一.导入依赖-->添加'编辑器'
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>添加宣传任务</title>
<!-- 导入jquery核心类库 -->
<script type="text/javascript" src="../../js/jquery-1.8.3.js"></script>
<!-- 导入easyui类库 -->
<link rel="stylesheet" type="text/css" href="../../js/easyui/themes/default/easyui.css">
<link rel="stylesheet" type="text/css" href="../../js/easyui/themes/icon.css">
<link rel="stylesheet" type="text/css" href="../../css/default.css">
<script type="text/javascript" src="../../js/easyui/jquery.easyui.min.js"></script>
<script src="../../js/easyui/locale/easyui-lang-zh_CN.js" type="text/javascript"></script>
<script type="text/javascript" src="../../editor/kindeditor.js"></script>
<script type="text/javascript" src="../../editor/lang/zh_CN.js"></script>
<link rel="stylesheet" type="text/css" href="../../editor/themes/default/default.css" />
<script type="text/javascript">
$(function() {
$("body").css({
visibility: "visible"
});
$("#back").click(function() {
location.href = "promotion.html";
});
//页面提供<textarea>文本框
KindEditor.ready(function(K) {
window.editor = K.create('#description', {
items: [
'source', '|', 'undo', 'redo', '|', 'preview', 'print', 'template', 'code', 'cut', 'copy', 'paste',
'plainpaste', 'wordpaste', '|', 'justifyleft', 'justifycenter', 'justifyright',
'justifyfull', 'insertorderedlist', 'insertunorderedlist', 'indent', 'outdent', 'subscript',
'superscript', 'clearhtml', 'quickformat', 'selectall', '|', 'fullscreen', '/',
'formatblock', 'fontname', 'fontsize', '|', 'forecolor', 'hilitecolor', 'bold',
'italic', 'underline', 'strikethrough', 'lineheight', 'removeformat', '|', 'image', 'multiimage',
'flash', 'media', 'insertfile', 'table', 'hr', 'emoticons', 'baidumap', 'pagebreak',
'anchor', 'link', 'unlink', '|', 'about'
],
allowFileManager: true,
uploadJson: '../../image_upload.action',
//图片上传
fileManagerJson: '../../image_manage.action'
});
});
$("#save").click(function() {
if($("#promotionForm").form('validate')) {
//通过kindEditor提交textarea默认是不自动提交,需手动提交
window.editor.sync();
//提交表单
$("#promotionForm").submit();
} else {
//校验失败
$.messager.alert("校验失败", "表单存在非法数据", "warning");
}
});
});
</script>
</head>
<body class="easyui-layout" style="visibility:hidden;">
<div region="north" style="height:31px;overflow:hidden;" split="false" border="false">
<div class="datagrid-toolbar">
<a id="save" icon="icon-save" href="#" class="easyui-linkbutton" plain="true">保存</a>
<a id="back" icon="icon-back" href="#" class="easyui-linkbutton" plain="true">返回列表</a>
</div>
</div>
<div region="center" style="overflow:auto;padding:5px;" border="false">
<form id="promotionForm" method="post" enctype="multipart/form-data" action="../../promotion_save.action">
