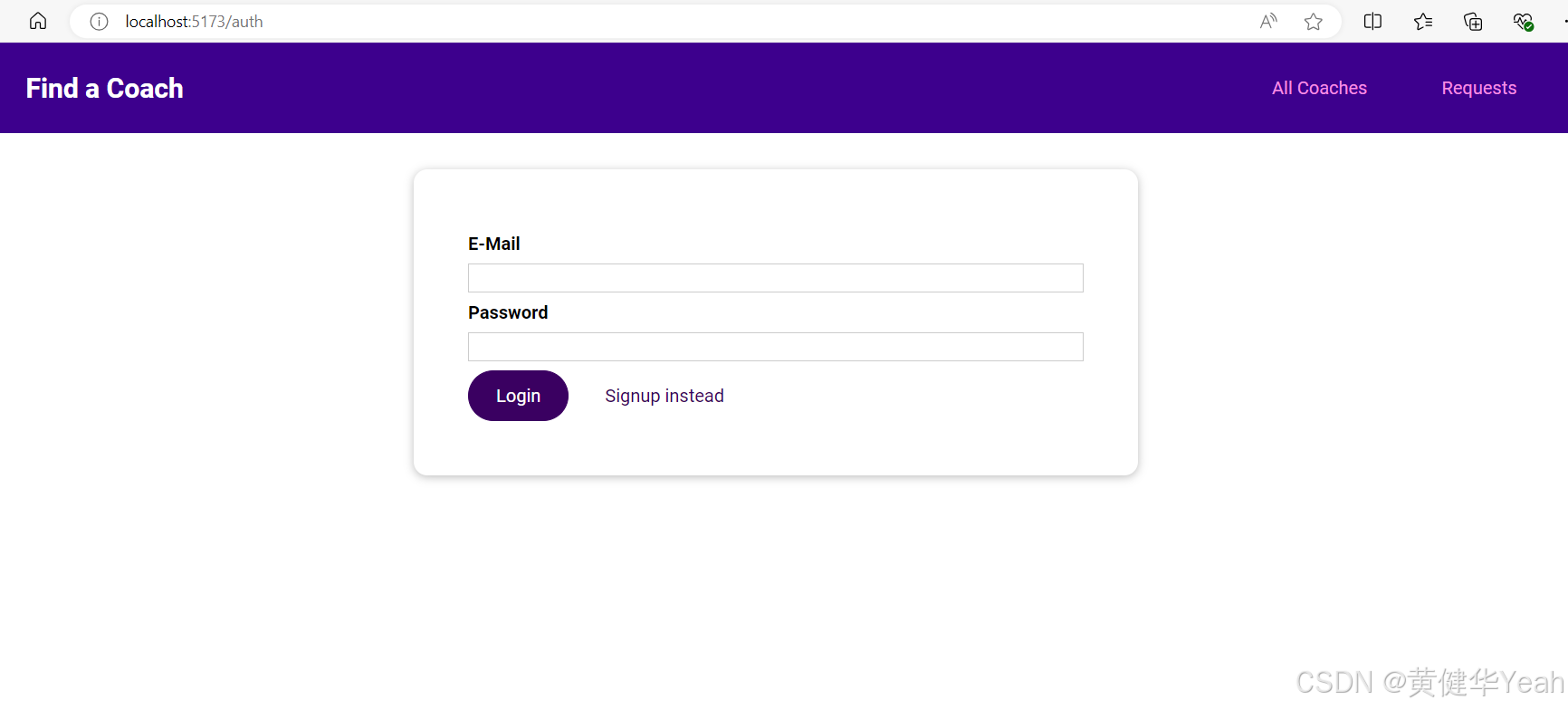
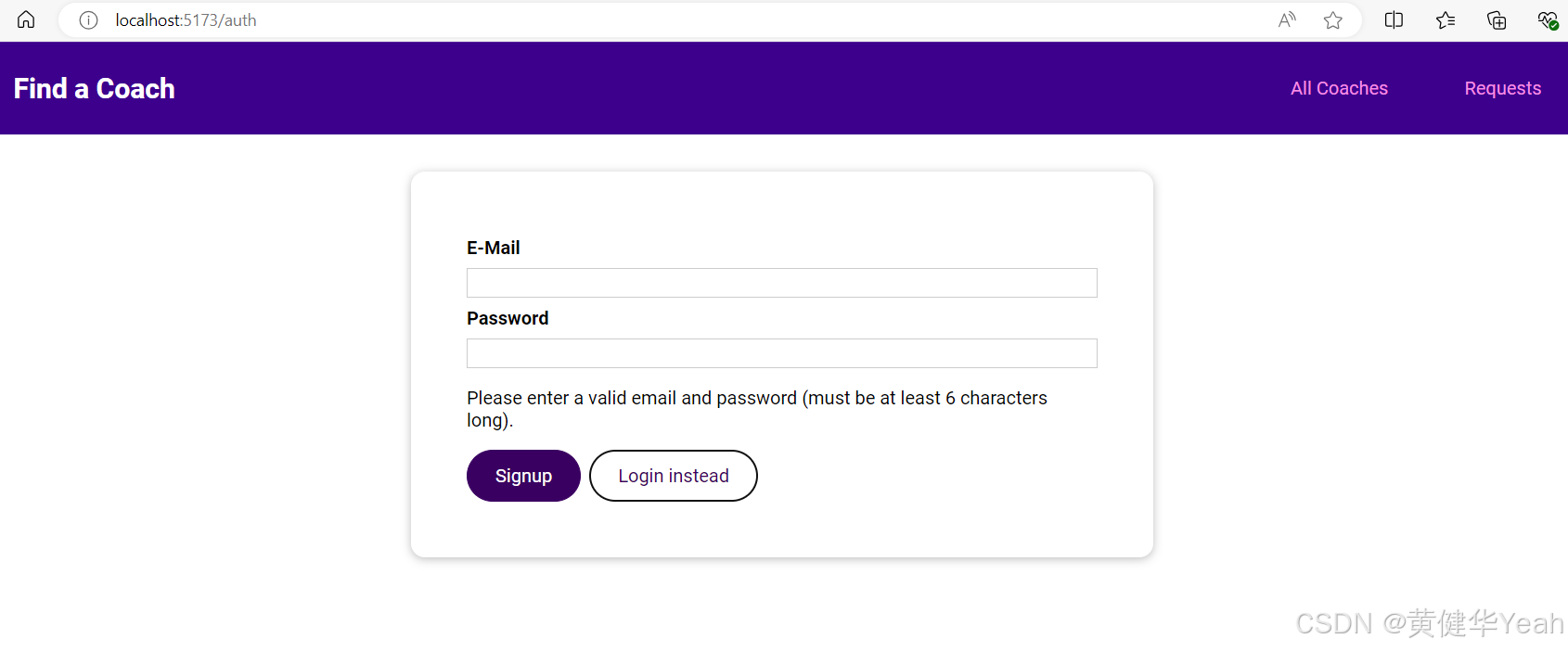
步骤
1、新建pages/auth/UserAuth.vue
<template>
<base-card>
<form @submit.prevent="submitForm">
<div class="form-control">
<label for="email">E-Mail</label>
<input type="email" id="email" v-model.trim="email" />
</div>
<div class="form-control">
<label for="password">Password</label>
<input type="password" id="password" v-model.trim="password" />
</div>
<p
v-if="!formIsValid"
>Please enter a valid email and password (must be at least 6 characters long).</p>
<base-button>{{ submitButtonCaption }}</base-button>
<base-button type="button" mode="flat" @click-button="switchAuthMode">{{ switchModeButtonCaption }}</base-button>
</form>
</base-card>
</template>
<script>
export default {
data() {
return {
email: '',
password: '',
formIsValid: true,
mode: 'login',
};
},
computed: {
submitButtonCaption() {
if (this.mode === 'login') {
return 'Login';
} else {
return 'Signup';
}
},
switchModeButtonCaption() {
if (this.mode === 'login') {
return 'Signup instead';
} else {
return 'Login instead';
}
},
},
methods: {
submitForm() {
this.formIsValid = true;
if (
this.email === '' ||
!this.email.includes('@') ||
this.password.length < 6
) {
this.formIsValid = false;
return;
}
// send http request...
},
switchAuthMode() {
console.log('switching auth mode...');
if (this.mode === 'login') {
this.mode = 'signup';
} else {
this.mode = 'login';
}
console.log('new mode:', this.mode);
},
},
};
</script>
<style scoped>
form {
margin: 1rem;
padding: 1rem;
}
.form-control {
margin: 0.5rem 0;
}
label {
font-weight: bold;
margin-bottom: 0.5rem;
display: block;
}
input,
textarea {
display: block;
width: 100%;
font: inherit;
border: 1px solid #ccc;
padding: 0.15rem;
}
input:focus,
textarea:focus {
border-color: #3d008d;
background-color: #faf6ff;
outline: none;
}
</style>
2、更新BaseButton.vue,添加emit
<template>
<button v-if="!link" :class="mode" @click="handleClick">
<slot></slot>
</button>
<router-link v-if="link" :to="to" :class="mode" @click="handleClick">
