基于spring mvc+mybatis的配置
1.引用jar包
如果你想使用本项目的jar包而不是直接引入类,你可以在这里下载各个版本的jar包(点击Download下的jar即可下载)
https://oss.sonatype.org/content/repositories/releases/com/github/pagehelper/pagehelper/
http://repo1.maven.org/maven2/com/github/pagehelper/pagehelper/
由于使用了sql解析工具,你还需要下载jsqlparser.jar(这个文件完全独立,不依赖其他):
http://repo1.maven.org/maven2/com/github/jsqlparser/jsqlparser/0.9.1/
http://git.oschina.net/free/Mybatis_PageHelper/attach_files
2. 在Mybatis配置xml中配置拦截器插件:
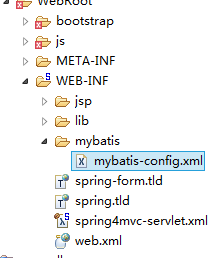
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<plugins>
<plugin interceptor="com.github.pagehelper.PageHelper">
<property name="dialect" value="mysql"/>
<property name="offsetAsPageNum" value="true"/>
<property name="rowBoundsWithCount" value="true"/>
<property name="pageSizeZero" value="true"/>
<property name="reasonable" value="false"/>
<property name="params" value="pageNum=start;pageSize=limit;"/>
<property name="returnPageInfo" value="check"/>
</plugin>
</plugins>
</configuration>
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
3.在applicationContext.xml中进行配置:
在这个bean中添加属性 :
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="configLocation" value="/WEB-INF/mybatis/mybatis-config.xml" />
也就是指定第二步文件的位置
4.Controller
@RequestMapping(value = "page")
@ResponseBody
public ModelAndView index(HttpServletRequest request, HttpServletResponse response) {
log.info("++++++++++++page+++++++++++++++++++++++++++++++++");
String pageNum = request.getParameter("pageNum");
String pageSize = request.getParameter("pageSize");
log.info("++++++++++++pageNum="+pageNum+"++++++++++++++++++++++++++++++");
log.info("++++++++++++pageSize="+pageSize+"++++++++++++++++++++++++++++++");
PageInfo<Data> page = service.queryByPage(pageNum, pageSize);
ModelAndView modelAndView = new ModelAndView();
modelAndView.addObject("pagehelper", page);
modelAndView.setViewName("data");
return modelAndView;
}
PageInfo: 是一个独有的封装类
5.Service
public PageInfo<Data> queryByPage(String pageNum, String pageSize) {
int num = 1;
int size = 3;
if (pageNum != null && !"".equals(pageNum)) {
num = Integer.parseInt(pageNum);
}
if (pageSize != null && !"".equals(pageSize)) {
size = Integer.parseInt(pageSize);
}
PageHelper.startPage(num, size);
List<Data> list = dao.select();
PageInfo<Data> page = new PageInfo<Data>(list);
return page;
}
PageHelper.startPage(num, size);就是核心方法。
num:第几页
size:每页的个数
需要注意的是:只有 紧跟着这个方法的查询会被分页
请求使用 :$(“body”).load(“page.html?pageNum=” + pageNum + “&pageSize=” + pageSize);
之后就没有特别的地方不做赘述。