字节流:
特点:能读写文本、图片、视频、音频等
主要字节流:
FileOutputStream:文件输出流
FileInputStream:文件输入流
案例:
需求:复制文件
public static void fun() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
fis = new FileInputStream("/Users/lanou/Desktop/Test/znb.txt");
fos = new FileOutputStream("/Users/lanou/Desktop/Test/haha.txt");
int len = 0;
byte[] b = new byte[1024];
while((len = fis.read(b)) != -1 ) {
fos.write(b, 0, len);
}
} catch (FileNotFoundException e) {
throw new RuntimeException("文件找不到");
} catch (IOException e) {
throw new RuntimeException("文件复制失败");
} finally {
try {
if(fis = null){
fis.close();
}
} catch (IOException e) {
throw new RuntimeException("资源关闭失败");
} finally {
try {
if(fos = null){
fos.close();
}
} catch (IOException e) {
throw new RuntimeException("资源关闭失败");
}
}
}
}
需求:复制文件夹
public static void copyDir(File src, File dest) throws IOException {
File newDir = new File(dest, src.getName());
newDir.mkdir();
File[] listFiles = src.listFile();
for(File subFile : listFiles) {
if (subFile.isFile()) {
FileInputStream fis = new FileInputStream(subFile);
File tempFile = new File(newDir, subFile.getName());
FileOutputStream fos = new FileOutputStream(tempFile);
int len = 0;
byte[] b = new byte[1024];
while((len = fis.read(b)) != -1) {
fos.write(b, 0, len);
}
fis.close();
fos.close();
} else {
copyDir(subFile, newDir);
}
}
}
public static void main(String[] args) throws IOException {
File src = new File("/Users/lanou/Desktop/Test");
File dest = new File("/Users/lanou/Desktop/XTest");
copyDir(src, dest);
}
需求:将指定文件下所有txt文件复制到另一个文件夹下
class MyFilerByTxt implements FileFiler {
public boolean accept(File pathname) {
if (pathname.isDirectory()) {
return ture;
}
return pathname.getName().endsWith("txt");
}
}
public static void copyDirTxtFile(File src, File dest) throws IOException {
File[] listFiles = src.listFile(new MyFilerByTxt())
for (File subFile : listFiles) {
if (subFile.isFile()) {
FileInputStream fis = new FileInputStream(subFile);
