Grade> getAll() throws Exception;
List<Grade> getgradeName(String gradeName) throws Exception;
int del(Grade grade);
int update(String gradeName, String gradeBZ, int id) throws Exception;
}
接下来是实现类
实现的效果虽然有,但是也有缺陷,但是也是我按自己的思路来写的
就是有一点 我这些代码里没有关闭资源 大家可以参考后修改
--------------------------------------------------
ClassDaoimpl 接口实现类:
package cn.jbit.dao.impl;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import cn.jbit.dao.BaseDao;
import cn.jbit.dao.ClassDao;
import cn.jbit.entity.Class;
public class ClassDaoimpl extends BaseDao implements ClassDao {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
public List<Class> getgradeId() throws Exception {
String sql = "select gradeid from Class";
rs = this.executeQuery(sql);
List<Class> classes = new ArrayList<Class>();
if (rs != null) {
while (rs.next()) {
Class class1 = new Class();
class1.setGradeId(rs.getInt("gradeid"));
classes.add(class1);
}
}
return classes;
}
}
GradeDaoimpl 接口实现类:
package cn.jbit.dao.impl;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JOptionPane;
import cn.jbit.dao.BaseDao;
import cn.jbit.dao.GradeDao;
import cn.jbit.entity.Grade;
public class GradeDaoimpl extends BaseDao implements GradeDao {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
Grade grade;
public int add(Grade grade) {
String sql = "insert into grade values(null,?,?)";
return myExecuteUpdate(sql, grade.getGradeName(), grade.getGradeRemarks());
}
public List<Grade> PD(String gradeName) throws Exception {
String sql = "select*from grade where gradeName=?";
List<Grade> gradelist = new ArrayList<Grade>();
rs = this.executeQuery(sql, gradeName);
if (rs != null) {
while (rs.next()) {
Grade grade = new Grade();
grade.setId(rs.getInt("id"));
grade.setGradeName(rs.getString("gradeName"));
grade.setGradeRemarks(rs.getString("gradeRemarks"));
gradelist.add(grade);
}
}
return gradelist;
}
public List<Grade> getAll() throws Exception {
String sql = "select*from grade";
List<Grade> gradelist = new ArrayList<Grade>();
rs = this.executeQuery(sql);
if (rs != null) {
while (rs.next()) {
Grade grade = new Grade();
grade.setId(rs.getInt("id"));
grade.setGradeName(rs.getString("gradeName"));
grade.setGradeRemarks(rs.getString("gradeRemarks"));
gradelist.add(grade);
}
}
return gradelist;
}
public List<Grade> getgradeName(String gradeName) throws Exception {
String sql = "select*from grade where gradeName like'%" + gradeName + "%'";
List<Grade> gradelist = new ArrayList<Grade>();
rs = this.executeQuery(sql);
if (rs != null) {
while (rs.next()) {
Grade grade = new Grade();
grade.setId(rs.getInt("id"));
grade.setGradeName(rs.getString("gradeName"));
grade.setGradeRemarks(rs.getString("gradeRemarks"));
gradelist.add(grade);
}
}
return gradelist;
}
public int del(Grade grade) {
String sql = "delete from grade where id=?";
return myExecuteUpdate(sql, grade.getId());
}
public int update(String gradeName, String gradeBZ, int id) throws Exception {
String sql = "update grade set gradeName='" + gradeName + "',gradeRemarks='" + gradeBZ + "' where id=" + id;
return myExecuteUpdate(sql);
}
}
UserDaoimpl 接口实现类:
package cn.jbit.dao.impl;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import cn.jbit.dao.BaseDao;
import cn.jbit.dao.UserDao;
import cn.jbit.entity.User;
public class UserDaoimpl extends BaseDao implements UserDao {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
public User Login(String userName, String userPwd) throws Exception {
String sql = "select * from user where userName=? and password=?";
ResultSet rs = this.executeQuery(sql, userName, userPwd);
