零、实验环境
Spring3.0+Hibernate3.3+Struct2.0+MySQL5.5+Tomcat6.0,在搭建好SSH框架以后再导入一个jar包:struts2-spring-plugin-2.0.11.2,下图是搭建好的目录。
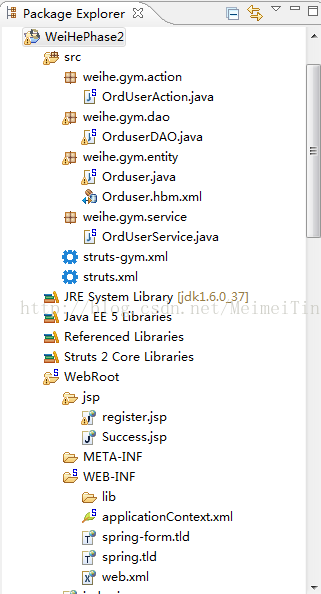
一、相关文件
接下来将从xml到java到jsp页面,粘贴文件!
1)web.xml中添加
<!-- 指定需加载的spring配置文件,注意最好写该文件的绝对路径 -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>
/WEB-INF/applicationContext.xml
</param-value>
</context-param>
2)applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans
xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-2.5.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-2.5.xsd">
<bean id="dataSource"
class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName"
value="com.mysql.jdbc.Driver">
</property>
<property name="url"
value="jdbc:mysql://localhost:3307/weihedatabase">
</property>
<property name="username" value="root"></property>
<property name="password" value="123456"></property>
</bean>
<bean id="sessionFactory"
class="org.springframework.orm.hibernate3.LocalSessionFactoryBean">
<property name="dataSource">
<ref bean="dataSource" />
</property>
<property name="hibernateProperties">
<props>
<prop key="hibernate.dialect">
org.hibernate.dialect.MySQLDialect
</prop>
</props>
</property>
<property name="mappingResources">
<list>
<value>weihe/gym/entity/Orduser.hbm.xml</value></list>
</property></bean>
<!-- 定义事务管理器(声明式的事务) -->
<bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager">
<property name="sessionFactory" ref="sessionFactory" />
</bean>
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<tx:attributes>
<tx:method name="*" propagation="REQUIRED" />
</tx:attributes>
</tx:advice>
<aop:config>
<aop:pointcut id="interceptorPointCuts"
expression="execution(* service.*.*(..))" />
<aop:advisor advice-ref="txAdvice"
pointcut-ref="interceptorPointCuts" />
</aop:config>
<!--给实体类ordUser换一个名字-->
<bean id="Orduser" class="weihe.gym.entity.Orduser"></bean>
<!--把DAO注入session工厂-->
<bean id="OrduserDAO" class="weihe.gym.dao.OrduserDAO">
<property name="sessionFactory">
<ref bean="sessionFactory" />
</property>
</bean>
<!--把service注入给DAO-->
<bean id="OrduserService" class="weihe.gym.service.OrdUserService">
<property name="dao" ref="OrduserDAO"> </property>
</bean>
<!--把Action注入给service-->
<bean id="OrduserAction" class="weihe.gym.action.OrdUserAction">
<!--注意property name 一定要和相应类中的变量名字一样才行 -->
<property name="user" ref="Orduser"></property>
<property name="service" ref="OrduserService"></property>
</bean>
</beans>
3)struts.xml主要添加下面代码
<include file="struts-gym.xml"/>
<!-- 一定要用这一项添加名为sturts.objectiveFactory的常量,把值设为spring,表示该Action由spring产生 -->
<constant name="struts.objectFactory" value="spring" />
<!--当是开发阶段时为true,当上线后可设为false -->
<constant name="struts.devMode" value="true" />
4)struts-gym.xml主要添加下面代码
<package name="gym" extends="struts-default">
<!--注意此处class最好和相应applicationContext文件中相应的bean id一致 -->
<action name="login" class="OrduserAction" >
<result name="success"> Success.jsp </result>
<result name="error"> error.jsp </result>
</action>
</package>
5)OrdUserAction.java
package weihe.gym.action;
import weihe.gym.entity.Orduser;
import weihe.gym.service.OrdUserService;
import com.opensymphony.xwork2.ActionSupport;
public class OrdUserAction extends ActionSupport {
private static final long serialVersionUID = 1L;
private Orduser user;
// private UserService service = new UserService();
private OrdUserService service;
public Orduser getUser() {
return user;
}
public void setUser(Orduser user) {
this.user = user;
}
public OrdUserService getService() {
return service;
}
public void setService(OrdUserService service) {
this.service = service;
}
public String execute(){
System.out.println("Begin to save in the action.");
System.out.println("In the userAction, "+"UserName:"+user.getUserName()+"Password: "+user.getPassword());
// System.out.println("The return value is "+service.save(user).getAdmId());
if (service.save(user)!=null) {
return SUCCESS;
}else {
return ERROR;
}
}
}
6)OrdUserService.java
package weihe.gym.service;
import weihe.gym.dao.OrduserDAO;
import weihe.gym.entity.Orduser;
public class OrdUserService {
private OrduserDAO dao;
public OrduserDAO getDao() {
return dao;
}
public void setDao(OrduserDAO dao) {
this.dao = dao;
}
public Orduser save(Orduser user){
System.out.println("Begin to save in the service.");
System.out.println("In the userAction, "+"UserName:"+user.getUserName()+"Password: "+user.getPassword());
this.dao.save(user);
System.out.println("In this process, it indicates that saving successfully. ");
return user;
}
}
7)Orduser.java和OrduserDAO.java和Orduser.hbm.xml是由hibernate反转生成的,参考下图!
