一、vite2创建项目
兼容性注意:Vite 需要 Node.js 版本 >= 12.0.0。
在创建项目文件目录下打开 cmd 运行以下命令
# npm 6.x
npm init @vitejs/app projectName --template
# npm 7+, 需要额外的双横线:
npm init @vitejs/app projectName -- --template
# yarn
yarn create @vitejs/app projectName --template
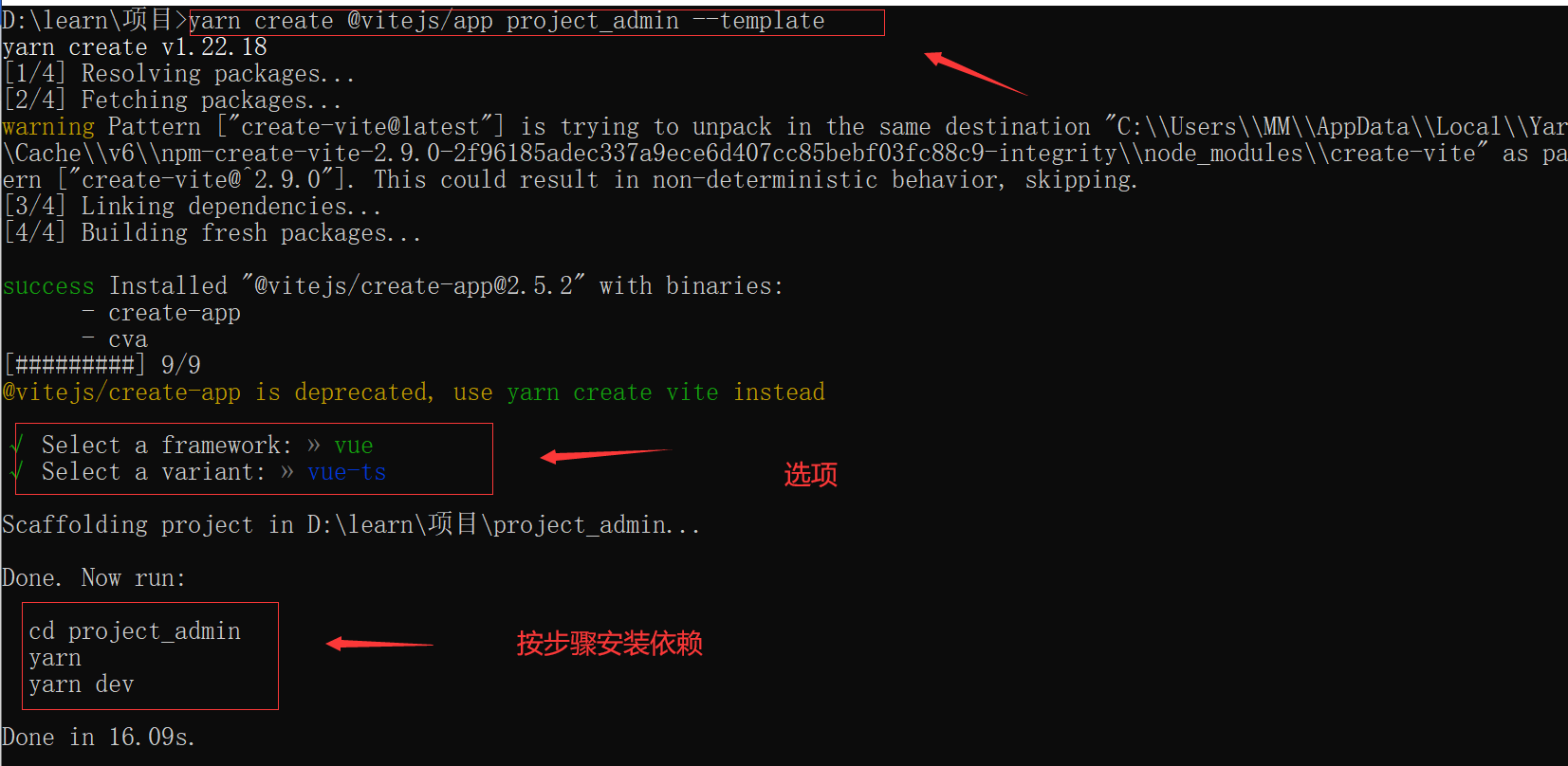
二、安装sass插件
//yarn
yarn add sass --dev
//npm
npm i sass -D
就直接使用了,不用像webpack 还要安装sass-loader
三、安装路由
//npm
npm install vue-router
//yarn
yarn add vue-router
- 在 src 文件下新增 router 文件夹 => index.ts 文件
import {
createRouter, createWebHistory, RouteRecordRaw } from 'vue-router'
const routes: RouteRecordRaw[] = [
{
path: '/',
name: 'Login',
component: () => import('xxxx'), // 注意这里要带上 文件后缀.vue
},
]
const router = createRouter({
history: createWebHistory(),
routes,
})
export default router
- mian.ts 引入
import {
createApp } from 'vue'
import App from './App.vue'
import router from './router/index'
const app = createApp(App)
//链式
app.use(router).mount('#app')
四、安装axios
yarn add axios
npm i axios -S
在 src 文件下新增 utils 文件夹,service.ts 和 request.ts
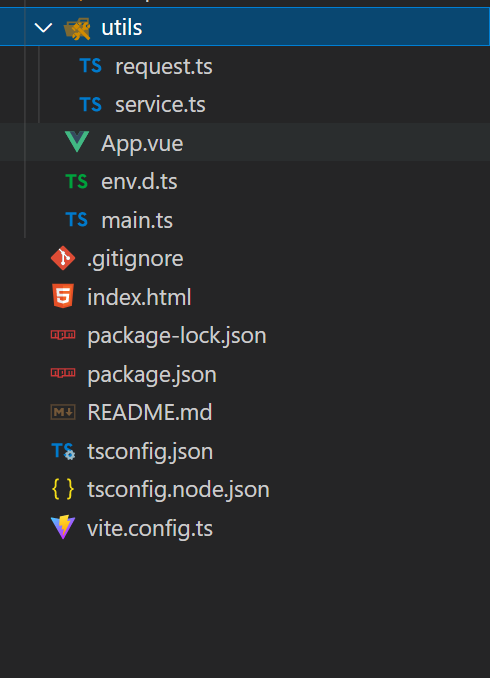
- service.ts:用与创建axios实例,统一配置请求拦截器、响应拦截…
/**
* axios 请求配置
*/
import axios, {
AxiosRequestConfig, AxiosResponse } from 'axios'
import {
ElNotification } from 'element-plus'
