一、上传
1、上传多个文件(自动上传)
情况一:正常上传,没有限制
<el-upload
class="upload-demo"
action="#"
ref="upload"
multiple
:file-list="fileList"
:on-change="
(file, fileList) => {
change(file, fileList, index);
}
"
:auto-upload="false"
:http-request="httprequest"
:on-remove="remove"
accept=".xlsx,.xls,.jpg,.jpeg,.gif,.png,.pdf,.doc,.docx"
>
<el-button
slot="trigger"
icon="el-icon-upload2"
style="width: 100%; text-align: left"
size="small"
class="dashedbtn"
>上传文件</el-button
>
</el-upload>
<script>
import { UploadData } from "@/api/Equipment";
export default {
data() {
return {
tableFormrules: [],
tableForm: {},
ta: [],
fileList: [], //文件列表
formData: new FormData(),
up: [],
List:[]
};
},
methods: {
// 导入
remove(file, fileList) {
this.fileList = fileList;
this.List = this.fileList.map((item, index) => {
return item.FileUrl;
});
},
change(file, fileList, index) {
this.fileList = []; // 清空图片已经上传的图片列表(绑定在上传组件的file-list)
let a = 0;
fileList.forEach((item, idx) => {
/*在此处,对比文件名,将文件名相同的对比次数累加,
相同的文件名累加值为 2 时,说明文件名已经重复,直接删掉。*/
if (file.name === item.name) {
a++;
// console.log(a, file, fileList);
if (a === 2) {
this.$mess.info("不能重复上传,已把重复文件删除");
fileList.splice(idx, 1);
}
}
});
this.fileList = fileList;
if (a !== 2) {
this.httprequest(file, index);
}
},
async httprequest(file, index) {
// 一个一个上传
var formData = new FormData();
formData.append("files", file.raw);
const res = await UploadData(formData);
// console.log(res, "123");
if (res.code === 200) {
const a = [];
a.push(res.data[0].FileUrl);
//赋值
this.List = a;
this.$mess.success("上传成功"); //这个是封装的,无论调用多少次,都只显示一次
}
},
},
};
</script>
情况二:上传前判断文件大小,超过100则不上传
<el-upload
class="upload-demo"
ref="upload"
action
multiple
:file-list="upList"
:show-file-list="false"
:on-change="changeA"
accept=".png,.jpg,.pdf,.docx,.xlsx,.opju,.Dwg,.dxf,.dws,.dwt"
>
<el-button
slot="trigger"
type="text"
size="small"
>
<img src="@/assets/upload.png" width="25px" />
</el-button>
</el-upload>
changeA(file, fileList) {
const issize = file.file.size / 1024 / 1024 > 100;
if (issize) {
this.uploadNum++;
// this.$message.error(file.file.name + "文件超过100M,请重新上传。");
} else {
this.http(file);
this.upList.unshift(file);
}
},
async http(file) {
this.treeloading = true;//加载中提示,是加在表单中的
var formData = new FormData();
formData.append("files", file.file);
formData.append("storageAddress", this.pas); //携带其他参数
const res = await uploadFileDev(formData);
if (res.code === 200) {
// this.$mess.success("上传成功"); //这个是封装的,无论调用多少次,都只显示一次
if (res.data.length > 0) {
this.treeData.path = res.data[0].fileDownloadPath;
this.treeData.lable = res.data[0].fileName;
}
const req = await AddBpmFile({
id: "",
dataid: this.Form.dataid,
node_uuid: this.List.node_uuid,
userid: this.userid,
username: this.name,
folderid: this.folderid,
filename: this.treeData.lable,
path: this.treeData.path,
});
if (req.code === 200) {
this.ind++;//是否是所有文件都上传成功了,是,则调用接口刷新页面
if (this.ind === this.upList.length) {
this.$emit("GetFromData", this.Form.dataid);
if (this.uploadNum > 0) {
this.$mess.success(
"添加附件成功,但本次上传有" +
this.uploadNum +
"个文件大小超过100M,已取消上传"
);
} else {
this.$mess.success("添加附件成功");
}
this.ind = 0;
this.upList = [];
this.uploadNum = 0;
this.treeloading = false;
this.$forceUpdate();
}
} else {
this.treeloading = false;
this.$mess.error(req.data);
}
} else {
this.treeloading = false;
this.$mess.error(res.msg);
}
},
2、上传多个文件(手动上传)
<el-upload
class="upload-demo"
action="#"
ref="upload"
multiple
:auto-upload="false"
:file-list="fileList"
:on-change="
(file, fileList) => {
change(file, fileList);
}
"
:on-remove="remove"
>
<div style="display: flex">
<el-button slot="trigger" size="small" class="addbtn">上传文件</el-button>
<div
slot="tip"
class="el-upload__tip"
style="margin: auto 0; margin-left: 10px; color: #c0c4cc"
>
文件扩展名:.rar .zip .doc .docx .pdf .ipg...
