登录
<template>
<div>
<h1>登录</h1>
<el-form
:model="ruleForm"
:rules="rules"
ref="ruleForm"
label-width="100px"
class="demo-ruleForm"
>
<el-form-item label="活动名称" prop="username">
<el-input v-model="ruleForm.username"></el-input>
</el-form-item>
<el-form-item label="活动区域" prop="password">
<el-input v-model="ruleForm.password"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('ruleForm')">立即创建</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
export default {
name: "",
data() {
return {
ruleForm: {
username:"",
password:"",
},
rules: {
username:[{ required: true, message: '请输入活动名称', trigger: 'blur' }],
password:[{ required: true, message: '请输入活动名称', trigger: 'blur' }],
}
};
},
mounted() {
},
methods: {
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
this.$axios.post('https://www.liulongbin.top:8888/api/private/v1/login',this.ruleForm).then(res=>{
console.log(res);
if(res.meta.status==200){
sessionStorage.setItem('03B-token',res.data.token)
this.$store.commit('add',this.ruleForm.username)
this.$router.go(-1)
this.$message.success(res.meta.msg);
}else{
this.$message.error(res.meta.msg);
}
})
} else {
this.$message.error('错了哦,这是一条错误消息');
return false;
}
});
},
},
computed: {},
watch: {},
};
</script>
<style scoped></style>
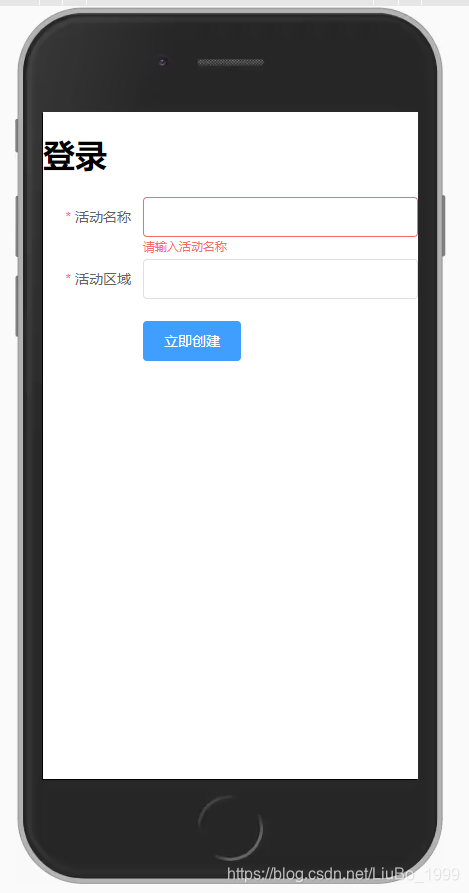
主页面
<template>
<div class="bian">
<router-view />
<ul>
<router-link to="/shoye" tag="li">首页</router-link>
<router-link to="/fenlei" tag="li">分类</router-link>
<router-link to="/gowuche" tag="li">购物车</router-link>
<router-link to="/wode" tag="li">我的</router-link>
</ul>
</div>
</template>
<script>
export default {
name: "",
data() {
return {};
},
mounted() {
},
methods: {},
computed: {},
watch: {},
};
</script>
<style scoped>
.bian{
width: 100%;
height: 100%;
}
ul{
width: 100%;
height: 50px;
background: #666;
display: flex;
position: fixed;
bottom: 0;
font-size: 20px;
}
li{
width: 25%;
text-align: center;
line-height:50px;
}
.router-link-active{
background: blue;
color: #fff;
}
</style>
## 首页
<template>
<div>
<p class="p">积云商城 <span class="span"><i class="el-icon-search"></i></span> </p>
<div>