User user = null;
if (rs != null) {
if (rs.next()) {
user = new User();
user.setId(rs.getInt("userid"));
user.setUserName(rs.getString("userName"));
user.setUserPwd(rs.getString("password"));
}
}
return user;
}
}
最后是窗体咯
FrmLogin 这是登录的页面哦
package cn.jbit.view;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import cn.jbit.dao.UserDao;
import cn.jbit.dao.impl.UserDaoimpl;
import cn.jbit.entity.User;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import java.awt.Font;
import javax.swing.ImageIcon;
import java.awt.Color;
import javax.swing.JTextField;
import javax.swing.JPasswordField;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class FrmLogin extends JFrame {
private JPanel contentPane;
private JTextField Login_UserName;
private JPasswordField Login_pwd;
public static User user;
UserDao userDao = new UserDaoimpl();
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
FrmLogin frame = new FrmLogin();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public FrmLogin() {
setTitle("\u5B66\u751F\u4FE1\u606F\u7BA1\u7406\u7CFB\u7EDF\u767B\u5F55\u9875\u9762");
setResizable(false);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(450, 269);
this.setLocationRelativeTo(null);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JLabel txtBT = new JLabel("\u5B66\u751F\u4FE1\u606F\u7BA1\u7406\u7CFB\u7EDF");
txtBT.setForeground(new Color(204, 0, 0));
txtBT.setBackground(new Color(46, 139, 87));
txtBT.setIcon(new ImageIcon(FrmLogin.class.getResource("/images/log.png")));
txtBT.setFont(new Font("华文行楷", Font.BOLD, 30));
txtBT.setBounds(49, 23, 324, 57);
contentPane.add(txtBT);
JLabel txtUserName = new JLabel("\u7528\u6237\u540D:");
txtUserName.setFont(new Font("华文楷体", Font.PLAIN, 19));
txtUserName.setIcon(new ImageIcon(FrmLogin.class.getResource("/images/userName.png")));
txtUserName.setBounds(82, 101, 130, 30);
contentPane.add(txtUserName);
JLabel txtPwd = new JLabel("\u5BC6\u7801:");
txtPwd.setIcon(new ImageIcon(FrmLogin.class.getResource("/images/password.png")));
txtPwd.setFont(new Font("华文楷体", Font.PLAIN, 19));
txtPwd.setBounds(92, 144, 130, 30);
contentPane.add(txtPwd);
Login_UserName = new JTextField();
Login_UserName.setBounds(185, 108, 121, 21);
contentPane.add(Login_UserName);
Login_UserName.setColumns(10);
Login_pwd = new JPasswordField();
Login_pwd.setBounds(185, 151, 121, 21);
contentPane.add(Login_pwd);
Login_UserName.setText("cmu");
Login_pwd.setText("123");
JButton button_Login = new JButton("\u767B\u5F55");
button_Login.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String userName = Login_UserName.getText().trim();
String pwdUser = new String(Login_pwd.getPassword());
if (userName == null || userName.equals("")) {
JOptionPane.showMessageDialog(null, "用户名不能为空!");
return;
}
if (pwdUser == null || pwdUser.equals("")) {
JOptionPane.showMessageDialog(null, "密码不能为空!");
return;
}
user = new User();
try {
user = userDao.Login(userName, pwdUser);
if (user != null) {
FrmMain frmMain = new FrmMain();
frmMain.setVisible(true);
dispose();
} else {
JOptionPane.showMessageDialog(null, "账号或密码错误,登录失败!");
return;
}
} catch (Exception e1) {
e1.printStackTrace();
}
}
});
button_Login.setIcon(new ImageIcon(FrmLogin.class.getResource("/images/login.png")));
button_Login.setBounds(76, 197, 95, 25);
contentPane.add(button_Login);
JButton button_Reset = new JButton("\u91CD\u7F6E");
button_Reset.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
Login_UserName.setText("");
Login_pwd.setText("");
}
});
button_Reset.setIcon(new ImageIcon(FrmLogin.class.getResource("/images/reset.png")));