</div>
</div>
</el-upload>
<el-button slot="trigger" size="small" class="addsub">保存</el-button >
<script>
import { UploadData } from "@/api/Equipment";
export default {
data() {
return {
form: {
equipmentid: 0, //设备id
filelist: [], //设备文件集合
},
fileList: [], //文件列表
formData: new FormData(),
};
},
methods: {
// 保存
async addsub() {
if (this.fileList.length > 0) {
this.http();
}
//其他操作
},
remove(file, fileList) {
this.fileList = fileList;
this.form.filelist = [];
this.fileList.forEach((item, index) => {
this.form.filelist.push({
filename: item.name,
fileurl: item.url,
});
});
// console.log(this.fileList, this.form.filelist)
},
change(file, fileList) {
this.fileList = []; // 清空图片已经上传的图片列表(绑定在上传组件的file-list)
let a = 0;
fileList.forEach((item, idx) => {
if (file.name === item.name) {
a++;
if (a === 2) {
this.$mess.info("不能重复上传,已把重复文件删除");
fileList.splice(idx, 1);
}
}
});
this.fileList = fileList;
},
// 自定义上传
async http(fileList) {
this.fileList.forEach((file) => {
this.formData.append("files", file.raw);
});
const res = await UploadData(this.formData);
// console.log(res, "123");
if (res.code === 200) {
res.data.forEach((item, index) => {
this.form.filelist.push({
filename: item.FileName,
fileurl: item.FileUrl,
});
this.fileList.push({
name: item.FileName,
url: item.FileUrl,
});
});
this.$message.success("上传成功");
}
},
},
},
};
</script>
3、上传文件前先判断条件,再上传
需求:判断是否选中,如果没有选中则提示并不出现弹窗(我这里做的是树状单选)
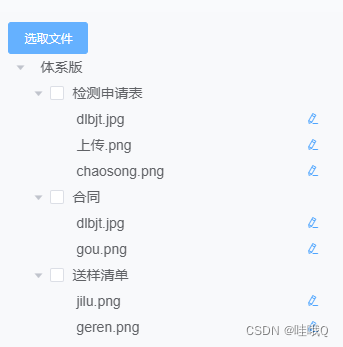
第一步,给上传的按钮添加事件
<el-upload
class="upload-demo"
ref="upload"
action=""
:show-file-list="false"
:limit="1"
:http-request="http"
>
<el-button
slot="trigger"
size="small"
type="primary"
@click="before"
>选取文件</el-button
>
</el-upload>
第二步:写js
methods: {
before(e) {
if (!this.folderid) {
this.$mess.error("请选择所需上传文件夹");
e.stopPropagation(); // 阻止事件冒泡到父元素
}
},
}
4、上传文件的同时携带其他参数
其他参数还是使用formData.append的方法添加,然后和文件一起传给后端
storageAddress就是后端的字段
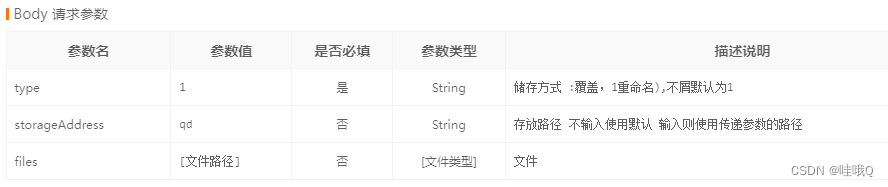
async httpA(file) {
var formDataA = new FormData();
formDataA.append("files", file.file);
let nu = this.pas + file.file.name;
formData.append("storageAddress", nu); //携带其他参数
const res = await uploadFileDev(formData);
}
二、导出文件
-
下载文件(可能是文件也可能是图片)
调用后端的接口,接口返回的是文件流
先在接口处添加responseType: "blob",
// 通用文件下载
export function download(params) {
return requestDEV({
url: "/dev/file/download",
method: "get",
responseType: "blob",
params,
});
}
然后再页面使用
async nodeClick(data, node, item) {
if (node.data.id) {
const res = await download({ id: node.data.id });//接口
const blob = new Blob([res]);
const elink = document.createElement("a");
elink.download = node.data.fileName;//文件名
elink.style.display = "none";
elink.href = URL.createObjectURL(blob);
document.body.appendChild(elink);
elink.click();
URL.revokeObjectURL(elink.href);
document.body.removeChild(elink);
}
},
2、导出xlsx(这里直接调用接口获取文件地址,然后下载)
// 导出
AccountMb(data) {
// window.open('/excel-mb.xlsx')
const link = document.createElement("a");
link.style.display = "none";
link.href = data; //下载地址
const fileName = "设备.xlsx";//文件名称