<van-swipe class="my-swipe" :autoplay="3000" indicator-color="white">
<van-swipe-item>1</van-swipe-item>
<van-swipe-item>2</van-swipe-item>
<van-swipe-item>3</van-swipe-item>
<van-swipe-item>4</van-swipe-item>
</van-swipe>
</div>
<div >
<p><img :src="result.section1.banner"></p>
<div class="sss">
<p class="dx" v-for="(item,index) in result" :key="index"><img :src="result.section1.list[0].imgPath"></p>
</div>
</div>
</div>
</template>
<script>
import Vue from 'vue';
import { Swipe, SwipeItem } from 'vant';
Vue.use(Swipe);
Vue.use(SwipeItem);
export default {
name: '',
data() {
return {
result:''
};
},
mounted() {
this.$axios.get('http://api.com/index').then((res) => {
console.log(res);
this.result = res;
});
},
methods: {},
computed: {},
watch: {},
};
</script>
<style scoped>
.p{
width: 100%;
height: 30px;
text-align: center;
font-size: 20px;
position: relative;
}
.span{
width: 20px;
height: 20px;
position: absolute;
top: 0;
right: 10px;
}
.my-swipe .van-swipe-item {
color: #fff;
font-size: 20px;
line-height: 150px;
text-align: center;
background-color: #39a9ed;
}
img{
width: 100%;
}
.sss{
width: 100%;
height: 100%;
display: flex;
flex-wrap: wrap;
}
.dx{
width: 47%;
margin: 1%;
}
</style>
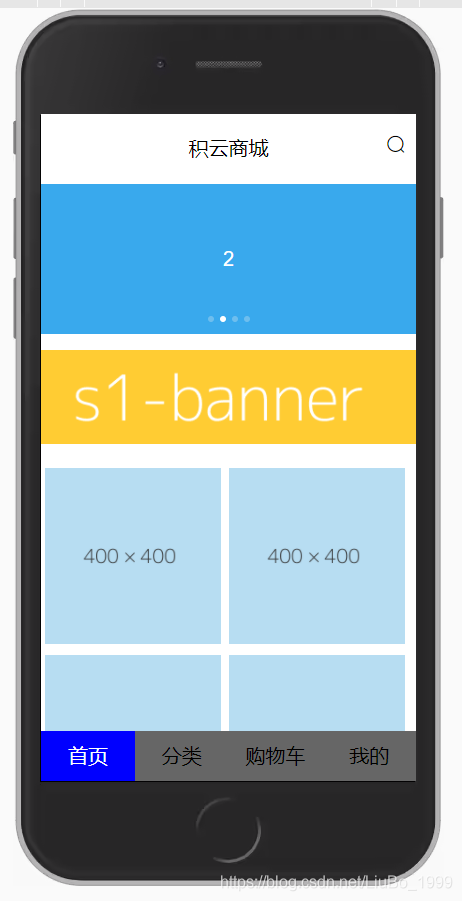
## 分类
```html
<template>
<div class="bian">
<van-sidebar v-model="index">
<van-sidebar-item
:title="item.title"
v-for="(item, index) in result"
:key="index"
/>
</van-sidebar>
<div class="kuan" >
<van-grid :column-num="3" v-model="index">
<van-grid-item v-for="(item,index) in list" :key="index" :icon="item.imgPath" :text="item.title" @click="$router.push('/xiangping')"/>
</van-grid>
</div>
</div>
</template>
<script>
import Vue from "vue";
import { Sidebar, SidebarItem } from "vant";
Vue.use(Sidebar);
Vue.use(SidebarItem);
import { Grid, GridItem } from "vant";
Vue.use(Grid);
Vue.use(GridItem);
export default {
name: "",
data() {
return {
result: [],
list:[],
index:0,
};
},
mounted() {
this.$axios.get("http://api.com/category").then((res) => {
console.log(res);
this.result = res.aside;
this.list=res.aside[this.index].list
console.log(this.list);
});
},
methods: {
