等额本金
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.util.HashMap;
import java.util.Map;
public class AverageCapitalUtils {
public static Map<Integer, BigDecimal> getPerMonthPrincipalInterest(double invest, double yearRate, int totalMonth) {
Map<Integer, BigDecimal> map = new HashMap<Integer, BigDecimal>();
BigDecimal monthPri = getPerMonthPrincipal(invest, totalMonth);
Map<Integer, BigDecimal> monthInterests = getPerMonthInterest(invest, yearRate, totalMonth);
for (Map.Entry<Integer, BigDecimal> entry : monthInterests.entrySet()) {
map.put(entry.getKey(), entry.getValue().add(monthPri).setScale(2, RoundingMode.CEILING));
}
return map;
}
public static Map<Integer, BigDecimal> getPerMonthInterest(double invest, double yearRate, int totalMonth) {
Map<Integer, BigDecimal> inMap = new HashMap<Integer, BigDecimal>();
BigDecimal monthPrincipal = getPerMonthPrincipal(invest, totalMonth);
double monthRate = yearRate / 12;
BigDecimal monthRateBigDecimal = new BigDecimal(String.valueOf(monthRate));
for (int i = 1; i <= totalMonth; i++) {
BigDecimal investBigDecimal = new BigDecimal(String.valueOf(invest));
BigDecimal finishPrincipal = monthPrincipal.multiply(new BigDecimal((i - 1)));
BigDecimal unfinish = investBigDecimal.subtract(finishPrincipal);
BigDecimal monthInterest = unfinish.multiply(monthRateBigDecimal);
inMap.put(i, monthInterest);
}
return inMap;
}
public static BigDecimal getPerMonthPrincipal(double invest, int totalMonth) {
return new BigDecimal(invest).divide(new BigDecimal(totalMonth), 4, BigDecimal.ROUND_DOWN);
}
public static BigDecimal getInterestCount(double invest, double yearRate, int totalMonth) {
BigDecimal count = new BigDecimal(0);
Map<Integer, BigDecimal> mapInterest = getPerMonthInterest(invest, yearRate, totalMonth);
for (Map.Entry<Integer, BigDecimal> entry : mapInterest.entrySet()) {
count = count.add(entry.getValue());
}
return count;
}
public static void main(String[] args) {
double invest = 20000;
double yearRate = 0.15;
int month = 12;
BigDecimal principal = getPerMonthPrincipal(invest, month);
Map<Integer, BigDecimal> mapInterest = getPerMonthInterest(invest, yearRate, month);
Map<Integer, BigDecimal> getPerMonthPrincipalInterest = getPerMonthPrincipalInterest(invest, yearRate, month);
System.out.println("序号\t利息\t\t本金\t\t每月还款本息");
for (Map.Entry<Integer, BigDecimal> entry : mapInterest.entrySet()) {
System.out.println(entry.getKey() + "\t" + entry.getValue().setScale(2, BigDecimal.ROUND_HALF_UP) + "\t" + principal.setScale(2, RoundingMode.CEILING) + "\t" + getPerMonthPrincipalInterest.get(entry.getKey()));
}
BigDecimal count = getInterestCount(invest, yearRate, month);
System.out.println("等额本金---总利息:" + count.setScale(2, BigDecimal.ROUND_HALF_UP));
}
}
等额本金
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.Map;
public class AverageCapitalPlusInterestUtils {
public static double getPerMonthPrincipalInterest(double invest, double yearRate, int totalmonth) {
double monthRate = yearRate / 12;
BigDecimal monthIncome = new BigDecimal(invest)
.multiply(new BigDecimal(monthRate * Math.pow(1 + monthRate, totalmonth)))
.divide(new BigDecimal(Math.pow(1 + monthRate, totalmonth) - 1), 2, BigDecimal.ROUND_HALF_UP);
return monthIncome.doubleValue();