button_Reset.setBounds(278, 197, 95, 25);
contentPane.add(button_Reset);
}
}
FrmMain 这是登入后的页面哦
package cn.jbit.view;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JInternalFrame;
import javax.swing.JPanel;
import javax.swing.JMenuBar;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.ImageIcon;
import javax.swing.JToolBar;
import javax.swing.border.EmptyBorder;
import javax.swing.JButton;
import javax.swing.JDesktopPane;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class FrmMain extends JFrame {
private JPanel contentPane;
JDesktopPane desktopPane;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
FrmMain frame = new FrmMain();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
private void centerFrm(JInternalFrame from) {
from.setVisible(true);
desktopPane.add(from);
int left = (desktopPane.getWidth() - from.getWidth()) / 2;
int top = (desktopPane.getHeight() - from.getHeight()) / 2;
from.setLocation(left, top);
}
public FrmMain() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setExtendedState(MAXIMIZED_BOTH);
setTitle("图书管理系统主界面(欢迎回来"+FrmLogin.user.getUserName()+")");
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
JMenu menu = new JMenu("\u57FA\u672C\u6570\u636E\u7EF4\u62A4");
menu.setIcon(new ImageIcon(FrmMain.class.getResource("/images/base.png")));
menuBar.add(menu);
JMenu mnNewMenu = new JMenu("\u5E74\u7EA7\u4FE1\u606F\u7BA1\u7406");
mnNewMenu.setIcon(new ImageIcon(FrmMain.class.getResource("/images/grade.png")));
menu.add(mnNewMenu);
contentPane.setLayout(new BorderLayout(0, 0));
desktopPane = new JDesktopPane();
contentPane.add(desktopPane);
JToolBar toolBar = new JToolBar();
toolBar.setBounds(0, 178, 432, 31);
contentPane.add(toolBar, BorderLayout.NORTH);
JButton button = new JButton("");
button.setToolTipText("\u56FE\u4E66\u7C7B\u522B\u6DFB\u52A0");
button.setIcon(new ImageIcon(FrmMain.class.getResource("/images/add.png")));
toolBar.add(button);
JButton button_1 = new JButton("");
button_1.setToolTipText("\u56FE\u4E66\u7C7B\u522B\u7EF4\u62A4");
button_1.setIcon(new ImageIcon(FrmMain.class.getResource("/images/edit.png")));
toolBar.add(button_1);
JMenuItem mntmNewMenuItem = new JMenuItem("\u6DFB\u52A0\u5E74\u7EA7\u4FE1\u606F");
mntmNewMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
FrmTypeAdd frmTypeAdd = new FrmTypeAdd();
centerFrm(frmTypeAdd);
}
});
mntmNewMenuItem.setIcon(new ImageIcon(FrmMain.class.getResource("/images/add.png")));
mnNewMenu.add(mntmNewMenuItem);
JMenuItem menuItem = new JMenuItem("\u5E74\u7EA7\u4FE1\u606F\u7EF4\u62A4");
menuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
FrmTypeMgr frmTypeMgr = new FrmTypeMgr();
centerFrm(frmTypeMgr);
}
});
menuItem.setIcon(new ImageIcon(FrmMain.class.getResource("/images/edit.png")));
mnNewMenu.add(menuItem);
JMenu mnNewMenu_1 = new JMenu("\u73ED\u7EA7\u4FE1\u606F\u7BA1\u7406");
mnNewMenu_1.setIcon(new ImageIcon(FrmMain.class.getResource("/images/class.png")));
menu.add(mnNewMenu_1);
JMenuItem mntmNewMenuItem_1 = new JMenuItem("\u6DFB\u52A0\u73ED\u7EA7\u4FE1\u606F");
mntmNewMenuItem_1.setIcon(new ImageIcon(FrmMain.class.getResource("/images/add.png")));
mnNewMenu_1.add(mntmNewMenuItem_1);
JMenuItem menuItem_1 = new JMenuItem("\u73ED\u7EA7\u4FE1\u606F\u7EF4\u62A4");
menuItem_1.setIcon(new ImageIcon(FrmMain.class.getResource("/images/edit.png")));
mnNewMenu_1.add(menuItem_1);
JMenu mnNewMenu_2 = new JMenu("\u5B66\u751F\u4FE1\u606F\u7BA1\u7406");
mnNewMenu_2.setIcon(new ImageIcon(FrmMain.class.getResource("/images/student.png")));
menu.add(mnNewMenu_2);