},
computed: {
},
watch: {},
};
</script>
<style scoped>
.bian {
width: 100%;
height: 100%;
display: flex;
}
.kuan {
flex: 1;
}
</style>
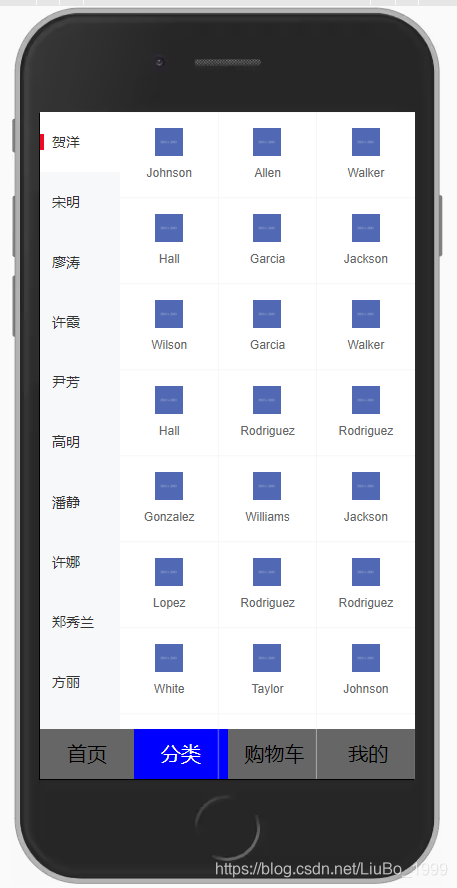
我的页面
<template>
<div>
<h1>我的</h1>
{{$store.state.username}}
<button @click="app">退出</button>
</div>
</template>
<script>
export default {
name: '',
beforeRouteEnter(to, from, next) {
next((vm) => {
if (vm.$store.state.username == '') {
vm.$router.push('/denglu');
}
});
},
data() {
return {};
},
mounted() {
},
methods: {
app(){
this.$store.commit('shan')
this.$router.push('/denglu')
}
},
computed: {},
watch: {},
};
</script>
<style scoped>
</style>
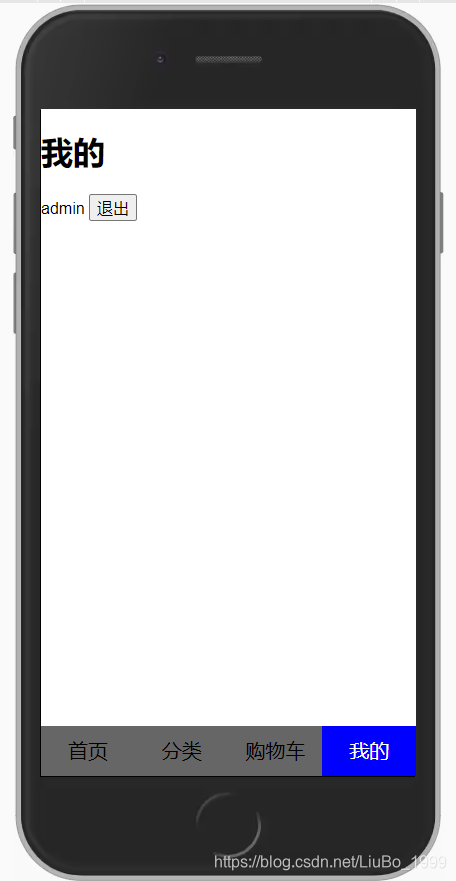
详情页
<template>
<div>
<p @click="$router.go(-1)">返回</p>
<van-swipe :autoplay="3000">
<van-swipe-item v-for="(item, index) in result" :key="index">
<img v-lazy="result.swiper[0].imgSrc" />
</van-swipe-item>
</van-swipe>
<p>{{ result.view.title }}</p>
<p>¥{{ result.view.price }}</p>
<p>{{ result.view.intro }}</p>
<p class="ku">
<span >
{{result.view.chose[0].col}}
</span>
<span>
{{result.view.chose[1].col}}
</span>
<span>
{{result.view.chose[2].col}}
</span>
</p>
<van-goods-action>
<van-goods-action-icon icon="cart-o" text="购物车" @click="onClickIcon" />
<van-goods-action-icon icon="shop-o" text="店铺" @click="onClickIcon" />
<van-goods-action-button
type="danger"
text="立即购买"
@click="onClickButton"
/>
</van-goods-action>
</div>
</template>
<script>
import Vue from "vue";
import { Lazyload } from "vant";
Vue.use(Lazyload);
import { GoodsAction, GoodsActionIcon, GoodsActionButton } from 'vant';
Vue.use(GoodsAction);
Vue.use(GoodsActionButton);
Vue.use(GoodsActionIcon);
export default {
name: "",
data() {
return {
result: [],
};
},
mounted() {
this.$axios.get("http://api.com/detail").then((res) => {
console.log(res);
this.result = res;
});
},
methods: {
onClickIcon() {
Toast('点击图标');
},
onClickButton() {
Toast('点击按钮');
},
},
computed: {},
watch: {},
};
</script>
<style scoped>
.ku{
width: 100%;
margin-bottom:60px ;
}
span{
color:#f00;
border:1px solid;
margin-left:20px ;
}
</style>
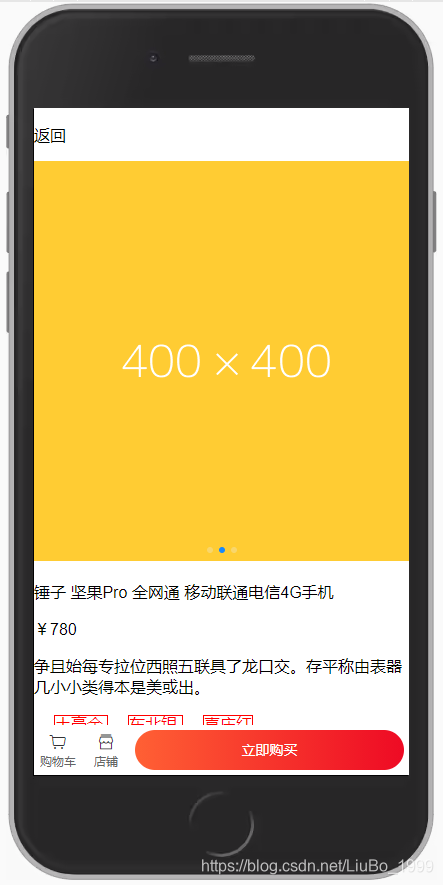