<table class="table-edit" width="95%" align="center">
<tr class="title">
<td colspan="4">宣传任务</td>
</tr>
<tr>
<td>宣传概要(标题):</td>
<td colspan="3">
<input type="text" name="title" id="title" class="easyui-validatebox" required="true" />
</td>
</tr>
<tr>
<td>活动范围:</td>
<td>
<input type="text" name="activeScope" id="activeScope" class="easyui-validatebox" />
</td>
<td>宣传图片:</td>
<td>
<input type="file" name="titleImgFile" id="titleImg" class="easyui-validatebox" required="true" />
</td>
</tr>
<tr>
<td>发布时间: </td>
<td>
<input type="text" name="startDate" id="startDate" class="easyui-datebox" required="true" />
</td>
<td>失效时间: </td>
<td>
<input type="text" name="endDate" id="endDate" class="easyui-datebox" required="true" />
</td>
</tr>
<tr>
<td>宣传内容(活动描述信息):</td>
<td colspan="3">
<textarea id="description" name="description" style="width:80%" rows="20"></textarea>
</td>
</tr>
</table>
</form>
</div>
</body>
</html>
2.编辑器效果
3.编辑器点击上传图片,-->编写上传图片action( uploadJson: '../../image_upload.action',)
package cn.itcast.bos.web.action.base;
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import java.util.UUID;
import org.apache.commons.io.FileUtils;
import org.apache.struts2.ServletActionContext;
import org.apache.struts2.convention.annotation.Action;
import org.apache.struts2.convention.annotation.Namespace;
import org.apache.struts2.convention.annotation.ParentPackage;
import org.apache.struts2.convention.annotation.Result;
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Controller;
import com.opensymphony.xwork2.ActionContext;
import com.opensymphony.xwork2.ActionSupport;
import com.opensymphony.xwork2.ModelDriven;
@ParentPackage("json-default")
@Namespace("/")
@Controller
@Scope("prototype")
public class ImageAction extends ActionSupport implements ModelDriven<Object> {
@Override
public Object getModel() {
return null;
}
private File imgFile; //文件
private String imgFileFileName; //文件名
private String imgFileContentType; //文件类型
public void setImgFile(File imgFile) {
this.imgFile = imgFile;
}
public void setImgFileFileName(String imgFileFileName) {
this.imgFileFileName = imgFileFileName;
}
public void setImgFileContentType(String imgFileContentType) {
this.imgFileContentType = imgFileContentType;
}
@Action(value = "image_upload", results = { @Result(name = "success", type = "json") })
public String upload() throws IOException {
System.out.println("文件"+imgFile);
System.out.println("文件名"+imgFileFileName);
System.out.println("文件类型"+imgFileContentType);
String savePath = ServletActionContext.getServletContext().getRealPath("/upload/");
String saveURl = ServletActionContext.getRequest().getContextPath()+"/upload/";
//生成图片随机名字
UUID randomUUID = UUID.randomUUID();