<slot></slot>
</router-link>
</template>
<script>
export default {
emits: ['click-button'],
props:{
mode:{
type: String,
required: false,
default: null
},
link:{
type: Boolean,
required: false,
default: false
},
to:{
type: String,
required: false,
default: '/'
}
},
methods: {
handleClick() {
this.$emit('click-button');
}
}
};
</script>
<style scoped>
button,
a {
text-decoration: none;
padding: 0.75rem 1.5rem;
font: inherit;
background-color: #3a0061;
border: 1px solid #3a0061;
color: white;
cursor: pointer;
border-radius: 30px;
margin-right: 0.5rem;
display: inline-block;
}
a:hover,
a:active,
button:hover,
button:active {
background-color: #270041;
border-color: #270041;
}
.flat {
background-color: transparent;
color: #3a0061;
border: none;
}
.outline {
background-color: transparent;
border-color: #270041;
color: #270041;
}
.flat:hover,
.flat:active,
.outline:hover,
.outline:active {
background-color: #edd2ff;
}
</style>
3、router.js中添加/auth
import { createRouter, createWebHistory } from "vue-router";
import CoachDetail from "./pages/coaches/CoachDetail.vue";
import CoachesList from "./pages/coaches/CoachesList.vue";
import CoachRegistration from "./pages/coaches/CoachRegistration.vue";
import ContactCoach from "./pages/requests/ContactCoach.vue";
import RequestsReceived from "./pages/requests/RequestsReceived.vue";
import UserAuth from "./pages/auth/UserAuth.vue";
import NotFound from "./pages/NotFound.vue";
const router = createRouter({
history: createWebHistory(),
routes: [
{ path: '/', redirect: '/coaches' },
{ path: '/coaches', component: CoachesList },
{
path: '/coaches/:id',
component: CoachDetail,
props: true, // make id available as a prop
children: [
{ path: 'contact', component: ContactCoach }, // /coaches/1234567890/contact
]
},
{ path: '/register', component: CoachRegistration },
{ path: '/requests', component: RequestsReceived },
{ path: '/auth', component: UserAuth },
{ path: '/:notFound(.*)', component: NotFound },
],
});
export default router;