//不建议全部复制,因为有多个游戏代码在里面,感谢支持!!!
#include <iostream> //贪吃蛇
#include <cstdlib>
#include <ctime>
#include <vector>
using namespace std;
// 定义贪吃蛇类
class Snake {
public:
Snake(int x, int y);
void move(int dx, int dy);
bool isAlive() const;
private:
vector<int> pos;
int length;
};
// 定义食物类
class Food {
public:
Food();
int getX() const;
int getY() const;
private:
int x;
int y;
};
// 游戏主类
class Game {
public:
Game(int width, int height);
void play();
private:
int width;
int height;
Snake snake;
Food food;
};
// 初始化蛇的移动方向和位置
void initSnake(Snake& snake, int x, int y) {
snake.pos.push_back(x);
snake.pos.push_back(y);
snake.length = 1;
}
// 随机生成食物的位置
void initFood(Food& food) {
srand(time(NULL)); // 设置随机数种子
do {
food.x = rand() % Game::getWidth() + 1;
food.y = rand() % Game::getHeight() + 1;
} while (food.x == snake.pos[0] && food.y == snake.pos[1]); // 避免食物和蛇头重合
}
// 初始化游戏环境
Game::Game(int width, int height) {
this->width = width;
this->height = height;
initSnake(snake, width / 2, height / 2); // 设置蛇头位置为屏幕中心
initFood(food); // 随机生成食物位置
}
// 控制蛇的移动
void Snake::move(int dx, int dy) {
pos.erase(pos.begin()); // 删除蛇尾位置
pos.push_back(pos.back() + dx); // 移动蛇身
pos.push_back(pos.back() + dy); // 移动蛇身
length++; // 增加蛇的长度
}
// 判断蛇是否吃到食物
bool Snake::isAlive() const {
return length > 1; // 只有当蛇身体长度大于1时才表示蛇是活着的
}
// 游戏主循环
void Game::play() {
bool gameOver = false;
while (!gameOver) {
// 随机生成食物
initFood(food);
// 检测玩家操作,控制蛇的移动
int dx = 0;
int dy = 0;
cout << "Press arrow keys to move the snake: ";
cin >> dx >> dy;
if (dx == 0 && dy == 0) continue; // 如果玩家没有操作,则跳过本次循环
snake.move(dx, dy); // 控制蛇的移动
// 判断蛇是否吃到食物
if (snake.isAlive() && food.getX() == snake.pos[0] && food.getY() == snake.pos[1]) {
cout << "You got the food!" << endl;
snake.length++; // 增加蛇的长度
initFood(food); // 重新生成食物位置
}
// 判断蛇是否碰到自己
for (int i = 1; i < snake.length; i++) {
if (snake.pos[i-1] == snake.pos[i] && snake.pos[i+1] == snake.pos[i]) {
gameOver = true;
break;
}
}
// 判断游戏是否结束
if (gameOver || !snake.isAlive()) {
cout << "Game over!" << endl;
break;
}
}
}
int main() {
Game game(80, 25);
game.play();
return 0;
}
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
#include <iostream> //坦克大战
#include <conio.h>
#include <windows.h>
#define WIDTH 40
#define HEIGHT 20
using namespace std;
// 游戏状态
enum GameState {
GS_START,
GS_PLAY,
GS_GameOver
};
// 坦克类
class Tank {
public:
int x, y;
bool dir;
tank(int x, int y) : x(x), y(y), dir(false) {}
void move();
};
// 移动坦克
void tank::move() {
if (dir) {
x += 2;
} else {
x -= 2;
}
if (x >= WIDTH || x <= 0) {
dir = !dir;
}
}
// 游戏窗口
class GameWindow {
public:
GameWindow() : gamestate(GS_START), score(0),难(' ') {
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
board[i][j] = ' ';
}
}
}
void draw();
void update();
void input();
private:
GameState gamestate;
int score;
char board[HEIGHT][WIDTH];
tank tanks[10];
int tankcount;
char难;
};
// 绘制游戏窗口
void GameWindow::draw() {
system("cls");
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
cout << board[i][j];
}
cout << endl;
}
cout << "Score: " << score << endl;
}
// 更新游戏状态
void GameWindow::update() {
switch (gamestate) {
case GS_START:
draw();
break;
case GS_Play:
for (int i = 0; i < tankcount; i++) {
tanks[i].move();
}
for (int i = 0; i < tankcount; i++) {
for (int j = 0; j < HEIGHT; j++) {
if (board[j][tanks[i].x] == '#') {
continue;
}
if (tanks[i].x == j) {
board[j][tanks[i].x] = 'O';
score++;
break;
}
board[j][tanks[i].x] = 'X';
break;
}
}
draw();
break;
case GS_GameOver:
cout << "Game Over!" << endl;
break;
}
}
// 处理输入事件
void GameWindow::input() {
if (_kbhit()) {
char c = _getch();
switch (c) {
case 'a':
case 'A':
for (int i = 0; i < tankcount; i++) {
tanks[i].x -= 2;
}
break;
case 'd':
case 'D':
for (int i = 0; i < tankcount; i++) {
tanks[i].x += 2;
}
break;
case 'w':
case 'W':
for (int i = 0; i < tankcount; i++) {
