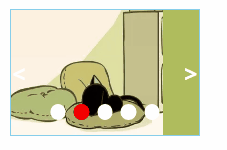
css样式设置
<style>
* {
margin: 0;
padding: 0;
}
ul,
ol,
li {
list-style: none;
}
.banner {
width: 500px;
height: 400px;
margin: 50px auto;
border: 2px solid red;
position: relative;
overflow: hidden;
}
img {
width: 100%;
height: 100%;
display: block;
}
.banner ul {
width: 400%;
position: absolute;
left: 0;
top: 0;
}
.banner ul li {
width: 500px;
height: 400px;
float: left;
}
.banner ol {
width: 300px;
height: 50px;
position: absolute;
bottom: 50px;
left: calc(50% - 150px);
display: flex;
justify-content: space-evenly;
}
.banner ol li {
width: 50px;
height: 50px;
border-radius: 50%;
background: #fff;
cursor: pointer;
}
.banner ol li.active {
background: red;
}
.banner div {
width: 100%;
height: 50px;
position: absolute;
left: 0;
top: 50%;
transform: translateY(-50%);
display: flex;
justify-content: space-between;
}
a,
a:hover,
a:active {
text-decoration: none;
}
.banner div a {
width: 50px;
height: 50px;
display: flex;
justify-content: center;
align-items: center;
color: #fff;
font-size: 50px;
}
</style>
JavaScript部分
<script src="/JS/move.js"></script>
<script>
const imgArr = [
{ id: 1, width: 456, height: 123, size: 111, type: 'jpg', src: 'https://img1.baidu.com/it/u=1067186258,453323346&fm=253&fmt=auto&app=138&f=JPG?w=400&h=222' },
{ id: 2, width: 457, height: 124, size: 111, type: 'jpg', src: 'https://img2.baidu.com/it/u=3476703181,570097117&fm=253&fmt=auto&app=138&f=JPEG?w=700&h=391' },
{ id: 3, width: 458, height: 125, size: 111, type: 'jpg', src: 'https://img2.baidu.com/it/u=1955807347,1850513573&fm=253&fmt=auto&app=138&f=JPEG?w=800&h=500' },
{ id: 4, width: 459, height: 126, size: 111, type: 'jpg', src: 'https://img2.baidu.com/it/u=994551759,2082548961&fm=253&fmt=auto&app=120&f=JPEG?w=1422&h=800' },
]
const oBanner = document.querySelector('.banner');
let oUl;
let oOl;
let oDiv;
let oOlLis;
let oUlLiWidth;
let length = imgArr.length + 2;//新的li个数,不然图会挤下去
let time;
let index = 1;//索引下标初始值是1,第一张原始图
let flag = true;//开关变量
setPage();
autoLoop();
setMouse();
setClick();
hide();
//动态生成页面
function setPage() {
oUl = document.createElement('ul');
oOl = document.createElement('ol');
oDiv = document.createElement('div');
let oUlLiStr = '';
let oOlLiStr = '';
imgArr.forEach((item, index) => {
oUlLiStr += `<li><img src="${item.src}"></li>`;
oOlLiStr += index === 0 ? `<li class="active" num="${index}"></li>` : `<li num="${index}"></li>`;
})
oUl.innerHTML = oUlLiStr;
oOl.innerHTML = oOlLiStr;
oDiv.innerHTML = '<a href="JavaScript:;"><</a><a href="JavaScript:;">></a>';
oBanner.appendChild(oUl);
oBanner.appendChild(oOl);
oBanner.appendChild(oDiv);
const oUlLiFirst = oUl.querySelector('li:first-child');
const oUlLiFirstClone = oUlLiFirst.cloneNode(true);
const oUlLiLast = oUl.querySelector('li:last-child');
const oUlLiLastClone = oUlLiLast.cloneNode(true);
oUl.appendChild(oUlLiFirstClone);
oUl.insertBefore(oUlLiLastClone, oUlLiFirst);
oUlLiWidth = oUlLiFirst.offsetWidth;
oUl.style.width = length * oUlLiWidth + 'px';
oUl.style.left = -oUlLiWidth + 'px';
oOlLis = oOl.querySelectorAll('li');
}
//自动轮播
function autoLoop() {
time = setInterval(() => {
index++;
setFocusStyle();//调用焦点按钮样式切换
move(oUl, { left: -index * oUlLiWidth }, loopEnd);
}, 1500)
}
//运动结束 回调函数
function loopEnd() {
if (index === length - 1) {//往右
index = 1;
oUl.style.left = -oUlLiWidth + 'px';
} else if (index === 0) {//往左
index = length - 2;
oUl.style.left = -index * oUlLiWidth + 'px';
}
flag = true;
}
//焦点按钮
function setFocusStyle() {
oOlLis.forEach((item) => {
item.classList.remove('active');
})
if (index === length - 1) {
oOlLis[0].classList.add('active');
} else if (index === 0) {
oOlLis[oOlLis.length - 1].classList.add('active');
} else {
oOlLis[index - 1].classList.add('active');
}
}
//鼠标移入移出
function setMouse() {
oBanner.addEventListener('mouseenter', () => {
clearInterval(time);
})
oBanner.addEventListener('mouseleave', () => {
autoLoop();
})
}
//鼠标点击
function setClick() {
oBanner.addEventListener('click', (event) => {
if (event.target.innerHTML === '<') {
if (!flag) return;
flag = false;
index--;
setFocusStyle();
move(oUl, { left: -index * oUlLiWidth }, loopEnd);//调用运动函数,运动切换
} else if (event.target.innerHTML === '>') {
if (!flag) return;
flag = false;
index++;
setFocusStyle();
move(oUl, { left: -index * oUlLiWidth }, loopEnd);
} else if (event.target.tagName === 'LI') {
if (!flag) return;
flag = false;
index = Number(event.target.getAttribute('num')) + 1;
setFocusStyle();
move(oUl, { left: -index * oUlLiWidth }, loopEnd);
}
})
}
//浏览器后台运行
function hide() {
document.querySelector('visibilitychange', () => {
if (document.visibilityState === 'hidden') {
clearInterval(time);
} else if (document.visibilityState === 'visible') {
autoLoop();
}
})
}
</script>