滑动轮播图
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<style>
body,
ul,
li {
margin: 0;
padding: 0;
}
#background {
width: 600px;
height: 400px;
margin: 100px auto;
position: relative;
border: 1px solid black;
overflow: hidden;
}
#pre {
width: 30px;
height: 100px;
left: 0;
top: 40%;
position: absolute;
opacity: 0.3;
transform: rotateZ(180deg);
}
#next {
width: 30px;
height: 100px;
right: 0;
top: 40%;
position: absolute;
opacity: 0.3;
}
#daizi {
width: 3000px;
height: 100%;
margin-left: 0px;
transition: all 1s linear;
}
#daizi img {
width: 600px;
height: 100%;
float: left;
}
ul {
width: 100px;
height: 20px;
bottom: 0;
left: 225px;
position: absolute;
}
ul li {
width: 15px;
height: 15px;
float: left;
border-radius: 50%;
margin-left: 10px;
background-color: white;
opacity: 0.4;
list-style-type: none;
}
</style>
<body>
<div id="background">
<div id="daizi">
<img id="img1" src="./img/1.jpg" alt="">
<img id="img2" src="./img/2.jpg" alt="">
<img id="img3" src="./img/3.jpg" alt="">
<img id="img4" src="./img/4.jpg" alt="">
<img id="img5" src="./img/1.jpg" alt="">
</div>
<ul>
<li id="id1"></li>
<li id="id2"></li>
<li id="id3"></li>
<li id="id4"></li>
</ul>
<img id="pre" src="./img/btn.jpg">
<img id="next" src="./img/btn.jpg">
</div>
</body>
<script>
var daizi = document.getElementById("daizi");
var backg = document.getElementById("background");
var pre = document.getElementById("pre");
var next = document.getElementById("next");
var btn = document.getElementsByTagName("li");
var img = 0;
var timer = null;
btn[img].style.backgroundColor = "red";
btn[img].style.opacity = 1;
timer = setInterval(function () {
tab1();
}, 2000)
function tab1() {
img++;
if (img > 4) {
img = 0;
btn[img].style.backgroundColor = "red";
btn[img].style.opacity = 1;
daizi.style.transition = "none";
daizi.style.marginLeft = -600 * img + "px";
setTimeout(tab1, 100);
} else {
daizi.style.transition = "all 1s linear";
daizi.style.marginLeft = -600 * img + "px";
for (var i = 0; i < 4; i++) {
btn[i].style.backgroundColor = "white";
btn[i].style.opacity = 0.4;
}
if (img == 4) {
btn[0].style.backgroundColor = "red";
btn[0].style.opacity = 1;
} else {
btn[img].style.backgroundColor = "red";
btn[img].style.opacity = 1;
}
}
}
function tab2() {
img--;
if (img < 0) {
img = 4;
daizi.style.transition = "none";
daizi.style.marginLeft = -600 * img + "px";
setTimeout(tab2, 100);
} else {
daizi.style.transition = "all 1s linear";
daizi.style.marginLeft = -600 * img + "px";
for (var i = 0; i < 4; i++) {
btn[i].style.backgroundColor = "white";
btn[i].style.opacity = 0.4;
}
if (img == 4) {
btn[0].style.backgroundColor = "red";
btn[0].style.opacity = 1;
} else {
btn[img].style.backgroundColor = "red";
btn[img].style.opacity = 1;
}
}
}
backg.onmouseenter = function () {
clearInterval(timer);
}
backg.onmouseleave = function () {
timer = setInterval(tab1, 2000)
}
pre.onclick = function () {
tab2();
}
next.onclick = function () {
tab1();
}
pre.onmouseenter = function () {
pre.style.opacity = "1";
}
pre.onmouseleave = function () {
pre.style.opacity = "0.3";
}
next.onmouseenter = function () {
next.style.opacity = "1";
}
next.onmouseleave = function () {
next.style.opacity = "0.3";
}
for (var i = 0; i < 4; i++) {
btn[i].onclick = function () {
img = this.id.substr(2, 1) - 2;
tab1();
}
}
</script>
</html>
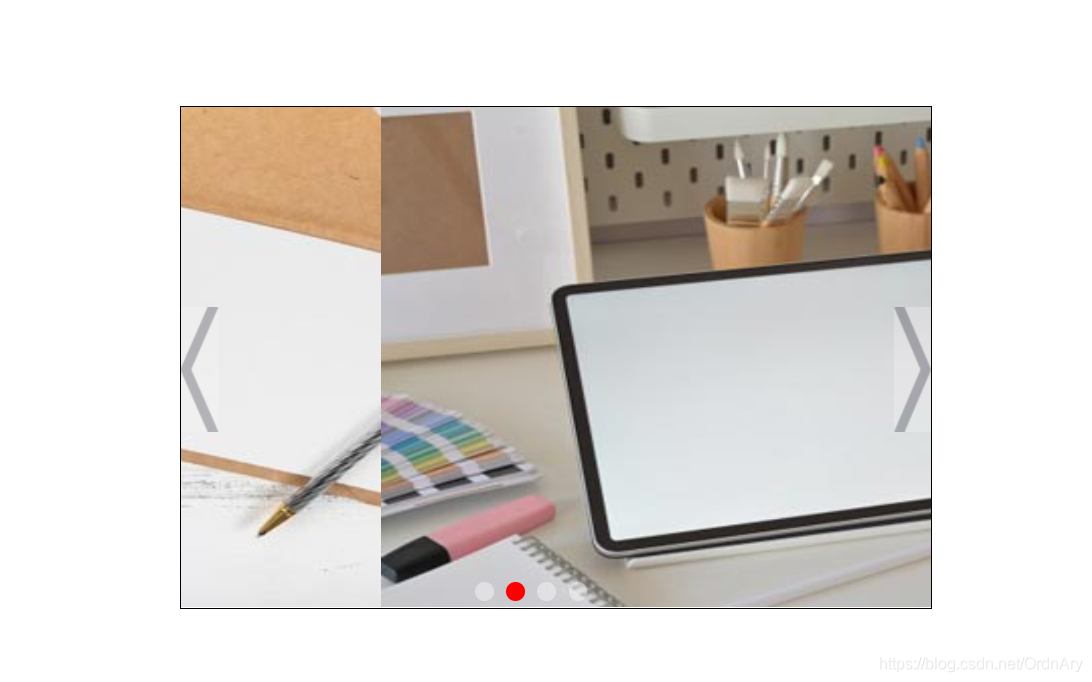
淡入染出轮播图
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<style>
#back {
width: 600px;
height: 400px;
margin: 100px auto;
position: relative;
border: 1px solid red;
}
#back #pre {
width: 30px;
height: 60px;
left: 0;
top: 170px;
z-index: 2;
position: absolute;
opacity: 0.3;
transform: rotateZ(180deg);
}
#back #next {
width: 30px;
height: 60px;
right: 0;
top: 170px;
z-index: 2;
opacity: 0.3;
position: absolute;
}
#daizi {
width: 100%;
height: 100%;
position: relative;
}
#daizi img {
width: 100%;
height: 100%;
position: absolute;
transition: all 1s linear;
}
#id1 {
z-index: 1;
}
#btn {
width: 120px;
height: 20px;
position: absolute;
left: 240px;
bottom: 0;
z-index: 2;
display: flex;
justify-content: space-between;
}
#btn span {
width: 20px;
height: 20px;
border-radius: 50%;
opacity: 0.3;
background-color: white;
}
</style>
<body>
<div id="back">
<div id="daizi">
<img src="./img/1.jpg" alt="" id="id1">
<img src="./img/2.jpg" alt="" id="id2">
<img src="./img/3.jpg" alt="" id="id3">
<img src="./img/4.jpg" alt="" id="id4">
</div>
<img src="./img/btn.jpg" id="pre"><img src="./img/btn.jpg" id="next">
<div id="btn">
<span></span>
<span></span>
<span></span>
<span></span>
</div>
</div>
</body>
<script>
var timer = null;
var img = document.getElementsByTagName("img");
var span = document.getElementsByTagName("span");
var num = 0;
span[0].style.opacity = 1;
span[0].style.backgroundColor = 'red';
timer = setInterval(function () {
num++;
tab();
}, 2000)
function tab() {
if (num >= 4) {
num = 0;
}
if (num < 0) {
num = 3
}
for (var i = 0; i < 4; i++) {
img[i].style.opacity = 0;
span[i].style.opacity = 0.3;
span[i].style.backgroundColor = 'white';
}
img[num].style.opacity = 1;
span[num].style.opacity = 1;
span[num].style.backgroundColor = 'red';
}
for (let i = 0; i < 4; i++) {
span[i].onclick = function () {
num = i;
tab();
console.log(i);
}
}
pre.onclick = function () {
setTimeout(function () {
num--;
tab();
}, 150)
}
next.onclick = function () {
setTimeout(function () {
num++;
tab();
}, 150)
}
back.onmouseenter = function () {
clearInterval(timer);
}
back.onmouseleave = function () {
timer = setInterval(function () {
num++;
tab();
}, 2000)
}
pre.onmouseenter = function () {
pre.style.opacity = '1';
}
pre.onmouseleave = function () {
pre.style.opacity = '0.3';
}
next.onmouseenter = function () {
next.style.opacity = '1';
}
next.onmouseleave = function () {
next.style.opacity = '0.3';
}
</script>
</html>
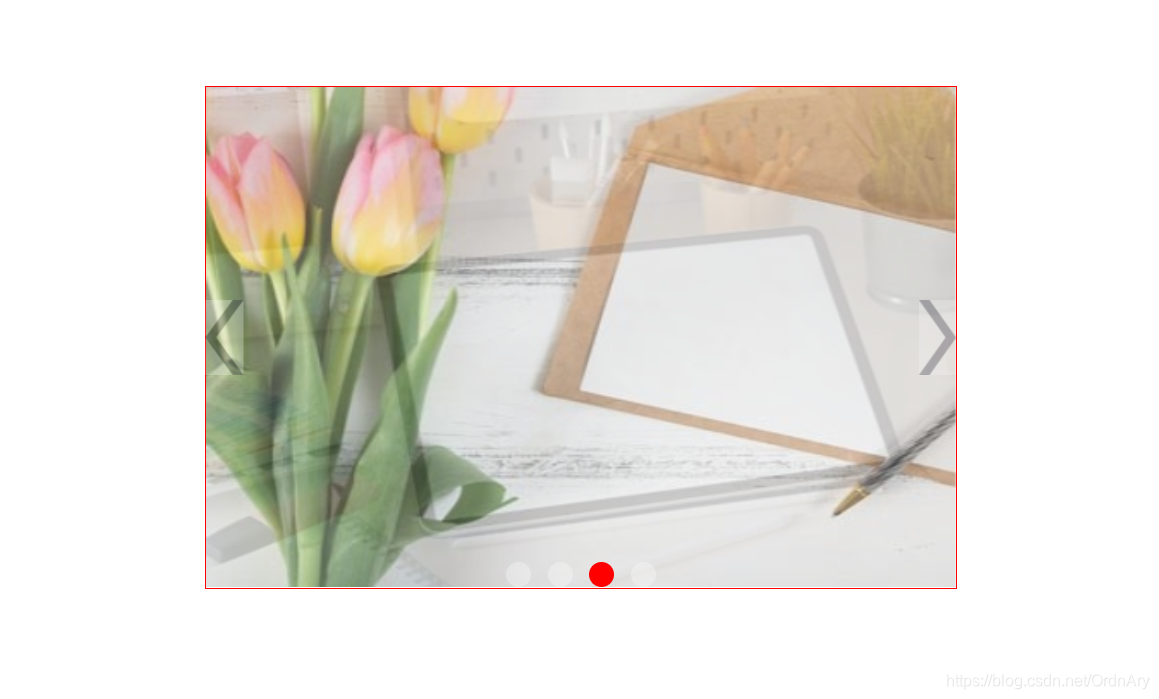