JMenuItem menuItem_2 = new JMenuItem("\u6DFB\u52A0\u5B66\u751F\u4FE1\u606F");
menuItem_2.setIcon(new ImageIcon(FrmMain.class.getResource("/images/add.png")));
mnNewMenu_2.add(menuItem_2);
JMenuItem mntmNewMenuItem_2 = new JMenuItem("\u5B66\u751F\u4FE1\u606F\u7EF4\u62A4");
mntmNewMenuItem_2.setIcon(new ImageIcon(FrmMain.class.getResource("/images/edit.png")));
mnNewMenu_2.add(mntmNewMenuItem_2);
JMenu menu_2 = new JMenu("\u5B89\u5168\u9000\u51FA");
menu_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
dispose();
}
});
menu_2.setIcon(new ImageIcon(FrmMain.class.getResource("/images/exit.png")));
menu.add(menu_2);
JMenu menu_1 = new JMenu("\u5173\u4E8E\u6211\u4EEC");
menu_1.setIcon(new ImageIcon(FrmMain.class.getResource("/images/about.png")));
menuBar.add(menu_1);
JMenuItem mntmt = new JMenuItem("\u5173\u4E8ET20");
mntmt.setIcon(new ImageIcon(FrmMain.class.getResource("/images/me.png")));
menu_1.add(mntmt);
}
}
下面的是子页面哦
FrmTypeAdd 这是添加页面
package cn.jbit.view;
import java.awt.EventQueue;
import javax.swing.JInternalFrame;
import javax.swing.ImageIcon;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JTextField;
import cn.jbit.dao.GradeDao;
import cn.jbit.dao.impl.GradeDaoimpl;
import cn.jbit.entity.Grade;
import javax.swing.JTextArea;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.util.List;
import java.awt.event.ActionEvent;
public class FrmTypeAdd extends JInternalFrame {
private JTextField txtMC;
Grade grade = new Grade();
GradeDao gDao = new GradeDaoimpl();
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
FrmTypeAdd frame = new FrmTypeAdd();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public FrmTypeAdd() {
setIconifiable(true);
setFrameIcon(new ImageIcon(FrmTypeAdd.class.getResource("/images/bookTypeManager.png")));
setClosable(true);
setTitle("\u5E74\u7EA7\u4FE1\u606F\u6DFB\u52A0");
setBounds(100, 100, 450, 288);
getContentPane().setLayout(null);
JLabel label = new JLabel("\u5E74\u7EA7\u540D\u79F0\uFF1A");
label.setBounds(80, 22, 66, 30);
getContentPane().add(label);
txtMC = new JTextField();
txtMC.setBounds(153, 27, 153, 21);
getContentPane().add(txtMC);
txtMC.setColumns(10);
JLabel label_1 = new JLabel("\u5907\u6CE8\uFF1A");
label_1.setBounds(91, 74, 41, 30);
getContentPane().add(label_1);
JTextArea txtMS = new JTextArea();
txtMS.setBounds(153, 79, 153, 95);
getContentPane().add(txtMS);
JButton bntAdd = new JButton("\u6DFB\u52A0");
bntAdd.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String gradeName = txtMC.getText().trim();
String gradeRemarks = txtMS.getText().trim();
boolean flag = true;
try {
List<Grade> gradelist=gDao.PD(gradeName);
if (gradeName==null||gradeName.equals("")) {
JOptionPane.showMessageDialog(null, "年级名称不能为空");
}else {
if(gradelist.size()!=0){
flag=false;
}else {
flag=true;
}
if (flag==true) {
grade.setGradeName(gradeName);
grade.setGradeRemarks(gradeRemarks);
int r = gDao.add(grade);
if (r != 0) {
JOptionPane.showMessageDialog(null, "添加成功!");
txtMC.setText("");
txtMS.setText("");
} else {
JOptionPane.showMessageDialog(null, "系统出现错误,添加失败");
}
}else {
JOptionPane.showMessageDialog(null, "已经有该年级");
}
}
} catch (Exception e1) {
JOptionPane.showMessageDialog(null, "系统发生一些错误,请联系管理员");
e1.printStackTrace();
}
}
});
bntAdd.setIcon(new ImageIcon(FrmTypeAdd.class.getResource("/images/add.png")));
bntAdd.setBounds(62, 202, 95, 25);
getContentPane().add(bntAdd);
JButton btnReset = new JButton("\u91CD\u7F6E");
btnReset.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
txtMC.setText("");
txtMS.setText("");
}
});