tanks[i].y -= 2;
}
break;
case 's':
case 'S':
for (int i = 0; i < tankcount; i++) {
tanks[i].y += 2;
}
break;
case ' ': //开始新游戏,清空游戏状态和分数,重新初始化坦克位置和方向,随机生成障碍物。
gamestate = GS_Play;
score = 0;
for (int i = 0; i < tankcount; i++) {
tanks[i].x = rand() % WIDTH;
tanks[i].y = rand() % HEIGHT;
tanks[i].dir = rand() % 2 == 0;
}
for (int i = 0; i < HEIGHT; i++) {
board[i][WIDTH - 1] = '#';
}
break;
case 'q':
case 'Q':
gamestate = GS_GameOver;
break;
default:
break;
}
}
}
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
#include <iostream> //猜数字
#include <cstdlib>
#include <ctime>
using namespace std;
int main()
{
int num, guess, tries = 0;
// 生成一个 1~100 的随机数
srand(time(NULL));
num = rand() % 100 + 1;
cout << "猜数字游戏\n";
do
{
cout << "请输入一个 1~100 的整数: ";
cin >> guess;
tries++;
if (guess > num)
{
cout << "太大了!\n";
}
else if (guess < num)
{
cout << "太小了!\n";
}
else
{
cout << "恭喜你,猜对了!你用了 " << tries << " 次猜对了!\n";
}
} while (guess != num);
return 0;
}
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
#include <iostream> //俄罗斯方块
#include <conio.h>
using namespace std;
const int ROWS = 20;
const int COLS = 10;
int blockTypes[4] = {
'{', '}', '|', '_'
};
int board[ROWS][COLS];
int currentType = 0;
void clearBoard()
{
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = ' ';
}
}
}
void printBoard()
{
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
cout << board[i][j];
}
cout << endl;
}
}
bool isvalid(int x, int y)
{
if (x < 0 || x >= COLS || y < 0 || y >= ROWS) {
return false;
}
return true;
}
void dropBlock(int type)
{
int x = ROWS - 1;
int y = COLS - 1;
while (isvalid(x, y)) {
board[x][y] = type + 48;
x--;
y--;
}
}
void shiftRowsDown()
{
for (int i = 0; i < ROWS - 1; i++) {
for (int j = 0; j < COLS; j++) {
board[i][j] = board[i+1][j];
}
}
board[ROWS-1][COLS-1] = ' '; // empty the last row.
}
void shiftRowsUp()
{
for (int i = ROWS - 1; i > 0; i--) {
for (int j = 0; j < COLS; j++) {
board[i][j] = board[i-1][j];
}
}
board[0][Cols-1] = ' '; // empty the first row.
}
void moveBlockLeft()
{
int x = ROWS - 1;
int y = COLS - 1;
while (isvalid(x, y)) {
board[x][y] = ' '; // make the space empty.
x--; y--; // move the pointer to the right.
} x++; y++; // bring the pointer back to its original position. we didn't count the last empty space in the loop above. so it needs to be empty now.
board[x][y] = currentType + 48; // set the block type back to its original value. remember that we subtracted 48 to get the actual ASCII value of the character. so we need to add it back to get the actual character. } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } } }}
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
——————————————————————————————————————————————————————————————————————————————————————
#include <iostream> //加解密钥
#include <string>
#include <unordered_map>
using namespace std;
string encrypt(string message, string key) {
unordered_map<char, char> cipher;
string result = "";
// 生成密钥映射表
for (int i = 0; i < key.length(); i++) {
cipher[key[i]] = message[i];
}
// 对消息进行加密
for (auto& pair : cipher) {
result += pair.second;
}
return result;
}
string decrypt(string message, string key) {
unordered_map<char, char> cipher;
string result = "";
// 根据密钥映射表进行解密
for (int i = 0; i < key.length(); i++) {
cipher[message[i]] = key[i];
}
// 对消息进行解密
for (int i = 0; i < message.length(); i++) {
result += cipher[message[i]];
}
return result;
}
int main() {
string message = "你好,世界!";
string key = "密匙";
cout << "原始消息: " << message << endl;
cout << "密钥: " << key << endl;
// 对消息进行加密
string encryptedMessage = encrypt(message, key);
cout << "加密后的消息: " << encryptedMessage << endl;
// 对加密后的消息进行解密
string decryptedMessage = decrypt(encryptedMessage, key);
cout << "解密后的消息: " << decryptedMessage << endl;
return 0;
}
c++游戏集合{干货}(全是干货)
于 2023-07-31 22:46:02 首次发布
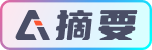