在企业的信息系统中,报表处理一直占比较重要的作用,iText是一种生成PDF报表的Java组件。通过在服务器端使用Jsp或JavaBean生成PDF报表,客户端采用超链接显示或下载得到生成的报表,这样就很好的解决了B/S系统的报表处理问题,iText用于具有下列条件之一的项目:
内容不固定,它是基于用户输入或实时数据库信息计算。
由于页数多或者文件较大而造成的内容过多而使得PDF文件不能手动生成,。
文件需要在无人值守模式下创建的,使用批处理过程。
内容需要自定义或个性化;例如,最终用户的名字需要被印在某一页中。
1、引入jar包
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.11</version>
</dependency>
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itext-asian</artifactId>
<version>5.2.0</version>
</dependency>
<dependency>
<groupId>org.apache.pdfbox</groupId>
<artifactId>pdfbox</artifactId>
<version>2.0.12</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>RELEASE</version>
</dependency>
2、创建一个工具类PDFTempletTicket
package com.text.util;
import cn.hutool.core.util.IdUtil;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.pdf.AcroFields;
import com.itextpdf.text.pdf.BaseFont;
import com.itextpdf.text.pdf.PdfReader;
import com.itextpdf.text.pdf.PdfStamper;
import com.text.BizException;
import com.text.OssHandler;
import com.text.User;
import org.apache.http.entity.ContentType;
import org.springframework.mock.web.MockMultipartFile;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.ArrayList;
import java.util.Map;
public class PDFTempletTicket {
private String templatePdfPath;
private String ttcPath;
private String targetPdfpath;
private User user;
public PDFTempletTicket() {
super();
}
public PDFTempletTicket(String templatePdfPath, String ttcPath,
String targetPdfpath, Map<String, Object> map) {
this.templatePdfPath= templatePdfPath;
this.ttcPath= ttcPath;
this.targetPdfpath= targetPdfpath;
this.user= user;
}
/**
* 录取通知书 PDF渲染
* @Author admin
**/
public String admissionNotice(File file,User user) throws Exception {
PdfReader reader = new PdfReader(templatePdfPath);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
PdfStamper ps = new PdfStamper(reader, bos);
/*使用中文字体,可以更换成自己电脑或者服务器上已有的字体 */
BaseFont bf = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
/*BaseFont bf = BaseFont.createFont(PDFTicket.class.getResource("/") + "org/csun/ns/util/simsun.ttc,1", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);*/
ArrayList<BaseFont> fontList = new ArrayList<BaseFont>();
fontList.add(bf);
//初始化AcroFields并填入数据
AcroFields s = ps.getAcroFields();
s.setSubstitutionFonts(fontList);
s.setField("01",user.getName());
s.setField("02",user.getIdCard());
//是否只读
ps.setFormFlattening(true);
ps.close();
FileInputStream fileInputStream = new FileInputStream(file);
FileOutputStream fos = new FileOutputStream(file);
fos.write(bos.toByteArray());
fos.close();
bos.close();
//把File转成MultipartFile格式