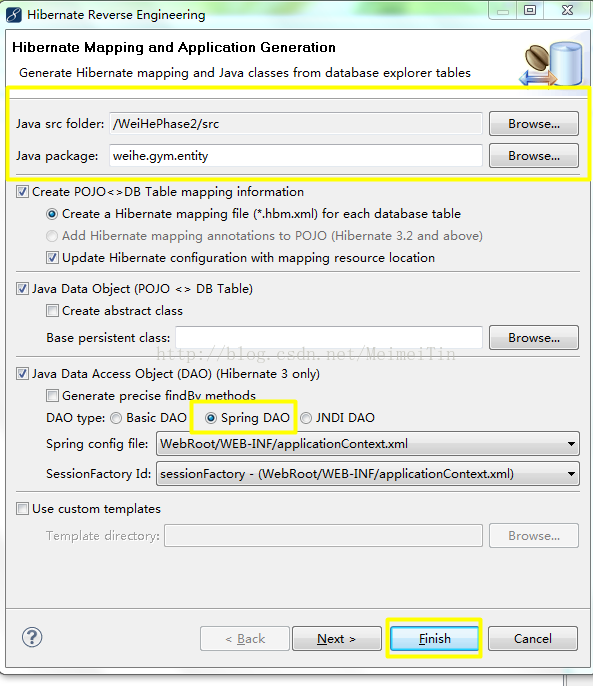
二、可能报错
1)不能加载相关配置,其实是spring和struts2没有关联起来,导入struts2-spring-plugin-2.0.11.2即可!
严重: Exception starting filter struts2
Unable to load configuration. - action - file:/D:/apache-tomcat-6.0.16/webapps/WeiHePhase2/WEB-INF/classes/struts-gym.xml:6:47
at org.apache.struts2.dispatcher.Dispatcher.init(Dispatcher.java:431)
at org.apache.struts2.dispatcher.ng.InitOperations.initDispatcher(InitOperations.java:69)
at org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter.init(StrutsPrepareAndExecuteFilter.java:51)
at org.apache.catalina.core.ApplicationFilterConfig.getFilter(ApplicationFilterConfig.java:275)
...
Caused by: Unable to load configuration. - action - file:/D:/apache-tomcat-6.0.16/webapps/WeiHePhase2/WEB-INF/classes/struts-gym.xml:6:47
at com.opensymphony.xwork2.config.ConfigurationManager.getConfiguration(ConfigurationManager.java:58)
at org.apache.struts2.dispatcher.Dispatcher.init_PreloadConfiguration(Dispatcher.java:374)
at org.apache.struts2.dispatcher.Dispatcher.init(Dispatcher.java:418)
... 29 more
Caused by: Action class [OrduserAction] not found - action - file:/D:/apache-tomcat-6.0.16/webapps/WeiHePhase2/WEB-INF/classes/struts-gym.xml:6:47
at com.opensymphony.xwork2.config.providers.XmlConfigurationProvider.verifyAction(XmlConfigurationProvider.java:409)
... 31 more
2013-12-13 14:38:35 org.apache.catalina.core.StandardContext start
严重: Error filterStart
2013-12-13 14:38:35 org.apache.catalina.core.StandardContext start
严重: Context [/WeiHePhase2] startup failed due to previous errors
2013-12-13 14:38:35 org.apache.catalina.core.ApplicationContext log
信息: Closing Spring root WebApplicationContext
2)空指针,这个问题可能是spring注入不成功导致的。可以参考的某些解决方法http://bbs.csdn.net/topics/390440780?page=1#post-394338828。这个链接也可以研读:http://www.tc5u.com/java/1555401.htm。
Exception report
message
description The server encountered an internal error () that prevented it from fulfilling this request.
exception
java.lang.NullPointerException
weihe.gym.action.OrdUserAction.execute(OrdUserAction.java:43)
sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39)
sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25)
...
3)主要是针对数据库中自增型的变量会报错,具体参考链接:http://database.51cto.com/art/201010/229076.htm。
SQLGrammarException: could not fetch initial value for increment generator...
4)可能的包冲突,我在第一次整合时报了这个错,参考链接:http://hi.baidu.com/29163077/item/dc890b110b3edfa6feded5a1解决问题。但第二次就没有出这个错了。
java.lang.NoClassDefFoundError: org/objectweb/asm/Type
5)在定义事务管理器(声明式的事务) 即编写applicationContext.xml文件时,由于用到了一些不认识的标签导致文件出错应该引入一些链接即可。头部改成下面的即可!
<beans
xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-2.5.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-2.5.xsd">
三、补充阅读
1)SSH整合常见错误:http://wenku.baidu.com/view/90cd3cd87f1922791688e8dd.html,还有这篇链接:http://g4-gc.iteye.com/blog/1000826。不过我还是建议遇到问题直接google!
2)这篇文章将配置文件讲得很细:http://blog.csdn.net/congcongsuiyue/article/details/17040265。
3)spring依赖注入解释文章:http://blog.csdn.net/chenssy/article/details/8171427以及http://blessht.iteye.com/blog/1162131。
To be continued!