File tempFile = new File(dest, subFile.getName());
FileOutputStream fos = new FileOutputStream(tempFile);
int len = 0;
byte[] b = new byte[1024];
while((len = fis.read(b)) != -1) {
fos.write(b, 0, len);
}
fis.close();
fos.close();
} else {
copyDirTxtFile(subFile, dest);
}
}
}
public static void main(String[] args) throws IOException {
File src = new File("/Users/lanou/Desktop/Test");
File dest = new File("/Users/lanou/Desktop/XTest");
copyDirTxtFile(src, dest);
}
字符流:
字符输出流(Writer):
是抽象类,是所有字符输出流的父类
注意:只能对文本文件进行操作
mac系统默认使用UTF-8的编码表(通用编码表),一个字符占3个字节。
Windows系统默认使用的GBK的编码表(简体中文),一个字符占2字节。
主要字符输出流:FileWriter
注意:字符输出流,写入文件时,要调用刷新方法。
字符输入流(Reader):
是抽象类,是所有字符输出流的父类
主要字符输入流:FileReader
注意:字符输入时, 不能字符串输入。
因为字符串很难界定,难以判断
转换流:
转换流的运行过程:
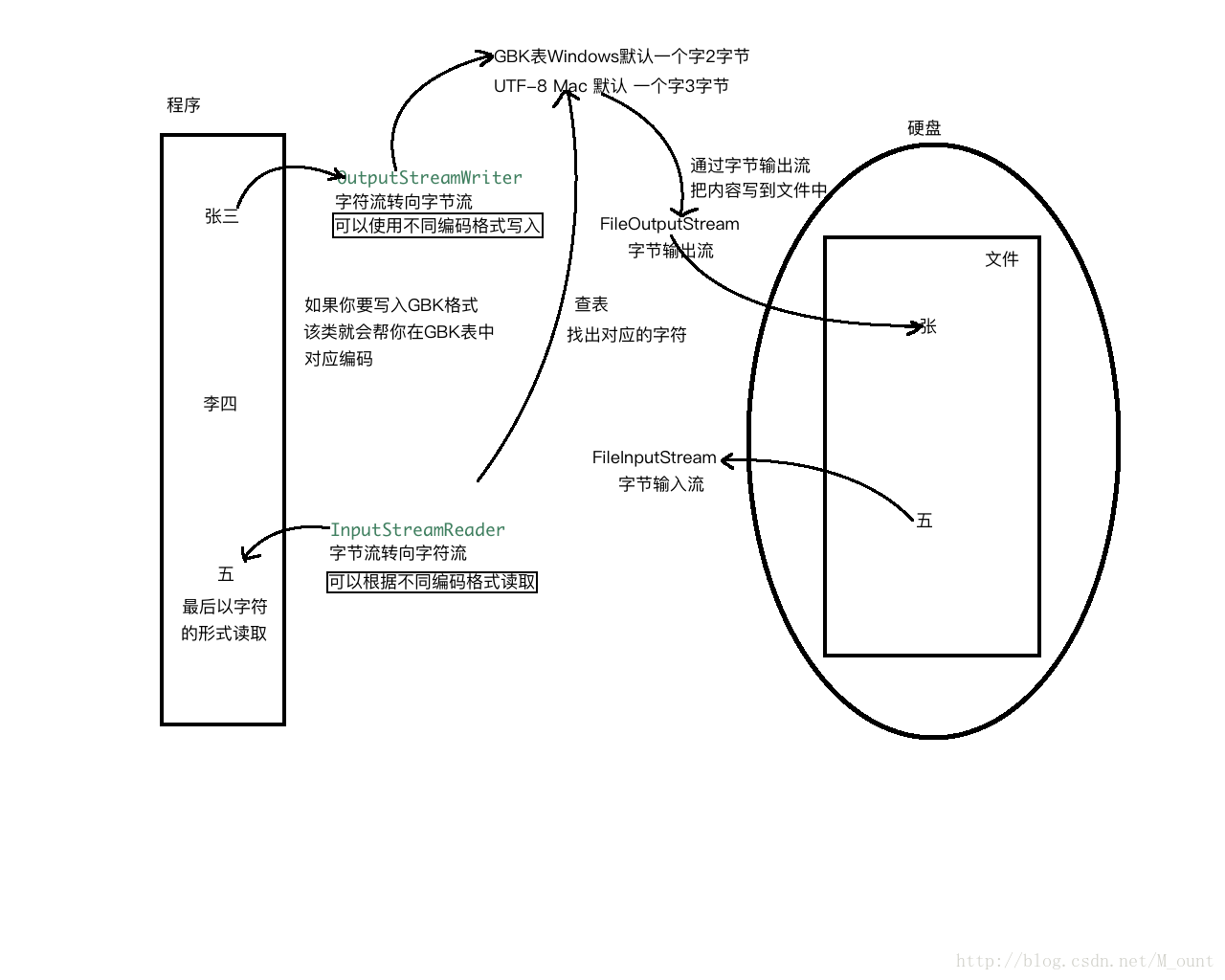
结论:
OutputStreamWriter(字符流转向字节流):
作用:根据不同编码格式写入
需要使用FileOutputStream类
作用:读取不同编码格式的文件
需要使用FileInputStream类
案例:
public static void getUTF8() throws IOException {
FileOutputStream fos = new FileOutputStream("/Users/lanou/Desktop/Test/utf8.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos);
osw.write("张三");
osw.close();
}
public static void readerUTF8() throws IOException {
FileInputStream fis = new FileInputStream("/Users/lanou/Desktop/Test/utf8.txt");
InputStreamReader isr = new InputStreamReader(fis);
int len = 0;
char[] c = new char[1024];
while ((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}
public static void getGBK() throws IOException {
FileOutputStream fos = new FileOutputStream("/Users/lanou/Desktop/Test/gbk.txt");
OutputStreamWriter osw = new OutputStreamWriter(fos, "GBK");
osw.write("张三");
osw.close();
}
public static void readerGBK() throws IOException {
FileInputStream fis = new FileInputStream("/Users/lanou/Desktop/Test/gbk.txt");
InputStreamReader isr = new InputStreamReader(fis, "GBK");
int len = 0;
char[] c = new char[1024];
while ((len = isr.read(c)) != -1) {
System.out.println(new String(c, 0, len));
}
isr.close();
}