}
public static Map<Integer, BigDecimal> getPerMonthInterest(double invest, double yearRate, int totalmonth) {
Map<Integer, BigDecimal> map = new HashMap<Integer, BigDecimal>();
double monthRate = yearRate / 12;
BigDecimal monthInterest;
for (int i = 1; i < totalmonth + 1; i++) {
BigDecimal multiply = new BigDecimal(invest).multiply(new BigDecimal(monthRate));
BigDecimal sub = new BigDecimal(Math.pow(1 + monthRate, totalmonth)).subtract(new BigDecimal(Math.pow(1 + monthRate, i - 1)));
monthInterest = multiply.multiply(sub).divide(new BigDecimal(Math.pow(1 + monthRate, totalmonth) - 1), 6, BigDecimal.ROUND_HALF_UP);
monthInterest = monthInterest.setScale(2, BigDecimal.ROUND_HALF_UP);
map.put(i, monthInterest);
}
return map;
}
public static Map<Integer, BigDecimal> getPerMonthPrincipal(double invest, double yearRate, int totalmonth) {
double monthRate = yearRate / 12;
BigDecimal monthIncome = new BigDecimal(invest)
.multiply(new BigDecimal(monthRate * Math.pow(1 + monthRate, totalmonth)))
.divide(new BigDecimal(Math.pow(1 + monthRate, totalmonth) - 1), 2, BigDecimal.ROUND_HALF_UP);
Map<Integer, BigDecimal> mapInterest = getPerMonthInterest(invest, yearRate, totalmonth);
Map<Integer, BigDecimal> mapPrincipal = new HashMap<Integer, BigDecimal>();
for (Map.Entry<Integer, BigDecimal> entry : mapInterest.entrySet()) {
mapPrincipal.put(entry.getKey(), monthIncome.subtract(entry.getValue()));
}
return mapPrincipal;
}
public static double getInterestCount(double invest, double yearRate, int totalmonth) {
BigDecimal count = new BigDecimal(0);
Map<Integer, BigDecimal> mapInterest = getPerMonthInterest(invest, yearRate, totalmonth);
for (Map.Entry<Integer, BigDecimal> entry : mapInterest.entrySet()) {
count = count.add(entry.getValue());
}
return count.doubleValue();
}
public static double getPrincipalInterestCount(double invest, double yearRate, int totalmonth) {
double monthRate = yearRate / 12;
BigDecimal perMonthInterest = new BigDecimal(invest)
.multiply(new BigDecimal(monthRate * Math.pow(1 + monthRate, totalmonth)))
.divide(new BigDecimal(Math.pow(1 + monthRate, totalmonth) - 1), 2, BigDecimal.ROUND_HALF_UP);
BigDecimal count = perMonthInterest.multiply(new BigDecimal(totalmonth));
count = count.setScale(2, BigDecimal.ROUND_HALF_UP);
return count.doubleValue();
}
public static void main(String[] args) {
double invest = 20000;
double yearRate = 0.15;
int month = 12;
double perMonthPrincipalInterest = getPerMonthPrincipalInterest(invest, yearRate, month);
Map<Integer, BigDecimal> mapInterest = getPerMonthInterest(invest, yearRate, month);
Map<Integer, BigDecimal> mapPrincipal = getPerMonthPrincipal(invest, yearRate, month);
System.out.println("序号\t利息\t\t本金\t\t每月还款本息");
for (Map.Entry<Integer, BigDecimal> entry : mapInterest.entrySet()) {
System.out.println(entry.getKey() + "\t" + entry.getValue() + "\t" + mapPrincipal.get(entry.getKey()) + "\t" + perMonthPrincipalInterest);
}
double count = getInterestCount(invest, yearRate, month);
System.out.println("等额本息---总利息:" + count);
double principalInterestCount = getPrincipalInterestCount(invest, yearRate, month);
System.out.println("等额本息---应还本息总和:" + principalInterestCount);
}
}
问题反馈
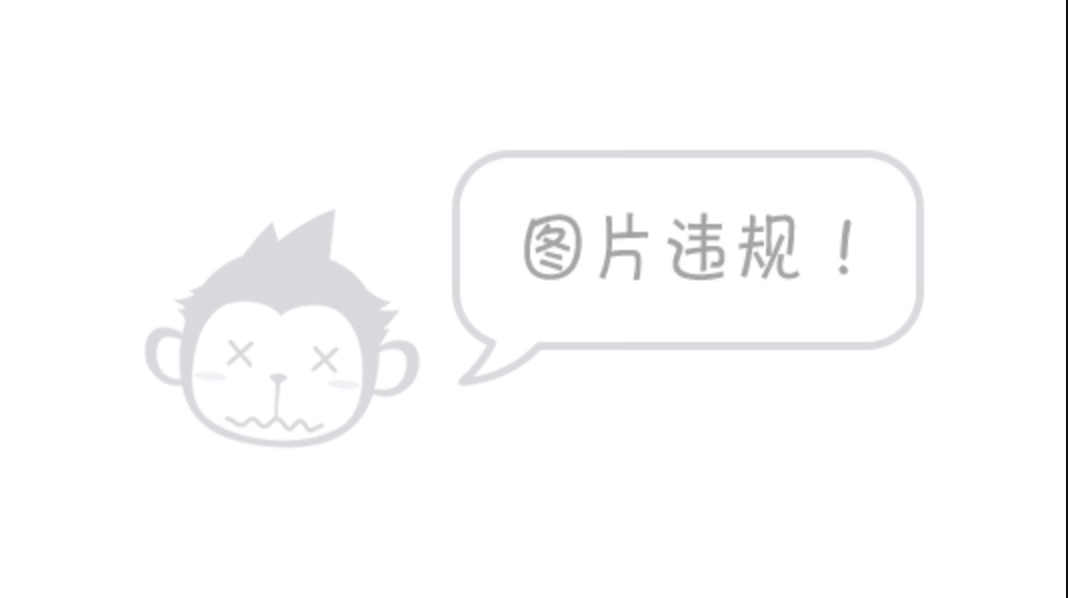