MultipartFile multipartFile = new MockMultipartFile(file.getName(),file.getName(),ContentType.APPLICATION_OCTET_STREAM.toString(),fileInputStream);
//上传到oss并获取返货的oss存储链接
String ossUrl = this.uploadOss(multipartFile);
return ossUrl;
}
/**
* @return the templatePdfPath
*/
public String getTemplatePdfPath() {
return templatePdfPath;
}
/**
* @param templatePdfPath the templatePdfPathto set
*/
public void setTemplatePdfPath(String templatePdfPath) {
this.templatePdfPath= templatePdfPath;
}
/**
* @return the ttcPath
*/
public String getTtcPath() {
return ttcPath;
}
/**
* @param ttcPath the ttcPath to set
*/
public void setTtcPath(String ttcPath) {
this.ttcPath= ttcPath;
}
/**
* @return the targetPdfpath
*/
public String getTargetPdfpath() {
return targetPdfpath;
}
/**
* @param targetPdfpath the targetPdfpath toset
*/
public void setTargetPdfpath(String targetPdfpath) {
this.targetPdfpath= targetPdfpath;
}
/**
* @return the ticket
*/
public User getTicket() {
return user;
}
/**
* @param notice the ticket to set
*/
public void setTicket(User user) {
this.user= user;
}
public String uploadOss(MultipartFile file) throws Exception {
Long size = file.getSize();
if (size > 5*1024*1024){
throw new BizException("文件大小应小于5M");
}
String uploadFileName = file.getOriginalFilename();
assert uploadFileName != null;
String extendName = uploadFileName.substring(uploadFileName.lastIndexOf(".") + 1);
// 把文件加上随机数,防止文件重复
String uuid = IdUtil.randomUUID().replace("-", "").toLowerCase();
String fileName = uuid + "." + extendName;
// 把文件加上随机数,防止文件重复
file.getOriginalFilename();
OssHandler ossHandler = new OssHandler(OssHandler._OSS_ENDPOINT_OUT, OssHandler._OSS_USER_UPLOAD_BUCKET);
String url = ossHandler.putObject(file.getInputStream(), fileName, false);
return url;
}
}
3、创建一个测试类TestTempletTicket
package com.text.util;
import com.text.User;
import java.io.File;
public class TestTempletTicket {
//引用文件的临时存储空间
private static final String FILE_PATH_TEMPLATE = System.getProperty("java.io.tmpdir") + "/tempdf/%s";
public static void main(String[] args) throws Exception {
User user = new User();
user.setName("张三");
user.setIdCard("1234567876543234567");
PDFTempletTicket pdfTT = new PDFTempletTicket();
//本地PDF文件路径,如果用网络路径直接填入oss地址
pdfTT.setTemplatePdfPath("/Users/ysxs/Desktop/录取告知书.pdf");
pdfTT.setTargetPdfpath(FILE_PATH_TEMPLATE);
pdfTT.setTicket(notice);
//渲染的pdf文件临时存放路径
File file = new File("/Users/ysxs/Desktop/q12121w231231e232r.pdf");
file.createNewFile();
pdfTT.admissionNotice(file,user);
}
}
#### 4、下载Adobe Acrobat工具对pdf文件惊喜网格选中便于我们渲染,搞成这个吊样子就行了
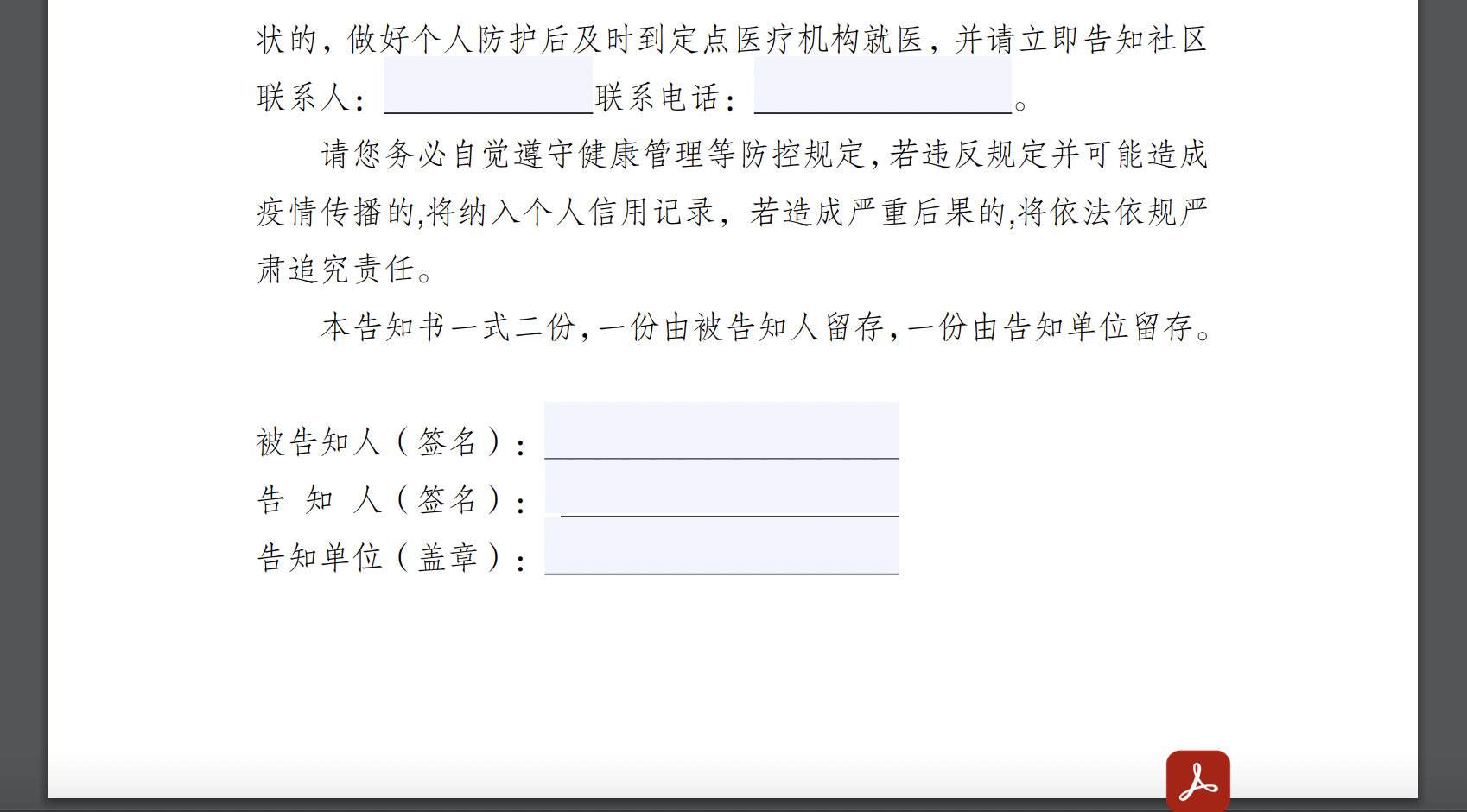