link.setAttribute("download", fileName);
document.body.appendChild(link);
link.click();
},
//导出按钮
async daochu() {
const res = await ExportEquipment();
this.AccountMb(res.data);
},
3、导出word文档(点击对应按钮,拿到地址,然后下载)
Bpm(command) {
//command.command_txt是"{url}/bpm_api/Task/GetContractFile?dataid={dataid}"
const a = command.command_txt.replace(/{dataid}/, this.Form.dataid);
const b = a.replace(/{url}/, "http://192.168.4.213:8011");
window.open(b, "_blank"); //相当于是打开新页面,然后直接下载
//window.location.href = b;//这也可以直接下载
},
或者是另一种方法(此方法如果不成功,需要在request.js文件对返回的状态码进行处理,下面有写)
// 自定义按钮---很多功能
Bpm(command) {
const a = command.command_txt.replace(/{dataid}/, this.Form.dataid);
const b = a.replace(/{url}/, "");
const c = b.split("?");
BPMALL(c[0], "get", { dataid: this.Form.dataid }, "blob").then((res) => {
// 这里是下载文件的方法
if (!res.code) {
const blob = new Blob([res], {
type: "application/vnd.openxmlformats-officedocument.wordprocessingml.document",
});
var fileName = command.name;
const elink = document.createElement("a");
elink.download = fileName;
elink.style.display = "none";
elink.href = URL.createObjectURL(blob);
document.body.appendChild(elink);
elink.click();
URL.revokeObjectURL(elink.href);
document.body.removeChild(elink);
} else {
// 其他方法
console.log(res);
}
});
},
src/api/Bpm.js
import requestBpm from "@/utils/request_bpm";
// 自定义按钮,根据后端返回的动态地址进行调用接口
export function BPMALL(url, method, params, responseType) {
return requestBpm({
url,
method,
responseType,
params,
});
}
src/utils/request_bpm.js
import axios from "axios";
import { MessageBox, Message } from "element-ui";
import store from "@/store";
import { getToken } from "@/utils/auth";
// import qs from "qs";
// axios.defaults.headers.get["Content-Type"] = "multipart/form-data";
// axios.defaults.headers.get["Content-Type"] = "application/json";
// create an axios instance
const service2 = axios.create({
baseURL: process.env.VUE_APP_BASE_API_BPM, // url = base url + request url
// withCredentials: true, // send cookies when cross-domain requests
timeout: 50000, // request timeout
});
// request interceptor
service2.interceptors.request.use(
(config) => {
// do something before request is sent
// if (config.method == "get") {
// config.data = qs.stringify(config.data);
// }
if (store.getters.token) {
// let each request carry token
// ['X-Token'] is a custom headers key
// please modify it according to the actual situation
config.headers["token"] = getToken();
}
return config;
},
(error) => {
// do something with request error
console.log(error); // for debug
return Promise.reject(error);
}
);
// response interceptor
service2.interceptors.response.use(
/**
* If you want to get http information such as headers or status
* Please return response => response
*/
/**
* Determine the request status by custom code
* Here is just an example
* You can also judge the status by HTTP Status Code
*/
(response) => {
const res = response.data;
// if the custom code is not 20000, it is judged as an error.