btnReset.setIcon(new ImageIcon(FrmTypeAdd.class.getResource("/images/reset.png")));
btnReset.setBounds(266, 202, 95, 25);
getContentPane().add(btnReset);
}
}
FrmTypeMgr 这是管理维护页面
package cn.jbit.view;
import java.awt.EventQueue;
import java.util.List;
import java.util.Vector;
import javax.swing.JInternalFrame;
import javax.swing.ImageIcon;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JTextField;
import javax.swing.JButton;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import cn.jbit.dao.ClassDao;
import cn.jbit.dao.GradeDao;
import cn.jbit.dao.impl.ClassDaoimpl;
import cn.jbit.dao.impl.GradeDaoimpl;
import cn.jbit.entity.Class;
import cn.jbit.entity.Grade;
import javax.swing.JPanel;
import javax.swing.border.TitledBorder;
import javax.swing.JTextArea;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
public class FrmTypeMgr extends JInternalFrame {
private JTextField txtgradeName;
private JTable table;
private JTextField txtid;
private JTextField txtGradeNa;
JTextArea txtBZ;
Class class1 = new Class();
ClassDao classDao = new ClassDaoimpl();
Grade grade = new Grade();
GradeDao gradeDao = new GradeDaoimpl();
List<Grade> grades = null;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
FrmTypeMgr frame = new FrmTypeMgr();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public FrmTypeMgr() {
setTitle("\u5E74\u7EA7\u4FE1\u606F\u7EF4\u62A4");
setClosable(true);
setIconifiable(true);
setFrameIcon(new ImageIcon(FrmTypeMgr.class.getResource("/images/bookTypeManager.png")));
setBounds(100, 100, 450, 452);
getContentPane().setLayout(null);
JLabel label_1 = new JLabel("\u5E74\u7EA7\u540D\u79F0\uFF1A");
label_1.setBounds(77, 10, 76, 30);
getContentPane().add(label_1);
txtgradeName = new JTextField();
txtgradeName.setBounds(156, 15, 111, 21);
getContentPane().add(txtgradeName);
txtgradeName.setColumns(10);
JButton btnNewButton = new JButton("\u67E5\u8BE2");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String gradeName = txtgradeName.getText().trim();
try {
grades = gradeDao.getgradeName(gradeName);
showgradeName(grades);
} catch (Exception e1) {
JOptionPane.showMessageDialog(null, "查询时出现一些错误,请联系管理员");
e1.printStackTrace();
}
}
});
btnNewButton.setIcon(new ImageIcon(FrmTypeMgr.class.getResource("/images/search.png")));
btnNewButton.setBounds(295, 13, 95, 25);
getContentPane().add(btnNewButton);
JScrollPane scrollPane = new JScrollPane();
scrollPane.setBounds(56, 64, 339, 117);
getContentPane().add(scrollPane);
table = new JTable();
table.addMouseListener(new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
int index = table.getSelectedRow();
Grade selectGrade = grades.get(index);
txtid.setText(selectGrade.getId() + "");
txtGradeNa.setText(selectGrade.getGradeName());
txtBZ.setText(selectGrade.getGradeRemarks());
}
});
table.setModel(new DefaultTableModel(new Object[][] {},
new String[] { "\u7F16\u53F7", "\u5E74\u7EA7\u540D\u79F0", "\u5907\u6CE8" }));
table.getColumnModel().getColumn(0).setPreferredWidth(62);
table.getColumnModel().getColumn(1).setPreferredWidth(122);
table.getColumnModel().getColumn(2).setPreferredWidth(126);
scrollPane.setViewportView(table);
JPanel panel = new JPanel();
panel.setBorder(
new TitledBorder(null, "\u8868\u5355\u64CD\u4F5C", TitledBorder.LEADING, TitledBorder.TOP, null, null));
panel.setBounds(56, 207, 339, 209);
getContentPane().add(panel);
panel.setLayout(null);
JButton btnNewButton_1 = new JButton("\u4FEE\u6539");
btnNewButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int index = table.getSelectedRow();
if (index == -1) {
