public class Animal {
public static void main(String[] args) {
Cat c = new Cat("花色的","波斯猫");
Dog d = new Dog("黑色的","藏獒");
System.out.print(c.getColor()+c.getBreed());
c.eat();
System.out.print(c.getColor()+c.getBreed());
c.catchMouse();
System.out.print(d.getColor()+d.getBreed());
d.eat();
System.out.print(d.getColor()+d.getBreed());
d.lookHome();
System.out.println("---------------------------");
c.setBreed("野猫");
c.setColor("白色的");
d.setBreed("哈士奇");
d.setColor("黑白的");
System.out.print(c.getColor()+c.getBreed());
c.eat();
System.out.print(c.getColor()+c.getBreed());
c.catchMouse();
System.out.print(d.getColor()+d.getBreed());
d.eat();
System.out.print(d.getColor()+d.getBreed());
d.lookHome();
}
}
public class Cat {
private String color;
private String breed;
public Cat() {
}
public Cat(String color, String breed) {
this.color = color;
this.breed = breed;
}
public void setColor(String color) {
this.color = color;
}
public void setBreed(String breed) {
this.breed = breed;
}
public String getColor() {
return color;
}
public String getBreed() {
return breed;
}
public void eat() {
System.out.println("猫正在吃鱼.....");
}
public void catchMouse() {
System.out.println("正在逮老鼠....");
}
}
public class Dog {
private String color;
private String breed;
public Dog() {
}
public Dog(String color, String breed) {
this.color = color;
this.breed = breed;
}
public void setColor(String color) {
this.color = color;
}
public void setBreed(String breed) {
this.breed = breed;
}
public String getColor() {
return color;
}
public String getBreed() {
return breed;
}
public void eat(){
System.out.println("正在啃骨头.....");
}
public void lookHome(){
System.out.println("正在看家.....");
}
}
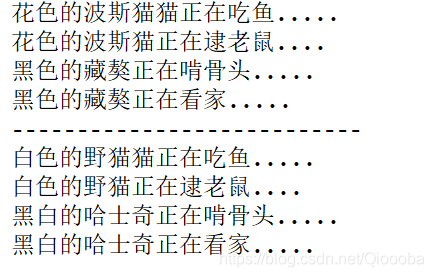