// 这是给下载文件流的,因为后端返回的没有code,直接是二进制
if (!res.code) {
return res;
}
if (res.code !== 200) {
Message({
message: res.msg || "Error",
type: "error",
duration: 5 * 1000,
});
// 50008: Illegal token; 50012: Other clients logged in; 50014: Token expired;
if (res.code === 50008 || res.code === 50012 || res.code === 50014) {
// to re-login
MessageBox.confirm(
"You have been logged out, you can cancel to stay on this page, or log in again",
"Confirm logout",
{
confirmButtonText: "Re-Login",
cancelButtonText: "Cancel",
type: "warning",
}
).then(() => {
store.dispatch("user/resetToken").then(() => {
location.reload();
});
});
}
return Promise.reject(new Error(res.msg || "Error"));
} else {
return res;
}
},
(error) => {
console.log("err" + error); // for debug
Message({
message: error.msg,
type: "error",
duration: 5 * 1000,
});
return Promise.reject(error);
}
);
export default service2;
补充说明type的填写类型
.doc application/msword
.docx application/vnd.openxmlformats-officedocument.wordprocessingml.document
.rtf application/rtf
.xls application/vnd.ms-excel application/x-excel
.xlsx application/vnd.openxmlformats-officedocument.spreadsheetml.sheet
.ppt application/vnd.ms-powerpoint
.pptx application/vnd.openxmlformats-officedocument.presentationml.presentation
.pps application/vnd.ms-powerpoint
.ppsx application/vnd.openxmlformats-officedocument.presentationml.slideshow
.pdf application/pdf
.swf application/x-shockwave-flash
.dll application/x-msdownload
.exe application/octet-stream
.msi application/octet-stream
.chm application/octet-stream
.cab application/octet-stream
.ocx application/octet-stream
.rar application/octet-stream
.tar application/x-tar
.tgz application/x-compressed
.zip application/x-zip-compressed
.z application/x-compress
.wav audio/wav
.wma audio/x-ms-wma
.wmv video/x-ms-wmv
.mp3 .mp2 .mpe .mpeg .mpg audio/mpeg
.rm application/vnd.rn-realmedia
.mid .midi .rmi audio/mid
.bmp image/bmp
.gif image/gif
.png image/png
.tif .tiff image/tiff
.jpe .jpeg .jpg image/jpeg
.txt text/plain
.xml text/xml
.html text/html
.css text/css
.js text/javascript
.mht .mhtml message/rfc822
".*"="application/octet-stream"
".001"="application/x-001"
".301"="application/x-301"
".323"="text/h323"
".906"="application/x-906"
".907"="drawing/907"
".a11"="application/x-a11"
".acp"="audio/x-mei-aac"
".ai"="application/postscript"
".aif"="audio/aiff"
".aifc"="audio/aiff"
".aiff"="audio/aiff"
".anv"="application/x-anv"
".asa"="text/asa"
".asf"="video/x-ms-asf"
".asp"="text/asp"
".asx"="video/x-ms-asf"
".au"="audio/basic"
".avi"="video/avi"
".awf"="application/vnd.adobe.workflow"
".biz"="text/xml"
".bmp"="application/x-bmp"
".bot"="application/x-bot"
".c4t"="application/x-c4t"
".c90"="application/x-c90"
".cal"="application/x-cals"
".cat"="application/vnd.ms-pki.seccat"
".cdf"="application/x-netcdf"
".cdr"="application/x-cdr"
".cel"="application/x-cel"
".cer"="application/x-x509-ca-cert"
".cg4"="application/x-g4"
".cgm"="application/x-cgm"
".cit"="application/x-cit"
responseType的类型
"" responseType 设为空字符串与设置为"text"相同,默认类型
"text" 返回的是包含在 DOMString 对象中的文本。
"document" 返回的是一个 HTML Document 或 XML XMLDocument
"arraybuffer" 返回的是一个包含二进制数据的 JavaScript ArrayBuffer
"blob" 返回的是一个包含二进制数据的 Blob 对象
"json" 返回的是一个 JavaScript 对象 。这个对象是通过将接收到的数据类型视为 JSON 解析得到的。
"ms-stream" 返回的是下载流的一部分 ;此响应类型仅允许下载请求,并且仅受Internet Explorer支持
这篇文章看完如果您觉得有所收获,认为还行的话,就点个赞收藏一下呗
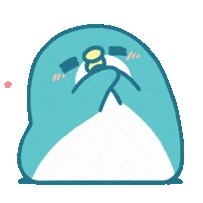