/**
* 请求失败后的错误统一处理
* @param status 请求失败的状态码
*/
const errorHandle = (status: number) => {
// 状态码判断
switch (status) {
case 302: ElNotification.error('接口重定向了!');
break;
case 400:
ElNotification.error("发出的请求有错误,服务器没有进行新建或修改数据的操作==>" + status)
break;
// 401: 未登录
// 未登录则跳转登录页面,并携带当前页面的路径
// 在登录成功后返回当前页面,这一步需要在登录页操作。
case 401: //重定向
ElNotification.error("token:登录失效==>" + status)
break;
// 403 token过期
// 清除token并跳转登录页
case 403:
ElNotification.error("登录过期,用户得到授权,但是访问是被禁止的==>" + status)
break;
case 404:
ElNotification.error("网络请求不存在==>" + status)
break;
case 406:
ElNotification.error("请求的格式不可得==>" + status)
break;
case 408: ElNotification.error(" 请求超时!")
break;
case 410:
ElNotification.error("请求的资源被永久删除,且不会再得到的==>" + status)
break;
case 422:
ElNotification.error("当创建一个对象时,发生一个验证错误==>" + status)
break;
case 500:
ElNotification.error("服务器发生错误,请检查服务器==>" + status)
break;
case 502:
ElNotification.error("网关错误==>" + status)
break;
case 503:
ElNotification.error("服务不可用,服务器暂时过载或维护==>" + status)
break;
case 504:
ElNotification.error("网关超时==>" + status)
break;
default:
ElNotification.error("其他错误错误==>" + status)
}
}
//axios创建实例
const service = axios.create({
//默认地址
baseURL: "",
// baseURL: "",
//请求超时时间
timeout: 3000,
//配置请求头
headers: