C++实现双链表的创建、插入、删除、查询、遍历、销毁等基本操作
1.双链表:每个节点(首尾节点除外)都包含指向后继和前驱指针,从某节点出发可向前(后)遍历。
2.代码
#include <iostream>
#include <malloc.h>
using namespace std;
typedef int ElementType;
typedef struct DNode{
ElementType data;
struct DNode *prior;
struct DNode *next;
} DNode, *DNodeList;
DNodeList Create(ElementType e);
bool Insert(DNodeList &L, int pos, ElementType e);
DNodeList Delete(DNodeList &L, int pos);
int SearchByData(DNodeList &L, ElementType e);
bool IsEmpty(DNodeList &L);
bool CheckPos(DNodeList &L, int pos);
int GetLen(DNodeList &L);
bool Destroy(DNodeList &L);
void Print(DNodeList &L);
DNodeList Create(ElementType e){
DNodeList ans = (DNodeList) malloc(sizeof(DNode));
if (ans->data == NULL) return NULL;
ans->data = e;
ans->prior = NULL;
ans->next = NULL;
return ans;
}
bool Insert(DNodeList &L, int pos, ElementType e){
if (!CheckPos(L, pos)) return false;
DNodeList n = Create(e);
if (n == NULL) return false;
DNodeList cur = L, prior;
while(cur != NULL && pos-- > 0){
prior = cur;
cur = cur->next;
}
if (cur != NULL){
cur->prior = n;
}
n->next = cur;
n->prior = prior;
prior->next = n;
return true;
}
DNodeList Delete(DNodeList &L, int pos){
if (!CheckPos(L, pos)) return NULL;
DNodeList cur = L, prior;
while(cur != NULL && pos-- > 0){
prior = cur;
cur = cur->next;
}
if (cur == NULL) return NULL;
if (cur->next != NULL){
cur->next->prior = prior;
}
prior->next = cur->next;
free(cur);
return cur;
}
int SearchByData(DNodeList &L, ElementType e){
DNodeList cur = L;
int pos = 1;
while(cur != NULL){
cur = cur->next;
if (cur->data == e) return pos;
pos++;
}
return -1;
}
bool IsEmpty(DNodeList &L){
return L->next == NULL;
}
bool CheckPos(DNodeList &L, int pos){
return pos > 0 || pos <= GetLen(L) + 1;
}
int GetLen(DNodeList &L){
int len = 0;
DNodeList cur = L;
while(cur->next != NULL){
len ++;
cur = cur->next;
}
return len;
}
bool Destroy(DNodeList &L){
DNodeList cur = L;
while(cur != NULL){
if(Delete(cur, 1) == NULL) return false;
cur = cur->next;
}
L = NULL;
free(L);
return true;
}
void Print(DNodeList &L){
printf("\n");
DNodeList cur = L;
while(cur->next != NULL){
cur = cur->next;
printf("%d ", cur->data);
}
printf("\n");
}
int main()
{
DNodeList L = Create(0);
if (L == NULL) printf("创建失败");
else printf("创建成功");
for(int i = 0;i < 10; i++){
Insert(L, i + 1, i + 1);
}
Print(L);
DNodeList del = Delete(L, 3);
if (del == NULL) printf("删除位置错误");
else {
printf("删除元素成功");
Print(L);
}
int e = 4, pos = SearchByData(L, e);
if (pos != -1) printf("元素%d位于:%d", e, pos);
else printf("未查找到元素%d", e);
printf("\n");
if (Destroy(L)) printf("已销毁");
else {
printf("销毁失败");
Print(L);
}
return 0;
}
3.效果
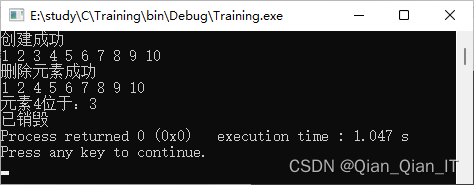