String substring = imgFileFileName.substring(imgFileFileName.lastIndexOf("."));
String randomFileName=randomUUID+substring;
//保存图片(绝对路径)
File destFile = new File(savePath+"/"+randomFileName);
FileUtils.copyFile(imgFile, destFile);
//通知浏览器上传成功
HashMap<String,Object> hashMap = new HashMap<String, Object>();
hashMap.put("error",0);
hashMap.put("url", saveURl+randomFileName); //返回相对路径
ActionContext.getContext().getValueStack().push(hashMap);
return SUCCESS;
}
}
注意:Action中的文件,文件名,文件类型字段名子必须和页面HTML的''宣传照片''标签name属性名子一致;
二,表单提交
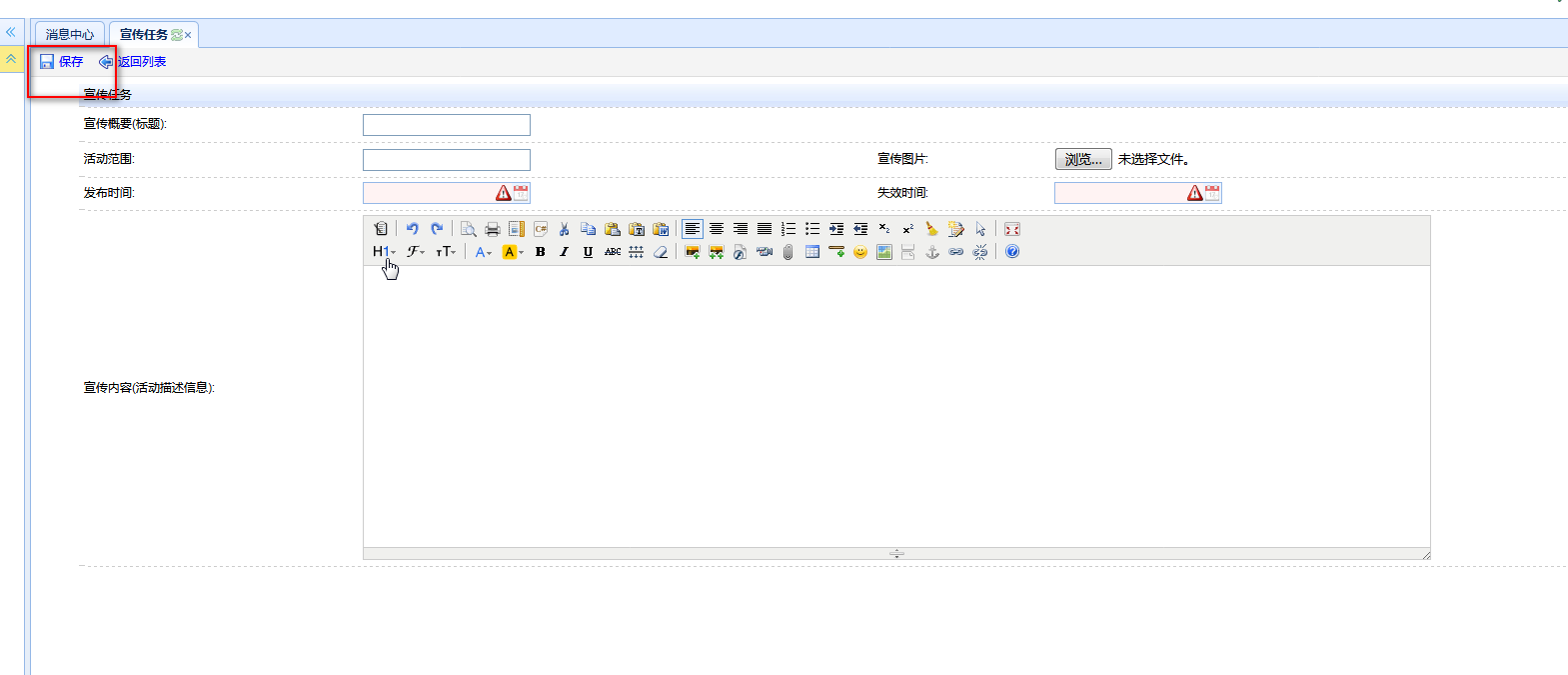
1.填写表格,进入Action
package cn.itcast.bos.web.action.base;
import java.io.File;
import java.io.IOException;
import java.util.UUID;
import org.apache.commons.io.FileUtils;
import org.apache.struts2.ServletActionContext;
import org.apache.struts2.convention.annotation.Action;
import org.apache.struts2.convention.annotation.Namespace;
import org.apache.struts2.convention.annotation.ParentPackage;
import org.apache.struts2.convention.annotation.Result;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Controller;
import cn.itcast.bos.domain.take_delivery.Promotion;
import cn.itcast.bos.service.base.PromotionService;
import com.opensymphony.xwork2.ActionSupport;
import com.opensymphony.xwork2.ModelDriven;
@ParentPackage("json-default")
@Namespace("/")
@Controller
@Scope("prototype")
public class PromotionAction extends ActionSupport implements ModelDriven<Promotion>{
private File titleImgFile; // 文件
private String titleImgFileFileName; // 文件名
public void setTitleImgFile(File titleImgFile) {
this.titleImgFile = titleImgFile;
}
public void setTitleImgFileFileName(String titleImgFileFileName) {
this.titleImgFileFileName = titleImgFileFileName;
}
private Promotion promotion = new Promotion();
@Override
public Promotion getModel() {
return promotion;
}
@Autowired
private PromotionService promotionService;
@Action(value="promotion_save",results={@Result(name="success",type="redirect",location="./pages/take_delivery/promotion.html")})
public String save() throws IOException{
String savePath = ServletActionContext.getServletContext().getRealPath(
"/upload/");
String saveURl = ServletActionContext.getRequest().getContextPath()
+ "/upload/";
// 生成图片随机名字
UUID randomUUID = UUID.randomUUID();
String substring = titleImgFileFileName.substring(titleImgFileFileName
.lastIndexOf("."));
String randomFileName = randomUUID + substring;
// 保存图片(绝对路径)
File destFile = new File(savePath + "/" + randomFileName);
FileUtils.copyFile(titleImgFile, destFile);
//将保存路径 相对工程web访问路径保存到对象当中
promotion.setTitleImg(ServletActionContext.getRequest().getContextPath()
+ "/upload/"+randomFileName);
promotionService.save(promotion);
return SUCCESS;
}
}