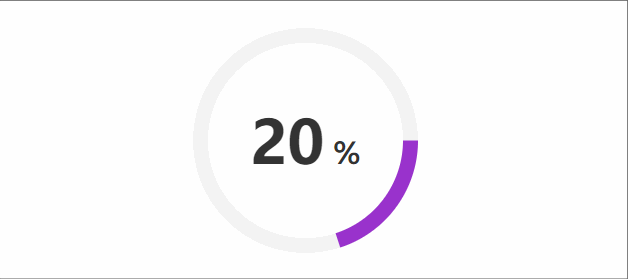
<div class="percent">
<svg>
<circle cx="70" cy="70" r="70" />
<circle id="circle" cx="70" cy="70" r="70" />
</svg>
<div class="number">
<h3>
<span id="percent">20</span>
<span>%</span>
</h3>
</div>
</div>
<script type="text/javascript">
const percentBox = document.querySelector("#percent")
const circle = document.querySelector("#circle")
let percent = 20
const timer = setInterval(() => {
percent += Math.ceil(Math.random() * 30)
if (percent > 100) {
percent = 100
clearInterval(timer)
}
percentBox.innerHTML = percent
circle.style.strokeDashoffset = `calc(440 - 440 * (${percent} / 100))`
}, 1500
)
</script>
.percent {
position: relative;
width: 150px;
height: 150px;
svg {
width: 150px;
height: 150px;
circle {
fill: none;
stroke-width: 10;
transform: translate(5px, 5px);
stroke-dasharray: 440;
stroke-dashoffset: 440;
&:nth-child(1) {
stroke-dashoffset: 0;
stroke: #f3f3f3;
}
&:nth-child(2) {
stroke-dashoffset: calc(440 - 440 * (20/100));
stroke: darkorchid;
}
}
}
.number {
position: absolute;
left: 0;
top: 0;
display: flex;
justify-content: center;
align-items: center;
width: 100%;
height: 100%;
color: #333;
span {
&:nth-child(1) {
font-size: 40px;
}
&:nth-child(2) {
font-size: 20px;
}
}
}
}