JOptionPane.showMessageDialog(null, "请选中其中一行");
return;
}
int num = JOptionPane.showConfirmDialog(null, "确认修改?");
if (num == 0) {
int txtgdid = Integer.valueOf(txtid.getText().trim());
String txtgdName = txtGradeNa.getText();
String txtgdBZ = txtBZ.getText();
Grade selectGrade = grades.get(index);
String HYName=selectGrade.getGradeName();
String HYBZ=selectGrade.getGradeRemarks();
try {
List<Grade> gradelist = gradeDao.getAll();
for (Grade grade : gradelist) {
if (txtgdName.equals(grade.getGradeName()) && grade.getId() != txtgdid) {
JOptionPane.showMessageDialog(null, "该年级名称已存在");
txtGradeNa.setText(HYName);
txtBZ.setText(HYBZ);
return;
}
}
int r = gradeDao.update(txtgdName, txtgdBZ, txtgdid);
if (r != 0) {
JOptionPane.showMessageDialog(null, "修改成功!");
} else {
JOptionPane.showMessageDialog(null, "修改失败!");
}
} catch (Exception e1) {
JOptionPane.showMessageDialog(null, "系统出现异常,请联系管理员");
e1.printStackTrace();
}
try {
grades = gradeDao.getAll();
showgradeName(grades);
txtid.setText("");
txtBZ.setText("");
txtGradeNa.setText("");
} catch (Exception e2) {
JOptionPane.showMessageDialog(null, "系统出现异常,请联系管理员");
e2.printStackTrace();
}
}
}
});
btnNewButton_1.setIcon(new ImageIcon(FrmTypeMgr.class.getResource("/images/modify.png")));
btnNewButton_1.setBounds(56, 174, 85, 25);
panel.add(btnNewButton_1);
JButton button = new JButton("\u5220\u9664");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int index = table.getSelectedRow();
if (index == -1) {
JOptionPane.showMessageDialog(null, "请选中其中一行");
return;
}
int num = JOptionPane.showConfirmDialog(null, "确认删除?");
if (num == 0) {
Grade selectGrade = grades.get(index);
int txtgdid = selectGrade.getId();
try {
List<Class> classes = classDao.getgradeId();
for (Class class1 : classes) {
if (txtgdid == class1.getGradeId()) {
JOptionPane.showMessageDialog(null, "不能删除此年级,因为已经存在其年级的班级!");
return;
}
}
} catch (Exception e2) {
e2.printStackTrace();
}
grade.setId(txtgdid);
int r = gradeDao.del(grade);
if (r != 0) {
JOptionPane.showMessageDialog(null, "删除成功!");
} else {
JOptionPane.showMessageDialog(null, "删除失败!");
}
try {
grades = gradeDao.getAll();
showgradeName(grades);
txtid.setText("");
txtBZ.setText("");
txtGradeNa.setText("");
} catch (Exception e1) {
JOptionPane.showMessageDialog(null, "系统出现异常,请联系管理员");
e1.printStackTrace();
}
}
}
});
button.setIcon(new ImageIcon(FrmTypeMgr.class.getResource("/images/delete.png")));
button.setBounds(192, 174, 95, 25);
panel.add(button);
JLabel txtbh = new JLabel("\u7F16\u53F7:");
txtbh.setBounds(32, 25, 54, 15);
panel.add(txtbh);
txtid = new JTextField();
txtid.setEnabled(false);
txtid.setBounds(68, 22, 66, 21);
panel.add(txtid);
txtid.setColumns(10);
JLabel label = new JLabel("\u5E74\u7EA7\u540D\u79F0\uFF1A");
label.setBounds(144, 17, 66, 30);
panel.add(label);
txtGradeNa = new JTextField();
txtGradeNa.setColumns(10);
txtGradeNa.setBounds(207, 22, 111, 21);
panel.add(txtGradeNa);
JLabel label_3 = new JLabel("\u5907\u6CE8:");
label_3.setBounds(44, 64, 54, 15);
panel.add(label_3);
txtBZ = new JTextArea();
txtBZ.setBounds(90, 61, 197, 94);
panel.add(txtBZ);
try {
grades = gradeDao.getAll();
showgradeName(grades);
} catch (Exception e) {
JOptionPane.showMessageDialog(null, "系统出现错误,请联系管理");
e.printStackTrace();
}
}
private void showgradeName(List<Grade> grades) {
DefaultTableModel dtm = (DefaultTableModel) table.getModel();
dtm.setRowCount(0);
for (Grade grade : grades) {
Vector<String> v = new Vector<>();
v.add(String.valueOf(grade.getId()));
v.add(grade.getGradeName());
v.add(grade.getGradeRemarks());
dtm.addRow(v);
}
}
}