/**
* map工具类
*/
public class MapUtils extends MapUtil {
public static final Map EMPTY_MAP = new EmptyMap();
/**
* map 分组
* param map
* param groupSize 分组大小
*/
public static <V extends Serializable> List<Map<String, V>> groupList(Map<String, V> map, int groupSize){
if(isEmpty(map)){
return null;
}
int total = map.size();
List<Map<String, V>> listEmailList = new ArrayList<Map<String, V>>();
if(total < groupSize){
listEmailList.add(map);
}else{
int pageCount = PageUtils.getPageCount(total, groupSize);
for(int i=1; i<=pageCount;i++){
listEmailList.add(sub(map, i, groupSize));
}
}
return listEmailList;
}
public static <V extends Serializable> Map<String, V> sub(Map<String, V> map, int pageNo, int size){
Map<String, V> newMap = null;
if(map instanceof LinkedHashMap)
newMap = new LinkedHashMap();
else if(map instanceof TreeMap)
newMap = new TreeMap();
else
newMap = new HashMap();
int start = (pageNo - 1) * size;
int dex = 0, end = start+size;
for (Map.Entry<String, V> entry : map.entrySet()) {
dex++;
if(dex < start)
continue;
else if(dex > end)
break;
newMap.put(entry.getKey(), entry.getValue());
}
return newMap;
}
public static void filterNullVal(Map map){
if(isEmpty(map)){
return;
}
List<Object> filterKeys = new ArrayList(5);
Object val = null;
for (Object key : map.keySet()) {
val = map.get(key);
if(val == null || val.toString().length() == 0){
filterKeys.add(key);
}
}
for (Object filterKey : filterKeys) {
map.remove(filterKey);
}
}
/**
* 去重复值
* @param map
* @return
*/
public static Map distinctVal(Map map){
if(isEmpty(map) || map.size() < 2)
return map;
Map newMap = new HashMap();
for (Object key : map.keySet()) {
if(!newMap.containsValue(map.get(key))){
newMap.put(key, map.get(key));
}
}
return newMap;
}
}
MapUtils
最新推荐文章于 2023-12-11 15:08:57 发布
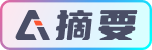